
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
- Pointer Arithmetics in C with Examples
- Applications of Pointers in C
- Passing Pointers to Functions in C
- C - Pointer to Pointer (Double Pointer)
- Chain of Pointers in C with Examples
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
NULL Pointer in C
Dangling, void , null and wild pointers in c.
- Near, Far and Huge Pointers in C
- restrict keyword in C
In C programming pointers are used to manipulate memory addresses, to store the address of some variable or memory location. But certain situations and characteristics related to pointers become challenging in terms of memory safety and program behavior these include Dangling ( when pointing to deallocated memory ) , Void ( pointing to some data location that doesn’t have any specific type), Null ( absence of a valid address ) , and Wild ( uninitialized ) pointers.
Dangling Pointer in C
A pointer pointing to a memory location that has been deleted (or freed) is called a dangling pointer. Such a situation can lead to unexpected behavior in the program and also serve as a source of bugs in C programs.
There are three different ways where a pointer acts as a dangling pointer:
1. De-allocation of Memory
When a memory pointed by a pointer is deallocated the pointer becomes a dangling pointer.
The below program demonstrates the deallocation of a memory pointed by ptr.
2. Function Call
When the local variable is not static and the function returns a pointer to that local variable. The pointer pointing to the local variable becomes dangling pointer.
The below example demonstrates a dangling pointer when the local variable is not static.
In the above example, p becomes dangling as the local variable (x) is destroyed as soon as the value is returned by the pointer. This can be solved by declaring the variable x as a static variable as shown in the below example.
3. Variable Goes Out of Scope
When a variable goes out of scope the pointer pointing to that variable becomes a dangling pointer.
Void Pointer in C
Void pointer is a specific pointer type – void * – a pointer that points to some data location in storage, which doesn’t have any specific type. Void refers to the type. Basically, the type of data that it points to can be any. Any pointer type is convertible to a void pointer hence it can point to any value.
Note: Void pointers cannot be dereferenced. It can however be done using typecasting the void pointer. Pointer arithmetic is not possible on pointers of void due to lack of concrete value and thus size.
The below program shows the use void pointer as it is convertible to any pointer type.
To know more refer to the void pointer article
NULL Pointer is a pointer that is pointing to nothing(i.e. not pointing to any valid object or memory location). In case, if we don’t have an address to be assigned to a pointer, then we can simply use NULL. NULL is used to represent that there is no valid memory address.
The below example demonstrates the value of the NULL pointer.
Note NULL vs Uninitialized pointe r – An uninitialized pointer stores an undefined value. A null pointer stores a defined value, but one that is defined by the environment to not be a valid address for any member or object. NULL vs Void Pointer – Null pointer is a value, while void pointer is a type
Wild pointer in C
A pointer that has not been initialized to anything (not even NULL) is known as a wild pointer . The pointer may be initialized to a non-NULL garbage value that may not be a valid address.
The below example demonstrates the undefined behavior of the Wild pointer.
If you like GeeksforGeeks and would like to contribute, you can also write an article using write.geeksforgeeks.org or mail your article to [email protected]. See your article appearing on the GeeksforGeeks main page and help other Geeks. Please write comments if you find anything incorrect, or if you want to share more information about the topic discussed above.
Please Login to comment...
Similar reads.
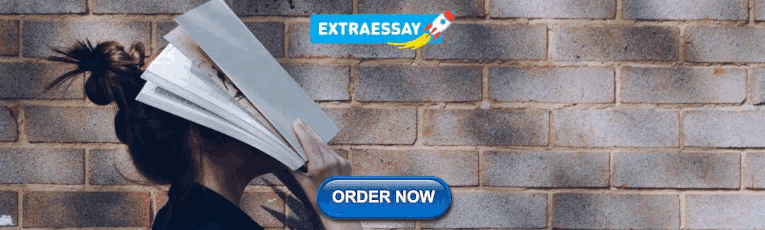
Improve your Coding Skills with Practice

What kind of Experience do you want to share?
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Bad pointer null assignment followed by malloc fail -- WHY?
I'm stumped! In dictionary.c I have coded the load function (with more code that follows the bug, but not needed for this question.) One small block of code is producing a problem! Assigning NULL to a pointer is producing an error that only shows up in valgrind. Every malloc that follows it will then fail in gdb.
Two questions:
1.) Why is the NULL assignment failing? What is bad about the assignment code? Why is it writing 4 bytes beyond the end of a block of size 4... what, the pointer? Could it be because my computer is a 64 bit machine? ( a 4-byte overwrite is 32 bits.)
2.) Why is this causing subsequent malloc commands to fail?
The line that is causing the grief is this:
The error I get is this:
In another experiment, I replaced the loop with multiple individual lines to assign each pointer to NULL. I discovered that as little as 3 assignments will produce the same failure.
Even with only one or two of the above lines, valgrind tells me the following about each one:
So, I know where the problem is, but I have no idea what the problem is!! Can anyone clue me in? Here is a more complete block of code that can be plugged into your dictionary.c file if you'd like to experiment.It contains only the code needed to duplicate the problem. (If you can't dupe the problem, let me know.)

The problem is because you're allocating the wrong size for root
the size of root is typically 4 bytes on 32-bit systems. You actually need to allocate sizeof node which is typically 1 (for is_word ) + 27 * 4 (for children ) + 3 (padding) = 112 bytes.
- That was it! Just goes to show how easy it is to make a simple mistake and not be able to see it at all! THANK YOU! I just wasn't seeing it, but knew I was dancing all around it! – Cliff B Commented Mar 25, 2015 at 3:18
You must log in to answer this question.
Not the answer you're looking for browse other questions tagged pset5 pointers malloc null ..
- Featured on Meta
- Upcoming sign-up experiments related to tags
Hot Network Questions
- In the US, are employers liable for torts caused by their employees working from home
- Is "Shopping malls are a posh place" grammatical when "malls" is a plural and "place" is a singular?
- Why is MSS important? Why can't we just rely on the MTU?
- Question about the sum of odd powers equation
- why std::is_same<int, *(int*)>::value is false?
- Why does setting a variable readonly in the outer scope prevents defining a local variable with the same name?
- Bringing a game console into Saudi Arabia
- Binding to an IP address on an interface that comes and goes
- Would a series of gravitational waves from a supernova affect time on a 200 year old clock just as water waves affected clocks on ships in rough seas?
- Is parapsychology a science?
- What did Jesus mean about the Temple as the Father's House in John 2:16?
- Is it better to freeze meat in butcher paper?
- What is the time-travel story where "ugly chickens" are trapped in the past and brought to the present to become ingredients for a soup?
- How often do snap elections end up in favor of the side that triggered them?
- Audio amplifier for school project
- If the supernatural were real, would we be able to study it scientifically?
- Schengen visa issued by Germany - Is a layover in Vienna okay before heading to Berlin?
- How does gravity overpower a vacuum?
- Origin of "That tracks" to mean "That makes sense."
- How do I know how many enemies have I not found in the Hunter's Journal?
- How to implement separate compilation?
- Is it possible to avoid ending Time Stop by making attacks from inside an Antimagic Field?
- What is this tool shown to us?
- The smell of wet gypsum

What does the error 'Null Pointer Assignment' mean?

The null pointer assignment error means your program has attempted to access a memory address that does not belong to your program. This typically occurs when accessing memory indirectly through a pointer:
int* p = nullptr; *p = 42; // Error: null pointer assignment
The above is the classic example of this type of error. The null address is typically the all-zeroes address (0x0) but, regardless of the physical address, it must never be accessed because it is a system address. We typically refer pointers to the null address when they are no longer in use or we don't have an address we can (yet) assign to them.
Passing unchecked pointers to functions is another common cause:
void f (int* p) {
*p = 42; // potential error
In the above example there's no guarantee p refers to a non-system address. Although we can easily test p is non-null before accessing it, that won't guarantee p refers to a non-system address. However, we can greatly reduce the risk of error by passing memory address via references instead of pointers:
void f (int& r) {
There's still potential that r refers to a system address if the address were passed via a pointer, however there is seldom any need to use unchecked pointer variables in C++. References and resource handles (or smart pointers) eliminate the need for pointers and are actually more efficient than pointers because testing for null becomes largely redundant.
The only time we really need a pointer is when "no object" is a valid argument:
if (p == nullptr) {
// the "no object" code
// code that operates on an object
Add your answer:

What is the difference between null and void pointers?
A void pointer is a pointer that has no type information attached to it.A null pointer is a pointer that points to "nothing". A null pointer can be of any type (void included, of course).
If null is compared with null what is the result-true or false or null or unknown?
You mean SQL? NULL = anything IS NULL NULL <> anything IS NULL ... NULL IS NULL = TRUE NULL IS NOT NULL = FALSE
Meaning c plus plus pointer to an array and pointer to a structure?
They both mean the same thing; an array is a type of data structure (a linear structure). A pointer variable is just a variable like any other, but one that is used to specifically store a memory address. That memory address may contain a primitive data type, an array or other data structure, an object or a function. The type of the pointer determines how the data being pointed at is to be treated. Pointers must always be initialised before they are accessed, and those that are not specifically pointing at any reference should always be zeroed or nullified with the NULL value. This ensures that any non-NULL pointer is pointing at something valid. Remember that pointer variables are no different to any other variable insofar as they occupy memory of their own, and can therefore point to other pointer variables.
What is an uninitialized pointer?
It means "nothing", there is no data provided at all; just an empty value. Contrary to the previous edit it does not mean "zero", "none" or "blank"; as zero is a number and none and blank can be regarded as data.
What does pointer to pointer finger mean?
yea that's why its called the point FINGER

Top Categories

|
| | | | | | | | What is a null pointer assignment error? What are bus errors, memory faults, and core dumps? ) These are all serious errors, symptoms of a wild pointer or subscript. Null pointer assignment is a message you might get when an MS-DOS program finishes executing. Some such programs can arrange for a small amount of memory to be available �where the NULL pointer points to (so to speak). If the program tries to write to that area, it will overwrite the data put there by the compiler. When the program is done, code generated by the compiler examines that area. If that data has been changed, the compiler-generated code complains with null pointer assignment. This message carries only enough information to get you worried. There�s no way to tell, just from a null pointer assignment message, what part of your program is responsible for the error. Some debuggers, and some compilers, can give you more help in finding the problem. Bus error: core dumped and Memory fault: core dumped are messages you might see from a program running under UNIX. They�re more programmer friendly. Both mean that a pointer or an array subscript was wildly out of bounds. You can get these messages on a read or on a write. They aren�t restricted to null pointer problems. The core dumped part of the message is telling you about a file, called core, that has just been written in your current directory. This is a dump of everything on the stack and in the heap at the time the program was running. With the help of a debugger, you can use the core dump to find where the bad pointer was used. That might not tell you why the pointer was bad, but it�s a step in the right direction. If you don�t have write permission in the current directory, you won�t get a core file, or the core dumped message. ) Other Interview Questions | - Help Center Home
- API Documentation
- Related Resources
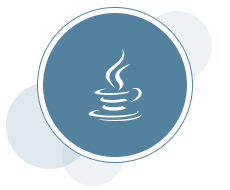 JDK 22 DocumentationJava Components page Looking for a different release? Other releases  - Release Notes
- Migration Guide
- Download the JDK
- Installation Guide
- Version-String Format
 - JDK Tool Specifications
- JShell User's Guide
- JavaDoc Guide
- Packaging Tool User Guide
 Language and Libraries- Language Updates
- Core Libraries
- JDK HTTP Client
- Java Tutorials
- Modular JDK
- Flight Recorder API Programmer’s Guide
- Internationalization Guide
 Specifications- Language and VM
- Java Security Standard Algorithm Names
- Java Native Interface (JNI)
- JVM Tool Interface (JVM TI)
- Serialization
- Java Debug Wire Protocol (JDWP)
- Documentation Comment Specification for the Standard Doclet
- Other specifications
 - Secure Coding Guidelines
- Security Guide
 HotSpot Virtual Machine- Java Virtual Machine Guide
- Garbage Collection Tuning
 Manage and Troubleshoot- Troubleshooting Guide
- Monitoring and Management Guide
 Client Technologies- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack OverflowFind centralized, trusted content and collaborate around the technologies you use most. Q&A for work Connect and share knowledge within a single location that is structured and easy to search. Get early access and see previews of new features. Assignment makes integer from pointer without a cast [-Werror]I keep getting this error message in response to hashtable[i]: assignment makes integer from pointer without a cast [-Werror]  2 Answers 2If hashtable is an array of integers then hashtable[i] expects an integer and NULL is a pointer. So you're trying to assign a pointer value to an integer variable(without a cast), this is usually just a warning but since you have -Werror all warnings turn into errors. Just use 0 instead of NULL .  - Ah, I see. I could also declare an array of pointers and store the value that way. I get it now. Thanks! – hannah Commented Jul 28, 2012 at 2:53
NULL is defined as (void*)0 in stddef.h If the hashtable is integer array, like this warning: assignment makes integer from pointer without a cast will be shown.  - What makes you believe the OP uses Linux? What makes you believe NULL must be defined in this particular way? Note that making assumptions by extrapolation from one system makes you in for surprises. Instead, reading and citing the ISO C Standard is likely to provide definitive answers. Such answers are independent of OS and header contents. – Jens Commented Jul 28, 2012 at 12:27
- 1 @Jens, Thanks. I will try to follow ISO C std :) – Jeyaram Commented Jul 29, 2012 at 3:48
Your AnswerReminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more Sign up or log inPost as a guest. Required, but never shown By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy . Not the answer you're looking for? Browse other questions tagged c int or ask your own question .- Featured on Meta
- Upcoming sign-up experiments related to tags
- Policy: Generative AI (e.g., ChatGPT) is banned
- The return of Staging Ground to Stack Overflow
- The 2024 Developer Survey Is Live
Hot Network Questions- Scheme interpreter in C
- 9-16-25 2D Matrix
- How to run the qiskit sampler after storing measurement results on classical qubits?
- Is this homebrew "Firemind Dragonborn" race overpowered?
- How to calculate velocity of air behind a propeller?
- What's this plant with saw-toothed leaves, scaly stems and white pom-pom flowers?
- Is it better to freeze meat in butcher paper?
- Is there a way knowledge checks can be done without an Intelligence trait?
- How to make a place windy
- What was God's original plan for humanity prior to the fall?
- Round Cake Pan with Parchment Paper
- In the US, are employers liable for torts caused by their employees working from home
- Why can't I connect a hose to this outdoor faucet?
- Is "Shopping malls are a posh place" grammatical when "malls" is a plural and "place" is a singular?
- Why was the 1540 a computer in its own right?
- Hubble gyro manufacturrer
- Aligning surveyed point layers in QGIS
- How does this tensegrity table work?
- Roadmap for self study in philosophy
- Are fiber glass perfboards more durable than phenolic perfboards?
- I buy retrocomputing devices, but they set my allergies off. Any advice on sanitizing new acquisitions?
- Parts of Humans
- What is the time-travel story where "ugly chickens" are trapped in the past and brought to the present to become ingredients for a soup?
- End Punctuation Checking using LuaLaTeX (like Reg-Ex)
 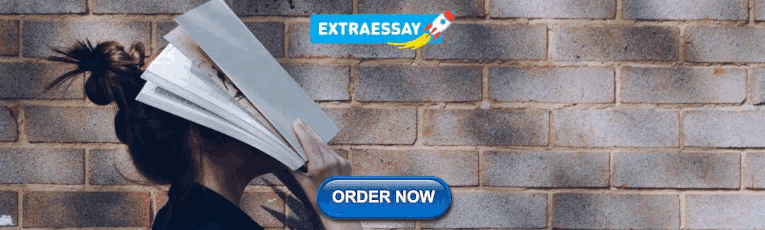 | | |
IMAGES
VIDEO
COMMENTS
A null pointer assignment error, or many other errors, can be assigned to this issue and example. In simpler architecture or programming environments, It can refer to any code which unintentionally ends up creating nulls as pointers, or creates a bug that in anyway halts the execution, like overwriting a byte in the return stack, overwriting ...
A null pointer is one that points to nowhere. When you dereference a pointer p, you say "give me the data at the location stored in "p". When p is a null pointer, the location stored in p is nowhere, you're saying "give me the data at the location 'nowhere'". Obviously, it can't do this, so it throws a null pointer exception.
NULL Pointer in C. The Null Pointer is the pointer that does not point to any location but NULL. According to C11 standard: "An integer constant expression with the value 0, or such an expression cast to type void *, is called a null pointer constant. If a null pointer constant is converted to a pointer type, the resulting pointer, called a ...
Technical Information Database TI500C.txt Null Pointer Assignment Errors Explained Category :General Platform :All Product :Borland C++ All Description: 1.
A null value (often shortened to null) is a special value that means something has no value. When a pointer is holding a null value, it means the pointer is not pointing at anything. Such a pointer is called a null pointer. The easiest way to create a null pointer is to use value initialization:
Assigning a pointer a value of 0x0 indicates that it is currently not pointing to any valid memory location. It represents a null pointer, signifying the absence of an object or data reference. It's important to note that the address zero is typically reserved and not accessible for storing valid data.
A NULL Pointer in C++ indicates the absence of a valid memory address in C++. It tells that the pointer is not pointing to any valid memory location In other words, it has the value "NULL" (or 'nullptr' since C++11). This is generally done at the time of variable declaration to check whether the pointer points to some valid memory ...
These are certain reasons for Null Pointer Exception as mentioned below: Invoking a method from a null object. Accessing or modifying a null object's field. Taking the length of null, as if it were an array. Accessing or modifying the slots of null objects, as if it were an array. Throwing null, as if it were a Throwable value.
NULL pointer in C - A null pointer is a pointer which points nothing.Some uses of the null pointer are:a) To initialize a pointer variable when that pointer variable isn't assigned any valid memory address yet.b) To pass a null pointer to a function argument when we don't want to pass any valid memory address.c) To.
C++ Null Pointers. It is always a good practice to assign the pointer NULL to a pointer variable in case you do not have exact address to be assigned. This is done at the time of variable declaration. A pointer that is assigned NULL is called a null pointer. The NULL pointer is a constant with a value of zero defined in several standard ...
Null pointer assignment is a message you might get when an MS-DOS program finishes executing. Some such programs can arrange for a small amount of memory to be available where the NULL pointer points to (so to speak). If the program tries to write to that area, it will overwrite the data put there by the compiler.
Null pointer. In computing, a null pointer or null reference is a value saved for indicating that the pointer or reference does not refer to a valid object. Programs routinely use null pointers to represent conditions such as the end of a list of unknown length or the failure to perform some action; this use of null pointers can be compared to ...
In the above code, we create a pointer *ptr and assigns a NULL value to the pointer, which means that it does not point any variable. After creating a pointer variable, we add the condition in which we check whether the value of a pointer is null or not. When we use the malloc () function. #include <stdio.h>.
A null pointer stores a defined value, but one that is defined by the environment to not be a valid address for any member or object. NULL vs Void Pointer - Null pointer is a value, while void pointer is a type. Wild pointer in C. A pointer that has not been initialized to anything (not even NULL) is known as a wild pointer. The pointer may ...
This error message means that somewhere in your program you have used a pointer-varible containing NULL-value. (Within an actual OS it with stop the program ...
In another experiment, I replaced the loop with multiple individual lines to assign each pointer to NULL. I discovered that as little as 3 assignments will produce the same failure. // replacing the FOR loop with these 3 lines will cause the same problem. root->children[0] = NULL; root->children[1] = NULL; root->children[2] = NULL;
The line *a = s1; copies s1 to the memory a is pointing to, although you didn't allocate any memory.. I think your actual intent was to load a with the address of s1:. a = &s1; You need to provide the address of an object to scanf to read from stdin:. scanf("%d",&a->roll_no); The line. scanf("%s",a->name);
The null pointer assignment error means your program has attempted to access a memory address that does not belong to your program. This typically occurs when ...
What is a null pointer assignment error? What are bus errors, memory faults, and core dumps? These are all serious errors, symptoms of a wild pointer or subscript. Null pointer assignment is a message you might get when an MS-DOS program finishes executing. Some such programs can arrange for a small amount of memory to be available "where the ...
Secure Coding Guidelines. Security Guide. Java Virtual Machine Guide. Garbage Collection Tuning. Troubleshooting Guide. Monitoring and Management Guide. JMX Guide. Java Accessibility Guide. The documentation for JDK 22 includes developer guides, API documentation, and release notes.
I using a old version of Borland for C lang. At the beginning of the program you enter the name (full name, FIO ), then 4 digits (as grades). The program calculates the average among 5 entered FIO and back a average number. #include <stdio.h>.
c error: assignment makes integer from pointer without a cast [-Werror=int-conversion] 0 assignment makes integer from pointer without a cast [-Werror=int-conversion]