
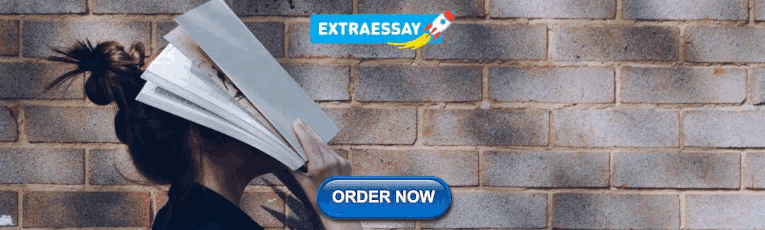
Python List: How To Create, Sort, Append, Remove, And More
The Python list is one of the most used data structures, together with dictionaries . The Python list is not just a list, but can also be used as a stack and even a queue. In this article, I’ll explain everything you might possibly want to know about Python lists:
- how to create lists,
- modify them,
- how to sort lists,
- loop over elements of a list with a for-loop or a list comprehension ,
- how to slice a list,
- append to Python lists,
- … and more!
I’ve included lots of working code examples to demonstrate.
Table of Contents
- 1 How to create a Python list
- 2 Accessing Python list elements
- 3 Adding and removing elements
- 4 How to get List length in Python
- 5 Counting element occurrence in a list
- 6 Check if an item is in a list
- 7 Find the index of an item in a list
- 8 Loop over list elements
- 9 Python list to string
- 10 Sorting Python lists
- 12 Reversing Python lists
- 13 Learn more about Python lists
How to create a Python list
Let’s start by creating a list:
Lists contain regular Python objects, separated by commas and surrounded by brackets. The elements in a list can have any data type , and they can be mixed. You can even create a list of lists. The following lists are all valid:
Using the list() function
Python lists, like all Python data types, are objects. The class of a list is called ‘list’, with a lower case L. If you want to convert another Python object to a list, you can use the list() function, which is actually the constructor of the list class itself. This function takes one argument: an object that is iterable.
So you can convert anything that is iterable into a list. E.g., you can materialize the range function into a list of actual values, or convert a Python set or tuple into a list:
Accessing Python list elements
To access an individual list element, you need to know the position of that element. Since computers start counting at 0, the first element is in position 0, the second element is in position 1, etcetera.
Here are a few examples:
As you can see, you can’t access elements that don’t exist. In this case, Python throws an IndexError exception , with the explanation ‘list index out of range’. In my article on exceptions and the try and except statements , I’ve written about this subject more in-depth in the section on best practices. I recommend you to read it.
Get the last element of a list
If you want to get elements from the end of the list, you can supply a negative value. In this case, we start counting at -1 instead of 0. E.g., to get the last element of a list, you can do this:
Accessing nested list elements
Accessing nested list elements is not that much different. When you access an element that is a list, that list is returned. So to request an element in that list, you need to again use a couple of brackets:
Adding and removing elements
Let’s see how we can add and remove data. There are several ways to remove data from a list. What you use, depends on the situation you’re in. I’ll describe and demonstrate them all in this section.
Append to a Python list
List objects have a number of useful built-in methods, one of which is the append method. When calling append on a list, we append an object to the end of the list:
Combine or merge two lists
Another way of adding elements is adding all the elements from one list to the other. There are two ways to combine lists:
- ‘Add’ them together with the + operator.
- Add all elements of one list to the other with the extend method.
Here’s how you can add two lists together. The result is a new, third list:
The original lists are kept intact. The alternative is to extend one list with another, using the extend method:
While l1 got extended with the elements of l2, l2 stayed the same. Extend appended all values from l2 to l1.
Pop items from a list
The pop() method removes and returns the last item by default unless you give it an index argument.
Here are a couple of examples that demonstrate both the default behavior and the behavior when given an index:
If you’re familiar with the concept of a stack , you can now build one using only the append and pop methods on a list!
Using del() to delete items
There are multiple ways to delete or remove items from a list. While pop returns the item that is deleted from the list, del removes it without returning anything. In fact, you can delete any object, including the entire list, using del:
Remove specific values from a Python list
If you want to remove a specific value instead, you use the remove method. E.g. if you want to remove the first occurrence of the number 2 in a list, you can do so as follows:
As you can see, repeated calls to remove will remove additional twos, until there are none left, in which case Python throws a ValueError exception .
Remove or clear all items from a Python list
To remove all items from a list, use the clear() method:
Remove duplicates from a list
There is no special function or method to remove duplicates from a list, but there are multiple tricks that we can use to do so anyway. The simplest, in my opinion, is using a Python set . Sets are collections of objects, like lists, but can only contain one of each element. More formally, sets are unordered collections of distinct objects.
By converting the list to a set and then back to a list again, we’ve effectively removed all duplicates:
In your own code, you probably want to use this more compact version:
Since sets are very similar to lists, you may not even have to convert them back into a list. If the set offers what you need, use it instead to prevent a double conversion, making your program a little bit faster and more efficient.
Replace items in a list
To replace list items, we assign a new value to a given list index, like so:
How to get List length in Python
In Python, we use the len function to get the length of objects. This is true for lists as well:
If you’re familiar with other programming languages, like Java, you might wonder why Python has a function for this. After all, it could have been one of the built-in methods of a list too, like my_list.len() . This is because, in other languages, this often results in various ways to get an object’s length. E.g., some will call this function len , others will call it length, and yet someone else won’t even implement the function but simply offer a public member variable. And this is exactly the reason why Python chose to standardize the naming of such a common operation!
Counting element occurrence in a list
Don’t confuse the count function with getting the list length, it’s totally different. The built-in count function counts occurrences of a particular value inside that list. Here’s an example:
Since the number 1 occurs three times in the list, my_list.count(1) returned 3.
Check if an item is in a list
To check if an item is in a list, use the following syntax:
Find the index of an item in a list
We can find where an item is inside a list with the index method. For example, in the following list the 4 is located at position 3 (remember that we start counting at zero):
The index method takes two optional parameters: start and stop. With these, we can continue looking for more of the same values. We don’t need to supply an end value if we supply a start value. Now, let’s find both 4’s in the list below:
If you want to do more advanced filtering of lists, you should read my article on list comprehensions .
Loop over list elements
A list is iterable , so we can use a for-loop over the elements of a list just like we can with any other iterable with the ‘for <element> in <iterable>’ syntax:
Python list to string
In Python, you can convert most objects to a string with the str function:
If you’re interested, str is actually the Python string ‘s base class and calling str() constructs a new str object by calling the constructor of the str class. This constructor inspects the provided object and looks for a special method (also called dunder method) called __str__ . If it is present, this method is called. There’s nothing more to it.
If you create your own classes and objects , you can implement the __str__ function yourself to offer a printable version of your objects.
Sorting Python lists
To sort a Python list, we have two options:
- Use the built-in sort method of the list itself.
- Use Python’s built-in sorted() function.
Option one, the built-in method, offers an in-place sort, which means the list itself is modified. In other words, this function does not return anything. Instead, it modifies the list itself.
Option two returns a new list, leaving the original intact. Which one you use depends on the situation you’re in.
In-place list sort in ascending order
Let’s start with the simplest use-case: sorting in ascending order:
In-place list sort in descending order
We can call the sort method with a reverse parameter. If this is set to True, sort reverses the order:
Using sorted()
The following example demonstrates how to sort lists in ascending order, returning a new list with the result:
As you can see from the last statement, the original list is unchanged. Let’s do that again, but now in descending order:
Unsortable lists
We can not sort all lists, since Python can not compare all types with each other. For example, we can sort a list of numbers, like integers and floats, because they have a defined order. We can sort a list of strings as well since Python is able to compare strings too.
However, lists can contain any type of object, and we can’t compare completely different objects, like numbers and strings, to each other. In such cases, Python throws a TypeError :
Although the error might look a bit cryptic, it’s only logical when you know what’s going on. To sort a list, Python needs to compare the objects to each other. So in its sorting algorithm, at some point, it checks if ‘a’ < 1, hence the error: '<' not supported between instances of 'str' and 'int' .
Sometimes you need to get parts of a list. Python has a powerful syntax to do so, called slicing, and it makes working with lists a lot easier compared to some other programming languages. Slicing works on Python lists, but it also works on any other sequence type like strings , tuples , and ranges .
The slicing syntax is as follows:
my_list[start:stop:step]
A couple of notes:
- start is the first element position to include
- stop is exclusive, meaning that the element at position stop won’t be included.
- step is the step size. more on this later.
- start , stop , and step are all optional.
- Negative values can be used too.
To explain how slicing works, it’s best to just look at examples, and try for yourself, so that’s what we’ll do:
The step value
The step value is 1 by default. If you increase it, you can step over elements. Let’s try this:
Going backward
Like with list indexing, we can also supply negative numbers with slicing. Here’s a little ASCII art to show you how counting backward in a list works:
Just remember that you need to set a negative step size in order to go backward:
Reversing Python lists
There are actually three methods you can use to reverse a list in Python:
- An in-place reverse, using the built-in reverse method that every list has natively
- Using list slicing with a negative step size results in a new list
- Create a reverse iterator , with the reversed() function
In the following code crumb, I demonstrate all three. They are explained in detail in the following sections:
Using the built-in reverse method
The list.reverse() method does an in-place reverse, meaning it reorders the list. In other words, the method does not return a new list object, with a reversed order. Here’s how to use reverse() :
Reverse a list with list slicing
Although you can reverse a list with the list.reverse() method that every list has, you can do it with list slicing too, using a negative step size of -1. The difference here is that list slicing results in a new, second list. It keeps the original list intact:
Creating a reverse iterator
Finally, you can use the reversed() built-in function, which creates an iterator that returns all elements of the given iterable (our list) in reverse. This method is quite cheap in terms of CPU and memory usage. All it needs to do is walk backward over the iterable object. It’s doesn’t need to move around data and it doesn’t need to reserve extra memory for a second list. So if you need to iterate over a (large) list in reverse, this should be your choice.
Here’s how you can use this function. Keep in mind, that you can only use the iterator once, but that it’s cheap to make a new one:
Learn more about Python lists
Because there’s a lot to tell about list comprehensions, I created a dedicated article for the topic. A Python list comprehension is a language construct that we use to create a list based on an existing list, without creating for-loops .
Some other resources you might like:
- The official Python docs about lists.
- If you are interested in the internals: lists are often implemented internally as a linked list .
- Python also has arrays , which are very similar and the term will be familiar to people coming from other programming languages. They are more efficient at storing data, but they can only store one type of data.
Get certified with our courses
Learn Python properly through small, easy-to-digest lessons, progress tracking, quizzes to test your knowledge, and practice sessions. Each course will earn you a downloadable course certificate.
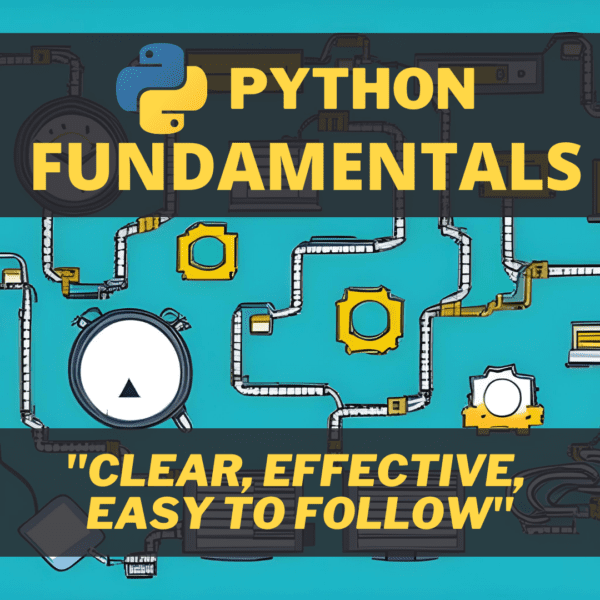
Related articles
- Python List Comprehension: Tutorial With Examples
- Python For Loop and While Loop
- Python Tuple: How to Create, Use, and Convert
- Pip Install: How To Install and Remove Python Packages
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Get Started With Python
- Your First Python Program
- Python Comments
Python Fundamentals
- Python Variables and Literals
- Python Type Conversion
- Python Basic Input and Output
- Python Operators
Python Flow Control
- Python if...else Statement
- Python for Loop
- Python while Loop
- Python break and continue
- Python pass Statement
Python Data types
- Python Numbers and Mathematics
Python List
Python Tuple
- Python String
- Python Dictionary
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files
- Reading CSV files in Python
- Writing CSV files in Python
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python
- Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
- Precedence and Associativity of Operators in Python
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs
Python Tutorials
Python List pop()
Python List extend()
Python List insert()
- Python List append()
- Python List index()
- Python List remove()
There are many built-in types in Python that allow us to group and store multiple items. Python lists are the most versatile among them.
For example, we can use a Python list to store a playlist of songs so that we can easily add, remove, and update songs as needed.
- Create a Python List
We create a list by placing elements inside square brackets [] , separated by commas. For example,
Here, the ages list has three items.
We can store data of different data types in a Python list. For example,
We can use the built-in list() function to convert other iterables (strings, dictionaries, tuples, etc.) to a list.
List Characteristics
- Ordered - They maintain the order of elements.
- Mutable - Items can be changed after creation.
- Allow duplicates - They can contain duplicate values.
- Access List Elements
Each element in a list is associated with a number, known as an index .
The index of first item is 0 , the index of second item is 1 , and so on.

We use these index numbers to access list items. For example,

More on Accessing List Elements
Python also supports negative indexing. The index of the last element is -1 , the second-last element is -2 , and so on.
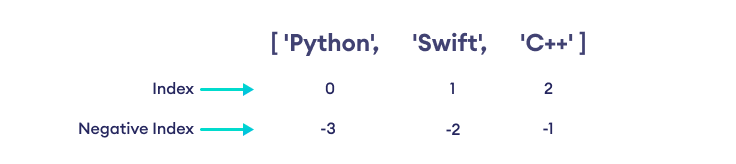
Negative indexing makes it easy to access list items from last.
Let's see an example,
In Python, it is possible to access a section of items from the list using the slicing operator : . For example,
To learn more about slicing, visit Python program to slice lists .
Note : If the specified index does not exist in a list, Python throws the IndexError exception.
- Add Elements to a Python List
We use the append() method to add an element to the end of a Python list. For example,
The insert() method adds an element at the specified index. For example,
We use the extend() method to add elements to a list from other iterables. For example,
- Change List Items
We can change the items of a list by assigning new values using the = operator. For example,
Here, we have replaced the element at index 2: 'Green' with 'Blue' .
- Remove an Item From a List
We can remove an item from a list using the remove() method. For example,
The del statement removes one or more items from a list. For example,
Note : We can also use the del statement to delete the entire list. For example,
- Python List Length
We can use the built-in len() function to find the number of elements in a list. For example,
- Iterating Through a List
We can use a for loop to iterate over the elements of a list. For example,
- Python List Methods
Python has many useful list methods that make it really easy to work with lists.
Method | Description |
---|---|
Adds an item to the end of the list | |
Adds items of lists and other iterables to the end of the list | |
Inserts an item at the specified index | |
Removes the specified value from the list | |
Returns and removes item present at the given index | |
Removes all items from the list | |
Returns the index of the first matched item | |
Returns the count of the specified item in the list | |
Sorts the list in ascending/descending order | |
Reverses the item of the list | |
Returns the shallow copy of the list |
More on Python Lists
List Comprehension is a concise and elegant way to create a list. For example,
To learn more, visit Python List Comprehension .
We use the in keyword to check if an item exists in the list. For example,
- orange is not present in fruits , so, 'orange' in fruits evaluates to False .
- cherry is present in fruits , so, 'cherry' in fruits evaluates to True .
Note: Lists are similar to arrays (or dynamic arrays) in other programming languages. When people refer to arrays in Python, they often mean lists, even though there is a numeric array type in Python.
- Python list()
Table of Contents
- Introduction
Write a function to find the largest number in a list.
- For input [1, 2, 9, 4, 5] , the return value should be 9 .
Video: Python Lists and Tuples
Sorry about that.
Related Tutorials
Python Library
Python Tutorial
- Google for Education
- Español – América Latina
- Português – Brasil
- Tiếng Việt
Python Lists
Python has a great built-in list type named "list". List literals are written within square brackets [ ]. Lists work similarly to strings -- use the len() function and square brackets [ ] to access data, with the first element at index 0. (See the official python.org list docs .)

Assignment with an = on lists does not make a copy. Instead, assignment makes the two variables point to the one list in memory.

The "empty list" is just an empty pair of brackets [ ]. The '+' works to append two lists, so [1, 2] + [3, 4] yields [1, 2, 3, 4] (this is just like + with strings).
Python's *for* and *in* constructs are extremely useful, and the first use of them we'll see is with lists. The *for* construct -- for var in list -- is an easy way to look at each element in a list (or other collection). Do not add or remove from the list during iteration.
If you know what sort of thing is in the list, use a variable name in the loop that captures that information such as "num", or "name", or "url". Since Python code does not have other syntax to remind you of types, your variable names are a key way for you to keep straight what is going on. (This is a little misleading. As you gain more exposure to python, you'll see references to type hints which allow you to add typing information to your function definitions. Python doesn't use these type hints when it runs your programs. They are used by other programs such as IDEs (integrated development environments) and static analysis tools like linters/type checkers to validate if your functions are called with compatible arguments.)
The *in* construct on its own is an easy way to test if an element appears in a list (or other collection) -- value in collection -- tests if the value is in the collection, returning True/False.
The for/in constructs are very commonly used in Python code and work on data types other than list, so you should just memorize their syntax. You may have habits from other languages where you start manually iterating over a collection, where in Python you should just use for/in.
You can also use for/in to work on a string. The string acts like a list of its chars, so for ch in s: print(ch) prints all the chars in a string.
The range(n) function yields the numbers 0, 1, ... n-1, and range(a, b) returns a, a+1, ... b-1 -- up to but not including the last number. The combination of the for-loop and the range() function allow you to build a traditional numeric for loop:
There is a variant xrange() which avoids the cost of building the whole list for performance sensitive cases (in Python 3, range() will have the good performance behavior and you can forget about xrange()).
Python also has the standard while-loop, and the *break* and *continue* statements work as in C++ and Java, altering the course of the innermost loop. The above for/in loops solves the common case of iterating over every element in a list, but the while loop gives you total control over the index numbers. Here's a while loop which accesses every 3rd element in a list:
List Methods
Here are some other common list methods.
- list.append(elem) -- adds a single element to the end of the list. Common error: does not return the new list, just modifies the original.
- list.insert(index, elem) -- inserts the element at the given index, shifting elements to the right.
- list.extend(list2) adds the elements in list2 to the end of the list. Using + or += on a list is similar to using extend().
- list.index(elem) -- searches for the given element from the start of the list and returns its index. Throws a ValueError if the element does not appear (use "in" to check without a ValueError).
- list.remove(elem) -- searches for the first instance of the given element and removes it (throws ValueError if not present)
- list.sort() -- sorts the list in place (does not return it). (The sorted() function shown later is preferred.)
- list.reverse() -- reverses the list in place (does not return it)
- list.pop(index) -- removes and returns the element at the given index. Returns the rightmost element if index is omitted (roughly the opposite of append()).
Notice that these are *methods* on a list object, while len() is a function that takes the list (or string or whatever) as an argument.
Common error: note that the above methods do not *return* the modified list, they just modify the original list.
List Build Up
One common pattern is to start a list as the empty list [], then use append() or extend() to add elements to it:
List Slices
Slices work on lists just as with strings, and can also be used to change sub-parts of the list.
Exercise: list1.py
To practice the material in this section, try the problems in list1.py that do not use sorting (in the Basic Exercises ).
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2024-07-23 UTC.
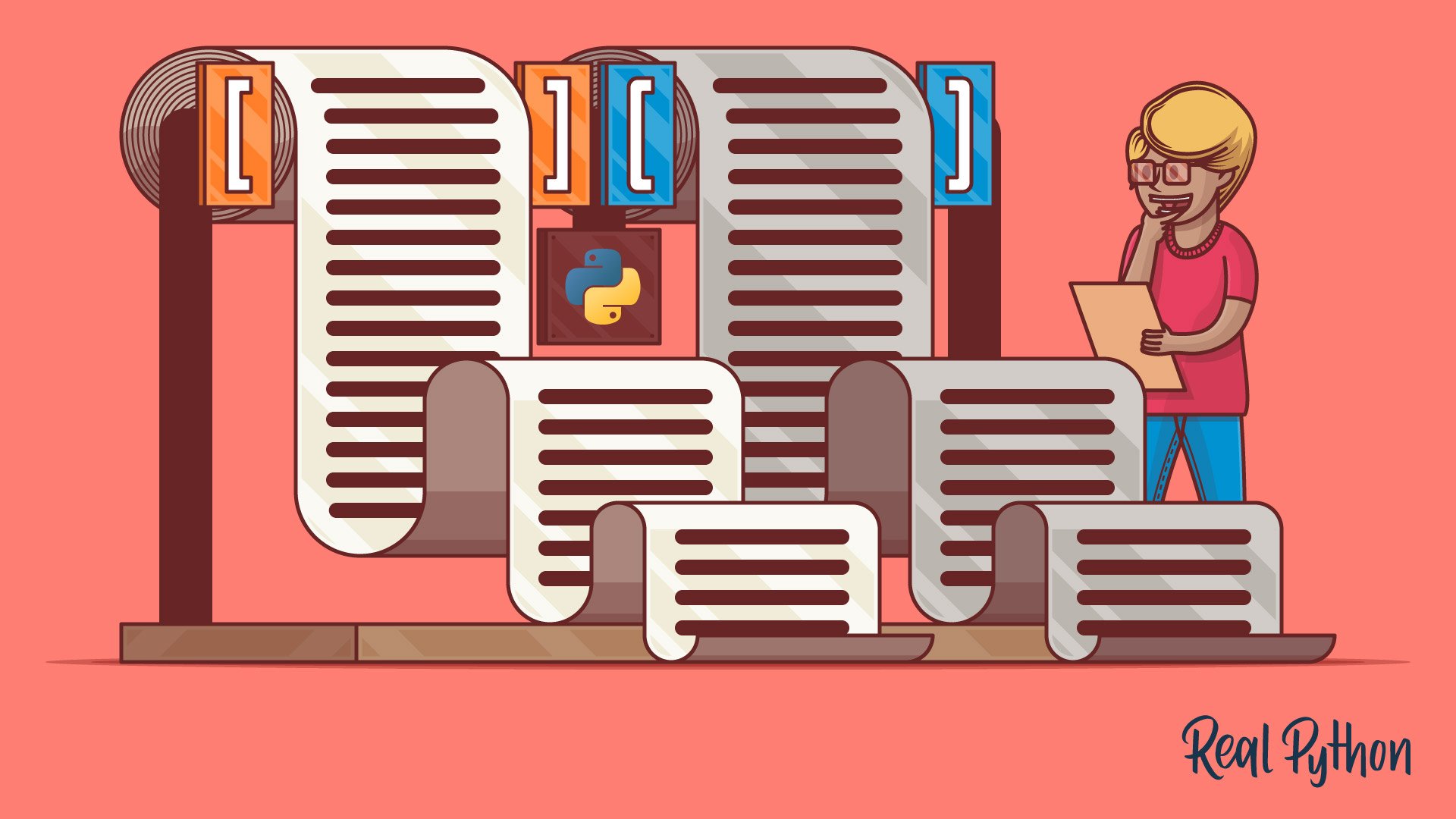
Lists and Tuples in Python
Table of Contents
Lists Are Ordered
Lists can contain arbitrary objects, list elements can be accessed by index, lists can be nested, lists are mutable, lists are dynamic, defining and using tuples, tuple assignment, packing, and unpacking.
Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: Lists and Tuples in Python
Lists and tuples are arguably Python’s most versatile, useful data types . You will find them in virtually every nontrivial Python program.
Here’s what you’ll learn in this tutorial: You’ll cover the important characteristics of lists and tuples. You’ll learn how to define them and how to manipulate them. When you’re finished, you should have a good feel for when and how to use these object types in a Python program.
Take the Quiz: Test your knowledge with our interactive “Python Lists and Tuples” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Test your understanding of Python lists and tuples.
Python Lists
In short, a list is a collection of arbitrary objects, somewhat akin to an array in many other programming languages but more flexible. Lists are defined in Python by enclosing a comma-separated sequence of objects in square brackets ( [] ), as shown below:
The important characteristics of Python lists are as follows:
- Lists are ordered.
- Lists can contain any arbitrary objects.
- List elements can be accessed by index.
- Lists can be nested to arbitrary depth.
- Lists are mutable.
- Lists are dynamic.
Each of these features is examined in more detail below.
A list is not merely a collection of objects. It is an ordered collection of objects. The order in which you specify the elements when you define a list is an innate characteristic of that list and is maintained for that list’s lifetime. (You will see a Python data type that is not ordered in the next tutorial on dictionaries.)
Lists that have the same elements in a different order are not the same:
A list can contain any assortment of objects. The elements of a list can all be the same type:
Or the elements can be of varying types:
Lists can even contain complex objects, like functions, classes, and modules, which you will learn about in upcoming tutorials:
A list can contain any number of objects, from zero to as many as your computer’s memory will allow:
(A list with a single object is sometimes referred to as a singleton list.)
List objects needn’t be unique. A given object can appear in a list multiple times:
Individual elements in a list can be accessed using an index in square brackets. This is exactly analogous to accessing individual characters in a string. List indexing is zero-based as it is with strings.
Consider the following list:
The indices for the elements in a are shown below:

Here is Python code to access some elements of a :
Virtually everything about string indexing works similarly for lists. For example, a negative list index counts from the end of the list:
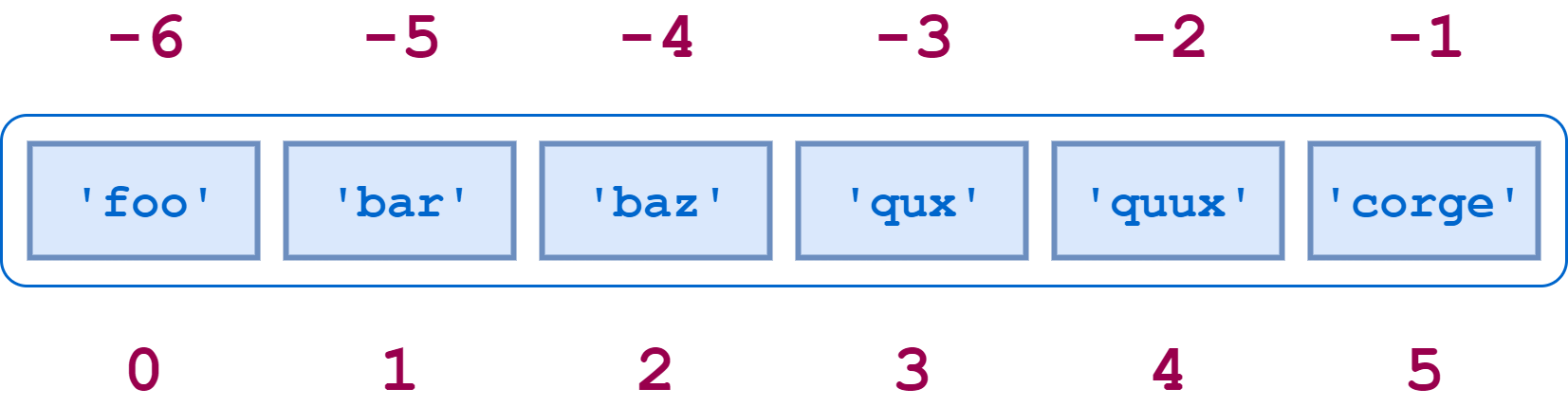
Slicing also works. If a is a list, the expression a[m:n] returns the portion of a from index m to, but not including, index n :
Other features of string slicing work analogously for list slicing as well:
Both positive and negative indices can be specified:
Omitting the first index starts the slice at the beginning of the list, and omitting the second index extends the slice to the end of the list:
You can specify a stride—either positive or negative:
The syntax for reversing a list works the same way it does for strings:
The [:] syntax works for lists. However, there is an important difference between how this operation works with a list and how it works with a string.
If s is a string, s[:] returns a reference to the same object:
Conversely, if a is a list, a[:] returns a new object that is a copy of a :
Several Python operators and built-in functions can also be used with lists in ways that are analogous to strings:
The in and not in operators:
The concatenation ( + ) and replication ( * ) operators:
The len() , min() , and max() functions:
It’s not an accident that strings and lists behave so similarly. They are both special cases of a more general object type called an iterable, which you will encounter in more detail in the upcoming tutorial on definite iteration.
By the way, in each example above, the list is always assigned to a variable before an operation is performed on it. But you can operate on a list literal as well:
For that matter, you can do likewise with a string literal:
You have seen that an element in a list can be any sort of object. That includes another list. A list can contain sublists, which in turn can contain sublists themselves, and so on to arbitrary depth.
Consider this (admittedly contrived) example:
The object structure that x references is diagrammed below:
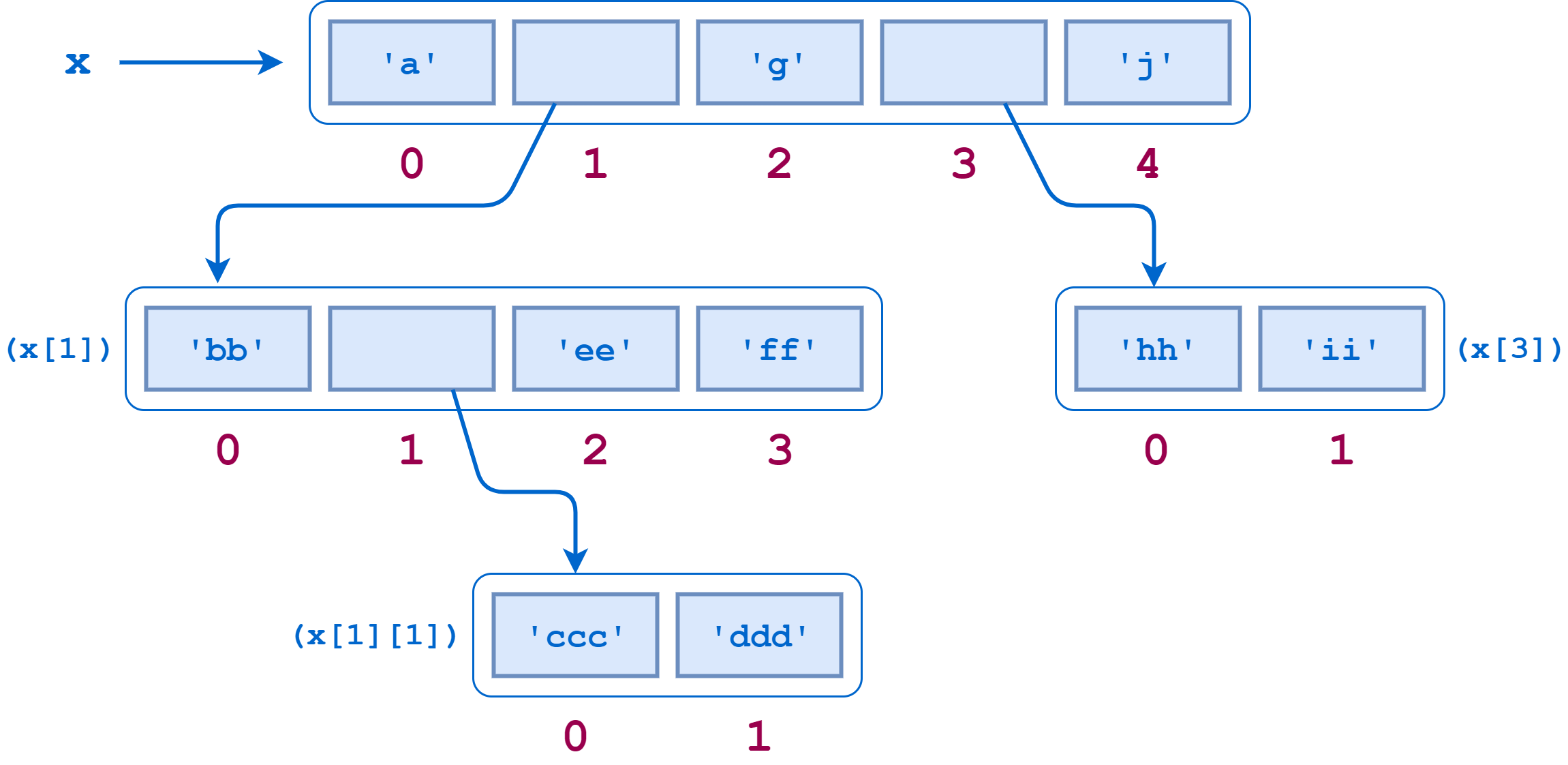
x[0] , x[2] , and x[4] are strings, each one character long:
But x[1] and x[3] are sublists:
To access the items in a sublist, simply append an additional index:
x[1][1] is yet another sublist, so adding one more index accesses its elements:
There is no limit, short of the extent of your computer’s memory, to the depth or complexity with which lists can be nested in this way.
All the usual syntax regarding indices and slicing applies to sublists as well:
However, be aware that operators and functions apply to only the list at the level you specify and are not recursive . Consider what happens when you query the length of x using len() :
x has only five elements—three strings and two sublists. The individual elements in the sublists don’t count toward x ’s length.
You’d encounter a similar situation when using the in operator:
'ddd' is not one of the elements in x or x[1] . It is only directly an element in the sublist x[1][1] . An individual element in a sublist does not count as an element of the parent list(s).
Most of the data types you have encountered so far have been atomic types. Integer or float objects, for example, are primitive units that can’t be further broken down. These types are immutable , meaning that they can’t be changed once they have been assigned. It doesn’t make much sense to think of changing the value of an integer. If you want a different integer, you just assign a different one.
By contrast, the string type is a composite type. Strings are reducible to smaller parts—the component characters. It might make sense to think of changing the characters in a string. But you can’t. In Python, strings are also immutable.
The list is the first mutable data type you have encountered. Once a list has been created, elements can be added, deleted, shifted, and moved around at will. Python provides a wide range of ways to modify lists.
Modifying a Single List Value
A single value in a list can be replaced by indexing and simple assignment:
You may recall from the tutorial Strings and Character Data in Python that you can’t do this with a string:
A list item can be deleted with the del command:
Modifying Multiple List Values
What if you want to change several contiguous elements in a list at one time? Python allows this with slice assignment , which has the following syntax:
Again, for the moment, think of an iterable as a list. This assignment replaces the specified slice of a with <iterable> :
The number of elements inserted need not be equal to the number replaced. Python just grows or shrinks the list as needed.
You can insert multiple elements in place of a single element—just use a slice that denotes only one element:
Note that this is not the same as replacing the single element with a list:
You can also insert elements into a list without removing anything. Simply specify a slice of the form [n:n] (a zero-length slice) at the desired index:
You can delete multiple elements out of the middle of a list by assigning the appropriate slice to an empty list. You can also use the del statement with the same slice:
Prepending or Appending Items to a List
Additional items can be added to the start or end of a list using the + concatenation operator or the += augmented assignment operator :
Note that a list must be concatenated with another list, so if you want to add only one element, you need to specify it as a singleton list:
Note: Technically, it isn’t quite correct to say a list must be concatenated with another list. More precisely, a list must be concatenated with an object that is iterable. Of course, lists are iterable, so it works to concatenate a list with another list.
Strings are iterable also. But watch what happens when you concatenate a string onto a list:
This result is perhaps not quite what you expected. When a string is iterated through, the result is a list of its component characters. In the above example, what gets concatenated onto list a is a list of the characters in the string 'corge' .
If you really want to add just the single string 'corge' to the end of the list, you need to specify it as a singleton list:
If this seems mysterious, don’t fret too much. You’ll learn about the ins and outs of iterables in the tutorial on definite iteration.
Methods That Modify a List
Finally, Python supplies several built-in methods that can be used to modify lists. Information on these methods is detailed below.
Note: The string methods you saw in the previous tutorial did not modify the target string directly. That is because strings are immutable. Instead, string methods return a new string object that is modified as directed by the method. They leave the original target string unchanged:
List methods are different. Because lists are mutable, the list methods shown here modify the target list in place.
a.append(<obj>)
Appends an object to a list.
a.append(<obj>) appends object <obj> to the end of list a :
Remember, list methods modify the target list in place. They do not return a new list:
Remember that when the + operator is used to concatenate to a list, if the target operand is an iterable, then its elements are broken out and appended to the list individually:
The .append() method does not work that way! If an iterable is appended to a list with .append() , it is added as a single object:
Thus, with .append() , you can append a string as a single entity:
a.extend(<iterable>)
Extends a list with the objects from an iterable.
Yes, this is probably what you think it is. .extend() also adds to the end of a list, but the argument is expected to be an iterable. The items in <iterable> are added individually:
In other words, .extend() behaves like the + operator. More precisely, since it modifies the list in place, it behaves like the += operator:
a.insert(<index>, <obj>)
Inserts an object into a list.
a.insert(<index>, <obj>) inserts object <obj> into list a at the specified <index> . Following the method call, a[<index>] is <obj> , and the remaining list elements are pushed to the right:
a.remove(<obj>)
Removes an object from a list.
a.remove(<obj>) removes object <obj> from list a . If <obj> isn’t in a , an exception is raised:
a.pop(index=-1)
Removes an element from a list.
This method differs from .remove() in two ways:
- You specify the index of the item to remove, rather than the object itself.
- The method returns a value: the item that was removed.
a.pop() simply removes the last item in the list:
If the optional <index> parameter is specified, the item at that index is removed and returned. <index> may be negative, as with string and list indexing:
<index> defaults to -1 , so a.pop(-1) is equivalent to a.pop() .
This tutorial began with a list of six defining characteristics of Python lists. The last one is that lists are dynamic. You have seen many examples of this in the sections above. When items are added to a list, it grows as needed:
Similarly, a list shrinks to accommodate the removal of items:
Python Tuples
Python provides another type that is an ordered collection of objects, called a tuple.
Pronunciation varies depending on whom you ask. Some pronounce it as though it were spelled “too-ple” (rhyming with “Mott the Hoople”), and others as though it were spelled “tup-ple” (rhyming with “supple”). My inclination is the latter, since it presumably derives from the same origin as “quintuple,” “sextuple,” “octuple,” and so on, and everyone I know pronounces these latter as though they rhymed with “supple.”
Tuples are identical to lists in all respects, except for the following properties:
- Tuples are defined by enclosing the elements in parentheses ( () ) instead of square brackets ( [] ).
- Tuples are immutable.
Here is a short example showing a tuple definition, indexing, and slicing:
Never fear! Our favorite string and list reversal mechanism works for tuples as well:
Note: Even though tuples are defined using parentheses, you still index and slice tuples using square brackets, just as for strings and lists.
Everything you’ve learned about lists—they are ordered, they can contain arbitrary objects, they can be indexed and sliced, they can be nested—is true of tuples as well. But they can’t be modified:
Why use a tuple instead of a list?
Program execution is faster when manipulating a tuple than it is for the equivalent list. (This is probably not going to be noticeable when the list or tuple is small.)
Sometimes you don’t want data to be modified. If the values in the collection are meant to remain constant for the life of the program, using a tuple instead of a list guards against accidental modification.
There is another Python data type that you will encounter shortly called a dictionary, which requires as one of its components a value that is of an immutable type. A tuple can be used for this purpose, whereas a list can’t be.
In a Python REPL session, you can display the values of several objects simultaneously by entering them directly at the >>> prompt, separated by commas:
Python displays the response in parentheses because it is implicitly interpreting the input as a tuple.
There is one peculiarity regarding tuple definition that you should be aware of. There is no ambiguity when defining an empty tuple, nor one with two or more elements. Python knows you are defining a tuple:
But what happens when you try to define a tuple with one item:
Doh! Since parentheses are also used to define operator precedence in expressions, Python evaluates the expression (2) as simply the integer 2 and creates an int object. To tell Python that you really want to define a singleton tuple, include a trailing comma ( , ) just before the closing parenthesis:
You probably won’t need to define a singleton tuple often, but there has to be a way.
When you display a singleton tuple, Python includes the comma, to remind you that it’s a tuple:
As you have already seen above, a literal tuple containing several items can be assigned to a single object:
When this occurs, it is as though the items in the tuple have been “packed” into the object:
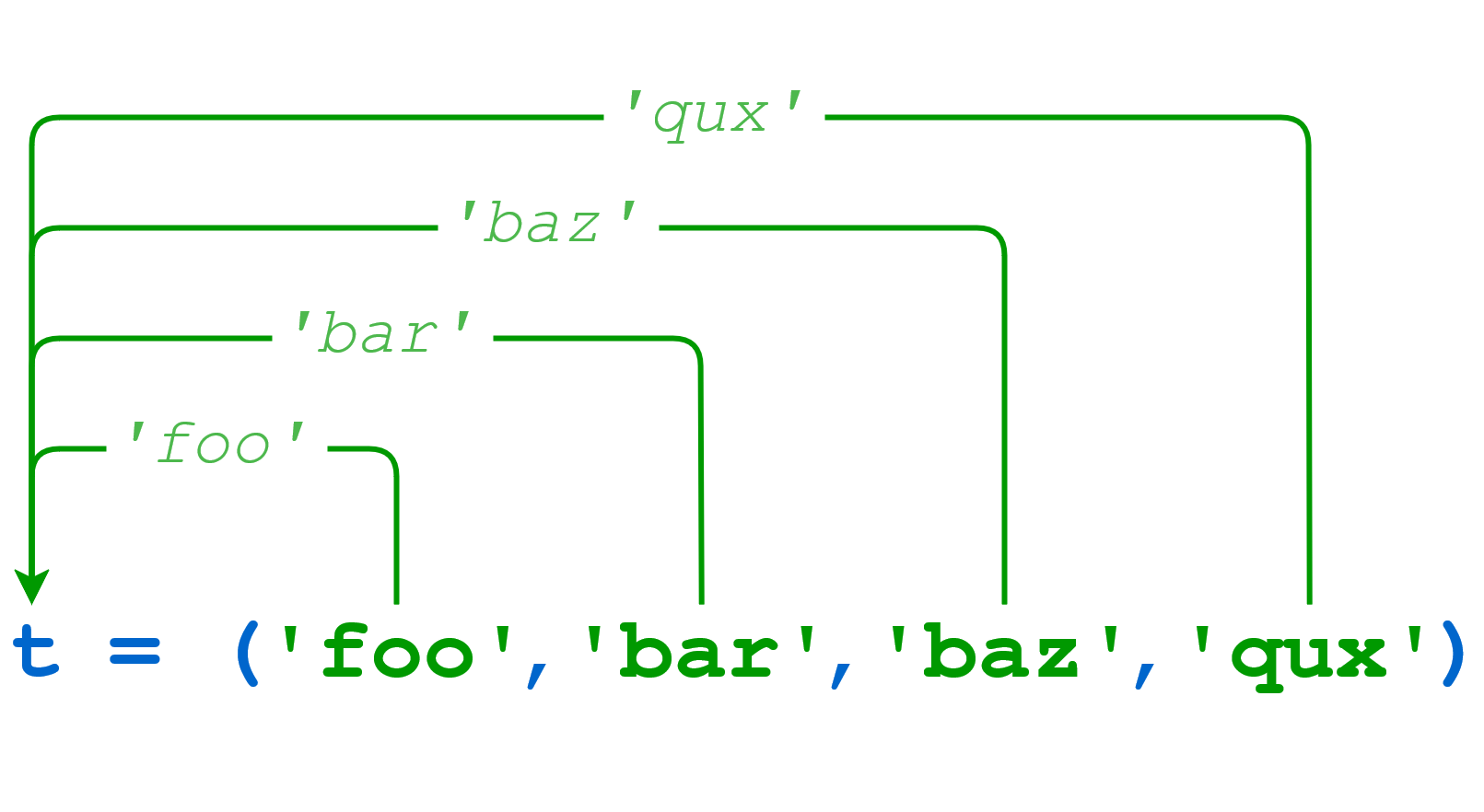
If that “packed” object is subsequently assigned to a new tuple, the individual items are “unpacked” into the objects in the tuple:

When unpacking, the number of variables on the left must match the number of values in the tuple:
Packing and unpacking can be combined into one statement to make a compound assignment:
Again, the number of elements in the tuple on the left of the assignment must equal the number on the right:
In assignments like this and a small handful of other situations, Python allows the parentheses that are usually used for denoting a tuple to be left out:
It works the same whether the parentheses are included or not, so if you have any doubt as to whether they’re needed, go ahead and include them.
Tuple assignment allows for a curious bit of idiomatic Python. Frequently when programming, you have two variables whose values you need to swap. In most programming languages, it is necessary to store one of the values in a temporary variable while the swap occurs like this:
In Python, the swap can be done with a single tuple assignment:
As anyone who has ever had to swap values using a temporary variable knows, being able to do it this way in Python is the pinnacle of modern technological achievement. It will never get better than this.
This tutorial covered the basic properties of Python lists and tuples , and how to manipulate them. You will use these extensively in your Python programming.
One of the chief characteristics of a list is that it is ordered. The order of the elements in a list is an intrinsic property of that list and does not change, unless the list itself is modified. (The same is true of tuples, except of course they can’t be modified.)
The next tutorial will introduce you to the Python dictionary: a composite data type that is unordered. Read on!
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
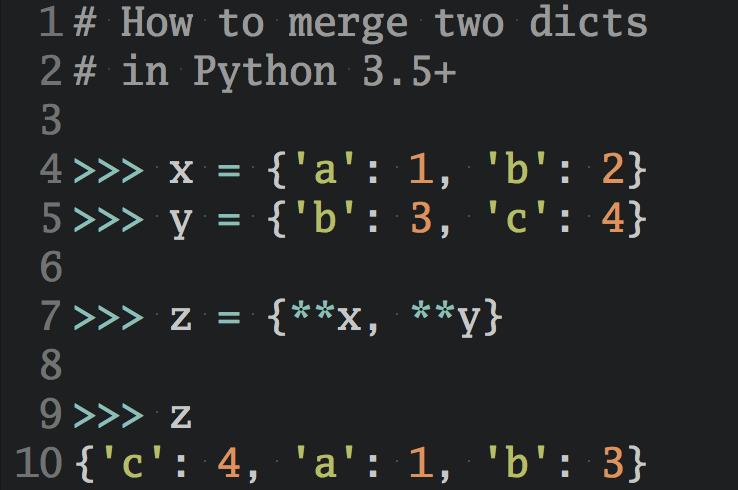
About John Sturtz
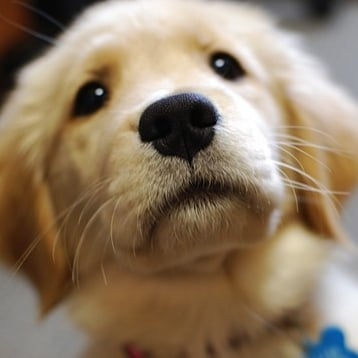
John is an avid Pythonista and a member of the Real Python tutorial team.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
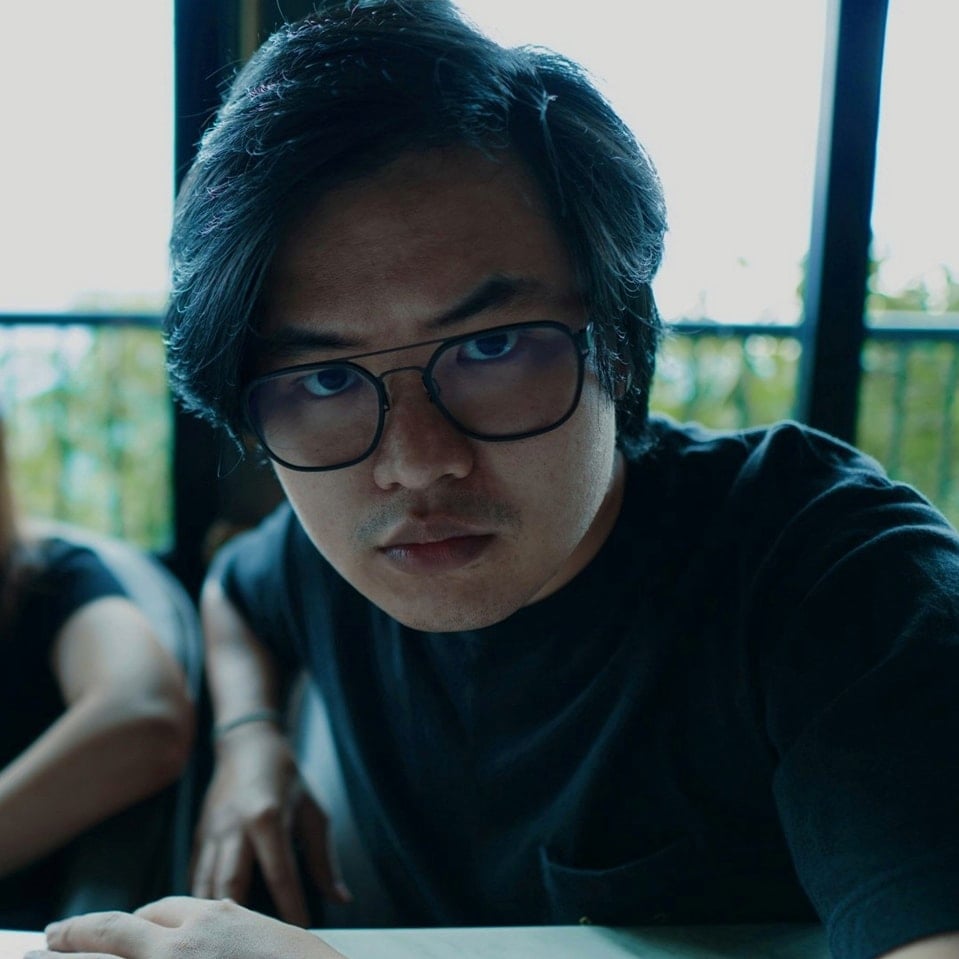
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: basics python
Recommended Video Course: Lists and Tuples in Python
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In
- Python »
- 3.12.5 Documentation »
- The Python Tutorial »
- 5. Data Structures
- Theme Auto Light Dark |
5. Data Structures ¶
This chapter describes some things you’ve learned about already in more detail, and adds some new things as well.
5.1. More on Lists ¶
The list data type has some more methods. Here are all of the methods of list objects:
Add an item to the end of the list. Equivalent to a[len(a):] = [x] .
Extend the list by appending all the items from the iterable. Equivalent to a[len(a):] = iterable .
Insert an item at a given position. The first argument is the index of the element before which to insert, so a.insert(0, x) inserts at the front of the list, and a.insert(len(a), x) is equivalent to a.append(x) .
Remove the first item from the list whose value is equal to x . It raises a ValueError if there is no such item.
Remove the item at the given position in the list, and return it. If no index is specified, a.pop() removes and returns the last item in the list. It raises an IndexError if the list is empty or the index is outside the list range.
Remove all items from the list. Equivalent to del a[:] .
Return zero-based index in the list of the first item whose value is equal to x . Raises a ValueError if there is no such item.
The optional arguments start and end are interpreted as in the slice notation and are used to limit the search to a particular subsequence of the list. The returned index is computed relative to the beginning of the full sequence rather than the start argument.
Return the number of times x appears in the list.
Sort the items of the list in place (the arguments can be used for sort customization, see sorted() for their explanation).
Reverse the elements of the list in place.
Return a shallow copy of the list. Equivalent to a[:] .
An example that uses most of the list methods:
You might have noticed that methods like insert , remove or sort that only modify the list have no return value printed – they return the default None . [ 1 ] This is a design principle for all mutable data structures in Python.
Another thing you might notice is that not all data can be sorted or compared. For instance, [None, 'hello', 10] doesn’t sort because integers can’t be compared to strings and None can’t be compared to other types. Also, there are some types that don’t have a defined ordering relation. For example, 3+4j < 5+7j isn’t a valid comparison.
5.1.1. Using Lists as Stacks ¶
The list methods make it very easy to use a list as a stack, where the last element added is the first element retrieved (“last-in, first-out”). To add an item to the top of the stack, use append() . To retrieve an item from the top of the stack, use pop() without an explicit index. For example:
5.1.2. Using Lists as Queues ¶
It is also possible to use a list as a queue, where the first element added is the first element retrieved (“first-in, first-out”); however, lists are not efficient for this purpose. While appends and pops from the end of list are fast, doing inserts or pops from the beginning of a list is slow (because all of the other elements have to be shifted by one).
To implement a queue, use collections.deque which was designed to have fast appends and pops from both ends. For example:
5.1.3. List Comprehensions ¶
List comprehensions provide a concise way to create lists. Common applications are to make new lists where each element is the result of some operations applied to each member of another sequence or iterable, or to create a subsequence of those elements that satisfy a certain condition.
For example, assume we want to create a list of squares, like:
Note that this creates (or overwrites) a variable named x that still exists after the loop completes. We can calculate the list of squares without any side effects using:
or, equivalently:
which is more concise and readable.
A list comprehension consists of brackets containing an expression followed by a for clause, then zero or more for or if clauses. The result will be a new list resulting from evaluating the expression in the context of the for and if clauses which follow it. For example, this listcomp combines the elements of two lists if they are not equal:
and it’s equivalent to:
Note how the order of the for and if statements is the same in both these snippets.
If the expression is a tuple (e.g. the (x, y) in the previous example), it must be parenthesized.
List comprehensions can contain complex expressions and nested functions:
5.1.4. Nested List Comprehensions ¶
The initial expression in a list comprehension can be any arbitrary expression, including another list comprehension.
Consider the following example of a 3x4 matrix implemented as a list of 3 lists of length 4:
The following list comprehension will transpose rows and columns:
As we saw in the previous section, the inner list comprehension is evaluated in the context of the for that follows it, so this example is equivalent to:
which, in turn, is the same as:
In the real world, you should prefer built-in functions to complex flow statements. The zip() function would do a great job for this use case:
See Unpacking Argument Lists for details on the asterisk in this line.
5.2. The del statement ¶
There is a way to remove an item from a list given its index instead of its value: the del statement. This differs from the pop() method which returns a value. The del statement can also be used to remove slices from a list or clear the entire list (which we did earlier by assignment of an empty list to the slice). For example:
del can also be used to delete entire variables:
Referencing the name a hereafter is an error (at least until another value is assigned to it). We’ll find other uses for del later.
5.3. Tuples and Sequences ¶
We saw that lists and strings have many common properties, such as indexing and slicing operations. They are two examples of sequence data types (see Sequence Types — list, tuple, range ). Since Python is an evolving language, other sequence data types may be added. There is also another standard sequence data type: the tuple .
A tuple consists of a number of values separated by commas, for instance:
As you see, on output tuples are always enclosed in parentheses, so that nested tuples are interpreted correctly; they may be input with or without surrounding parentheses, although often parentheses are necessary anyway (if the tuple is part of a larger expression). It is not possible to assign to the individual items of a tuple, however it is possible to create tuples which contain mutable objects, such as lists.
Though tuples may seem similar to lists, they are often used in different situations and for different purposes. Tuples are immutable , and usually contain a heterogeneous sequence of elements that are accessed via unpacking (see later in this section) or indexing (or even by attribute in the case of namedtuples ). Lists are mutable , and their elements are usually homogeneous and are accessed by iterating over the list.
A special problem is the construction of tuples containing 0 or 1 items: the syntax has some extra quirks to accommodate these. Empty tuples are constructed by an empty pair of parentheses; a tuple with one item is constructed by following a value with a comma (it is not sufficient to enclose a single value in parentheses). Ugly, but effective. For example:
The statement t = 12345, 54321, 'hello!' is an example of tuple packing : the values 12345 , 54321 and 'hello!' are packed together in a tuple. The reverse operation is also possible:
This is called, appropriately enough, sequence unpacking and works for any sequence on the right-hand side. Sequence unpacking requires that there are as many variables on the left side of the equals sign as there are elements in the sequence. Note that multiple assignment is really just a combination of tuple packing and sequence unpacking.
5.4. Sets ¶
Python also includes a data type for sets . A set is an unordered collection with no duplicate elements. Basic uses include membership testing and eliminating duplicate entries. Set objects also support mathematical operations like union, intersection, difference, and symmetric difference.
Curly braces or the set() function can be used to create sets. Note: to create an empty set you have to use set() , not {} ; the latter creates an empty dictionary, a data structure that we discuss in the next section.
Here is a brief demonstration:
Similarly to list comprehensions , set comprehensions are also supported:
5.5. Dictionaries ¶
Another useful data type built into Python is the dictionary (see Mapping Types — dict ). Dictionaries are sometimes found in other languages as “associative memories” or “associative arrays”. Unlike sequences, which are indexed by a range of numbers, dictionaries are indexed by keys , which can be any immutable type; strings and numbers can always be keys. Tuples can be used as keys if they contain only strings, numbers, or tuples; if a tuple contains any mutable object either directly or indirectly, it cannot be used as a key. You can’t use lists as keys, since lists can be modified in place using index assignments, slice assignments, or methods like append() and extend() .
It is best to think of a dictionary as a set of key: value pairs, with the requirement that the keys are unique (within one dictionary). A pair of braces creates an empty dictionary: {} . Placing a comma-separated list of key:value pairs within the braces adds initial key:value pairs to the dictionary; this is also the way dictionaries are written on output.
The main operations on a dictionary are storing a value with some key and extracting the value given the key. It is also possible to delete a key:value pair with del . If you store using a key that is already in use, the old value associated with that key is forgotten. It is an error to extract a value using a non-existent key.
Performing list(d) on a dictionary returns a list of all the keys used in the dictionary, in insertion order (if you want it sorted, just use sorted(d) instead). To check whether a single key is in the dictionary, use the in keyword.
Here is a small example using a dictionary:
The dict() constructor builds dictionaries directly from sequences of key-value pairs:
In addition, dict comprehensions can be used to create dictionaries from arbitrary key and value expressions:
When the keys are simple strings, it is sometimes easier to specify pairs using keyword arguments:
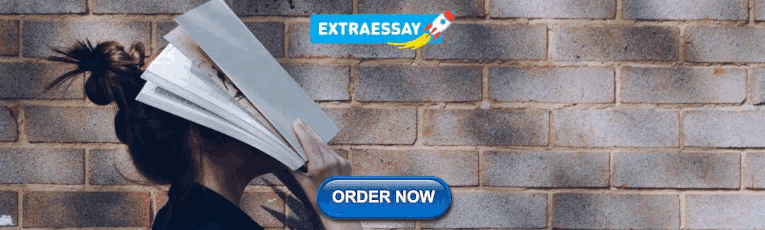
5.6. Looping Techniques ¶
When looping through dictionaries, the key and corresponding value can be retrieved at the same time using the items() method.
When looping through a sequence, the position index and corresponding value can be retrieved at the same time using the enumerate() function.
To loop over two or more sequences at the same time, the entries can be paired with the zip() function.
To loop over a sequence in reverse, first specify the sequence in a forward direction and then call the reversed() function.
To loop over a sequence in sorted order, use the sorted() function which returns a new sorted list while leaving the source unaltered.
Using set() on a sequence eliminates duplicate elements. The use of sorted() in combination with set() over a sequence is an idiomatic way to loop over unique elements of the sequence in sorted order.
It is sometimes tempting to change a list while you are looping over it; however, it is often simpler and safer to create a new list instead.
5.7. More on Conditions ¶
The conditions used in while and if statements can contain any operators, not just comparisons.
The comparison operators in and not in are membership tests that determine whether a value is in (or not in) a container. The operators is and is not compare whether two objects are really the same object. All comparison operators have the same priority, which is lower than that of all numerical operators.
Comparisons can be chained. For example, a < b == c tests whether a is less than b and moreover b equals c .
Comparisons may be combined using the Boolean operators and and or , and the outcome of a comparison (or of any other Boolean expression) may be negated with not . These have lower priorities than comparison operators; between them, not has the highest priority and or the lowest, so that A and not B or C is equivalent to (A and (not B)) or C . As always, parentheses can be used to express the desired composition.
The Boolean operators and and or are so-called short-circuit operators: their arguments are evaluated from left to right, and evaluation stops as soon as the outcome is determined. For example, if A and C are true but B is false, A and B and C does not evaluate the expression C . When used as a general value and not as a Boolean, the return value of a short-circuit operator is the last evaluated argument.
It is possible to assign the result of a comparison or other Boolean expression to a variable. For example,
Note that in Python, unlike C, assignment inside expressions must be done explicitly with the walrus operator := . This avoids a common class of problems encountered in C programs: typing = in an expression when == was intended.
5.8. Comparing Sequences and Other Types ¶
Sequence objects typically may be compared to other objects with the same sequence type. The comparison uses lexicographical ordering: first the first two items are compared, and if they differ this determines the outcome of the comparison; if they are equal, the next two items are compared, and so on, until either sequence is exhausted. If two items to be compared are themselves sequences of the same type, the lexicographical comparison is carried out recursively. If all items of two sequences compare equal, the sequences are considered equal. If one sequence is an initial sub-sequence of the other, the shorter sequence is the smaller (lesser) one. Lexicographical ordering for strings uses the Unicode code point number to order individual characters. Some examples of comparisons between sequences of the same type:
Note that comparing objects of different types with < or > is legal provided that the objects have appropriate comparison methods. For example, mixed numeric types are compared according to their numeric value, so 0 equals 0.0, etc. Otherwise, rather than providing an arbitrary ordering, the interpreter will raise a TypeError exception.
Table of Contents
- 5.1.1. Using Lists as Stacks
- 5.1.2. Using Lists as Queues
- 5.1.3. List Comprehensions
- 5.1.4. Nested List Comprehensions
- 5.2. The del statement
- 5.3. Tuples and Sequences
- 5.5. Dictionaries
- 5.6. Looping Techniques
- 5.7. More on Conditions
- 5.8. Comparing Sequences and Other Types
Previous topic
4. More Control Flow Tools
- Report a Bug
- Show Source
Python Programming
Python List Exercise with Solutions
Updated on: December 8, 2021 | 200 Comments
Python list is the most widely used data structure, and a good understanding of it is necessary. This Python list exercise aims to help developers learn and practice list operations. All questions are tested on Python 3.
Also Read :
- Python List
- Python List Quiz
This Python list exercise includes the following : –
The exercise contains 10 questions and solutions provided for each question. You need to solve and practice different list programs, questions, problems, and challenges.
Questions cover the following list topics:
- list operations and manipulations
- list functions
- list slicing
- list comprehension
When you complete each question, you get more familiar with the Python list type. Let us know if you have any alternative solutions in the comment section below.
- Use Online Code Editor to solve exercise questions .
- Read the Complete guide on Python List to solve this exercise.
Table of contents
Exercise 1: reverse a list in python, exercise 2: concatenate two lists index-wise, exercise 3: turn every item of a list into its square, exercise 4: concatenate two lists in the following order, exercise 5: iterate both lists simultaneously, exercise 6: remove empty strings from the list of strings, exercise 7: add new item to list after a specified item, exercise 8: extend nested list by adding the sublist, exercise 9: replace list’s item with new value if found, exercise 10: remove all occurrences of a specific item from a list..
Expected output:
Use the list function reverse()
Solution 1 : list function reverse()
Solution 2 : Using negative slicing
-1 indicates to start from the last item.
Write a program to add two lists index-wise. Create a new list that contains the 0th index item from both the list, then the 1st index item, and so on till the last element. any leftover items will get added at the end of the new list.
Use list comprehension with the zip() function
Use the zip() function. This function takes two or more iterables (like list, dict, string), aggregates them in a tuple, and returns it.
Given a list of numbers. write a program to turn every item of a list into its square.
Iterate numbers from a list one by one using a for loop and calculate the square of the current number
Solution 1 : Using loop and list method
- Create an empty result list
- Iterate a numbers list using a loop
- In each iteration, calculate the square of a current number and add it to the result list using the append() method.
Solution 2 : Use list comprehension
Use a list comprehension to iterate two lists using a for loop and concatenate the current item of each list.
Given a two Python list. Write a program to iterate both lists simultaneously and display items from list1 in original order and items from list2 in reverse order.
Use the zip() function. This function takes two or more iterables (like list, dict , string), aggregates them in a tuple , and returns it.
- The zip() function can take two or more lists, aggregate them in a tuple, and returns it.
- Pass the first argument as a list1 and seconds argument as a list2[::-1] (reverse list using list slicing)
- Iterate the result using a for loop
Use a filter() function to remove the None / empty type from the list
Use a filter() function to remove None type from the list
Write a program to add item 7000 after 6000 in the following Python List
The given list is a nested list. Use indexing to locate the specified item, then use the append() method to add a new item after it.
Use the append() method
You have given a nested list. Write a program to extend it by adding the sublist ["h", "i", "j"] in such a way that it will look like the following list.
Given List :
Expected Output:
The given list is a nested list. Use indexing to locate the specified sublist item, then use the extend() method to add new items after it.
You have given a Python list. Write a program to find value 20 in the list, and if it is present, replace it with 200. Only update the first occurrence of an item.
- Use list method index(20) to get the index number of a 20
- Next, update the item present at the location using the index number
Given a Python list, write a program to remove all occurrences of item 20.
Solution 1 : Use the list comprehension
Solution 2 : while loop (slow solution)
Did you find this page helpful? Let others know about it. Sharing helps me continue to create free Python resources.
About Vishal

I’m Vishal Hule , the Founder of PYnative.com. As a Python developer, I enjoy assisting students, developers, and learners. Follow me on Twitter .
Related Tutorial Topics:
Python exercises and quizzes.
Free coding exercises and quizzes cover Python basics, data structure, data analytics, and more.
- 15+ Topic-specific Exercises and Quizzes
- Each Exercise contains 10 questions
- Each Quiz contains 12-15 MCQ
Loading comments... Please wait.
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .
Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Online Python Code Editor
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
Python Enhancement Proposals
- Python »
- PEP Index »
PEP 572 – Assignment Expressions
The importance of real code, exceptional cases, scope of the target, relative precedence of :=, change to evaluation order, differences between assignment expressions and assignment statements, specification changes during implementation, _pydecimal.py, datetime.py, sysconfig.py, simplifying list comprehensions, capturing condition values, changing the scope rules for comprehensions, alternative spellings, special-casing conditional statements, special-casing comprehensions, lowering operator precedence, allowing commas to the right, always requiring parentheses, why not just turn existing assignment into an expression, with assignment expressions, why bother with assignment statements, why not use a sublocal scope and prevent namespace pollution, style guide recommendations, acknowledgements, a numeric example, appendix b: rough code translations for comprehensions, appendix c: no changes to scope semantics.
This is a proposal for creating a way to assign to variables within an expression using the notation NAME := expr .
As part of this change, there is also an update to dictionary comprehension evaluation order to ensure key expressions are executed before value expressions (allowing the key to be bound to a name and then re-used as part of calculating the corresponding value).
During discussion of this PEP, the operator became informally known as “the walrus operator”. The construct’s formal name is “Assignment Expressions” (as per the PEP title), but they may also be referred to as “Named Expressions” (e.g. the CPython reference implementation uses that name internally).
Naming the result of an expression is an important part of programming, allowing a descriptive name to be used in place of a longer expression, and permitting reuse. Currently, this feature is available only in statement form, making it unavailable in list comprehensions and other expression contexts.
Additionally, naming sub-parts of a large expression can assist an interactive debugger, providing useful display hooks and partial results. Without a way to capture sub-expressions inline, this would require refactoring of the original code; with assignment expressions, this merely requires the insertion of a few name := markers. Removing the need to refactor reduces the likelihood that the code be inadvertently changed as part of debugging (a common cause of Heisenbugs), and is easier to dictate to another programmer.
During the development of this PEP many people (supporters and critics both) have had a tendency to focus on toy examples on the one hand, and on overly complex examples on the other.
The danger of toy examples is twofold: they are often too abstract to make anyone go “ooh, that’s compelling”, and they are easily refuted with “I would never write it that way anyway”.
The danger of overly complex examples is that they provide a convenient strawman for critics of the proposal to shoot down (“that’s obfuscated”).
Yet there is some use for both extremely simple and extremely complex examples: they are helpful to clarify the intended semantics. Therefore, there will be some of each below.
However, in order to be compelling , examples should be rooted in real code, i.e. code that was written without any thought of this PEP, as part of a useful application, however large or small. Tim Peters has been extremely helpful by going over his own personal code repository and picking examples of code he had written that (in his view) would have been clearer if rewritten with (sparing) use of assignment expressions. His conclusion: the current proposal would have allowed a modest but clear improvement in quite a few bits of code.
Another use of real code is to observe indirectly how much value programmers place on compactness. Guido van Rossum searched through a Dropbox code base and discovered some evidence that programmers value writing fewer lines over shorter lines.
Case in point: Guido found several examples where a programmer repeated a subexpression, slowing down the program, in order to save one line of code, e.g. instead of writing:
they would write:
Another example illustrates that programmers sometimes do more work to save an extra level of indentation:
This code tries to match pattern2 even if pattern1 has a match (in which case the match on pattern2 is never used). The more efficient rewrite would have been:
Syntax and semantics
In most contexts where arbitrary Python expressions can be used, a named expression can appear. This is of the form NAME := expr where expr is any valid Python expression other than an unparenthesized tuple, and NAME is an identifier.
The value of such a named expression is the same as the incorporated expression, with the additional side-effect that the target is assigned that value:
There are a few places where assignment expressions are not allowed, in order to avoid ambiguities or user confusion:
This rule is included to simplify the choice for the user between an assignment statement and an assignment expression – there is no syntactic position where both are valid.
Again, this rule is included to avoid two visually similar ways of saying the same thing.
This rule is included to disallow excessively confusing code, and because parsing keyword arguments is complex enough already.
This rule is included to discourage side effects in a position whose exact semantics are already confusing to many users (cf. the common style recommendation against mutable default values), and also to echo the similar prohibition in calls (the previous bullet).
The reasoning here is similar to the two previous cases; this ungrouped assortment of symbols and operators composed of : and = is hard to read correctly.
This allows lambda to always bind less tightly than := ; having a name binding at the top level inside a lambda function is unlikely to be of value, as there is no way to make use of it. In cases where the name will be used more than once, the expression is likely to need parenthesizing anyway, so this prohibition will rarely affect code.
This shows that what looks like an assignment operator in an f-string is not always an assignment operator. The f-string parser uses : to indicate formatting options. To preserve backwards compatibility, assignment operator usage inside of f-strings must be parenthesized. As noted above, this usage of the assignment operator is not recommended.
An assignment expression does not introduce a new scope. In most cases the scope in which the target will be bound is self-explanatory: it is the current scope. If this scope contains a nonlocal or global declaration for the target, the assignment expression honors that. A lambda (being an explicit, if anonymous, function definition) counts as a scope for this purpose.
There is one special case: an assignment expression occurring in a list, set or dict comprehension or in a generator expression (below collectively referred to as “comprehensions”) binds the target in the containing scope, honoring a nonlocal or global declaration for the target in that scope, if one exists. For the purpose of this rule the containing scope of a nested comprehension is the scope that contains the outermost comprehension. A lambda counts as a containing scope.
The motivation for this special case is twofold. First, it allows us to conveniently capture a “witness” for an any() expression, or a counterexample for all() , for example:
Second, it allows a compact way of updating mutable state from a comprehension, for example:
However, an assignment expression target name cannot be the same as a for -target name appearing in any comprehension containing the assignment expression. The latter names are local to the comprehension in which they appear, so it would be contradictory for a contained use of the same name to refer to the scope containing the outermost comprehension instead.
For example, [i := i+1 for i in range(5)] is invalid: the for i part establishes that i is local to the comprehension, but the i := part insists that i is not local to the comprehension. The same reason makes these examples invalid too:
While it’s technically possible to assign consistent semantics to these cases, it’s difficult to determine whether those semantics actually make sense in the absence of real use cases. Accordingly, the reference implementation [1] will ensure that such cases raise SyntaxError , rather than executing with implementation defined behaviour.
This restriction applies even if the assignment expression is never executed:
For the comprehension body (the part before the first “for” keyword) and the filter expression (the part after “if” and before any nested “for”), this restriction applies solely to target names that are also used as iteration variables in the comprehension. Lambda expressions appearing in these positions introduce a new explicit function scope, and hence may use assignment expressions with no additional restrictions.
Due to design constraints in the reference implementation (the symbol table analyser cannot easily detect when names are re-used between the leftmost comprehension iterable expression and the rest of the comprehension), named expressions are disallowed entirely as part of comprehension iterable expressions (the part after each “in”, and before any subsequent “if” or “for” keyword):
A further exception applies when an assignment expression occurs in a comprehension whose containing scope is a class scope. If the rules above were to result in the target being assigned in that class’s scope, the assignment expression is expressly invalid. This case also raises SyntaxError :
(The reason for the latter exception is the implicit function scope created for comprehensions – there is currently no runtime mechanism for a function to refer to a variable in the containing class scope, and we do not want to add such a mechanism. If this issue ever gets resolved this special case may be removed from the specification of assignment expressions. Note that the problem already exists for using a variable defined in the class scope from a comprehension.)
See Appendix B for some examples of how the rules for targets in comprehensions translate to equivalent code.
The := operator groups more tightly than a comma in all syntactic positions where it is legal, but less tightly than all other operators, including or , and , not , and conditional expressions ( A if C else B ). As follows from section “Exceptional cases” above, it is never allowed at the same level as = . In case a different grouping is desired, parentheses should be used.
The := operator may be used directly in a positional function call argument; however it is invalid directly in a keyword argument.
Some examples to clarify what’s technically valid or invalid:
Most of the “valid” examples above are not recommended, since human readers of Python source code who are quickly glancing at some code may miss the distinction. But simple cases are not objectionable:
This PEP recommends always putting spaces around := , similar to PEP 8 ’s recommendation for = when used for assignment, whereas the latter disallows spaces around = used for keyword arguments.)
In order to have precisely defined semantics, the proposal requires evaluation order to be well-defined. This is technically not a new requirement, as function calls may already have side effects. Python already has a rule that subexpressions are generally evaluated from left to right. However, assignment expressions make these side effects more visible, and we propose a single change to the current evaluation order:
- In a dict comprehension {X: Y for ...} , Y is currently evaluated before X . We propose to change this so that X is evaluated before Y . (In a dict display like {X: Y} this is already the case, and also in dict((X, Y) for ...) which should clearly be equivalent to the dict comprehension.)
Most importantly, since := is an expression, it can be used in contexts where statements are illegal, including lambda functions and comprehensions.
Conversely, assignment expressions don’t support the advanced features found in assignment statements:
- Multiple targets are not directly supported: x = y = z = 0 # Equivalent: (z := (y := (x := 0)))
- Single assignment targets other than a single NAME are not supported: # No equivalent a [ i ] = x self . rest = []
- Priority around commas is different: x = 1 , 2 # Sets x to (1, 2) ( x := 1 , 2 ) # Sets x to 1
- Iterable packing and unpacking (both regular or extended forms) are not supported: # Equivalent needs extra parentheses loc = x , y # Use (loc := (x, y)) info = name , phone , * rest # Use (info := (name, phone, *rest)) # No equivalent px , py , pz = position name , phone , email , * other_info = contact
- Inline type annotations are not supported: # Closest equivalent is "p: Optional[int]" as a separate declaration p : Optional [ int ] = None
- Augmented assignment is not supported: total += tax # Equivalent: (total := total + tax)
The following changes have been made based on implementation experience and additional review after the PEP was first accepted and before Python 3.8 was released:
- for consistency with other similar exceptions, and to avoid locking in an exception name that is not necessarily going to improve clarity for end users, the originally proposed TargetScopeError subclass of SyntaxError was dropped in favour of just raising SyntaxError directly. [3]
- due to a limitation in CPython’s symbol table analysis process, the reference implementation raises SyntaxError for all uses of named expressions inside comprehension iterable expressions, rather than only raising them when the named expression target conflicts with one of the iteration variables in the comprehension. This could be revisited given sufficiently compelling examples, but the extra complexity needed to implement the more selective restriction doesn’t seem worthwhile for purely hypothetical use cases.
Examples from the Python standard library
env_base is only used on these lines, putting its assignment on the if moves it as the “header” of the block.
- Current: env_base = os . environ . get ( "PYTHONUSERBASE" , None ) if env_base : return env_base
- Improved: if env_base := os . environ . get ( "PYTHONUSERBASE" , None ): return env_base
Avoid nested if and remove one indentation level.
- Current: if self . _is_special : ans = self . _check_nans ( context = context ) if ans : return ans
- Improved: if self . _is_special and ( ans := self . _check_nans ( context = context )): return ans
Code looks more regular and avoid multiple nested if. (See Appendix A for the origin of this example.)
- Current: reductor = dispatch_table . get ( cls ) if reductor : rv = reductor ( x ) else : reductor = getattr ( x , "__reduce_ex__" , None ) if reductor : rv = reductor ( 4 ) else : reductor = getattr ( x , "__reduce__" , None ) if reductor : rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
- Improved: if reductor := dispatch_table . get ( cls ): rv = reductor ( x ) elif reductor := getattr ( x , "__reduce_ex__" , None ): rv = reductor ( 4 ) elif reductor := getattr ( x , "__reduce__" , None ): rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
tz is only used for s += tz , moving its assignment inside the if helps to show its scope.
- Current: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) tz = self . _tzstr () if tz : s += tz return s
- Improved: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) if tz := self . _tzstr (): s += tz return s
Calling fp.readline() in the while condition and calling .match() on the if lines make the code more compact without making it harder to understand.
- Current: while True : line = fp . readline () if not line : break m = define_rx . match ( line ) if m : n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v else : m = undef_rx . match ( line ) if m : vars [ m . group ( 1 )] = 0
- Improved: while line := fp . readline (): if m := define_rx . match ( line ): n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v elif m := undef_rx . match ( line ): vars [ m . group ( 1 )] = 0
A list comprehension can map and filter efficiently by capturing the condition:
Similarly, a subexpression can be reused within the main expression, by giving it a name on first use:
Note that in both cases the variable y is bound in the containing scope (i.e. at the same level as results or stuff ).
Assignment expressions can be used to good effect in the header of an if or while statement:
Particularly with the while loop, this can remove the need to have an infinite loop, an assignment, and a condition. It also creates a smooth parallel between a loop which simply uses a function call as its condition, and one which uses that as its condition but also uses the actual value.
An example from the low-level UNIX world:
Rejected alternative proposals
Proposals broadly similar to this one have come up frequently on python-ideas. Below are a number of alternative syntaxes, some of them specific to comprehensions, which have been rejected in favour of the one given above.
A previous version of this PEP proposed subtle changes to the scope rules for comprehensions, to make them more usable in class scope and to unify the scope of the “outermost iterable” and the rest of the comprehension. However, this part of the proposal would have caused backwards incompatibilities, and has been withdrawn so the PEP can focus on assignment expressions.
Broadly the same semantics as the current proposal, but spelled differently.
Since EXPR as NAME already has meaning in import , except and with statements (with different semantics), this would create unnecessary confusion or require special-casing (e.g. to forbid assignment within the headers of these statements).
(Note that with EXPR as VAR does not simply assign the value of EXPR to VAR – it calls EXPR.__enter__() and assigns the result of that to VAR .)
Additional reasons to prefer := over this spelling include:
- In if f(x) as y the assignment target doesn’t jump out at you – it just reads like if f x blah blah and it is too similar visually to if f(x) and y .
- import foo as bar
- except Exc as var
- with ctxmgr() as var
To the contrary, the assignment expression does not belong to the if or while that starts the line, and we intentionally allow assignment expressions in other contexts as well.
- NAME = EXPR
- if NAME := EXPR
reinforces the visual recognition of assignment expressions.
This syntax is inspired by languages such as R and Haskell, and some programmable calculators. (Note that a left-facing arrow y <- f(x) is not possible in Python, as it would be interpreted as less-than and unary minus.) This syntax has a slight advantage over ‘as’ in that it does not conflict with with , except and import , but otherwise is equivalent. But it is entirely unrelated to Python’s other use of -> (function return type annotations), and compared to := (which dates back to Algol-58) it has a much weaker tradition.
This has the advantage that leaked usage can be readily detected, removing some forms of syntactic ambiguity. However, this would be the only place in Python where a variable’s scope is encoded into its name, making refactoring harder.
Execution order is inverted (the indented body is performed first, followed by the “header”). This requires a new keyword, unless an existing keyword is repurposed (most likely with: ). See PEP 3150 for prior discussion on this subject (with the proposed keyword being given: ).
This syntax has fewer conflicts than as does (conflicting only with the raise Exc from Exc notation), but is otherwise comparable to it. Instead of paralleling with expr as target: (which can be useful but can also be confusing), this has no parallels, but is evocative.
One of the most popular use-cases is if and while statements. Instead of a more general solution, this proposal enhances the syntax of these two statements to add a means of capturing the compared value:
This works beautifully if and ONLY if the desired condition is based on the truthiness of the captured value. It is thus effective for specific use-cases (regex matches, socket reads that return '' when done), and completely useless in more complicated cases (e.g. where the condition is f(x) < 0 and you want to capture the value of f(x) ). It also has no benefit to list comprehensions.
Advantages: No syntactic ambiguities. Disadvantages: Answers only a fraction of possible use-cases, even in if / while statements.
Another common use-case is comprehensions (list/set/dict, and genexps). As above, proposals have been made for comprehension-specific solutions.
This brings the subexpression to a location in between the ‘for’ loop and the expression. It introduces an additional language keyword, which creates conflicts. Of the three, where reads the most cleanly, but also has the greatest potential for conflict (e.g. SQLAlchemy and numpy have where methods, as does tkinter.dnd.Icon in the standard library).
As above, but reusing the with keyword. Doesn’t read too badly, and needs no additional language keyword. Is restricted to comprehensions, though, and cannot as easily be transformed into “longhand” for-loop syntax. Has the C problem that an equals sign in an expression can now create a name binding, rather than performing a comparison. Would raise the question of why “with NAME = EXPR:” cannot be used as a statement on its own.
As per option 2, but using as rather than an equals sign. Aligns syntactically with other uses of as for name binding, but a simple transformation to for-loop longhand would create drastically different semantics; the meaning of with inside a comprehension would be completely different from the meaning as a stand-alone statement, while retaining identical syntax.
Regardless of the spelling chosen, this introduces a stark difference between comprehensions and the equivalent unrolled long-hand form of the loop. It is no longer possible to unwrap the loop into statement form without reworking any name bindings. The only keyword that can be repurposed to this task is with , thus giving it sneakily different semantics in a comprehension than in a statement; alternatively, a new keyword is needed, with all the costs therein.
There are two logical precedences for the := operator. Either it should bind as loosely as possible, as does statement-assignment; or it should bind more tightly than comparison operators. Placing its precedence between the comparison and arithmetic operators (to be precise: just lower than bitwise OR) allows most uses inside while and if conditions to be spelled without parentheses, as it is most likely that you wish to capture the value of something, then perform a comparison on it:
Once find() returns -1, the loop terminates. If := binds as loosely as = does, this would capture the result of the comparison (generally either True or False ), which is less useful.
While this behaviour would be convenient in many situations, it is also harder to explain than “the := operator behaves just like the assignment statement”, and as such, the precedence for := has been made as close as possible to that of = (with the exception that it binds tighter than comma).
Some critics have claimed that the assignment expressions should allow unparenthesized tuples on the right, so that these two would be equivalent:
(With the current version of the proposal, the latter would be equivalent to ((point := x), y) .)
However, adopting this stance would logically lead to the conclusion that when used in a function call, assignment expressions also bind less tight than comma, so we’d have the following confusing equivalence:
The less confusing option is to make := bind more tightly than comma.
It’s been proposed to just always require parentheses around an assignment expression. This would resolve many ambiguities, and indeed parentheses will frequently be needed to extract the desired subexpression. But in the following cases the extra parentheses feel redundant:
Frequently Raised Objections
C and its derivatives define the = operator as an expression, rather than a statement as is Python’s way. This allows assignments in more contexts, including contexts where comparisons are more common. The syntactic similarity between if (x == y) and if (x = y) belies their drastically different semantics. Thus this proposal uses := to clarify the distinction.
The two forms have different flexibilities. The := operator can be used inside a larger expression; the = statement can be augmented to += and its friends, can be chained, and can assign to attributes and subscripts.
Previous revisions of this proposal involved sublocal scope (restricted to a single statement), preventing name leakage and namespace pollution. While a definite advantage in a number of situations, this increases complexity in many others, and the costs are not justified by the benefits. In the interests of language simplicity, the name bindings created here are exactly equivalent to any other name bindings, including that usage at class or module scope will create externally-visible names. This is no different from for loops or other constructs, and can be solved the same way: del the name once it is no longer needed, or prefix it with an underscore.
(The author wishes to thank Guido van Rossum and Christoph Groth for their suggestions to move the proposal in this direction. [2] )
As expression assignments can sometimes be used equivalently to statement assignments, the question of which should be preferred will arise. For the benefit of style guides such as PEP 8 , two recommendations are suggested.
- If either assignment statements or assignment expressions can be used, prefer statements; they are a clear declaration of intent.
- If using assignment expressions would lead to ambiguity about execution order, restructure it to use statements instead.
The authors wish to thank Alyssa Coghlan and Steven D’Aprano for their considerable contributions to this proposal, and members of the core-mentorship mailing list for assistance with implementation.
Appendix A: Tim Peters’s findings
Here’s a brief essay Tim Peters wrote on the topic.
I dislike “busy” lines of code, and also dislike putting conceptually unrelated logic on a single line. So, for example, instead of:
instead. So I suspected I’d find few places I’d want to use assignment expressions. I didn’t even consider them for lines already stretching halfway across the screen. In other cases, “unrelated” ruled:
is a vast improvement over the briefer:
The original two statements are doing entirely different conceptual things, and slamming them together is conceptually insane.
In other cases, combining related logic made it harder to understand, such as rewriting:
as the briefer:
The while test there is too subtle, crucially relying on strict left-to-right evaluation in a non-short-circuiting or method-chaining context. My brain isn’t wired that way.
But cases like that were rare. Name binding is very frequent, and “sparse is better than dense” does not mean “almost empty is better than sparse”. For example, I have many functions that return None or 0 to communicate “I have nothing useful to return in this case, but since that’s expected often I’m not going to annoy you with an exception”. This is essentially the same as regular expression search functions returning None when there is no match. So there was lots of code of the form:
I find that clearer, and certainly a bit less typing and pattern-matching reading, as:
It’s also nice to trade away a small amount of horizontal whitespace to get another _line_ of surrounding code on screen. I didn’t give much weight to this at first, but it was so very frequent it added up, and I soon enough became annoyed that I couldn’t actually run the briefer code. That surprised me!
There are other cases where assignment expressions really shine. Rather than pick another from my code, Kirill Balunov gave a lovely example from the standard library’s copy() function in copy.py :
The ever-increasing indentation is semantically misleading: the logic is conceptually flat, “the first test that succeeds wins”:
Using easy assignment expressions allows the visual structure of the code to emphasize the conceptual flatness of the logic; ever-increasing indentation obscured it.
A smaller example from my code delighted me, both allowing to put inherently related logic in a single line, and allowing to remove an annoying “artificial” indentation level:
That if is about as long as I want my lines to get, but remains easy to follow.
So, in all, in most lines binding a name, I wouldn’t use assignment expressions, but because that construct is so very frequent, that leaves many places I would. In most of the latter, I found a small win that adds up due to how often it occurs, and in the rest I found a moderate to major win. I’d certainly use it more often than ternary if , but significantly less often than augmented assignment.
I have another example that quite impressed me at the time.
Where all variables are positive integers, and a is at least as large as the n’th root of x, this algorithm returns the floor of the n’th root of x (and roughly doubling the number of accurate bits per iteration):
It’s not obvious why that works, but is no more obvious in the “loop and a half” form. It’s hard to prove correctness without building on the right insight (the “arithmetic mean - geometric mean inequality”), and knowing some non-trivial things about how nested floor functions behave. That is, the challenges are in the math, not really in the coding.
If you do know all that, then the assignment-expression form is easily read as “while the current guess is too large, get a smaller guess”, where the “too large?” test and the new guess share an expensive sub-expression.
To my eyes, the original form is harder to understand:
This appendix attempts to clarify (though not specify) the rules when a target occurs in a comprehension or in a generator expression. For a number of illustrative examples we show the original code, containing a comprehension, and the translation, where the comprehension has been replaced by an equivalent generator function plus some scaffolding.
Since [x for ...] is equivalent to list(x for ...) these examples all use list comprehensions without loss of generality. And since these examples are meant to clarify edge cases of the rules, they aren’t trying to look like real code.
Note: comprehensions are already implemented via synthesizing nested generator functions like those in this appendix. The new part is adding appropriate declarations to establish the intended scope of assignment expression targets (the same scope they resolve to as if the assignment were performed in the block containing the outermost comprehension). For type inference purposes, these illustrative expansions do not imply that assignment expression targets are always Optional (but they do indicate the target binding scope).
Let’s start with a reminder of what code is generated for a generator expression without assignment expression.
- Original code (EXPR usually references VAR): def f (): a = [ EXPR for VAR in ITERABLE ]
- Translation (let’s not worry about name conflicts): def f (): def genexpr ( iterator ): for VAR in iterator : yield EXPR a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a simple assignment expression.
- Original code: def f (): a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): if False : TARGET = None # Dead code to ensure TARGET is a local variable def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a global TARGET declaration in f() .
- Original code: def f (): global TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): global TARGET def genexpr ( iterator ): global TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Or instead let’s add a nonlocal TARGET declaration in f() .
- Original code: def g (): TARGET = ... def f (): nonlocal TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def g (): TARGET = ... def f (): nonlocal TARGET def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Finally, let’s nest two comprehensions.
- Original code: def f (): a = [[ TARGET := i for i in range ( 3 )] for j in range ( 2 )] # I.e., a = [[0, 1, 2], [0, 1, 2]] print ( TARGET ) # prints 2
- Translation: def f (): if False : TARGET = None def outer_genexpr ( outer_iterator ): nonlocal TARGET def inner_generator ( inner_iterator ): nonlocal TARGET for i in inner_iterator : TARGET = i yield i for j in outer_iterator : yield list ( inner_generator ( range ( 3 ))) a = list ( outer_genexpr ( range ( 2 ))) print ( TARGET )
Because it has been a point of confusion, note that nothing about Python’s scoping semantics is changed. Function-local scopes continue to be resolved at compile time, and to have indefinite temporal extent at run time (“full closures”). Example:
This document has been placed in the public domain.
Source: https://github.com/python/peps/blob/main/peps/pep-0572.rst
Last modified: 2023-10-11 12:05:51 GMT
How-To Geek
You can build a simple to-do list app in python, here's how.

Your changes have been saved
Email is sent
Email has already been sent
Please verify your email address.
You’ve reached your account maximum for followed topics.
Python is a versatile language, and even beginners can design and implement something cool with only basic knowledge. We’ll look at developing a simple to-do list app with Python that you can actually use when you’re done!
Basic Python Concepts
By now, you should know what Python is and how it works. Before diving into the code, we’ll revisit a few of the core concepts we’ll use for this to-do list application.
Variables and Data Types
A variable is a placeholder for storing data, while a data type defines the kind of data a variable can hold, such as numbers, strings, or booleans.
User Input and Output
User Input and output are the core of our application. We want users to be able to add and delete tasks and mark them as completed, failed, or deferred.
Lists and Basic List Manipulation
We’ll also use an advanced data structure called a list for this task. Lists are just collections of other data types. You can think about it like a library shelf, with each element being one of the books on that shelf.
Breaking Down the Code
We've already set up Visual Studio for Python previously, so this gives us a chance to test it out. We'll make a new Python project file and store it somewhere, then decide what concepts we need for this application.
To keep things simple, we're just using words (strings) to define our tasks. We’re going to use a list of strings to keep track of those tasks. In Python, creating a list is as simple as typing:
This creates a list of tasks that we can add to. The app we’re developing needs to do three things:
- Remove a task
- Show us all the tasks in the queue
Adding a Task
So now that we have a list of tasks, we can add to it. Let’s create an add_task() function:
The function adds the specified task and then tells us that the task was added successfully. The confirmation is necessary because it informs us that the function is working.
Removing a Task
After adding a task, we want to be able to remove the task if it’s completed. That’s also pretty simple since lists are so powerful. Let’s add a new function to remove a task:
The code above will allow you to remove a single task once you enter the name of it. If it finds the task, it’ll remove it. What happens if the task is spelled wrong or just doesn’t exist? In that case, the code will tell us that the task doesn’t exist because it’ll look through the list and fail to find the task it’s looking for.
Showing the Task Queue
Now that we can add and remove tasks, we’ve got to have a way of displaying the list. Let’s develop a display_tasks() function that will handle this for us:
This isn’t too complicated a piece of code to understand. The first line, “if tasks:” asks Python to check if the tasks list is empty. If it isn’t, we’ll loop through it using a for loop.
For loops have a defined starting and ending point. In this example, we’ll enumerate the tasks list to get the number of tasks in the list. Then, we’ll loop through each entry and print it to the screen.
If the list is empty, we’ll just tell the user that their to-do list is empty.
Testing to See if Our Functions Work
Now that we’ve written a few functions, we must test them to see if they work. In any code, testing is crucial to ensure our code does what we want it to do. We can do this by using some test statements:
When we add this to the bottom of our Python code, we should get this result:
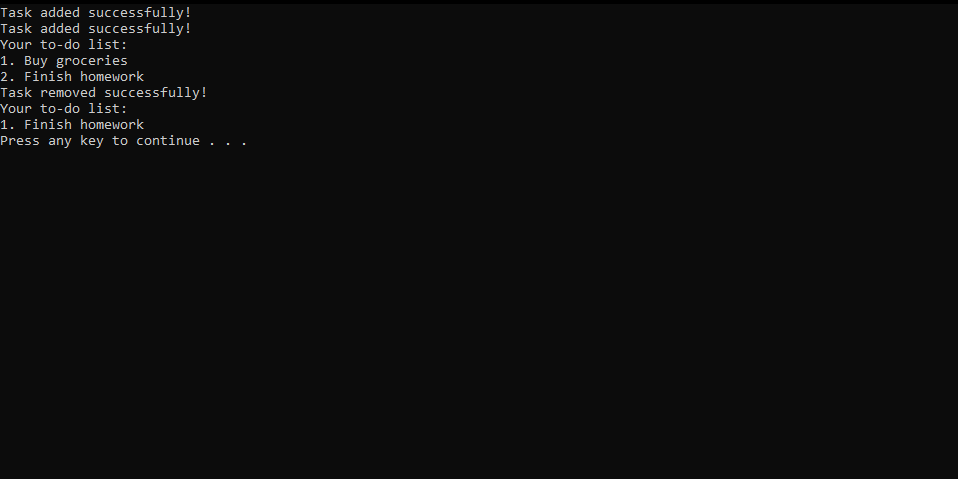
Now that we know our functions work, they’re still sort of useless because the user can’t enter their own tasks. Let’s fix that.
We need three functions: one for taking input to add a new task, one to remove an old task, and one to display the task list:
Each of these functions returns whatever the user inputs to the screen. We can think about these functions as handlers to collect input from the user and use that input immediately for something else. You can think of a "return" as what you get back from the function after calling it.
Now, we want to display a menu so the user can choose which of these tasks they want to perform:
Let’s take a look at what this does. When we run the main() function, we will display a list of options for the user to enter (a menu). The menu prompts the user for one of three entries, then performs the selected task based on what they enter.
Suppose the user enters A to add a task. In that case, the program will wait for the user to type something in, take that input, and feed it to the add_task() function we created earlier to add a new task; the same goes for if the user types R as their input, except it runs the remove_task() function on the input instead.
If they choose D, the program displays the list of their tasks. If they enter anything else, the program prompts them that the input doesn’t fit any of the options on the menu.
This main function will continue to run indefinitely because we encapsulate it inside of a “while” loop.
Finally, the bottom line will start the main() function when we tell the program to run.
After you put these functions in, you can remove the testing code:
Testing and Iteration
You can find the final iteration of this code, including comments, on my GitHub if you’re interested. If you followed along properly, your output should look something like this:
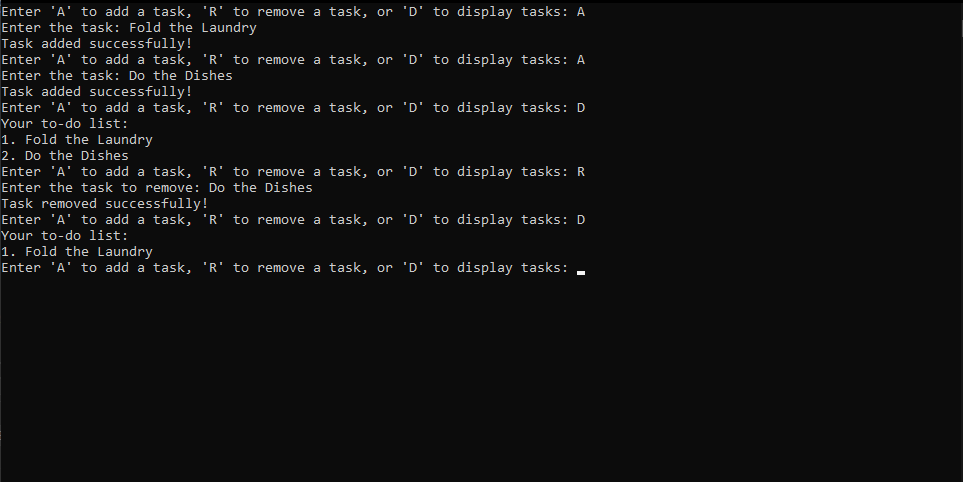
Feel free to experiment with adding and removing tasks. Congratulations on completing your first fully functional Python to-do list!
Where to Go From Here
As you’ve probably noticed, the interface for this application is minimal. It looks boring with a terminal or console entry. If you’d like a challenge, you can figure out how to build a GUI for this application or expand it to include things like editing the tasks. Each of these can help you learn Python programming a bit more by exploring more complex concepts.
Depending on what you want to do with this app, you can add or change things to make it more useful for you. That’s the joy in coding—you can take a simple idea and turn it into something that suits you perfectly.
- Programming
- Today's news
- Reviews and deals
- Climate change
- 2024 election
- Newsletters
- Fall allergies
- Health news
- Mental health
- Sexual health
- Family health
- So mini ways
- Unapologetically
- Buying guides
Entertainment
- How to Watch
- My watchlist
- Stock market
- Biden economy
- Personal finance
- Stocks: most active
- Stocks: gainers
- Stocks: losers
- Trending tickers
- World indices
- US Treasury bonds
- Top mutual funds
- Highest open interest
- Highest implied volatility
- Currency converter
- Basic materials
- Communication services
- Consumer cyclical
- Consumer defensive
- Financial services
- Industrials
- Real estate
- Mutual funds
- Credit cards
- Balance transfer cards
- Cash back cards
- Rewards cards
- Travel cards
- Online checking
- High-yield savings
- Money market
- Home equity loan
- Personal loans
- Student loans
- Options pit
- Fantasy football
- Pro Pick 'Em
- College Pick 'Em
- Fantasy baseball
- Fantasy hockey
- Fantasy basketball
- Download the app
- Daily fantasy
- Scores and schedules
- GameChannel
- World Baseball Classic
- Premier League
- CONCACAF League
- Champions League
- Motorsports
- Horse racing
New on Yahoo
- Privacy Dashboard
Python hunt: Florida has dangerous animals that can kill. Here's a list of the deadliest
Florida’s 10-day Python Challenge is underway as hunters take to the Everglades for glory, a $10,000 prize, and to help rid the state of its invasive Burmese python problem.
Wild Burmese python s can pose some risk to humans, even those who aren't purposefully pursuing them.
Traffic accidents involving large pythons crossing roads are one concern. A January 2023 report that compiled decades of python research also noted that there are potential risks, especially for children, coming into conflict with the monster constrictors at the edges of water.
For the general public, pythons are easy enough to avoid. They aren't venomous, but they are killers and known to consume large alligators and deer, sometimes to explosive effects .
More: Participants from all over the country and Canada come for Florida Python Challenge this week
They join many other animals in Florida — some native like the alligator, some invasive like the Burmese pythons — that pose risks to humans or can even kill you.
With a wide area of swamps, from the massive Everglades to major parks like Big Cypress Natural Preserve, to heavily wooded areas, to major lakes and canals, to the Atlantic coastline and Gulf of Mexico, Florida is a natural habitat for many creatures.
Some are cute, like key deer and North American river otters. Others are postcard-perfect, like flamingos and manatees.
But some can be deadly.
Here are just a few of them.
What's a list of Florida's deadliest creatures without alligators? These ancient monsters can certainly kill you and there have been many stories of serious injuries. Now they normally won't attack you if you aren't invading their territory .... then again, Florida is pretty much their territory.
One notable and tragic death occurred in 2016 at Disney's Grand Floridian Resort and Spa where a 2-year-old from Nebraska was snatched from the shore of a lake by a gator.
Yes, Florida has crocs as well as gators. But these guys, which normally are bigger than gators, are shy and reclusive and live mainly along the very southern reaches of the Florida coast in brackish or saltwater areas. These aren't the huge crocs you see on YouTube grabbing a water buffalo in Africa but you also wouldn't want to meet one in a dark, brackish, saltwater alley.
Sharks get a bad rap (Thank you Peter Benchley ) but truth is most shark bites don't kill people. Experts believe sharks have no taste for human flesh; they just confuse us with fish, and usually let go when they get a taste. While most sharks off Florida's coast aren't big (spinners and black tips), some bigger sharks like hammerheads , bulls and tigers do get close to shore at times. Is it safe to go in the water? The odds are in your favor.
Most shark bites in Florida are not deadly. However, there was an unfortunate 2010 attack where a 38-year-old Stuart man who was kitesurfing suffered multiple shark bites and died from his injuries. In 2023, Florida had the most unprovoked shark attacks in the U.S., accounting for 16 of the 36 cases in the country.
Of the 44 species of snakes in Florida, only six are venomous, according to the Florida Fish and Wildlife Conservation Commission. Those would be different species of cottonmouths and rattlesnakes and the small and colorful eastern coral snake, which happens to look very similar to the non-venomous king snake. If you're a Floridian you know the rhyme to differentiate between a king and coral snake which is which: "Red meets black, friend of Jack; red meets yellow, deadly fellow."
Anacondas, non-native constrictors like the python, also live in Florida, though there have been relatively few sightings: mostly around central and north central Florida, according to the FWC.
The biggest cats in Florida, they are 5 to 7 feet long and can weigh between 60 to 160 pounds. They live primarily south of Orlando and are endangered. But these felines aren't like lions on the savanna or tigers in the jungle. In fact, there's been only one instance of a likely panther attack in Florida; In 2014, a hunter said he was attacked . Because he was wearing multiple layers, he wasn't seriously injured.
These predators can live surprisingly close to dense population areas and they are related to wolves, but they're much smaller (18 to 44 pounds). They'll avoid humans at all costs, but small pets? While not part of their normal diet, they could become lunch for a coyote.
They're actually more ubiquitous in Florida than you'd think. They're not known to attack humans but it has happened. An elderly couple in Fort Lauderdale was attacked supposedly by a bobcat a few years ago, though they suffered minor injuries; bobcats, after all, aren't that big (between 25 and 35 pounds). They're actually common around urban apartment complexes because trash in outside receptacles attracts rats and other rodents, which bobcats love to eat.
VIDEO : A Florida lawyer who pushed a raccoon into the ocean may be in trouble with the Florida Bar
There are black bears all over Florida, but most live in the Panhandle, parts of north Florida, parts of central and the southwest. And they tend to be hard to catch sight of. While it's never smart to agitate any wildlife, we're lucky in that we likely have the least aggressive of the major bear species. But what should you do if you encounter a bear? Another rhyme to help you decide: "If it's black, fight back; if it's brown lay down (and play dead); if it's polar, forget it, it's over." So no polar bears. At least we have that going for us, which is nice.
Do you live near bears? Use this interactive map , provided by the Fish and Wildlife Conservation Commission.
Florida has all kinds, but bites aren't life-threatening, unless it's from the Black Widow, the most venomous spider in North America.
Florida's tropical climate is perfect for lizards, but none of our native or invasive lizards are venomous or big enough to seriously hurt you. But if you're new to the area, you may be surprised at the size of some of them: like iguanas, anoles, Argentine black and white tegus, nile monitors and Peter’s rock agama lizards.
The cane toad, also known as the bufo, giant or marine toad, is a big invasive amphibian that are poisonous to most animals that try to bite or eat them.
Their sting will get your attention. Some people can develop mild rashes; some who suffer extreme allergic reactions can even die. Just stay away from them, whether in the water or if you see them washed on the beach because those stingers could still be working.
Portuguese man-of-war
Portuguese man-of-war, so named for the battleship-shaped balloon that keeps them buoyant, have tentacles stacked with coiled, barbed tubes of venom that stream out as far as 100 feet from the man-of-war’s gas-filled sail. They can pack a sting that can swell lymph nodes, cause nausea, leave lines of red welts from their tentacles and cause trouble breathing.
Although often confused with a jellyfish, the man-of-war is actually a siphonophore, according to the National Oceanic and Atmospheric Administration. A siphonophore is comprised of different organisms with various functions all working together as one.
Florida has a lot of species of mosquitoes, and many can transmit diseases that can be life-threatening.
Mosquitoes can spread diseases such West Nile virus, dengue, or malaria.
The Centers for Disease Control and Prevention call mosquitoes the world's deadliest animal because each year they kill more people than any other creature.
Kimberly Miller is a veteran journalist for The Palm Beach Post, part of the USA Today Network of Florida. She covers weather, climate and the environment and has a certificate in Weather Forecasting from Penn State. Contact Kim by email at at [email protected] or on Twitter at @KmillerWeather.
This article originally appeared on Palm Beach Post: Snakes, alligators, sharks, pythons: Florida's most dangerous animals
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
//= | x //= 3 | x = x // 3 | |
**= | x **= 3 | x = x ** 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |
Related Pages

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Assignment Operators in Python
The Python Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, and bitwise computations. The value the operator operates on is known as the Operand. Here, we will cover Different Assignment operators in Python .
Operators |
| ||
---|---|---|---|
| = | Assign the value of the right side of the expression to the left side operand | c = a + b |
| += | Add right side operand with left side operand and then assign the result to left operand | a += b |
| -= | Subtract right side operand from left side operand and then assign the result to left operand | a -= b |
| *= | Multiply right operand with left operand and then assign the result to the left operand | a *= b |
| /= | Divide left operand with right operand and then assign the result to the left operand | a /= b |
| %= | Divides the left operand with the right operand and then assign the remainder to the left operand | a %= b |
| //= | Divide left operand with right operand and then assign the value(floor) to left operand | a //= b |
| **= | Calculate exponent(raise power) value using operands and then assign the result to left operand | a **= b |
| &= | Performs Bitwise AND on operands and assign the result to left operand | a &= b |
| |= | Performs Bitwise OR on operands and assign the value to left operand | a |= b |
| ^= | Performs Bitwise XOR on operands and assign the value to left operand | a ^= b |
| >>= | Performs Bitwise right shift on operands and assign the result to left operand | a >>= b |
| <<= | Performs Bitwise left shift on operands and assign the result to left operand | a <<= b |
| := | Assign a value to a variable within an expression | a := exp |
Here are the Assignment Operators in Python with examples.
Assignment Operator
Assignment Operators are used to assign values to variables. This operator is used to assign the value of the right side of the expression to the left side operand.
Addition Assignment Operator
The Addition Assignment Operator is used to add the right-hand side operand with the left-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the addition assignment operator which will first perform the addition operation and then assign the result to the variable on the left-hand side.
S ubtraction Assignment Operator
The Subtraction Assignment Operator is used to subtract the right-hand side operand from the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the subtraction assignment operator which will first perform the subtraction operation and then assign the result to the variable on the left-hand side.
M ultiplication Assignment Operator
The Multiplication Assignment Operator is used to multiply the right-hand side operand with the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the multiplication assignment operator which will first perform the multiplication operation and then assign the result to the variable on the left-hand side.
D ivision Assignment Operator
The Division Assignment Operator is used to divide the left-hand side operand with the right-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the division assignment operator which will first perform the division operation and then assign the result to the variable on the left-hand side.
M odulus Assignment Operator
The Modulus Assignment Operator is used to take the modulus, that is, it first divides the operands and then takes the remainder and assigns it to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the modulus assignment operator which will first perform the modulus operation and then assign the result to the variable on the left-hand side.
F loor Division Assignment Operator
The Floor Division Assignment Operator is used to divide the left operand with the right operand and then assigs the result(floor value) to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the floor division assignment operator which will first perform the floor division operation and then assign the result to the variable on the left-hand side.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator is used to calculate the exponent(raise power) value using operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the exponentiation assignment operator which will first perform exponent operation and then assign the result to the variable on the left-hand side.
Bitwise AND Assignment Operator
The Bitwise AND Assignment Operator is used to perform Bitwise AND operation on both operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise AND assignment operator which will first perform Bitwise AND operation and then assign the result to the variable on the left-hand side.
Bitwise OR Assignment Operator
The Bitwise OR Assignment Operator is used to perform Bitwise OR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise OR assignment operator which will first perform bitwise OR operation and then assign the result to the variable on the left-hand side.
Bitwise XOR Assignment Operator
The Bitwise XOR Assignment Operator is used to perform Bitwise XOR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise XOR assignment operator which will first perform bitwise XOR operation and then assign the result to the variable on the left-hand side.
Bitwise Right Shift Assignment Operator
The Bitwise Right Shift Assignment Operator is used to perform Bitwise Right Shift Operation on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise right shift assignment operator which will first perform bitwise right shift operation and then assign the result to the variable on the left-hand side.
Bitwise Left Shift Assignment Operator
The Bitwise Left Shift Assignment Operator is used to perform Bitwise Left Shift Opertator on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise left shift assignment operator which will first perform bitwise left shift operation and then assign the result to the variable on the left-hand side.
Walrus Operator
The Walrus Operator in Python is a new assignment operator which is introduced in Python version 3.8 and higher. This operator is used to assign a value to a variable within an expression.
Example: In this code, we have a Python list of integers. We have used Python Walrus assignment operator within the Python while loop . The operator will solve the expression on the right-hand side and assign the value to the left-hand side operand ‘x’ and then execute the remaining code.
Assignment Operators in Python – FAQs
What are assignment operators in python.
Assignment operators in Python are used to assign values to variables. These operators can also perform additional operations during the assignment. The basic assignment operator is = , which simply assigns the value of the right-hand operand to the left-hand operand. Other common assignment operators include += , -= , *= , /= , %= , and more, which perform an operation on the variable and then assign the result back to the variable.
What is the := Operator in Python?
The := operator, introduced in Python 3.8, is known as the “walrus operator”. It is an assignment expression, which means that it assigns values to variables as part of a larger expression. Its main benefit is that it allows you to assign values to variables within expressions, including within conditions of loops and if statements, thereby reducing the need for additional lines of code. Here’s an example: # Example of using the walrus operator in a while loop while (n := int(input("Enter a number (0 to stop): "))) != 0: print(f"You entered: {n}") This loop continues to prompt the user for input and immediately uses that input in both the condition check and the loop body.
What is the Assignment Operator in Structure?
In programming languages that use structures (like C or C++), the assignment operator = is used to copy values from one structure variable to another. Each member of the structure is copied from the source structure to the destination structure. Python, however, does not have a built-in concept of ‘structures’ as in C or C++; instead, similar functionality is achieved through classes or dictionaries.
What is the Assignment Operator in Python Dictionary?
In Python dictionaries, the assignment operator = is used to assign a new key-value pair to the dictionary or update the value of an existing key. Here’s how you might use it: my_dict = {} # Create an empty dictionary my_dict['key1'] = 'value1' # Assign a new key-value pair my_dict['key1'] = 'updated value' # Update the value of an existing key print(my_dict) # Output: {'key1': 'updated value'}
What is += and -= in Python?
The += and -= operators in Python are compound assignment operators. += adds the right-hand operand to the left-hand operand and assigns the result to the left-hand operand. Conversely, -= subtracts the right-hand operand from the left-hand operand and assigns the result to the left-hand operand. Here are examples of both: # Example of using += a = 5 a += 3 # Equivalent to a = a + 3 print(a) # Output: 8 # Example of using -= b = 10 b -= 4 # Equivalent to b = b - 4 print(b) # Output: 6 These operators make code more concise and are commonly used in loops and iterative data processing.
Please Login to comment...
Similar reads.
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Rays Recall Junior Caminero
- Dodgers’ River Ryan To Undergo Tommy John Surgery
- Mariners Sign Víctor Robles To Extension
- Dodgers Designate Amed Rosario For Assignment, Activate Mookie Betts
- Pirates Place Marco Gonzales On 60-Day IL With Forearm Strain
- Guardians To Activate Matthew Boyd
- Hoops Rumors
- Pro Football Rumors
- Pro Hockey Rumors
MLB Trade Rumors
Padres Reinstate Joe Musgrove, Designate Carl Edwards
By Anthony Franco | August 12, 2024 at 5:12pm CDT
As expected, the Padres reinstated Joe Musgrove from the 60-day injured list to start tonight’s game against the Pirates. San Diego designated reliever Carl Edwards Jr. for assignment to open space on both the active and 40-man rosters.
Musgrove has been sidelined since the end of May on account of elbow inflammation. This was his second elbow-related IL stint of the season. He hasn’t looked like himself around the injuries, struggling to a 5.66 ERA over 10 starts. His 92.4 MPH fastball speed was a bit below last season’s 93.1 MPH average. Opponents teed off on both his four-seam and cutter. Musgrove allowed nearly two home runs per nine innings and saw his strikeout rate fall to 20.6% — his lowest clip in six seasons.
After a few months away, Musgrove will try to recapture his 2021-23 form. The San Diego-area native combined for a 3.05 ERA across 459 2/3 innings over his first three seasons with his hometown club. While Musgrove’s 2023 campaign was cut short by a shoulder issue, he looked like a #2 or high-end #3 starter over the preceding two and a half seasons. He steps back into the Friars rotation as they look to at least hang onto a Wild Card spot. They currently occupy the second NL Wild Card spot and are four games clear of the #6 seed Braves. San Diego is tied with the Diamondbacks for second place in the NL West. They’re 3.5 games behind the Dodgers in the division.
Musgrove’s return nudges Randy Vásquez out of the rotation. San Diego already optioned Vásquez last week with the knowledge that their All-Star righty would get the ball tonight. Musgrove slots behind Dylan Cease and Michael King and in front of Matt Waldron and Martín Pérez in the starting five. The Padres have been without Yu Darvish for five weeks as he attends to a family matter. Dennis Lin of the Athletic reported this afternoon that Darvish set up a live batting practice session at a local high school over the weekend. It’s still not clear whether he’ll be able to return this season, but the veteran righty is keeping his arm in shape in case he can get back.
Edwards was just selected onto the MLB roster late last week. He pitched once and failed to retire any of three batters faced. The 32-year-old righty has otherwise spent this season in Triple-A. Splitting his time between the Cubs and Padres organizations, he owns a 3.30 ERA across 46 1/3 frames in the minors. His 22.2% strikeout rate and lofty 14.3% walk percentage aren’t great, though, so he hasn’t gotten much of an MLB opportunity this year.
San Diego will put Edwards on waivers in the next couple days. He has more than enough service time to decline a minor league assignment if he goes unclaimed, though he’d likely be limited to minor league offers if he elects free agency.
10 Comments

21 hours ago
I told you he was over but I didn’t think they’d figure it out this fast. Did he even pitch?
Once. 3 batters faced. 2 walks, 1 hit
Sounds about right.
18 hours ago
I thought you guys were talking about Darvish pitching against high school boys, and I thought, “Whoa, those three boys must feel great right now, and the rest of the team must be disappointed that he just stopped after three hitters.”
Yep 7,8,9 hitters and didn’t get an out.
20 hours ago
I expected him to be the DFA (and agree) option but really, I’m giving him a pass on that last outing due to his schedule. The guy was with the AAA team the night before, drove 2 hours to an airport in Texas at like 7 am to fly and arrive at the ballpark, I think around the 2nd inning.
Tough circumstances to at least not overlook when talking about his stat line is all I’m saying.

He had a rough go in 19 with the Padres as well. I’ve seen him be good other places. I hope he lands on his feet.
Darvish out since end of May. This August. Five weeks? Don’t tell me he’s gambling too…..
12 hours ago
No no…the other Japanese player who DHs
Sorry CARL, Back to NASCAR !!
Leave a Reply Cancel reply
Please login to leave a reply.
Log in Register

- Feeds by Team
- Commenting Policy
- Privacy Policy
MLB Trade Rumors is not affiliated with Major League Baseball, MLB or MLB.com
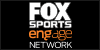
Username or Email Address
Remember Me
- SI SWIMSUIT
- SI SPORTSBOOK
Colorado Rockies' Charlie Condon Tops Draft Newcomers in Updated MLB Prospect Rankings
Sam connon | 1 hour ago.
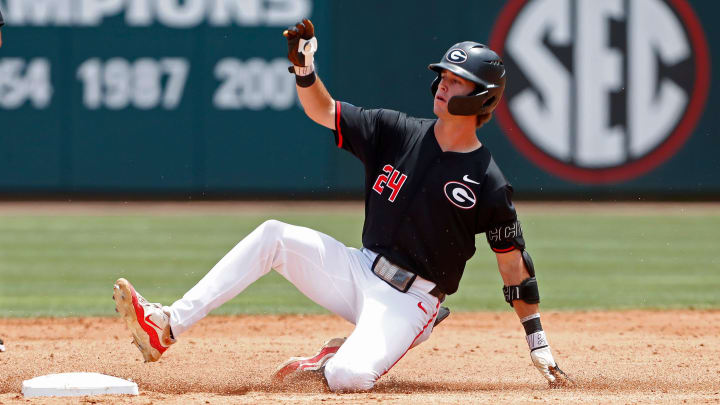
- Colorado Rockies
A month removed from the 2024 MLB Draft, Charlie Condon remains one of the most hyped young players in baseball.
MLB Pipeline updated its Top 100 Prospect Rankings on Wednesday, shifting players around and adding draft newcomers. Condon, who the Colorado Rockies selected No. 3 overall in July , debuted at No. 12.
That is higher than any other player who was taken in this year's draft. No. 1 overall pick Travis Bazzana, now a second baseman in the Cleveland Guardians' farm system, came in at No. 13.
New @MLBPipeline rankings have arrived! pic.twitter.com/yDewRAsRVo — MLB (@MLB) August 14, 2024
Condon is also the No. 1 prospect in the Rockies' system, leapfrogging right-handed pitcher Chase Dollander. Colorado selected Dollander No. 9 overall in the 2023 MLB Draft.
The Rockies gave Condon a $9.25 million signing bonus on July 19, which is tied for the largest in league history. The franchise then assigned the 21-year-old to High-A Spokane, where he is batting .280 with one home run, two RBI, two stolen bases and a .788 OPS through six games.
Condon has been spending most of his time in left field, but he has also started one game at third base and two at designated hitter. Across his two seasons at the University of Georgia, Condon played all three outfield positions, plus first and third base.
Initially a walk-on, Condon redshirted the 2022 campaign, then earned unanimous National Freshman of the Year honors in 2023. He swept the national honors in 2024, most notably winning SEC Player of the Year and the Golden Spikes Award.
In 116 games of NCAA action, Condon hit .410 with a 1.433 OPS. He racked up 181 hits, 62 home runs, 145 RBI and 401 total bases, recording more walks – 90 – than strikeouts – 86.
The Rockies now boast six prospects in the Top 100, which is tied for the most by any team. Outfielder Jordan Beck could be on the verge of graduating, though, considering he has 83 MLB at-bats under his belt and was recalled from Triple-A again on Monday.
Colorado's farm system ranks No. 10 in baseball with 205 prospect points.
Follow Fastball on FanNation on social media
Continue to follow our FanNation on SI coverage on social media by liking us on Facebook and by following us on Twitter @FastballFN .
You can also follow Sam Connon on Twitter @SamConnon .
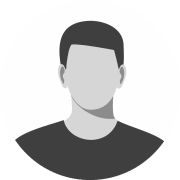
Sam Connon is a Staff Writer for Fastball on the Sports Illustrated/FanNation networks. He previously covered UCLA Athletics for Sports Illustrated/FanNation's All Bruins, 247Sports' Bruin Report Online, Rivals' Bruin Blitz, the Bleav Podcast Network and the Daily Bruin, with his work as a sports columnist receiving awards from the College Media Association and Society of Professional Journalists. Connon also wrote for Sports Illustrated/FanNation's New England Patriots site, Patriots Country, and he was on the Patriots and Boston Red Sox beats at Prime Time Sports Talk.
Follow SamConnon
Phillies reinstate Walker from injured list; option Marte to Lehigh Valley
Prior to tonight’s game against the Miami Marlins, the Phillies returned right-handed pitcher Taijuan Walker from his rehab assignment with double-A Reading and reinstated him from the 15-day injured list. To make room for him on the 26-man roster, right-handed pitcher Yunior Marte was optioned to triple-A Lehigh Valley following Sunday’s game.
Phillies President of Baseball Operations Dave Dombrowski made the announcement.
Walker , 32, went 3-3 with a 5.60 ERA (33 ER, 53.0 IP) and 43 strikeouts over his first 10 starts for the Phillies this season. He made two rehab starts with Reading and combined for a 3.52 ERA (3 ER, 7.2 IP) with six strikeouts to one walk and a 0.91 WHIP (6 hits). Walker was placed on the injured list with right index finger inflammation on June 23 (retroactive to June 22).
Originally selected by the Seattle Mariners in the first round (43 rd overall) of the 2010 MLB Draft, Walker was signed by the Phillies as a free agent on Dec. 16, 2022.
Marte , 29, has a 7.13 ERA (19 ER, 24.0 IP) in his 22 relief appearances for the Phillies this season. Originally signed by the Kansas City Royals as an amateur free agent on July 19, 2012, he was acquired by the Phillies from the San Francisco Giants on Jan. 8, 2023.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Python list of lists assignment
One thing first: No, this is not a question about why
gives [1,1,1,1,1,1,1,1,1,1].
My question is a bit more tricky: I do the folling:
Now I assign a value:
And now another one:
It gives me an IndexError: list assignment index out of range.
How can I make this work? As you can see, I want an empty list that contains 3 other lists to which i can append values.
Thanks in advance!

- 1 Did you look at what x[0] = 1 actually does? It replaces one of your nested lists with the number 1. If you want to append values, you should use append , not item assignment. – BrenBarn Commented Apr 29, 2014 at 8:19
- yes you are right. but this is only an example. in my implementation, i do use append ;) – PKlumpp Commented Apr 29, 2014 at 8:31
4 Answers 4
I want an empty list that contains 3 other lists to which i can append values.
Your problem correctly stated is: "I want a list of three empty lists" (something cannot be empty and contain things at the same time).
If you want that, why are you doing it in such a convoluted way? What's wrong with the straightforward approach:
If you have such a list, when you do empty_list[0] = 1 , what you end up with is:
Instead, you should do empty_list[0].append(1) , which will give you:
If you want to create an arbitrary number of nested lists:
- good question! the answer is simple: my 3 lists in one list were only an example. originally, i wanted to put 15 lists into a list. of course, your way is working, but it looks somewhat weird if i implement it that way. i just wanted to learn if there is an advanced way of doing this. but thanks ;) – PKlumpp Commented Apr 29, 2014 at 8:29
gives [1,1,1,1,1,1,1,1,1,1] ? It's an index error (you can't assign at a nonexisting index), because actually []*10 == [] . Your code does exactly the same (I mean an index error). This line
is short (or not) for
so x[1] is an index error.
- sry, you are right, i forgot brackets there. Yes i know the index does not exist, that is what the error message already told me. but how do i solve my problem? – PKlumpp Commented Apr 29, 2014 at 8:27
- @ZerO I want an empty list that contains 3 other lists I don't understand - it can be empty and contain something at the same time. If you want to append something to a list, then use .append . See Burhan's answer. – freakish Commented Apr 29, 2014 at 9:04
Maybe something like this could work.
The output would be:
If you would like to access/add elements, you could:
- yes, this does work. but i need a multi-assignment ;) – PKlumpp Commented Apr 29, 2014 at 11:35
Comparing the output of:
may help answer your finding.
- are you kidding me? Did you even read my question? I think i clearly showed that this is NOT the problem -.- – PKlumpp Commented Apr 30, 2014 at 6:56
- @ZerO: Did you even try the above code? I see no bearing on your question apart from the subtle but significant difference shown by the above. You have a 10 in your first example, and a 3 in your second. Why? If you already knew the difference between multiplication of lists versus generators, then print i and print i[0] and print i[1] as well and see why you are getting an IndexError . I am on SO thinking I like to learn and help, and I may be wrong in my answer. – vapace Commented Apr 30, 2014 at 16:32
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python list or ask your own question .
- The Overflow Blog
- Scaling systems to manage all the metadata ABOUT the data
- Navigating cities of code with Norris Numbers
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Tag hover experiment wrap-up and next steps
Hot Network Questions
- Transit from intl to domestic in Zurich on Swiss
- How can I cross an overpass in "Street View" without being dropped to the roadway below?
- Can this Integer Linear Programming problem be solved in polynomial time?
- Density of perfect numbers
- Would several years of appointment as a lecturer hurt you when you decide to go for a tenure-track position later on?
- "Not many can say that is there."
- Why do instructions for various goods sold in EU nowadays lack pages in English?
- I need to better understand this clause in an independent contract agreement for Waiverability:
- Will The Cluster World hold onto an atmosphere for a useful length of time without further intervention?
- How to follow Outside-In TDD with Micro-services and Micro-frontends?
- Automotive Controller LDO Failures
- Why did Borland ignore the Macintosh market?
- Do space stations have anything that big spacecraft (such as the Space Shuttle and SpaceX Starship) don't have?
- In zsh, annotate each line in a file to which both stdout and stderr have been redirected with the line's source (stdout or stderr)
- Decent 900 MHz radome material from a hardware store
- Functional derivative under a path integral sign
- Could a 3D sphere of fifths reveal more insights than the 2D circle of fifths?
- The minimal Anti-Sudoku
- What is this fruit from Cambodia? (orange skin, 3cm, orange juicy flesh, very stick white substance)
- What are those bars in subway train or bus called?
- How to define a function in Verilog?
- Why didn't Walter White choose to work at Gray Matter instead of becoming a drug lord in Breaking Bad?
- Why would luck magic become obsolete in the modern era?
- Should I pay off my mortgage if the cash is available?
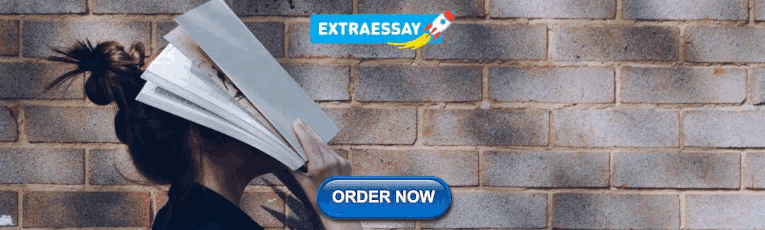
COMMENTS
List. Lists are used to store multiple items in a single variable. Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple, Set, and Dictionary, all with different qualities and usage. Lists are created using square brackets:
Python's list is a flexible, versatile, powerful, and popular built-in data type. It allows you to create variable-length and mutable sequences of objects. In a list, you can store objects of any type. You can also mix objects of different types within the same list, although list elements often share the same type.
Learn how to work with Python lists. This covers all your needs, like append, remove, sort, replace, reverse, convert, and so on.
139 Python docs says that slicing a list returns a new list. Now if a "new" list is being returned I've the following questions related to "Assignment to slices" a = [1, 2, 3] a[0:2] = [4, 5] print a
Python list is a built-in data structure in Python used to store a collection of items. Lists are mutable, means they can be changed after they are created.
Python lists store multiple data together in a single variable. In this tutorial, we will learn about Python lists (creating lists, changing list items, removing items, and other list operations) with the help of examples.
list = ['larry', 'curly', 'moe'] The for/in constructs are very commonly used in Python code and work on data types other than list, so you should just memorize their syntax. You may have habits from other languages where you start manually iterating over a collection, where in Python you should just use for/in.
Learn how to use Python's assignment operator to create, initialize, and update variables in your code. Explore different types of assignment statements and best practices.
Python list is an ordered sequence of items. In this article you will learn the different methods of creating a list, adding, modifying, and deleting elements in the list. Also, learn how to iterate the list and access the elements in the list in detail. Nested Lists and List Comprehension are also discussed in detail with examples.
Lists and tuples are arguably Python's most versatile, useful data types. You will find them in virtually every nontrivial Python program. Here's what you'll learn in this tutorial: You'll cover the important characteristics of lists and tuples. You'll learn how to define them and how to manipulate them.
Data Structures — Python 3.12.5 documentation. 5. Data Structures ¶. This chapter describes some things you've learned about already in more detail, and adds some new things as well. 5.1. More on Lists ¶. The list data type has some more methods. Here are all of the methods of list objects:
This Python list exercise contains list programs and questions for practice. This exercise contains 10 Python list questions with solutions.
We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object.
Create a List of Lists Using append () Function. In this example the code initializes an empty list called `list_of_lists` and appends three lists using append () function to it, forming a 2D list. The resulting structure is then printed using the `print` statement. Python.
There is one special case: an assignment expression occurring in a list, set or dict comprehension or in a generator expression (below collectively referred to as "comprehensions") binds the target in the containing scope, honoring a nonlocal or global declaration for the target in that scope, if one exists.
Python is a versatile language, and even beginners can design and implement something cool with only basic knowledge. We'll look at developing a simple to-do list app with Python that you can actually use when you're done!
Python employs assignment unpacking when you have an iterable being assigned to multiple variables like above. In Python3.x this has been extended, as you can also unpack to a number of variables that is less than the length of the iterable using the star operator: >>> a,b,*c = [1,2,3,4] >>> a. 1. >>> b. 2. >>> c.
There are many deadly animals in Florida, the Everglades. Facts on these dangerous animals: Alligators, sharks, panthers, snakes, spiders and pythons.
Twins shortstop Carlos Correa has started doing sprints, but he told reporters "it's not quite where we want it to be yet" when it comes to beginning a rehab assignment. Carlos Correa said ...
Python Assignment Operators. Assignment operators are used to assign values to variables: Operator. Example. Same As. Try it. =. x = 5. x = 5.
Outfielder Eli White was optioned to Triple-A to open an active roster spot while right-hander Parker Dunshee was designated for assignment in a corresponding 40-man move.
The Python Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, and bitwise computations. The value the operator operates on is known as the Operand. Here, we will cover Different Assignment operators in Python.
As expected, the Padres reinstated Joe Musgrove from the 60-day injured list to start tonight's game against the Pirates. San Diego designated reliever Carl Edwards Jr. for assignment to open ...
The Cardinals designate a former Dodgers relief pitcher for assignment after activating Riley O'Brien from the injured list. Tuesday, the Angels claimed him off waivers.
Your list starts out empty because of this: a = [] then you add 2 elements to it, with this code: a.append(3) a.append(7) this makes the size of the list just big enough to hold 2 elements, the two you added, which has an index of 0 and 1 (python lists are 0-based). In your code, further down, you then specify the contents of element j which ...
The Los Angeles Dodgers have designated infielder Amed Rosario for assignment, the team announced Monday afternoon. Rosario lost his roster spot to eight-time All-Star Mookie Betts, who was ...
In python, a variable name is a reference to the underlying variable. Both list1 and list2 refer to the same list, so when you insert 9 into that list, you see the change in both.
Charlie Condon, who the Colorado Rockies selected with the No. 3 overall pick in the 2024 MLB Draft, has been pegged as the No. 12 prospect in all of baseball.
Prior to tonight's game against the Miami Marlins, the Phillies returned right-handed pitcher Taijuan Walker from his rehab assignment with double-A Reading and reinstated him from the 15-day injured list. To make room for him on the 26-man roster, right-handed pitcher Yunior Marte was optioned to triple-A Lehigh Valley following
Python list of lists assignment Asked 10 years, 3 months ago Modified 10 years, 3 months ago Viewed 7k times