
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- Stack Overflow Public questions & answers
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Talent Build your employer brand
- Advertising Reach developers & technologists worldwide
- Labs The future of collective knowledge sharing
- About the company
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How to Encode a String into a Valid Python Variable Name and Decode It Back Efficiently?
I need to encode arbitrary strings into valid Python variable names and be able to decode them back to the original strings. The strings may contain any characters, including those that are not valid in Python variable names.
Here are the requirements:
Valid Python Variable Name: The encoded string must be a valid Python variable name, which means it can only contain letters, digits, and underscores, and must start with a letter or an underscore. Reversible: The encoding should be reversible, allowing me to decode the variable name back to the original string. Efficiency: The encoding and decoding process should be efficient enough for use in a server application. I’ve tried using base64 encoding but ran into issues with characters like - and _. I also considered using werkzeug.security to hash the string, but it’s too slow for my needs in a server application. Here’s the solution I’ve come up with so far:
Considerations: Werkzeug Security: I considered using werkzeug.security for hashing the string to ensure a valid variable name, but it proved to be too slow for my server application. Questions: Is this approach reliable for all possible input strings? Are there any edge cases I might have missed that could cause the encoded variable name to be invalid? Is there a more efficient method to achieve the same goal? Any suggestions or improvements would be greatly appreciated!

- Please visit the help center to remind yourself how to ask good questions here. For starters, a question should contain ONE question, not many. Also note: this site isn't a "here are my requirements, here is my code, is that good" service. In other words: your question boils down to "can someone help with my assignment", and that isn't a legit question around here. – GhostCat Commented May 17 at 14:10
- 4 Why are you messing around with encoding, or variable names at all? ANY string works just fine as-is, as a key in a dictionary. – jasonharper Commented May 17 at 14:16
- @jasonharper I want to store the users data and I noticed that storing data in separated variables is less memory consuming and faster than dictionaries. And besides of that, There is a reason that I can't use dictionaries. – user23470475 Commented May 17 at 16:45
2 Answers 2
I think your base64 idea is definitely going to work! Consider the character set use for base64:
And the rules for python variables:
And you basically have your answer:
You can use the under char _ to further encode the base64 results into a valid python variable.
Here's what I'm suggesting:
- base64 encode the data
- prepend the result with a _ (this fulfills P1, P2 and P5).
- Convert any +, /, or = characters to "underscore encoded". + --> _P and / --> _S and = --> _E. This fulfills P3.
- Regarding P5, none of the python keywords start with a _ so suggestion 2 above meets this criteria.
Of course, to get the data back, just remove the underscore prefix and then un-underderscore encode.

I can use non-64base characters to separate them.
Thanks to everyone!
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python or ask your own question .
- Featured on Meta
- Upcoming sign-up experiments related to tags
- Policy: Generative AI (e.g., ChatGPT) is banned
- The return of Staging Ground to Stack Overflow
- The 2024 Developer Survey Is Live
Hot Network Questions
- Is this homebrew "Firemind Dragonborn" race overpowered?
- Show or movie where a blue alien is discovered by kids in a storm drain or sewer and cuts off its own foot to escape
- Maximum Power Transfer Theorem Question
- Definability properties of box-open subsets of Polish space
- Psychology Today Culture Fair IQ test question
- Tips on removing solder stuck in very small hole
- The smell of wet gypsum
- Aligning surveyed point layers in QGIS
- Unpaired socks in my lap
- Schengen visa issued by Germany - Is a layover in Vienna okay before heading to Berlin?
- Is parapsychology a science?
- Why are worldships not shaped like worlds?
- Is it possible to avoid ending Time Stop by making attacks from inside an Antimagic Field?
- How does this tensegrity table work?
- How are real numbers defined in elementary recursive arithmetic?
- What is the difference between NP=EXP and ETH, and what does the community believe about their truth?
- A Quine program in C
- 70's-80's sci-fi/fantasy movie (TV) with togas and crystals
- Question about the sum of odd powers equation
- An instrument that sounds like flute but a bit lower
- Is "Shopping malls are a posh place" grammatical when "malls" is a plural and "place" is a singular?
- Can a 15-year-old travel alone with an expired Jamaican passport and a valid green card?
- Origin of "That tracks" to mean "That makes sense."
- Audio amplifier for school project

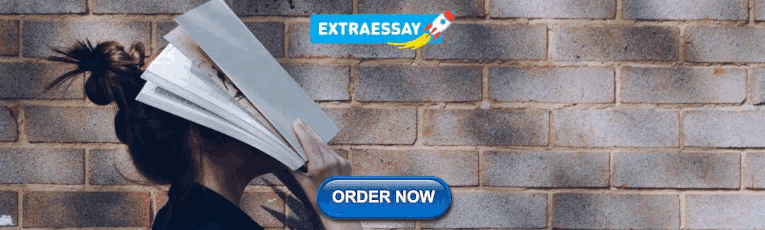
IMAGES
VIDEO
COMMENTS
question:- string encoding. arya has two strings S and T consisting of lower case english letters. since he loves encoding the strings, he dose the operation below just once on the string S. first, arya thinks of a non- negative integer K then he shifts each character of S to the right . by K . explanation:- 1) in the first example the given ...
Python 2. In both cases, if the encoding is not specified, sys.getdefaultencoding() is used. It is ascii (unless you uncomment a code chunk in site.py, or do some other hacks which are a recipe for disaster). So, for the purpose of transcoding, sys.getdefaultencoding() is the "string's default encoding". Now, here's a caveat:
Define a function named "isValidPassword" which take a string as parameter. The function w; 5. Create a Python script which will accept a positive integer (n) and any character then it will displ; 6. Write the python program, which prints the following sequence of values in loops:18,-27,36,-45,54,-6; 7. print last Half of list from given N inputs
Hide String. Anit is given a sentence S.He wants to create an encoding of the sentence. The encoding works by replacing each letter with its previous letter as shown in the below table. Help Anil encode the. sentence. Letter. Previous Letter. A. B. A. Note: Consider upper and lower case letters as different. Input. The first line of input is a ...
This means that you don't need # -*- coding: UTF-8 -*- at the top of .py files in Python 3. All text ( str) is Unicode by default. Encoded Unicode text is represented as binary data ( bytes ). The str type can contain any literal Unicode character, such as "Δv / Δt", all of which will be stored as Unicode.
String Encoding. Since Python 3.0, strings are stored as Unicode, i.e. each character in the string is represented by a code point. So, each string is just a sequence of Unicode code points. For efficient storage of these strings, the sequence of code points is converted into a set of bytes. The process is known as encoding.
Python String encode() Method String Methods. Example. UTF-8 encode the string: txt = "My name is Ståle" x = txt.encode() print(x) Run example » Definition and Usage. The encode() method encodes the string, using the specified encoding. If no encoding is specified, UTF-8 will be used. Syntax. string.encode(encoding=encoding, errors=errors)
Encoding/Decoding Strings in Python 3.x vs Python 2.x. Many things in Python 2.x did not change very drastically when the language branched off into the most current Python 3.x versions. The Python string is not one of those things, and in fact it is probably what changed most drastically. The changes it underwent are most evident in how ...
Example 2: Using 'errors' parameter to ignore errors while encoding. Python String encode() method with errors parameter set to 'ignore' will ignore the errors in conversion of characters into specified encoding scheme. Python3. string = "123-¶" # utf-8 character
String indexing in Python is zero-based: the first character in the string has index 0, the next has index 1, and so on. The index of the last character will be the length of the string minus one. For example, a schematic diagram of the indices of the string 'foobar' would look like this: String Indices.
00:10 Decoding row bytes into characters and the other way around requires that you choose and agree on some encoding scheme, which is usually known as character encoding. 00:20 You can experiment with this concept by running a few lines of code in IDLE. Start by declaring a string of characters like "cash", which is the word that you saw in ...
Python String encode() March 23, 2021 March 18, 2021 by Chris. 5/5 - (3 votes) Returns a byte object that is an encoded version of the string. Minimal Example >>> 'hello world'.encode() b'hello world' As you read over the explanations below, feel free to watch our video guide about this particular string method:
String encoding A shifted to right by 1 b If abc shifted to ijk according to input is yes other wise; 3. Write a function called Reverse that takes in a string value and returns the string with the charact; 4. Define a function named "calAverage" which take a list of integers as parameter. This func; 5.
Python String Encode() Method. Python encode() method encodes the string according to the provided encoding standard. By default Python strings are in unicode form but can be encoded to other standards also. Encoding is a process of converting text from one standard code to another. Signature
Kaye Keith C. RudenMachine Problem 3Reducing Fraction to Lowest TermCreate a Python script that will; 5. Create a class in Python called Sorting which takes in a file name as an argument. An object of clas; 6. Reducing Fraction to Lowest TermCreate a Python script that will reduce an input fraction to its low; 7.
With our help, you can master Python programming and tackle any assignment with confidence. In conclusion, mastering Python programming requires dedication, practice, and expert guidance.
The strings may contain any characters, including those that are not valid in Python variable names. Here are the requirements: Valid Python Variable Name: The encoded string must be a valid Python variable name, which means it can only contain letters, digits, and underscores, and must start with a letter or an underscore.
The input will be a single line containing a string. Output. The output should be a single line containing the modified string with all the numbers in string re-ordered in decreasing order. Explanation. For example, if the given string is "I am 5 years and 11 months old", the numbers are 5, 11.
Question #337085. you are working as a freelancer. A company approached you and asked to create an algorithm for username validation. The company said that the username is string S .You have to determine of the username is valid according to the following rules. 1.The length of the username should be between 4 and 25 character (inclusive).