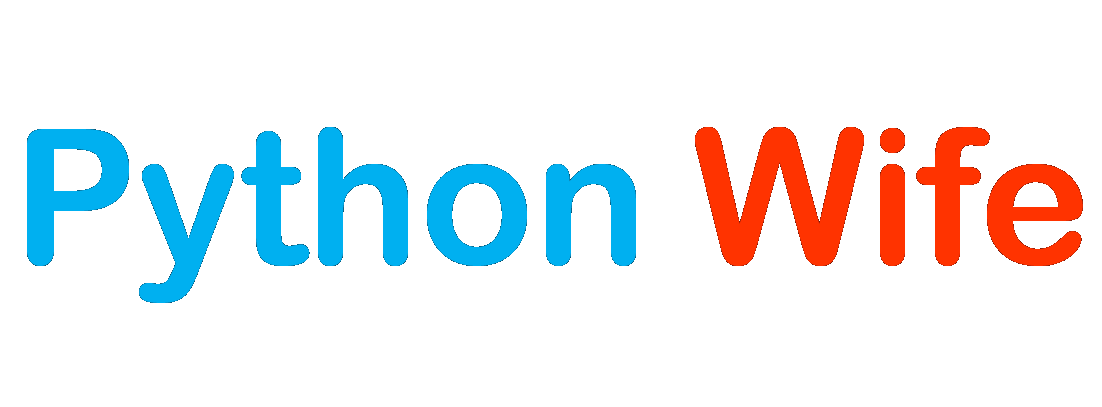
- Computer Vision
- Problem Solving in Python
- Intro to DS and Algo
- Analysis of Algorithm
- Dictionaries
- Linked Lists
- Doubly Linked Lists
- Circular Singly Linked List
- Circular Doubly Linked List
- Tree/Binary Tree
- Binary Search Tree
- Binary Heap
- Sorting Algorithms
- Searching Algorithms
- Single-Source Shortest Path
- Topological Sort
- Dijkstra’s
- Bellman-Ford’s
- All Pair Shortest Path
- Minimum Spanning Tree
- Kruskal & Prim’s
Problem-solving is the process of identifying a problem, creating an algorithm to solve the given problem, and finally implementing the algorithm to develop a computer program .
An algorithm is a process or set of rules to be followed while performing calculations or other problem-solving operations. It is simply a set of steps to accomplish a certain task.
In this article, we will discuss 5 major steps for efficient problem-solving. These steps are:
- Understanding the Problem
- Exploring Examples
- Breaking the Problem Down
- Solving or Simplification
- Looking back and Refactoring
While understanding the problem, we first need to closely examine the language of the question and then proceed further. The following questions can be helpful while understanding the given problem at hand.
- Can the problem be restated in our own words?
- What are the inputs that are needed for the problem?
- What are the outputs that come from the problem?
- Can the outputs be determined from the inputs? In other words, do we have enough information to solve the given problem?
- What should the important pieces of data be labeled?
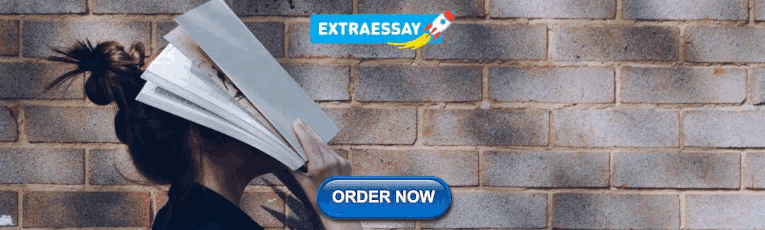
Example : Write a function that takes two numbers and returns their sum.
- Implement addition
- Integer, Float, etc.
Once we have understood the given problem, we can look up various examples related to it. The examples should cover all situations that can be encountered while the implementation.
- Start with simple examples.
- Progress to more complex examples.
- Explore examples with empty inputs.
- Explore examples with invalid inputs.
Example : Write a function that takes a string as input and returns the count of each character
After exploring examples related to the problem, we need to break down the given problem. Before implementation, we write out the steps that need to be taken to solve the question.
Once we have laid out the steps to solve the problem, we try to find the solution to the question. If the solution cannot be found, try to simplify the problem instead.
The steps to simplify a problem are as follows:
- Find the core difficulty
- Temporarily ignore the difficulty
- Write a simplified solution
- Then incorporate that difficulty
Since we have completed the implementation of the problem, we now look back at the code and refactor it if required. It is an important step to refactor the code so as to improve efficiency.
The following questions can be helpful while looking back at the code and refactoring:
- Can we check the result?
- Can we derive the result differently?
- Can we understand it at a glance?
- Can we use the result or mehtod for some other problem?
- Can you improve the performance of the solution?
- How do other people solve the problem?
Trending Posts You Might Like
- File Upload / Download with Streamlit
- Seaborn with STREAMLIT
- Dijkstra’s Algorithm in Python
- Greedy Algorithms in Python
Author : Bhavya
- For Individuals
- For Businesses
- For Universities
- For Governments
- Online Degrees
- Find your New Career
- Join for Free

Python Basics: Problem Solving with Code
This course is part of Python Basics for Online Research Specialization

Instructor: Seth Frey
Financial aid available

What you'll learn
Explore your own web browsing habits and manage code complexity and reading manuals.
Discuss how Python understands complex and real world things, and practice looking under the hood of a single tweet.
Apply the building blocks of Python and turn it into a little language for drawing pictures.
Practice "debugging" code and learn to think the way code thinks.
Details to know

Add to your LinkedIn profile
See how employees at top companies are mastering in-demand skills
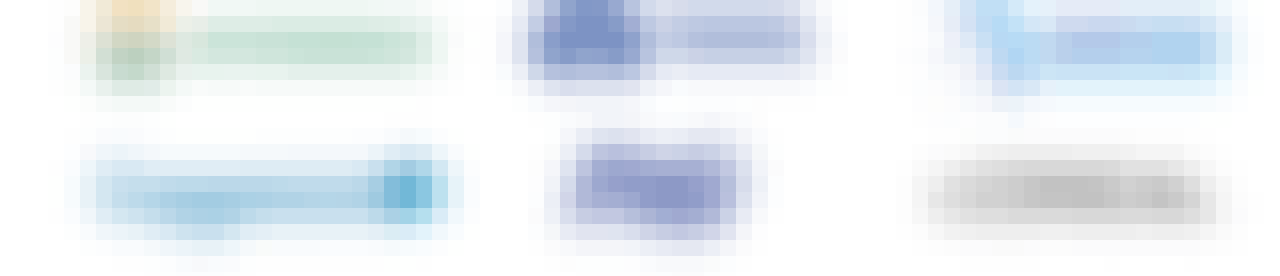
Build your subject-matter expertise
- Learn new concepts from industry experts
- Gain a foundational understanding of a subject or tool
- Develop job-relevant skills with hands-on projects
- Earn a shareable career certificate
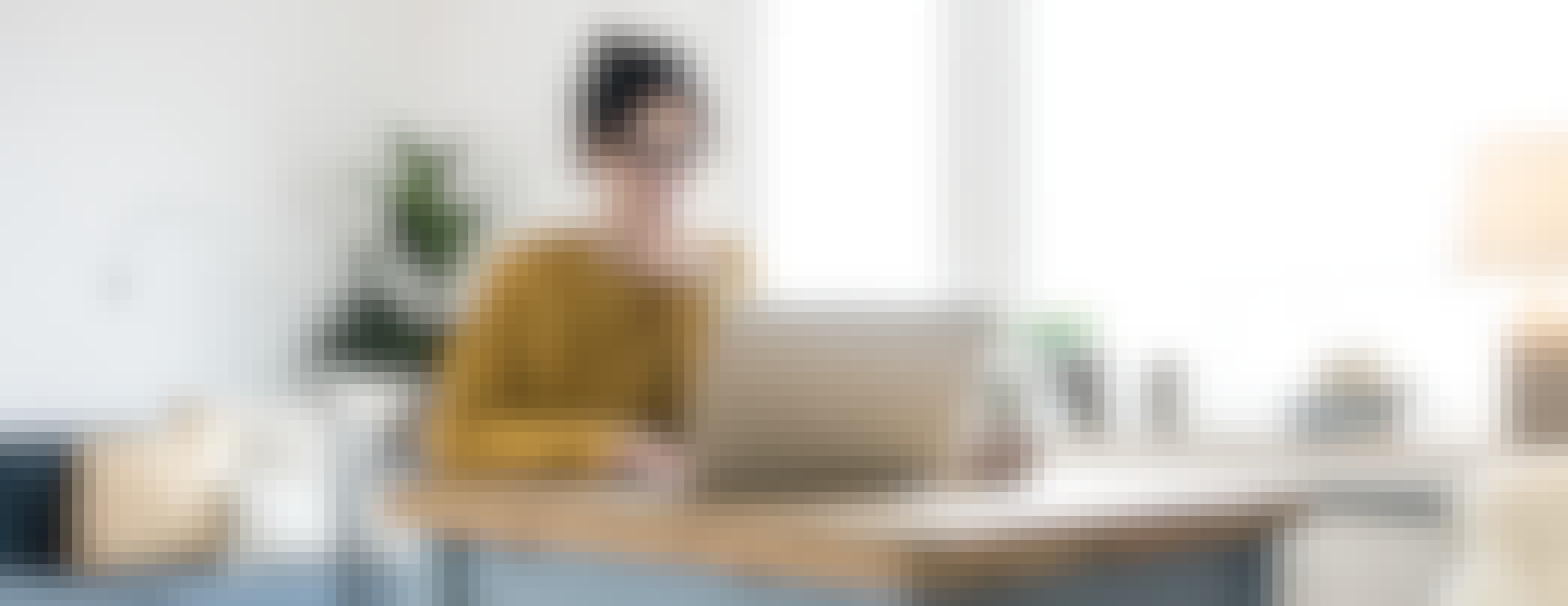
Earn a career certificate
Add this credential to your LinkedIn profile, resume, or CV
Share it on social media and in your performance review
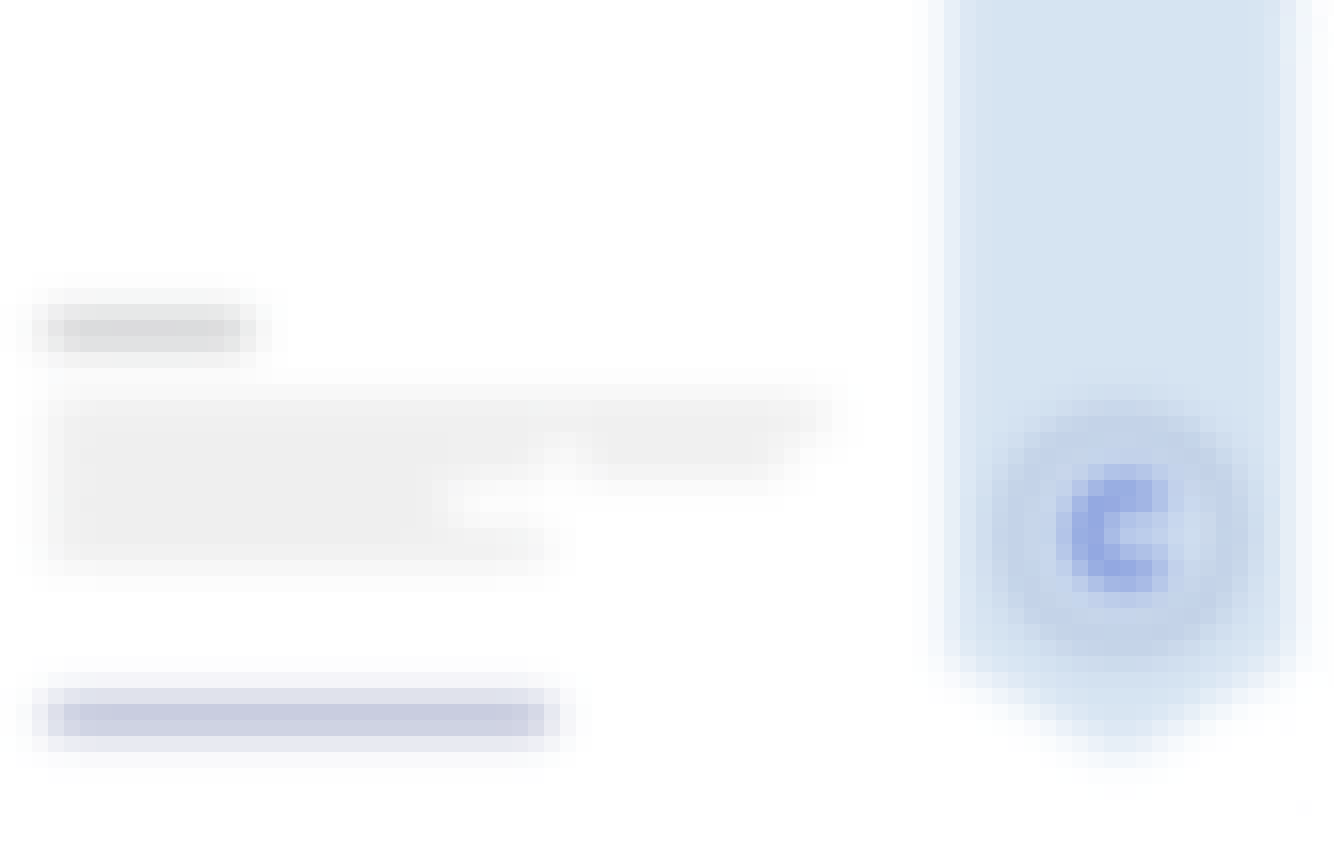
There are 4 modules in this course
A lot of code is building up from the most basic primitive elements of the language to increasingly faithful and meaningful things. In this course you will see how to author more complex ideas and capabilities in Python. In technical terms, you will learn dictionaries and how to work with them and nest them, functions, refactoring, and debugging, all of which are also thinking tools for the art of problem solving. We'll use this knowledge to explore our browsing history, interrogate a tweet, and draw pictures.
Examining Your Own Web Browsing Habits in Python
Can you use code to learn about...yourself? We're starting this course with a module in which you explore web browsing habits. If you installed the plugins for tracking your web history, you'll be able to explore your own. As this code gets more involved, you'll also get more advice on managing code complexity and reading manuals. Let's get started!
What's included
3 videos 5 readings 1 quiz 1 programming assignment 2 discussion prompts
3 videos • Total 20 minutes
- Course Introduction • 3 minutes • Preview module
- Hiding from the Complexity • 3 minutes
- Demo: Web History Walkthrough • 13 minutes
5 readings • Total 65 minutes
- A Note From UC Davis • 10 minutes
- Internet Mapping Glitch • 10 minutes
- Jupyter Notebook Tutorials • 30 minutes
- Demo and Code Lesson Walkthrough "How To" • 5 minutes
- SETUP: Self-Surveillance with Privacy Badger • 10 minutes
1 quiz • Total 15 minutes
- Module 1 Quiz • 15 minutes
1 programming assignment • Total 30 minutes
- Demo: Web History • 30 minutes
2 discussion prompts • Total 20 minutes
- Learning Goals • 10 minutes
- Web History • 10 minutes
Representing Complex Ideas in Python
Understanding how Python understands the world brings us to "dictionaries", which are kind of like lists, but they allow more variety and structure. This module will build up to representing increasingly complex real world things. We will build up to looking under the hood of a single tweet, and understanding the "social" in "social media".
10 videos 1 quiz 1 programming assignment 1 discussion prompt
10 videos • Total 47 minutes
- Introduction • 2 minutes • Preview module
- Python "Dictionaries" • 4 minutes
- Checking for Things in Dictionaries • 1 minute
- How is This not a List? • 2 minutes
- Collections in Collections • 2 minutes
- Dictionaries in Dictionaries • 3 minutes
- Looking at a Complex Dictionary • 3 minutes
- Looping Through a Dictionary • 9 minutes
- Representing Things in the Real World • 5 minutes
- Bringing it all Together • 11 minutes
1 quiz • Total 30 minutes
- Module 2 Quiz • 30 minutes
1 programming assignment • Total 100 minutes
- Code Lesson: Dictionaries and Nested Dictionaries • 100 minutes
1 discussion prompt • Total 10 minutes
- Obstacles and Opportunities • 10 minutes
Making Pictures with Robots
Everything you've learned in this course about Python is just basic building blocks that programmers use to build bigger building blocks of their own. In this module, we'll do precisely that, turning Python into a little language for drawing pictures, a DIY MS Paint.
8 videos 1 reading 1 quiz 1 programming assignment 1 discussion prompt
8 videos • Total 56 minutes
- Introduction • 3 minutes • Preview module
- Creating Functions • 6 minutes
- Functions are Flexible • 9 minutes
- Functions as Little Worlds • 4 minutes
- Turtle Graphics and Building Meaning with Functions • 7 minutes
- Functions for Avoiding Tedium • 8 minutes
- "Refactoring" • 11 minutes
- Bringing it all Together • 5 minutes
1 reading • Total 30 minutes
- Algorithmic Bias • 30 minutes
- Module 3 Quiz • 30 minutes
1 programming assignment • Total 120 minutes
- Code Lesson: Adding Functionality to Code • 120 minutes
- Why Code? • 10 minutes
A Strategy for Hunting Bugs
A major part of programming that no one ever tells you about is "debugging": spending seconds, minutes, hours, or even days going through code that should work to understand why it doesn't. This demoralizing subject stops a lot of beginners, but there is a way to be good at it, and that is a major part of learning to think the way code thinks.
5 videos 2 readings 1 quiz 2 discussion prompts
5 videos • Total 57 minutes
- How to Debug Code, Part 1 • 19 minutes • Preview module
- How to Debug Code, Part 2 • 21 minutes
- How to Debug Code, Part 3 • 3 minutes
- Becoming a Good Coder • 10 minutes
- Course Summary • 2 minutes
2 readings • Total 20 minutes
- Additional Resources • 10 minutes
- Course Credits • 10 minutes
- Module 4 Quiz • 15 minutes
2 discussion prompts • Total 40 minutes
- Debugging • 30 minutes
- Self-Reflection • 10 minutes

UC Davis, one of the nation’s top-ranked research universities, is a global leader in agriculture, veterinary medicine, sustainability, environmental and biological sciences, and technology. With four colleges and six professional schools, UC Davis and its students and alumni are known for their academic excellence, meaningful public service and profound international impact.
Recommended if you're interested in Algorithms
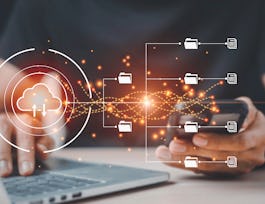
University of California, Davis
Python Basics: Retrieving Online Data
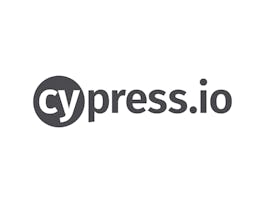
Coursera Project Network
Cypress end to end testing and intercepting network call
Guided Project
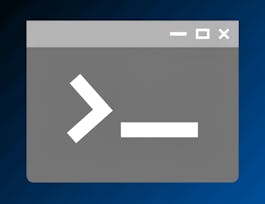
Duke University
Python Scripting
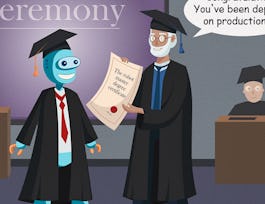
Deploy Models with TensorFlow Serving and Flask
Why people choose coursera for their career.

New to Algorithms? Start here.

Open new doors with Coursera Plus
Unlimited access to 7,000+ world-class courses, hands-on projects, and job-ready certificate programs - all included in your subscription
Advance your career with an online degree
Earn a degree from world-class universities - 100% online
Join over 3,400 global companies that choose Coursera for Business
Upskill your employees to excel in the digital economy
Frequently asked questions
When will i have access to the lectures and assignments.
Access to lectures and assignments depends on your type of enrollment. If you take a course in audit mode, you will be able to see most course materials for free. To access graded assignments and to earn a Certificate, you will need to purchase the Certificate experience, during or after your audit. If you don't see the audit option:
The course may not offer an audit option. You can try a Free Trial instead, or apply for Financial Aid.
The course may offer 'Full Course, No Certificate' instead. This option lets you see all course materials, submit required assessments, and get a final grade. This also means that you will not be able to purchase a Certificate experience.
What will I get if I subscribe to this Specialization?
When you enroll in the course, you get access to all of the courses in the Specialization, and you earn a certificate when you complete the work. Your electronic Certificate will be added to your Accomplishments page - from there, you can print your Certificate or add it to your LinkedIn profile. If you only want to read and view the course content, you can audit the course for free.
What is the refund policy?
If you subscribed, you get a 7-day free trial during which you can cancel at no penalty. After that, we don’t give refunds, but you can cancel your subscription at any time. See our full refund policy Opens in a new tab .
Is financial aid available?
Yes. In select learning programs, you can apply for financial aid or a scholarship if you can’t afford the enrollment fee. If fin aid or scholarship is available for your learning program selection, you’ll find a link to apply on the description page.
How to Solve Algorithmic Problems in Python
Understanding the Basics of Algorithmic Problems
An algorithm is a step-by-step procedure for solving a problem or accomplishing a specific task. Algorithmic problems are challenges that require an algorithm to solve. These challenges often involve data manipulation or optimization.
Step 1: Understand the Problem
The first step in solving any problem, algorithmic or otherwise, is to understand the problem thoroughly. This involves identifying the problem's inputs, expected outputs, and constraints.
Step 2: Design the Algorithm
Once you understand the problem, the next step is to design an algorithm to solve it. This involves breaking down the problem into smaller, more manageable parts and designing a solution for each.
Example: Solving a Sorting Problem
Let's look at a simple algorithmic problem: sorting a list of numbers in ascending order. Here is a Python function that uses the Bubble Sort algorithm to solve this problem:
The bubble_sort function sorts the list of numbers by repeatedly swapping adjacent numbers if they are in the wrong order.
Step 3: Implement the Algorithm
After designing the algorithm, you must implement it in Python. This involves writing Python code that follows the steps outlined in your algorithm.
The code above creates a list of numbers, sorts it using the bubble_sort function, and then prints the sorted list.
Step 4: Test the Algorithm
After implementing the algorithm, you should test it to ensure it works correctly. This involves running your Python code and checking that it produces the expected output for various input values.
The code snippet above shows the output of running the bubble_sort.py script in a shell. As you can see, it correctly sorts the list of numbers.
Step 5: Analyze the Algorithm
You should analyze your algorithm to understand its efficiency. This involves calculating your algorithm's time and space complexity, which are measures of how the algorithm's resource usage scales with the input size.
For Bubble Sort, the time complexity is O(n^2), and the space complexity is O(1). This means Bubble Sort can be inefficient for large lists but uses a small, constant amount of memory.
In conclusion, solving algorithmic problems in Python involves understanding the problem, designing an algorithm to solve it, implementing it in Python, testing it, and analyzing its efficiency. This process is a fundamental part of Python programming and general software development. If you're looking to hire Python developers who are proficient in solving algorithmic problems, check out Reintech.
If you're interested in enhancing this article or becoming a contributing author, we'd love to hear from you.
Please contact Sasha at [email protected] to discuss the opportunity further or to inquire about adding a direct link to your resource. We welcome your collaboration and contributions!
An algorithm is a step-by-step procedure or set of instructions designed to solve a problem or achieve a specific goal. In computer programming, algorithms are used to perform tasks, manipulate data, and make decisions based on certain conditions. Developing efficient algorithms is a crucial aspect of computer science and software engineering.
To learn more about algorithms, visit the Wikipedia page on the subject.
Bubble Sort
Bubble Sort is a simple comparison-based sorting algorithm. It works by repeatedly swapping adjacent elements if they are in the wrong order. This process continues until no more swaps are needed, indicating that the list is sorted. Although it's straightforward and easy to implement, Bubble Sort is not efficient for large datasets compared to other sorting algorithms like Quick Sort or Merge Sort. More info .
Hire Python Developers with Cloudfront Skill for Your Remote Tech Team
Boost Your Technical Excellence With Python JWT Skilled Remote Developers
Hire Expert Python Developers Skilled in Shopify API


- Runestone in social media: Follow @iRunestone Our Facebook Page
- Table of Contents
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- This Chapter
- 1. Introduction' data-toggle="tooltip" >
Problem Solving with Algorithms and Data Structures using Python ¶

By Brad Miller and David Ranum, Luther College
There is a wonderful collection of YouTube videos recorded by Gerry Jenkins to support all of the chapters in this text.
- 1.1. Objectives
- 1.2. Getting Started
- 1.3. What Is Computer Science?
- 1.4. What Is Programming?
- 1.5. Why Study Data Structures and Abstract Data Types?
- 1.6. Why Study Algorithms?
- 1.7. Review of Basic Python
- 1.8.1. Built-in Atomic Data Types
- 1.8.2. Built-in Collection Data Types
- 1.9.1. String Formatting
- 1.10. Control Structures
- 1.11. Exception Handling
- 1.12. Defining Functions
- 1.13.1. A Fraction Class
- 1.13.2. Inheritance: Logic Gates and Circuits
- 1.14. Summary
- 1.15. Key Terms
- 1.16. Discussion Questions
- 1.17. Programming Exercises
- 2.1.1. A Basic implementation of the MSDie class
- 2.2. Making your Class Comparable
- 3.1. Objectives
- 3.2. What Is Algorithm Analysis?
- 3.3. Big-O Notation
- 3.4.1. Solution 1: Checking Off
- 3.4.2. Solution 2: Sort and Compare
- 3.4.3. Solution 3: Brute Force
- 3.4.4. Solution 4: Count and Compare
- 3.5. Performance of Python Data Structures
- 3.7. Dictionaries
- 3.8. Summary
- 3.9. Key Terms
- 3.10. Discussion Questions
- 3.11. Programming Exercises
- 4.1. Objectives
- 4.2. What Are Linear Structures?
- 4.3. What is a Stack?
- 4.4. The Stack Abstract Data Type
- 4.5. Implementing a Stack in Python
- 4.6. Simple Balanced Parentheses
- 4.7. Balanced Symbols (A General Case)
- 4.8. Converting Decimal Numbers to Binary Numbers
- 4.9.1. Conversion of Infix Expressions to Prefix and Postfix
- 4.9.2. General Infix-to-Postfix Conversion
- 4.9.3. Postfix Evaluation
- 4.10. What Is a Queue?
- 4.11. The Queue Abstract Data Type
- 4.12. Implementing a Queue in Python
- 4.13. Simulation: Hot Potato
- 4.14.1. Main Simulation Steps
- 4.14.2. Python Implementation
- 4.14.3. Discussion
- 4.15. What Is a Deque?
- 4.16. The Deque Abstract Data Type
- 4.17. Implementing a Deque in Python
- 4.18. Palindrome-Checker
- 4.19. Lists
- 4.20. The Unordered List Abstract Data Type
- 4.21.1. The Node Class
- 4.21.2. The Unordered List Class
- 4.22. The Ordered List Abstract Data Type
- 4.23.1. Analysis of Linked Lists
- 4.24. Summary
- 4.25. Key Terms
- 4.26. Discussion Questions
- 4.27. Programming Exercises
- 5.1. Objectives
- 5.2. What Is Recursion?
- 5.3. Calculating the Sum of a List of Numbers
- 5.4. The Three Laws of Recursion
- 5.5. Converting an Integer to a String in Any Base
- 5.6. Stack Frames: Implementing Recursion
- 5.7. Introduction: Visualizing Recursion
- 5.8. Sierpinski Triangle
- 5.9. Complex Recursive Problems
- 5.10. Tower of Hanoi
- 5.11. Exploring a Maze
- 5.12. Dynamic Programming
- 5.13. Summary
- 5.14. Key Terms
- 5.15. Discussion Questions
- 5.16. Glossary
- 5.17. Programming Exercises
- 6.1. Objectives
- 6.2. Searching
- 6.3.1. Analysis of Sequential Search
- 6.4.1. Analysis of Binary Search
- 6.5.1. Hash Functions
- 6.5.2. Collision Resolution
- 6.5.3. Implementing the Map Abstract Data Type
- 6.5.4. Analysis of Hashing
- 6.6. Sorting
- 6.7. The Bubble Sort
- 6.8. The Selection Sort
- 6.9. The Insertion Sort
- 6.10. The Shell Sort
- 6.11. The Merge Sort
- 6.12. The Quick Sort
- 6.13. Summary
- 6.14. Key Terms
- 6.15. Discussion Questions
- 6.16. Programming Exercises
- 7.1. Objectives
- 7.2. Examples of Trees
- 7.3. Vocabulary and Definitions
- 7.4. List of Lists Representation
- 7.5. Nodes and References
- 7.6. Parse Tree
- 7.7. Tree Traversals
- 7.8. Priority Queues with Binary Heaps
- 7.9. Binary Heap Operations
- 7.10.1. The Structure Property
- 7.10.2. The Heap Order Property
- 7.10.3. Heap Operations
- 7.11. Binary Search Trees
- 7.12. Search Tree Operations
- 7.13. Search Tree Implementation
- 7.14. Search Tree Analysis
- 7.15. Balanced Binary Search Trees
- 7.16. AVL Tree Performance
- 7.17. AVL Tree Implementation
- 7.18. Summary of Map ADT Implementations
- 7.19. Summary
- 7.20. Key Terms
- 7.21. Discussion Questions
- 7.22. Programming Exercises
- 8.1. Objectives
- 8.2. Vocabulary and Definitions
- 8.3. The Graph Abstract Data Type
- 8.4. An Adjacency Matrix
- 8.5. An Adjacency List
- 8.6. Implementation
- 8.7. The Word Ladder Problem
- 8.8. Building the Word Ladder Graph
- 8.9. Implementing Breadth First Search
- 8.10. Breadth First Search Analysis
- 8.11. The Knight’s Tour Problem
- 8.12. Building the Knight’s Tour Graph
- 8.13. Implementing Knight’s Tour
- 8.14. Knight’s Tour Analysis
- 8.15. General Depth First Search
- 8.16. Depth First Search Analysis
- 8.17. Topological Sorting
- 8.18. Strongly Connected Components
- 8.19. Shortest Path Problems
- 8.20. Dijkstra’s Algorithm
- 8.21. Analysis of Dijkstra’s Algorithm
- 8.22. Prim’s Spanning Tree Algorithm
- 8.23. Summary
- 8.24. Key Terms
- 8.25. Discussion Questions
- 8.26. Programming Exercises
Acknowledgements ¶
We are very grateful to Franklin Beedle Publishers for allowing us to make this interactive textbook freely available. This online version is dedicated to the memory of our first editor, Jim Leisy, who wanted us to “change the world.”
Indices and tables ¶
Search Page

- Docs »
- Lesson notes »
- Lesson 3 - Problem solving techniques
- Edit on GitHub
Lesson 3 - Problem solving techniques ¶
In the last lesson, we learned control flow statements such as if-else and for .
In this lesson, let us try to write a program for this problem:
“Given a day, the program should print if it is a weekday or weekend.”
First, we need to accept the input from the command line.:
Now, we need to find what type of day it is. The most straightforward way of doing it is checking the input against each day, like so:
Notice, how multiple if conditions can be combined using elif .
The program works but there are some issues with it. First, code looks a bit cluttered. Second, what if you need to write a similar program but with a bigger list? You can’t keep adding elif statements.
What we are trying to do here is to see if a given input (name of the day in this case) belongs to a group (such as “weekday” or “weekend”). Such problems can be solved by using lists. Since there are two types of days here for the purpose of this problem, let us define two lists.:
We can simply check if the input is in the first list or second list:
Notice how the second version is much smaller and much easier to read. In general, you should try to use types such as lists (and others provided by Python which we will see later) to solve problems.
Also note how we added else clause at the very end. Now our program gives a proper error message if the input is not valid. This is another thing you need to keep in mind when you accept inputs. Your code needs to be prepared to handle both valid and invalid inputs . In the case of latter, the program should provide a good error message.
Problem solving techniques ¶
- Divide the problem into smaller ones and solve them. Once smaller problems are solved, you just need to figure out how to combine them. This technique is called divide and conquer .
- Don’t try to write a perfect working program in the first go. Instead, write something that works and slowly improve it.
- Don’t try to write the program directly. First solve the problem for some example inputs and see how you are solving it (you can use paper and pencil for this). Then, try to convert the solution into a program.
Assignment ¶
Given a word, your program should print number of vowels in the word, like so:
You will be using most of the concepts you learned in this lesson and the previous ones to solve this problem, so go over the notes if required.
Mastering Algorithms for Problem Solving in Python
This course is about getting hands-on experience solving algorithms in Python.
Intermediate
Certificate of Completion
This course includes
Course Overview
As a developer, mastering the concepts of algorithms and being proficient in implementing them is essential to improving problem-solving skills. This course aims to equip you with an in-depth understanding of algorithms and how they can be utilized for problem-solving in Python. Starting with the basics, you'll gain a foundational understanding of what algorithms are, with topics ranging from simple multiplication algorithms to analyzing algorithms. Then, you’ll delve into more advanced topics like recursi... Show More
What You'll Learn
A comprehensive understanding of algorithms and their applications in problem solving
Proficiency in implementing recursion and backtracking in Python for complex tasks
An understanding of the concept of memoization and dynamic programming
Ability to apply memoization and dynamic programming for efficient computation in Python
Hands-on experience solving algorithmic challenges in Python
Course Content
Getting Started
Introduction to Algorithm
Backtracking
Dynamic Programming
Greedy Algorithms
Prove Your Skills: A Five-Chapter Assessment
Basic Graph Algorithms
Depth-First Search
Minimum Spanning Trees
Shortest Paths
All-Pairs Shortest Paths
Pushing Your Limits: A Comprehensive Assessment
Wrapping up
Trusted by 1.4 million developers working at companies
Anthony Walker
@_webarchitect_
Emma Bostian 🐞
@EmmaBostian
Evan Dunbar
ML Engineer
Carlos Matias La Borde
Software Developer
Souvik Kundu
Front-end Developer
Vinay Krishnaiah
Musician/Entrepeneur
Kenan Eyvazov
DevOps Engineer
See how Educative uses AI to make your learning more immersive than ever before.
Instant Code Feedback
AI-Powered Mock Interviews
Adaptive Learning
Explain with AI
AI Code Mentor
Looking for something else?
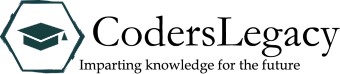
Python Problem Solving
Q. how to create backups in python using zip files, q. how to iterate through multi dimensional arrays , q. how to take input from the user with a user interface (tkinter gui), q. how to update/change values dynamically on a gui (tkinter), q. how to remove the newline (/n) character while reading from files, q. how to create a spreadsheet (tkinter gui), q. how to create an undo button (tkinter gui), q. how to edit a file, q. how to improve tkinter gui screen resolution (fix for blurriness), q. how to skip captcha and bot checks while web scraping, q. how to create a save and load system for a game or application, q. how to dynamically update values in a matplotlib graph, q. how to create multiple tkinter gui windows , q. how to take a password as input from the user (tkinter), q. how to create an open file dialog, q. how to check if a specific file paths exists, q. how to change the font type and size in tkinter , q. trigger a function if user tries to close tkinter window, q. how to make a time/date based expiration for my software, q. how to disable resizing in a tkinter window , q. how to make my tkinter window start up in the center, q. how to subtract two dates from each other.
Walkthrough
Programming problem solving walkthrough.
A programming problem solving walkthrough is a written guided description of the journey from a problem to a solution. It aims to teach how to solve programming problems in a methodical and thoughtful manner using the model. In other words, the knowledge to be learned is focused on the "how", and not on the programming language per se.
The walkthrough, as a teaching method, is based on two concepts: worked example from learning sciences and literate programming from computer science.
A worked example is a step-by-step demonstration of how to solve a problem. Learning scientists found out that worked examples are most effective for novices (i.e., the audience of a walkthrough), while performing problem-solving is more beneficial for experts. There are multiple ways of presenting and supporting worked examples , and one of the evidence-based techniques is to include sub-goal labeling, which is about labeling groups of steps in the worked example.
Literate programming by Donald E. Knuth is a programming paradigm in which a program is written as interspersed snippets of executable code and text. The text, which is written in ordinary human language, explains the logic of the code and explains the programmer's thoughts and decisions. Thus a program is perceived much more like an essay.
The walkthrough combines these two powerful ideas for learning the craft of problem solving by programming. It uses the programming problem solving model and its supplements to give a framework for establishing the learning objectives, as well as defining the walkthrough's structure and flow.
The learning objective of a walkthrough is rooted in one of the phases (for example, acquiring the ability to use a specific design strategy). That's in addition to the always-present learning objective of mastering the instrumentation of end-to-end problem solving .
Many times the solving process is hidden, and one gets only the final result, the executable code. Therefore, an essential feature of a walkthrough is making the reasoning explicit, as suggested by literate programming. In other words, it brings the solving process to the surface and documents the train of thought of the problem-solver as they go through each of the phases. In fact, a walkthrough aims to prompt self-explanation by the learners.
A similar but different flavor of a walkthrough is one that is developed by the learners. They choose a programming problem and fill in a provided walkthrough template. The learners improve their ability to solve problems by explicitly documenting their own process.
This page was written with Python in mind, with Jupyter Notebook that serves as the medium for the walkthrough. Notebooks are natural for literate programming , with their capability to mix text, media, and code in cells. Nevertheless, a walkthrough is a teaching method which is beyond one programming language or another, and it can also be developed as a source code file.
Few (opinionated) Principles and Practices
A walkthrough is an active learning activity. It is similar to a tutorial in the sense that it is most effective if the reader follows along by actually performing the tasks being described. In our particular case the tasks are based on the phases of the problem-solving model .
For example:
- Reinterpret the Problem phase - suggest input-output instances.
- Design a Solution phase - write about choosing a data structure, what attributes make it fit.
While it is important to focus on one particular phase so as not to overwhelm the learner, other phases should not be neglected. To keep the student engaged throughout the walkthrough it is suggested to use less demanding, yet active, tasks.
Tasks suggested by phase appear later on this page.
The walkthrough is designed to achieve teachable moments , in which it leads the learners to a point where they discover or apply a concept, an idea or a technique from the learning objectives.
At the same time a walkthrough must manage cognitive load , with a great focus on the external one.
The walkthrough is a "better version of reality" that focuses on the learning, and not on accurate journaling of the problem solving process . By its nature, this process is often messy and non-linear. Meanwhile, the goal of the walkthrough is to guide the learner through solving the problem in a logical and clear manner, without recording all the twists and turns. In that sense, the walkthrough is a "better version of reality", in which the actual steps are filtered and distilled to support the designer's learning objectives efficiently.
The text and code should be written in expository style . Even if a program has a hierarchical (tree) design, it this might not mean that this structure is the best for its development.
A problem should be solved and explored in a psychologically correct order , following the solver's "stream of consciousness" . The objective is to go through the process of solving, and a walkthrough inherently performs a "linearization" of this path. As Donald E. Knuth wrote:
My experiences have led me to believe that a person reading a program is likewise, ready to comprehend it by learning its various parts in approximately the order in which it was written.
The walkthrough is intended to be perceived as a dialogue with the learner . Of course, this is impossible due to the non-interactive format, but aimed as aspiration.
Walkthroughs are not stand alone but should be considered in context of a unit or a course. After the learners worked on the walkthrough, a wrap-up session (that might include a presentation or live coding of partial or complete solution) should take place.
Use of real-word problems or cover story can increase the motivation of the learners.
It is advised to limit the external permitted materials for the learners.
Checklist / Rubric
This is an opinionated checklist of all the points that a decent walkthrough should fulfill. It refers to the complete walkthrough after a learner performs all the tasks. It is up to the developer to decide which parts are already presented at the beginning and which are left to the learners.
- Get something working and keep it working:
- First writing code in cells, testing it and only then encapsulating into functions
Reinterpret the Problem
- Rephrasing the problem statement in their own words
- Writing the solution contract
- Meaning and (Python) type
- One or few input-output pairs of concrete instances (it doesn't need to be comprehensive right now, it is not the Test phase)
Design a Solution
- Ultimately, a walkthrough should break down the problem into hierarchical sub-problems; in other words, it should present an outline of the solution decomposed into sub-problems.
- Following the DRY (Don't Repeat Yourself ) design principle - Identifying repeating sub-problems
- A mapping between the information in the problem/solution domain to a data-structure (either primitive or composed, flat or nested) in Python
- Describing the meaning of the data structure, the relationship between its components and the required operation (a.k.a questions)
- Transforming well the design into code
- Pythonic Code - Python Idioms
- Meaningful Names
- Using Comments
- Using Helper Functions when needed (e.g., for following DRY)
- Black box (e.g., trivial cases, simplest non-trivial cases, edge cases, corner cases - but it is not mandatory to write the type)
- White box - coverage / path-complete
- Scenario - Using the language of the problem phase/domain
- Instance - Concrete Input-Output pairs
- The test case follows the simplicity principle - the instance(s) should be the simplest possible to test the scenario.
- Following the empirical approach for debugging (reproduce, diagnose, fix, repeat, reflect)
- Diagnose - Focus on reasoning on the available clues (e.g., the bug itself - input/output and trace-back, reading the code with a critical eye, using the print function)
Evaluate & Reflect
- Functionality
- Design & Code
- Readability, Style & Documentation
- Alignment between the presented problem solving process and the takeaways
- Postmortem of the problem solving or debugging process
- Self-debugging - what the programmer can learn from solving this problem.
Solution Program - Solving the Problem
- Copy-paste and organize all the necessary code for a complete solution of the problem in one cell or py file, and execute it to solve the original problem. Note that it might require asking the learners to combine code snippets from different parts of the notebook.
- The code in the solution section is self-contained for execution, and doesn't depend on other snippets of code from the previous steps.
- This section also contains tests of the solution code.
Tips for Developing a Walkthrough
The Carpentries Curriculum Development Handbook is an excellent resource for how to develop a curriculum in computing and what it says is equally applicable to developing a walkthrough.
First of all, set the learning objectives using the programming problem solving model terminology.
Form a problem - choose an algorithmic one or real-world one.
Solve the problem, document your process based on the model, pay attention to your mistakes and bugs.
Reflect on your problem solving process and try to distill it into steps and teachable moments.
Refactor your code and remove clutter. While it doesn't have to be the best possible, make sure to adjust for the required ability of your learners.
Write an outline of the walkthrough and embed your code in the relevant sections. Validate it with a checklist .
Decide what tasks the learners should do, making sure they align with the learning objectives.
Write the complete text of the walkthrough that guides the learners. It is advised to use the plural first person pronoun "we".
Run a pilot of the walkthrough on a small group of learners.
Repeat and improve!
Suggested Tasks by Phase
- Phrase the problem in your own words
- Write three examples of input-output pairs for the problem
- Write the solution of the problem or of a function (in the docstring)
- Write a design (either as text or as a diagram) for a problem or a sub-problem
- Choose a data structure and reason about it
- Describe how to solve the problem "by hand" for one specific input
- Solve a Parson's Puzzle (or the two-dimensional flavor ). It can be created and embedded in Jupyter Notebook with http://parsons.problemsolving.io
- Write code according to a design (either done by the learner or given in the walkthrough)
- Draw an environment diagram
- Answer questions about it
- Write a docstring to a function
- Give meaningful names for variables in the code
- Extract its design (e.g., write a "design tweet" with a maximum of 240 characters)
- Discuss its design - why did the solver choose that particular design and not another, especially pay attention to the data structures
- Write test cases for the problem or function
- Fix a bug in a given piece of code
- Describe a bug you had while solving the problem, and explain how you fixed it
- Evaluate a given piece of code
- Evaluate your code
- Reflect on your problem solving process
Repeat & Improve
- Refactor a given piece of code (e.g., because of speed or design issues)
Additional ideas and inspiration for tasks can be found in the Exercise types chapter from "Teaching Tech Together" and in the Catalog of pedagogical patterns chapter from "Teaching and Learning with Jupyter".
- A Walkthrough is a written guided description of the journey from a problem to a solution.
- It aims to teach how to solve programming problems in a methodical and thoughtful manner using the model.
- The conceptual roots of the walkthrough as a teaching method are the ideas of worked examples and literate programming .
- It is designed to prompt self-explanation by the learners.
- Jupyter Notebook serves as the medium, and it includes active learning tasks.
- Walkthroughs - Open Education Resources
- Worked and faded examples - MIT Open Learning
- Skudder, B., & Luxton-Reilly, A. (2014, January). Worked examples in computer science . In Proceedings of the Sixteenth Australasian Computing Education Conference-Volume 148 (pp. 59-64). Australian Computer Society, Inc..
- Lauren Margulieux - Research and Papers - Subgoal Labels and Worked Examples
- Literate Programming website
- Knuth, D. E. (1984). Literate programming . The Computer Journal, 27(2), 97-111.
- Exercise Types - Teaching Tech Together
- Catalog of Pedagogical Patterns - Teaching and Learning with Jupyter
- The Carpentries Curriculum Development Handbook
Copyright © 2020 Shlomi Hod. All rights reserved.
results matching " "
No results matching " ".

How to Solve Any Coding Problem

Rajat Sharma
The Pythoneers
Ever felt stuck while coding? It happens to everyone! But here’s the thing: cracking coding problems is more about strategy than raw brainpower. Whether you’re a pro or just starting out, learning how to approach problems is the real game-changer.
In this article, we’re going to break down the secrets of problem-solving in coding. We’ll show you step by step how to tackle any challenge that comes your way, from understanding the problem to finding smart solutions. Forget the jargon — we’re keeping it simple and practical. So, join us as we uncover the tricks to mastering coding problems with ease!
Understand The Problem
Understanding the problem is the crucial first step in solving any coding challenge. It involves carefully examining the problem statement, identifying the key requirements, constraints, and objectives, and ensuring a clear comprehension of what needs to be achieved. Let’s break down this process in detail:
- Read the Problem Statement: Start by reading the problem statement thoroughly. Pay close attention to every detail, including input/output requirements, any given constraints, and the problem’s context or scenario. This initial reading helps you get a broad understanding of what the problem entails.
- Identify Key Requirements: Once you’ve read the problem statement, identify the core requirements. What is the problem asking you to do? Are there specific tasks or functionalities that need to be implemented? For example, if the problem involves sorting a list of numbers, your core requirement might be to implement a sorting algorithm.
- Break Down the Problem: Sometimes, coding problems can seem overwhelming at first glance. Breaking the problem down into smaller, more manageable parts can make it easier to understand and solve. Identify the individual steps or components required to solve the problem. This might involve splitting the problem into sub-problems or identifying common patterns or algorithms that can be applied.
- Understand Constraints and Edge Cases: Many coding problems come with constraints or limitations that you need to consider when designing your solution. These constraints could include limitations on time, space, or the range of input values. Additionally, it’s essential to consider edge cases — scenarios that lie at the extremes or boundaries of the problem’s requirements — and ensure that your solution handles them correctly.
- Ask Questions: If anything in the problem statement is unclear or ambiguous, don’t hesitate to ask questions or seek clarification. This could involve discussing the problem with peers, consulting online forums or communities, or reaching out to the problem setter (if applicable). Clarifying any uncertainties upfront can save you time and prevent misunderstandings later on.
- Visualize the Problem: Sometimes, drawing diagrams, creating flowcharts, or visualizing the problem in some other way can help you understand it better. Visual representations can provide clarity and help you identify patterns or relationships between different components of the problem.
- Identify Test Cases: As you’re working to understand the problem, start thinking about potential test cases that you can use to verify your solution. Test cases should cover a range of scenarios, including typical cases, edge cases, and any special cases mentioned in the problem statement. Having a clear understanding of the expected input/output for different scenarios can guide your solution development process.
Clarify The Problem
Clarifying your approach involves devising a plan or strategy for solving the coding problem based on your understanding of its requirements and constraints. This step bridges the gap between understanding the problem and implementing a solution. Here’s how you can clarify your approach:
- Choose an Algorithm or Strategy: Based on your understanding of the problem, select an appropriate algorithmic approach or strategy to solve it. Consider factors such as the problem’s complexity, input size, and any specific requirements mentioned in the problem statement. Common algorithmic approaches include brute force, greedy algorithms, dynamic programming, and various types of search algorithms (e.g., depth-first search, breadth-first search).
- Break Down the Problem : If the problem is complex, consider breaking it down into smaller sub-problems or tasks that can be solved independently. Breaking down the problem can make it more manageable and help you focus on solving one piece at a time.
- Consider Data Structures: Think about the data structures that will be most effective for representing and manipulating the problem’s data. Choose data structures that align with the requirements of the chosen algorithm or strategy. Common data structures include arrays, linked lists, stacks, queues, trees, graphs, and hash tables.
- Pseudocode or Plan of Action: Outline your approach in pseudocode or a step-by-step plan of action. Pseudocode is a high-level description of the algorithm or solution logic, written in plain language without worrying about syntax. It helps you organize your thoughts and ensures that you have a clear roadmap for implementing your solution.
- Consider Optimization Opportunities : Look for opportunities to optimize your approach to improve its efficiency or reduce its time or space complexity. Optimization techniques may include memoization , pruning unnecessary branches in search algorithms, or reducing redundant computations.
- Anticipate Challenges: Think about potential challenges or pitfalls that you might encounter during implementation and consider how you’ll address them. This could involve handling edge cases, avoiding common mistakes, or dealing with tricky aspects of the problem that may not be immediately obvious.
- Validate Your Approach: Before diving into implementation, take a moment to validate your approach. Double-check that your chosen algorithm or strategy aligns with the problem’s requirements and constraints. Consider whether your approach is likely to produce a correct and efficient solution given the problem’s characteristics.
Start Coding
Once you’ve clarified your approach and have a clear plan of action, it’s time to start coding! This phase involves translating your algorithmic approach or pseudocode into actual code using a programming language of your choice. Here’s how to approach the coding phase effectively:
- Set Up Your Environment : Before you start coding, make sure your development environment is set up and ready to go. This includes ensuring that you have the necessary tools, libraries, and dependencies installed, as well as creating any project files or directories you’ll need.
- Translate Pseudocode into Code : If you’ve outlined your approach using pseudocode , use it as a guide to start writing your code. Begin by translating each step of the pseudocode into actual code statements in your chosen programming language. Focus on writing clear, concise, and readable code that accurately reflects your algorithmic approach.
- Start with the Main Function or Entry Point: Identify the main function or entry point of your program where execution will begin. This is typically where you’ll define any necessary input parameters, initialize variables, and call other functions or methods as needed.
- Implement Helper Functions or Modules: Depending on the complexity of the problem, you may need to implement additional helper functions or modules to handle specific tasks or sub-problems. Break down your solution into modular components and implement them incrementally, testing each component as you go along.
- Use Meaningful Variable Names and Comments: Write code that is easy to understand by using meaningful variable names and adding comments to explain complex or non-obvious sections of code. Comments can also serve as reminders of your thought process and rationale behind certain design decisions.
- Test Your Code Frequently: Test your code frequently as you write it to catch any errors or bugs early on. Start by testing individual components or functions in isolation to ensure they behave as expected, and then gradually integrate them into your main solution. Use a variety of test cases to validate your code’s correctness and robustness.
- Debug and Refactor as Needed: If you encounter any errors or unexpected behavior while testing your code, don’t panic! Debugging is a natural part of the coding process. Use debugging tools provided by your programming environment to identify and fix any issues, and don’t hesitate to refactor or optimize your code as needed to improve clarity, efficiency, or maintainability.
- Iterate and Improve: Coding is an iterative process, so don’t expect to get everything right on the first try. Keep iterating on your code, testing different scenarios, and refining your solution until you’re satisfied with the results. Don’t be afraid to seek feedback from peers or mentors if you’re stuck or unsure about the best approach.
Refine and Optimize
Refining and optimizing your code is a crucial step in the coding process that involves improving the efficiency, readability, and overall quality of your solution. Here’s how to refine and optimize your code effectively:
- Review and Debug: Before optimizing your code, review it thoroughly to identify any errors, inefficiencies, or areas for improvement. Use debugging tools and techniques to pinpoint and fix any bugs or unexpected behavior. Ensuring that your code works correctly is the first step towards optimization.
- Analyze Performance: Analyze the performance of your code to identify bottlenecks or areas where optimization is needed. This may involve measuring the runtime complexity (Big O notation) of your algorithms, profiling your code to identify hotspots, or using built-in performance monitoring tools provided by your programming environment.
- Optimize Algorithms: If your code contains inefficient algorithms or data structures, consider optimizing them for better performance. This may involve replacing inefficient algorithms with more efficient ones, optimizing loop structures, or reducing redundant computations. Look for opportunities to minimize time complexity (e.g., by using binary search instead of linear search) or space complexity (e.g., by using in-place algorithms instead of allocating extra memory).
- Refactor Code: Refactoring involves restructuring your code to improve its readability, maintainability, and overall design without changing its external behavior. Look for areas of your code that are overly complex, repetitive, or poorly organized, and refactor them to make them cleaner and more understandable. This may involve extracting reusable functions or classes, eliminating code duplication, or simplifying nested logic.
- Use Built-in Functions and Libraries : Take advantage of built-in functions and libraries provided by your programming language or framework to streamline your code. Built-in functions are often optimized for performance and reliability, so using them can lead to more efficient and concise solutions. However, be mindful of the overhead associated with external dependencies and avoid unnecessary imports or function calls.
- Optimize Data Structures: Choose data structures that are well-suited to the problem at hand and optimize their usage to minimize memory overhead and improve performance. Consider using specialized data structures (e.g., sets, dictionaries, heaps) that provide efficient operations for common tasks such as searching, insertion, and deletion. Additionally, optimize the usage of arrays and collections by pre-allocating memory where possible and avoiding unnecessary resizing or copying.
- Benchmark and Test: After making optimizations to your code, benchmark its performance against the original version to measure the impact of your changes. Use profiling tools to identify any remaining bottlenecks or areas for improvement, and continue iterating on your code until you’re satisfied with its performance. Don’t forget to test your optimized code thoroughly to ensure that it still produces correct results across a variety of test cases.
- Document Changes: Finally, document any significant changes or optimizations you’ve made to your code to make it easier for others (and your future self) to understand and maintain. Use comments, commit messages, or documentation files to explain the rationale behind your optimizations and any trade-offs you’ve made in terms of performance, readability, or complexity.
In the end, mastering coding problem-solving is a journey of continuous learning and improvement. By honing your skills, practicing regularly, and embracing challenges, you can become a more proficient and confident coder. So, roll up your sleeves, dive into the code, and let your problem-solving prowess shine! Keep coding , we will met soon.

Written by Rajat Sharma
I am a Developer/Analyst, I will geek you about Python, Machine Learning, Databases, Programming methods and Data Structures
Text to speech
Python Practice for Beginners: 15 Hands-On Problems

- online practice
Want to put your Python skills to the test? Challenge yourself with these 15 Python practice exercises taken directly from our Python courses!
There’s no denying that solving Python exercises is one of the best ways to practice and improve your Python skills . Hands-on engagement with the language is essential for effective learning. This is exactly what this article will help you with: we've curated a diverse set of Python practice exercises tailored specifically for beginners seeking to test their programming skills.
These Python practice exercises cover a spectrum of fundamental concepts, all of which are covered in our Python Data Structures in Practice and Built-in Algorithms in Python courses. Together, both courses add up to 39 hours of content. They contain over 180 exercises for you to hone your Python skills. In fact, the exercises in this article were taken directly from these courses!
In these Python practice exercises, we will use a variety of data structures, including lists, dictionaries, and sets. We’ll also practice basic programming features like functions, loops, and conditionals. Every exercise is followed by a solution and explanation. The proposed solution is not necessarily the only possible answer, so try to find your own alternative solutions. Let’s get right into it!
Python Practice Problem 1: Average Expenses for Each Semester
John has a list of his monthly expenses from last year:
He wants to know his average expenses for each semester. Using a for loop, calculate John’s average expenses for the first semester (January to June) and the second semester (July to December).
Explanation
We initialize two variables, first_semester_total and second_semester_total , to store the total expenses for each semester. Then, we iterate through the monthly_spending list using enumerate() , which provides both the index and the corresponding value in each iteration. If you have never heard of enumerate() before – or if you are unsure about how for loops in Python work – take a look at our article How to Write a for Loop in Python .
Within the loop, we check if the index is less than 6 (January to June); if so, we add the expense to first_semester_total . If the index is greater than 6, we add the expense to second_semester_total .
After iterating through all the months, we calculate the average expenses for each semester by dividing the total expenses by 6 (the number of months in each semester). Finally, we print out the average expenses for each semester.
Python Practice Problem 2: Who Spent More?
John has a friend, Sam, who also kept a list of his expenses from last year:
They want to find out how many months John spent more money than Sam. Use a for loop to compare their expenses for each month. Keep track of the number of months where John spent more money.
We initialize the variable months_john_spent_more with the value zero. Then we use a for loop with range(len()) to iterate over the indices of the john_monthly_spending list.
Within the loop, we compare John's expenses with Sam's expenses for the corresponding month using the index i . If John's expenses are greater than Sam's for a particular month, we increment the months_john_spent_more variable. Finally, we print out the total number of months where John spent more money than Sam.
Python Practice Problem 3: All of Our Friends
Paul and Tina each have a list of their respective friends:
Combine both lists into a single list that contains all of their friends. Don’t include duplicate entries in the resulting list.
There are a few different ways to solve this problem. One option is to use the + operator to concatenate Paul and Tina's friend lists ( paul_friends and tina_friends ). Afterwards, we convert the combined list to a set using set() , and then convert it back to a list using list() . Since sets cannot have duplicate entries, this process guarantees that the resulting list does not hold any duplicates. Finally, we print the resulting combined list of friends.
If you need a refresher on Python sets, check out our in-depth guide to working with sets in Python or find out the difference between Python sets, lists, and tuples .
Python Practice Problem 4: Find the Common Friends
Now, let’s try a different operation. We will start from the same lists of Paul’s and Tina’s friends:
In this exercise, we’ll use a for loop to get a list of their common friends.
For this problem, we use a for loop to iterate through each friend in Paul's list ( paul_friends ). Inside the loop, we check if the current friend is also present in Tina's list ( tina_friends ). If it is, it is added to the common_friends list. This approach guarantees that we test each one of Paul’s friends against each one of Tina’s friends. Finally, we print the resulting list of friends that are common to both Paul and Tina.
Python Practice Problem 5: Find the Basketball Players
You work at a sports club. The following sets contain the names of players registered to play different sports:
How can you obtain a set that includes the players that are only registered to play basketball (i.e. not registered for football or volleyball)?
This type of scenario is exactly where set operations shine. Don’t worry if you never heard about them: we have an article on Python set operations with examples to help get you up to speed.
First, we use the | (union) operator to combine the sets of football and volleyball players into a single set. In the same line, we use the - (difference) operator to subtract this combined set from the set of basketball players. The result is a set containing only the players registered for basketball and not for football or volleyball.
If you prefer, you can also reach the same answer using set methods instead of the operators:
It’s essentially the same operation, so use whichever you think is more readable.
Python Practice Problem 6: Count the Votes
Let’s try counting the number of occurrences in a list. The list below represent the results of a poll where students were asked for their favorite programming language:
Use a dictionary to tally up the votes in the poll.
In this exercise, we utilize a dictionary ( vote_tally ) to count the occurrences of each programming language in the poll results. We iterate through the poll_results list using a for loop; for each language, we check if it already is in the dictionary. If it is, we increment the count; otherwise, we add the language to the dictionary with a starting count of 1. This approach effectively tallies up the votes for each programming language.
If you want to learn more about other ways to work with dictionaries in Python, check out our article on 13 dictionary examples for beginners .
Python Practice Problem 7: Sum the Scores
Three friends are playing a game, where each player has three rounds to score. At the end, the player whose total score (i.e. the sum of each round) is the highest wins. Consider the scores below (formatted as a list of tuples):
Create a dictionary where each player is represented by the dictionary key and the corresponding total score is the dictionary value.
This solution is similar to the previous one. We use a dictionary ( total_scores ) to store the total scores for each player in the game. We iterate through the list of scores using a for loop, extracting the player's name and score from each tuple. For each player, we check if they already exist as a key in the dictionary. If they do, we add the current score to the existing total; otherwise, we create a new key in the dictionary with the initial score. At the end of the for loop, the total score of each player will be stored in the total_scores dictionary, which we at last print.
Python Practice Problem 8: Calculate the Statistics
Given any list of numbers in Python, such as …
… write a function that returns a tuple containing the list’s maximum value, sum of values, and mean value.
We create a function called calculate_statistics to calculate the required statistics from a list of numbers. This function utilizes a combination of max() , sum() , and len() to obtain these statistics. The results are then returned as a tuple containing the maximum value, the sum of values, and the mean value.
The function is called with the provided list and the results are printed individually.
Python Practice Problem 9: Longest and Shortest Words
Given the list of words below ..
… find the longest and the shortest word in the list.
To find the longest and shortest word in the list, we initialize the variables longest_word and shortest_word as the first word in the list. Then we use a for loop to iterate through the word list. Within the loop, we compare the length of each word with the length of the current longest and shortest words. If a word is longer than the current longest word, it becomes the new longest word; on the other hand, if it's shorter than the current shortest word, it becomes the new shortest word. After iterating through the entire list, the variables longest_word and shortest_word will hold the corresponding words.
There’s a catch, though: what happens if two or more words are the shortest? In that case, since the logic used is to overwrite the shortest_word only if the current word is shorter – but not of equal length – then shortest_word is set to whichever shortest word appears first. The same logic applies to longest_word , too. If you want to set these variables to the shortest/longest word that appears last in the list, you only need to change the comparisons to <= (less or equal than) and >= (greater or equal than), respectively.
If you want to learn more about Python strings and what you can do with them, be sure to check out this overview on Python string methods .
Python Practice Problem 10: Filter a List by Frequency
Given a list of numbers …
… create a new list containing only the numbers that occur at least three times in the list.
Here, we use a for loop to iterate through the number_list . In the loop, we use the count() method to check if the current number occurs at least three times in the number_list . If the condition is met, the number is appended to the filtered_list .
After the loop, the filtered_list contains only numbers that appear three or more times in the original list.
Python Practice Problem 11: The Second-Best Score
You’re given a list of students’ scores in no particular order:
Find the second-highest score in the list.
This one is a breeze if we know about the sort() method for Python lists – we use it here to sort the list of exam results in ascending order. This way, the highest scores come last. Then we only need to access the second to last element in the list (using the index -2 ) to get the second-highest score.
Python Practice Problem 12: Check If a List Is Symmetrical
Given the lists of numbers below …
… create a function that returns whether a list is symmetrical. In this case, a symmetrical list is a list that remains the same after it is reversed – i.e. it’s the same backwards and forwards.
Reversing a list can be achieved by using the reverse() method. In this solution, this is done inside the is_symmetrical function.
To avoid modifying the original list, a copy is created using the copy() method before using reverse() . The reversed list is then compared with the original list to determine if it’s symmetrical.
The remaining code is responsible for passing each list to the is_symmetrical function and printing out the result.
Python Practice Problem 13: Sort By Number of Vowels
Given this list of strings …
… sort the list by the number of vowels in each word. Words with fewer vowels should come first.
Whenever we need to sort values in a custom order, the easiest approach is to create a helper function. In this approach, we pass the helper function to Python’s sorted() function using the key parameter. The sorting logic is defined in the helper function.
In the solution above, the custom function count_vowels uses a for loop to iterate through each character in the word, checking if it is a vowel in a case-insensitive manner. The loop increments the count variable for each vowel found and then returns it. We then simply pass the list of fruits to sorted() , along with the key=count_vowels argument.
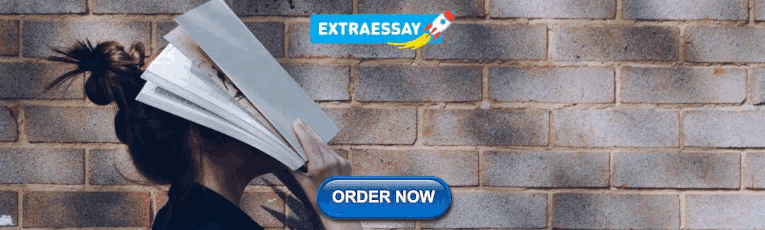
Python Practice Problem 14: Sorting a Mixed List
Imagine you have a list with mixed data types: strings, integers, and floats:
Typically, you wouldn’t be able to sort this list, since Python cannot compare strings to numbers. However, writing a custom sorting function can help you sort this list.
Create a function that sorts the mixed list above using the following logic:
- If the element is a string, the length of the string is used for sorting.
- If the element is a number, the number itself is used.
As proposed in the exercise, a custom sorting function named custom_sort is defined to handle the sorting logic. The function checks whether each element is a string or a number using the isinstance() function. If the element is a string, it returns the length of the string for sorting; if it's a number (integer or float), it returns the number itself.
The sorted() function is then used to sort the mixed_list using the logic defined in the custom sorting function.
If you’re having a hard time wrapping your head around custom sort functions, check out this article that details how to write a custom sort function in Python .
Python Practice Problem 15: Filter and Reorder
Given another list of strings, such as the one below ..
.. create a function that does two things: filters out any words with three or fewer characters and sorts the resulting list alphabetically.
Here, we define filter_and_sort , a function that does both proposed tasks.
First, it uses a for loop to filter out words with three or fewer characters, creating a filtered_list . Then, it sorts the filtered list alphabetically using the sorted() function, producing the final sorted_list .
The function returns this sorted list, which we print out.
Want Even More Python Practice Problems?
We hope these exercises have given you a bit of a coding workout. If you’re after more Python practice content, head straight for our courses on Python Data Structures in Practice and Built-in Algorithms in Python , where you can work on exciting practice exercises similar to the ones in this article.
Additionally, you can check out our articles on Python loop practice exercises , Python list exercises , and Python dictionary exercises . Much like this article, they are all targeted towards beginners, so you should feel right at home!
You may also like
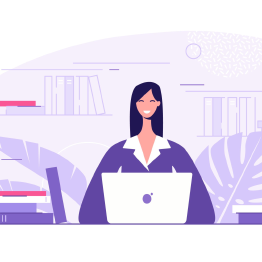
How Do You Write a SELECT Statement in SQL?
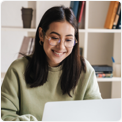
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
- The Anaconda Distribution of Python
- Installing Anaconda on Windows
- Installing Anaconda on MacOS
- Installing Anaconda on Linux
- Installing Python from Python.org
- Review Questions
Why Python?
You might be wondering "Why should I solve problems with Python?" There are other programming languages in the world such as MATLAB, LabView, C++ and Java. What makes Python useful for solving problems?
Python is a powerful programming language
Python defines the types of objects you build into your code. Unlike some other languages such as C, you do not need to declare the object type. The object type is also mutable, you can change the type of object easily and on the fly. There is a wide array of object types built into Python. Objects can change in size. Python objects can also contain mixed data types. Strings and floating point numbers can be part of the same list.
Python has an extensive Standard Library. A huge number of object types, functions and methods are available for use without importing any external modules. These include math functions, list methods, and calls to a computer's system. There is a lot that can be done with the Python Standard Library. The first couple of chapters of this book will just use the standard library. It can do a lot.
Python has over 100,000 external packages available for download and use. They are easy to install off of the Python Package Index, commonly called PyPI ("pie pee eye"). There is a Python package for just about everything. There are packages which can help you: interact with the web, make complex computations, calculate unit conversions, plot data, work with .csv, .xls, and .pdf files, manipulate images and video, read data from sensors and test equipment, train machine learning algorithms, design web apps, work with GIS data, work with astronautical data. There are and many more Python packages added to PyPI every day. In this book, we will use some of the more useful Python packages for problem solvers such as NumPy, Matplotlib, and SymPy.
Python is easy to learn and use
One way Problem solvers code solutions faster in Python faster than coding solutions in other programming languages is that Python is easy to learn and use. Python programs tend to be shorter and quicker to write than a program which completes a similar function in another languages. In the rapid design, prototype, test, iterate cycle programming solutions in Python can be written and tested quickly. Python is also an easy language for fellow problem solvers on your team to learn. Python's language syntax is also quite human readable. While programmers can become preoccupied with a program's runtime, it is development time that takes the longest.
Python is transportable
Python can be installed and run on each of the three major operating systems: Windows, Mac and Linux. On Mac and Linux Python comes installed out of the box. Just open up a terminal in on a MacOS or Linux machine and type python . That's it, you are now using Python. On Windows, I recommend downloading and installing the Anaconda distribution of Python. The Anaconda distribution of Python is free and can be installed on all three major operating systems.
Python is free
Some computer languages used for problem solving such as MATLAB and LabView cost money to download and install. Python is free to download and use. Python is also open source and individuals are free to modify, contribute to, and propose improvements to Python. All of the packages available on the Python Package Index are free to download and install. Many more packages, scripts and utilities can be found in open source code repositories on GitHub and BitBucket.
Python is growing
Python is growing in popularity. Python is particularly growing in the data sciences and in use with GIS systems, physical modeling, machine learning and computer vision. These are growing team problem-solving areas for engineers.

Member-only story
Best Way to Solve Python Coding Questions
Learn how to effectively tackle python coding questions.

Luay Matalka
Towards Data Science
There is certainly some controversy regarding the benefits of Python coding websites such as codewars or leetcode, and whether or not using them actually makes us better programmers. Despite that, many people still use them to prepare for Python interview questions, keeping their Python programming skills sharp, and/or just for fun. Nevertheless, there’s definitely a place for these resources for any Python programmer or data scientist.
In this tutorial, we’ll look at the best way to extract the most utility out of these python coding problems. We will look at a fairly simple Python coding question and work through the proper steps to solve it. This includes first coming up with a plan or outline using pseudocode, and then solving it in different ways starting with the simplest solution.
Python Coding Question
We need to write a function that takes a single integer value as input, and returns the sum of the integers from zero up to and including that input. The function should return 0 if a non-integer value is passed in.
So if we pass in the number 5 to the function, then it would return the sum of the integers 0 through 5, or (0+1+2+3+4+5) , which equals 15. If we…
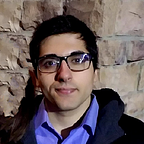
Written by Luay Matalka
Lead Machine Learning Engineer, specializing in MLOps, with a passion for teaching.
Text to speech
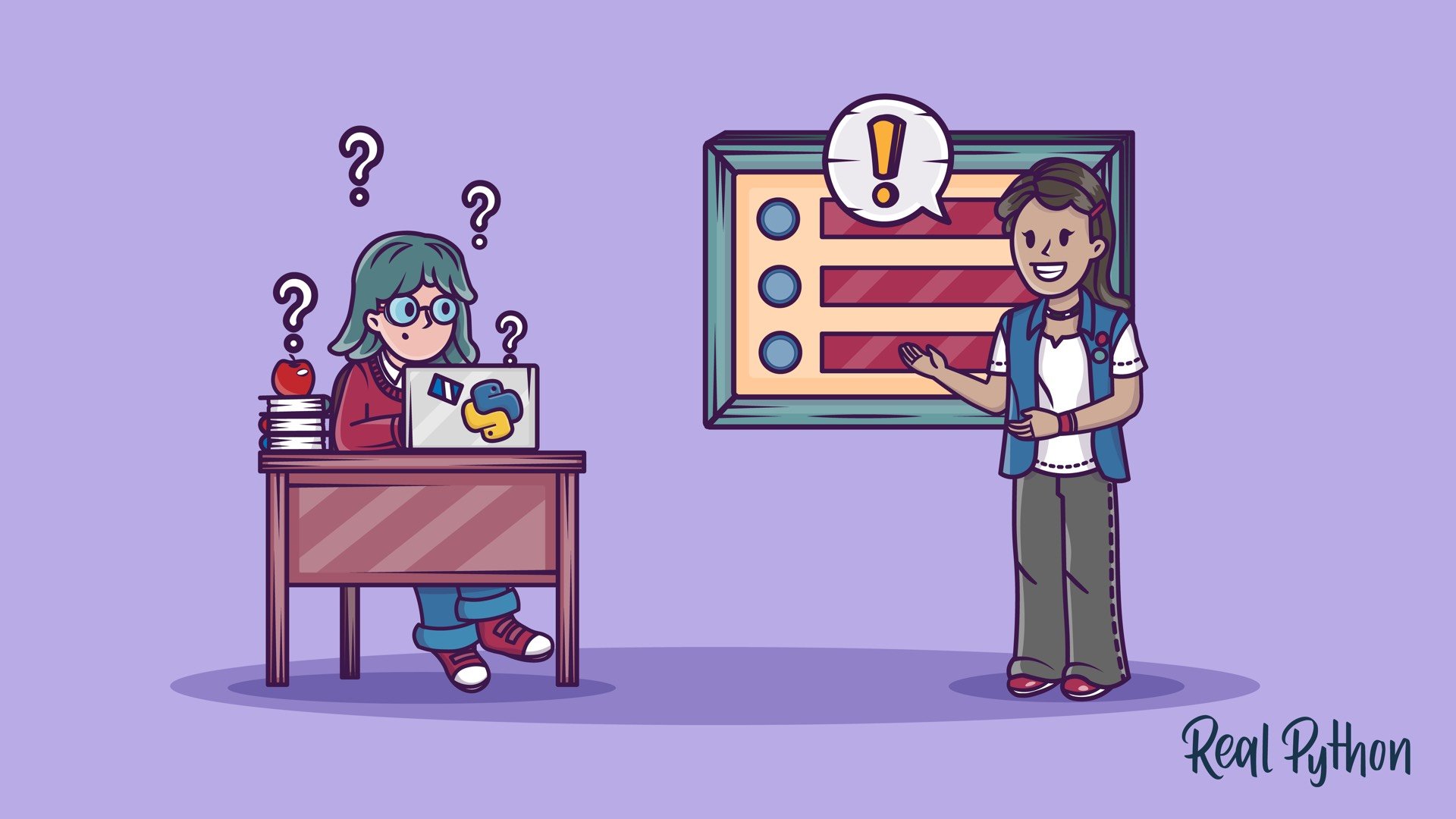
11 Beginner Tips for Learning Python Programming
Table of Contents
Tip #1: Code Everyday
Tip #2: write it out, tip #3: go interactive, tip #4: take breaks, tip #5: become a bug bounty hunter, tip #6: surround yourself with others who are learning, tip #7: teach, tip #8: pair program, tip #9: ask “good” questions, tip #10: build something, anything, tip #11: contribute to open source, go forth and learn.
Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: 11 Beginner Tips for Learning Python
We are so excited that you have decided to embark on the journey of learning Python ! One of the most common questions we receive from our readers is “What’s the best way to learn Python?”
I believe that the first step in learning any programming language is making sure that you understand how to learn. Learning how to learn is arguably the most critical skill involved in computer programming.
Why is knowing how to learn so important? The answer is simple: as languages evolve, libraries are created, and tools are upgraded. Knowing how to learn will be essential to keeping up with these changes and becoming a successful programmer.
In this article, we will offer several learning strategies that will help jump start your journey of becoming a rockstar Python programmer!
Free Download: Get a sample chapter from Python Basics: A Practical Introduction to Python 3 to see how you can go from beginner to intermediate in Python with a complete curriculum, up-to-date for Python 3.8.
Make It Stick
Here are some tips to help you make the new concepts you are learning as a beginner programmer really stick:
Consistency is very important when you are learning a new language. We recommend making a commitment to code every day. It may be hard to believe, but muscle memory plays a large part in programming. Committing to coding everyday will really help develop that muscle memory. Though it may seem daunting at first, consider starting small with 25 minutes everyday and working your way up from there.
Check out the First Steps With Python Guide for information on setup as well as exercises to get you started.
As you progress on your journey as a new programmer, you may wonder if you should be taking notes. Yes, you should! In fact, research suggests that taking notes by hand is most beneficial for long-term retention. This will be especially beneficial for those working towards the goal of becoming a full-time developer, as many interviews will involve writing code on a whiteboard.
Once you start working on small projects and programs, writing by hand can also help you plan your code before you move to the computer. You can save a lot of time if you write out which functions and classes you will need, as well as how they will interact.
Whether you are learning about basic Python data structures (strings, lists, dictionaries, etc.) for the first time, or you are debugging an application, the interactive Python shell will be one of your best learning tools. We use it a lot on this site too!
To use the interactive Python shell (also sometimes called a “Python REPL” ), first make sure Python is installed on your computer. We’ve got a step-by-step tutorial to help you do that. To activate the interactive Python shell, simply open your terminal and run python or python3 depending on your installation. You can find more specific directions here .
Note: For a full guide to the standard Python REPL, check out The Python Standard REPL: Try Out Code and Ideas Quickly .
Now that you know how to start the shell, here are a few examples of how you can use the shell when you are learning:
Learn what operations can be performed on an element by using dir():
The elements returned from dir() are all of the methods (i.e. actions) that you can apply to the element. For example:
Notice that we called the upper() method. Can you see what it does? It makes all of the letters in the string uppercase! Learn more about these built-in methods under “Manipulating strings” in this tutorial .
Learn the type of an element:
Use the built-in help system to get full documentation:
Import libraries and play with them:
Run shell commands:
When you are learning, it is important to step away and absorb the concepts. The Pomodoro Technique is widely used and can help: you work for 25 minutes, take a short break, and then repeat the process. Taking breaks is critical to having an effective study session, particularly when you are taking in a lot of new information.
Breaks are especially important when you are debugging. If you hit a bug and can’t quite figure out what is going wrong, take a break. Step away from your computer, go for a walk, or chat with a friend.
In programming, your code must follow the rules of a language and logic exactly, so even missing a quotation mark will break everything. Fresh eyes make a big difference.
Speaking of hitting a bug, it is inevitable once you start writing complex programs that you will run into bugs in your code. It happens to all of us! Don’t let bugs frustrate you. Instead, embrace these moments with pride and think of yourself as a bug bounty hunter.
When debugging, it is important to have a methodological approach to help you find where things are breaking down. Going through your code in the order in which it is executed and making sure each part works is a great way to do this.
Once you have an idea of where things might be breaking down, insert the following line of code into your script import pdb; pdb.set_trace() and run it. This is the Python debugger and will drop you into interactive mode. The debugger can also be run from the command line with python -m pdb <my_file.py> .
Make It Collaborative
Once things start to stick, expedite your learning through collaboration. Here are some strategies to help you get the most out of working with others.
Though coding may seem like a solitary activity, it actually works best when you work together. It is extremely important when you are learning to code in Python that you surround yourself with other people who are learning as well. This will allow you to share the tips and tricks you learn along the way.
Don’t worry if you don’t know anyone. There are plenty of ways to meet others who are passionate about learning Python! Find local events or Meetups or join PythonistaCafe , a peer-to-peer learning community for Python enthusiasts like you!
It is said that the best way to learn something is to teach it. This is true when you are learning Python. There are many ways to do this: whiteboarding with other Python lovers, writing blog posts explaining newly learned concepts, recording videos in which you explain something you learned, or simply talking to yourself at your computer. Each of these strategies will solidify your understanding as well as expose any gaps in your understanding.
Pair programming is a technique that involves two developers working at one workstation to complete a task. The two developers switch between being the “driver” and the “navigator.” The “driver” writes the code, while the “navigator” helps guide the problem solving and reviews the code as it is written. Switch frequently to get the benefit of both sides.
Pair programming has many benefits: it gives you a chance to not only have someone review your code, but also see how someone else might be thinking about a problem. Being exposed to multiple ideas and ways of thinking will help you in problem solving when you got back to coding on your own.
People always say there is no such thing as a bad question, but when it comes to programming, it is possible to ask a question badly. When you are asking for help from someone who has little or no context on the problem you are trying to solve, its best to ask GOOD questions by following this acronym:
- G : Give context on what you are trying to do, clearly describing the problem.
- O : Outline the things you have already tried to fix the issue.
- O : Offer your best guess as to what the problem might be. This helps the person who is helping you to not only know what you are thinking, but also know that you have done some thinking on your own.
- D : Demo what is happening. Include the code, a traceback error message, and an explanation of the steps you executed that resulted in the error. This way, the person helping does not have to try to recreate the issue.
Good questions can save a lot of time. Skipping any of these steps can result in back-and-forth conversations that can cause conflict. As a beginner, you want to make sure you ask good questions so that you practice communicating your thought process, and so that people who help you will be happy to continue helping you.
Make Something
Most, if not all, Python developers you speak to will tell you that in order to learn Python, you must learn by doing. Doing exercises can only take you so far: you learn the most by building.
For beginners, there are many small exercises that will really help you become confident with Python, as well as develop the muscle memory that we spoke about above. Once you have a solid grasp on basic data structures (strings, lists, dictionaries, sets), object-oriented programming , and writing classes, it’s time to start building!
What you build is not as important as how you build it. The journey of building is truly what will teach you the most. You can only learn so much from reading Real Python articles and courses. Most of your learning will come from using Python to build something. The problems you will solve will teach you a lot.
There are many lists out there with ideas for beginner Python projects. Here are some ideas to get you started:
- Number guessing game
- Simple calculator app
- Dice roll simulator
- Bitcoin Price Notification Service
If you find it difficult to come up with Python practice projects to work on, watch this video . It lays out a strategy you can use to generate thousands of project ideas whenever you feel stuck.
In the open-source model, software source code is available publicly, and anyone can collaborate. There are many Python libraries that are open-source projects and take contributions. Additionally, many companies publish open-source projects. This means you can work with code written and produced by the engineers working in these companies.
Contributing to an open-source Python project is a great way to create extremely valuable learning experiences. Let’s say you decide to submit a bugfix request: you submit a “pull request” for your fix to be patched into the code.
Next, the project managers will review your work, providing comments and suggestions. This will enable you to learn best practices for Python programming, as well as practice communicating with other developers.
For additional tips and tactics that will help you break into the open-source world, check out the video embedded below:
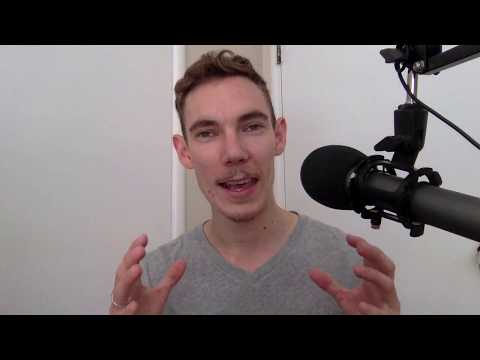
Now that you have these strategies for learning, you are ready to begin your Python journey! Find Real Python’s Beginners Roadmap for Learning here ! We also offer a beginner’s level Python course , which uses interesting examples to help you learn programming and web development.
Happy Coding!
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
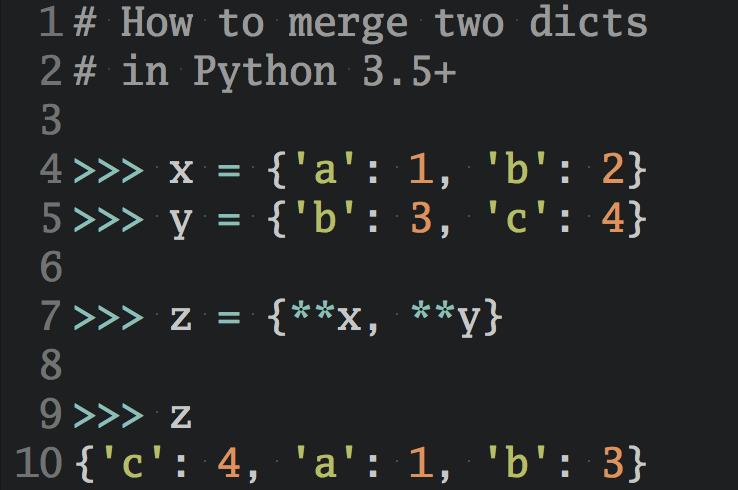
About Krishelle Hardson-Hurley
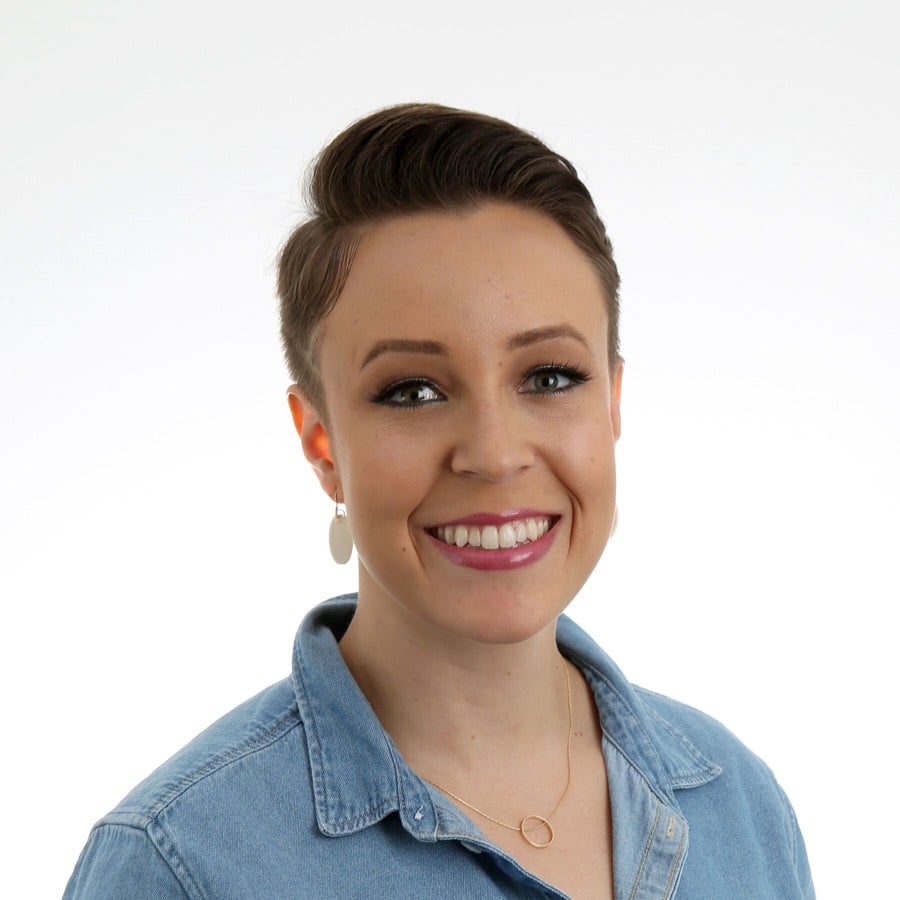
After 6 years of teaching high school math, Krishelle switched careers and now works as a Site Reliability Engineer at Dropbox in San Francisco, CA.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
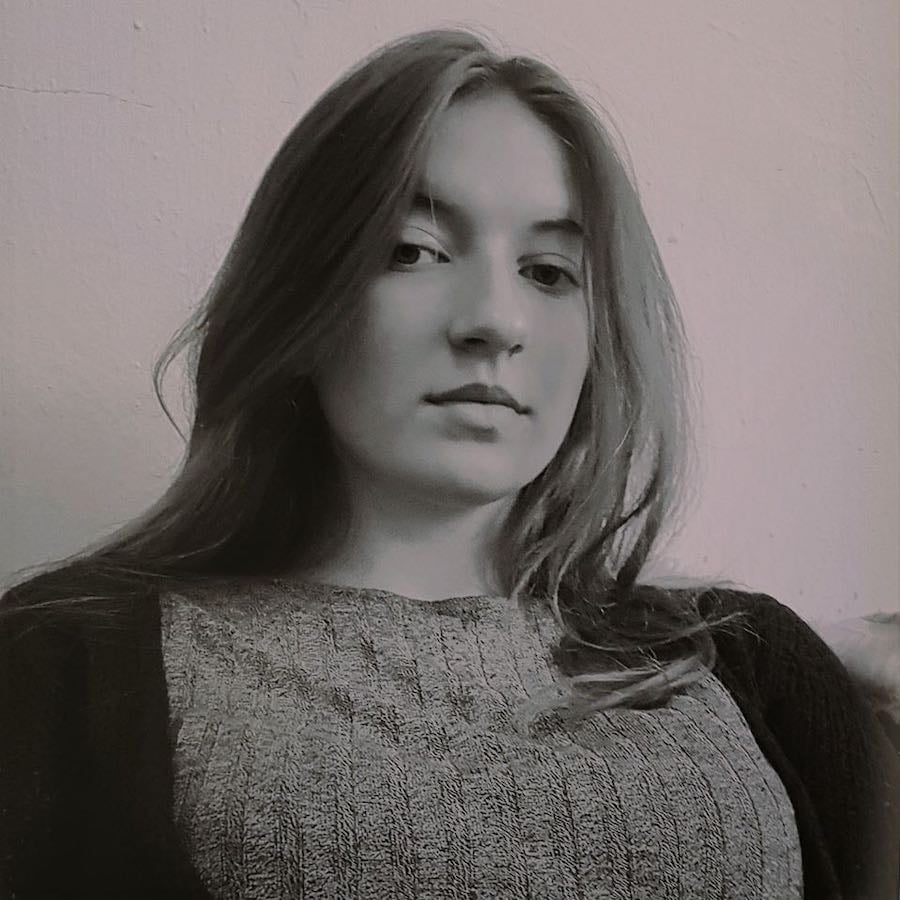
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: basics career
Recommended Video Course: 11 Beginner Tips for Learning Python
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In
Almost there! Complete this form and click the button below to gain instant access:
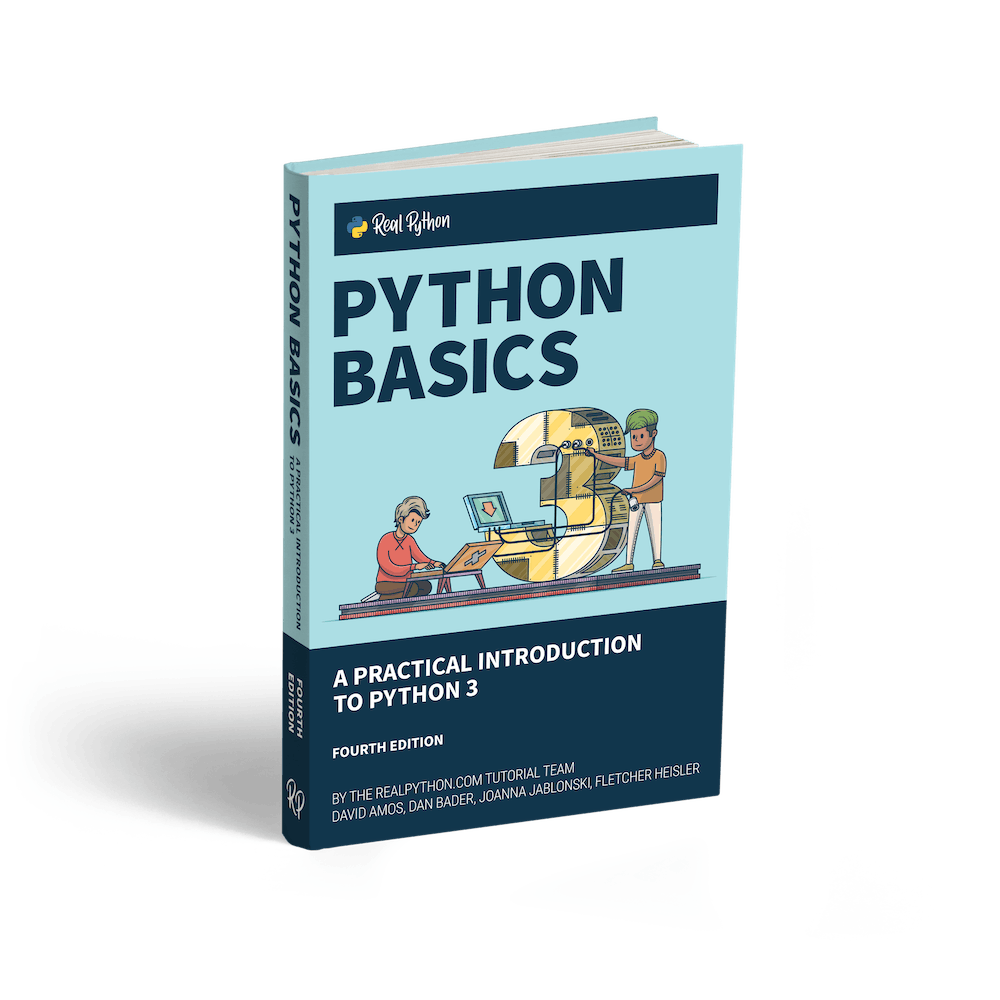
"Python Basics: A Practical Introduction to Python 3" – Free Sample Chapter (PDF)
🔒 No spam. We take your privacy seriously.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Simplest way to solve mathematical equations in Python
I want to solve a set of equations, linear, or sometimes quadratic. I don't have a specific problem, but often, I have been in this situation often.
It is simple to use wolframalpha.com , the web equivalent of Mathematica, to solve them. But that doesn't provide the comfort and convenience of an iPython shell.
Is there a simple library to work on linear and quadratic equations from a python shell?
Personally, I find it extremely convenient to use the Casio 991 MS scientific calculator. I know how to set variables, solve equations, and do a lot. I want such a tool preferably usable from within an ipython shell. I am surprised not to have found any. I'm not impressed enough by sage; perhaps I am missing something.

- Do you know the Maxima Language and the WxMaxima interface? I find it cozier than IPython – Foad S. Farimani Commented Nov 24, 2017 at 9:31
16 Answers 16
sympy is exactly what you're looking for.
- Upvoted. Let's see a demo! I just posted an answer with a demo of sympy to help get people started in it. – Gabriel Staples Commented Aug 20, 2022 at 7:26
You discount the best answer as unacceptable.
Your question is "I want a free Computer Algebra System that I can use in Python."
The answer is "SAGE does that."
Have you looked at maxima/macsyma? SAGE provides bindings for it, and that's one of the more powerful free ones.
http://maxima.sourceforge.net/
Here is how to solve your original question using Python (via Sage). This basically clarifies the remark Paul McMillan makes above.
For inexact solutions, read up on linear programming and sequential quadratic optimization , then search for Python libraries that performs such optimizations for you.
If the equations require integer solutions, you should search for Diophantine equation solvers for Python.
Just note that using a simple solver for Project Euler is missing the point. The fun, and educational part, is learning how to solve it yourself using primitive methods!
- they can give exact solution - depends on model, constraints & tolerance ... for Continuous Problems - use Gradient Descend Algos... for Mixed Integer Problems see Branch and Bound Method - but it is used in LP Solvers internally and you should not care about algo itself, find libs (scipy.optimize, pulp, GEKKO, Networkx etc depending on task to solve) – JeeyCi Commented Nov 13, 2023 at 6:42
A free web-service for solving large-scale systems of nonlinear equations (1 million+) is APMonitor.com. There is a browser interface and an API to Python / MATLAB. The API to Python is a single script (apm.py) that is available for download from the apmonitor.com homepage. Once the script is loaded into a Python code, it gives the ability to solve problems of:
- Nonlinear equations
- Differential and algebraic equations
- Least squares model fitting
- Moving horizon estimation
- Nonlinear model predictive control
For the new user, the APM Python software has a Google Groups forum where a user can post questions. There are bi-weekly webinars that showcase optimization problems in operations research and engineering.
Below is an example of an optimization problem (hs71.apm).
The optimization problem is solved with the following Python script:
Have you looked at SciPy ?
It has an example in the tutorials on solving linear algebra:
http://docs.scipy.org/doc/scipy/reference/tutorial/linalg.html#solving-linear-system

For reference: Wolfram Alpha's solution :
In python, using sympy's solver module (note that it assumes all equations are set equal to zero):
And of course, a != 1000, as a-1000 is the denominator of the two equations.
I have just started using GNU Scientific Library , which however is C library. Looks like there are Python bindings too. So, it might be worth looking at.
I'd use Octave for this but I agree, the syntax of Octave isn't what I'd call thrilling (and the docs always confuse me more than they help, too).
SymPy symbolic Python library demo: symbolically solving math and integrals & pretty-printing the output
From @Autoplectic:
While I have a tendency to write some of the longest answers on Stack Overflow, that is the shortest answer I've seen on Stack Overflow.
Let's add a basic demo.
References and tutorials you'll need:
- Basic Operations
- Simplification
Calculus demo I came up with (see here for the main tutorial I looked at to get started):
Short version:
Longer version:
eRCaGuy_hello_world/math/sympy_integral_and_printing.py :
Note that using pprint(integral) is the same as print(pretty(integral)) .
Output of running the above commands:
The SymPy symbolic math library in Python can do pretty much any kind of math, solving equations, simplifying, factoring, substituting values for variables, pretty printing, converting to LaTeX format, etc. etc. It seems to be a pretty robust solver in my very limited use so far. I recommend trying it out.
Installing it, for me (tested on Linux Ubuntu), was as simple as:

I don't think there is a unified way of dealing with both linear and quadratic (or generally nonlinear) equations simultaneously. With linear systems, python has bindings to linear algebra and matrix packages. Nonlinear problems tend to be solved on a case by case basis.
- SAGE's maxima bindings can deal with pretty much anything you throw at them. – Paul McMillan Commented Oct 29, 2009 at 9:00
It depends on your needs:
If you want an interactive graphical interface, then sage is probably the best solution.
If you want to avoid using a graphical interface, but you still want to do computer algebra, then sympy or maxima may cover your needs. (sympy looks very promising, but it still have a long way to go before they can replace mathematica).
If you don't really need symbolic algrebra, but you need a way to program with matrices, solve differential equations, and minimize functions, then scipy or octave are excelent starting points.
Take a look at this:
http://openopt.org/FuncDesignerDoc#Solving_systems_of_nonlinear_equations
It is extremely easy to use and quite powerful
Well, I just googled into this page by accident. I see many suggestions regarding this and that software tool, but does any tool actually provide an answer? The actual answer is:
[a,b,c] = [200,375,425]
How did I get this? By writing a quick program in the Maxima programming language to find it via "brute force" searching. It only took about 10 minutes to write, seeing as how I'm familiar with the Maxima language. It took a few seconds for the program to run. Here is the program:
euler_solve():= block ( [ a, b, A, B, end:1000],
You can just cut and paste the above code into the wxMaxima user interface, which I run under Ubuntu and not MS Windows. Then you just enter the function name: euler_solve(), hit return, wait a few seconds, and out pops the answer. This particular kind of problem is so simple that you could use any general-purpose programming language to do the search.
- 2 [200, 375, 425] is one potential solution, but there's an infinite set of solutions to this set of equations. [-499000, 999, 499001] is another. One numeric solution is probably not what the OP is looking for. – Nate Commented Mar 16, 2012 at 15:41
- This answer is not relevant since the question is specific Python. The comment about infinite solutions isn't either since the problem that this question refers to (Project Euler 9) has constraints and specifically limits its solution to a single tuple. – anddam Commented Jun 4, 2014 at 10:10
Try applying Bisection method in py to find the root given an interval:

On second thoughts, I went through sage in detail and clearly it is the best math free software available.
Just some of the different python math related libraries, it integrates is absolutely awesome.
Mathematics packages contained in Sage:
Other packages contained in Sage:
- 11 It's impolite to answer your own question with a duplicate of answers provided by others, and then to accept your own answer. Instead, accept someone else's correct answer, and edit your question to include the information you found useful, or reply in a comment. – Paul McMillan Commented Jan 6, 2011 at 6:57
- Paul, these points are not the primary driving force of me, nor the site, I think. Get over that obsession. People are here for sharing information. – lprsd Commented Jan 6, 2011 at 12:25
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python math numpy scipy equation or ask your own question .
- The Overflow Blog
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- Conservation of the determinant of density matrix
- Doesn't counting hole and electron current lead to double-counting of actual current?
- How much easier/harder would it be to colonize space if humans found a method of giving ourselves bodies that could survive in almost anything?
- What should be the affiliation of PhD student who submitted thesis but yet to defend, in a conference talk slides?
- Is consciousness a prerequisite for knowledge?
- Soldering RG179 coaxial cable directly to PCB
- Identifications in differential geometry
- Who owns code contributed to a license-free repository?
- Is it fine to call a 26 year old character a young adult?
- In what instances are 3-D charts appropriate?
- Driveway electric run using existing service poles
- What does "mdw" in "mdwtab"/"mdwlist/mdwmath" packages mean?
- Create random points using minimum distance calculated on the fly using ModelBuilder in ArcGIS Pro
- Does an airplane fly less or more efficiently after an mid-flight engine failure?
- Expensive constructors. Should they exist? Should they be replaced?
- Lore reasons for being faithless
- Reheating beans makes them mushy and unpeeled
- Why is Namibia killing game animals for food rather than allowing more big game hunting?
- How is an inverting opamp adder circuit able to regulate its feedback?
- Is a company liable for "potential" harms?
- Percentage changes versus absolute changes when comparing rankings
- Do passengers transiting in YVR (Vancouver) from international to US go through Canadian immigration?
- If Starliner returns safely on autopilot, can this still prove that it's safe? Could it be launched back up to the ISS again to complete its mission?
- I have two identical LaTeX files, with different file names. Only one yields "undefined references." Why is this happening?
Say "Hello, World!" With Python Easy Max Score: 5 Success Rate: 96.19%
Python if-else easy python (basic) max score: 10 success rate: 89.61%, arithmetic operators easy python (basic) max score: 10 success rate: 97.35%, python: division easy python (basic) max score: 10 success rate: 98.67%, loops easy python (basic) max score: 10 success rate: 98.07%, write a function medium python (basic) max score: 10 success rate: 90.28%, print function easy python (basic) max score: 20 success rate: 97.30%, list comprehensions easy python (basic) max score: 10 success rate: 97.64%, find the runner-up score easy python (basic) max score: 10 success rate: 94.21%, nested lists easy python (basic) max score: 10 success rate: 91.80%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
Python Programming
Python Basic Exercise for Beginners
Updated on: August 29, 2024 | 498 Comments
This essential Python exercise helps Python beginners learn necessary Python skills quickly.
Immerse yourself in the practice of Python’s foundational concepts, such as loops, control flow, data types, operators, list, strings, input-output, and built-in functions. This beginner’s exercise is sure to elevate your understanding of Python.
Also, See :
- Python Quizzes : Solve quizzes to test your knowledge of fundamental concepts.
- Python Basics : Learn the basics to solve this exercise.
What questions are included in this Python fundamental exercise?
- The exercise contains 15 Python programs to solve , ranging from beginner to intermediate difficulty.
- The hints and solutions are provided for each question.
- Tips and essential learning resources accompany each question . These will assist you in solving the exercise and empower you with the knowledge to tackle similar problems in the future, boosting your confidence and capability. As you progress, you’ll become more familiar with the basics of Python.
Use Online Code Editor to solve exercises.
This Python exercise covers questions on the following topics :
- Python for loop and while loop
- Python list , set , tuple , dictionary , input, and output
Also, try to solve the basic Python Quiz for beginners
Exercise 1: Calculate the multiplication and sum of two numbers
Given two integer numbers, write a Python code to return their product only if the product is equal to or lower than 1000. Otherwise, return their sum.
Expected Output :
- Accept user input in Python
- Calculate an Average in Python
- Create a function that will take two numbers as parameters
- Next, Inside a function, multiply two numbers and save their product in a product variable
- Next, use the if condition to check if the product >1000 . If yes, return the product
- Otherwise, use the else block to calculate the sum of two numbers and return it.
Exercise 2: Print the Sum of a Current Number and a Previous number
Write a Python code to iterate the first 10 numbers, and in each iteration, print the sum of the current and previous number.
Reference article for help :
- Python range() function
- Calculate sum and average in Python
- Create a variable called previous_num and assigning it to 0
- Next, iterate through the first 10 numbers using the for loop and range() function
- Next, display the current number ( i ), the previous number, and the addition of both numbers in each iteration of a loop
- Finally, you need to update the previous_num for the next iteration. To do this, assign the value of the current number to the previous number ( previous_num = i).
Exercise 3: Print characters present at an even index number
Write a Python code to accept a string from the user and display characters present at an even index number.
For example, str = "PYnative" . so your code should display ‘P’, ‘n’, ‘t’, ‘v’.
Reference article for help: Python Input and Output
- Use the Python input() function to accept a string from a user.
- Calculate the length of the string using the len() function
- Next, iterate characters of a string using the loop and range() function.
- Use start = 0, stop = len(s)-1, and step =2. the step is 2 because we want only even index numbers
- In each iteration of a loop, use s[i] to print characters present at the current even index number
Solution 1 :
Solution 2 : Using list slicing
Exercise 4: Remove first n characters from a string
Write a Python code to remove characters from a string from 0 to n and return a new string.
For Example:
- remove_chars("PYnative", 4) so output must be tive . Here, you need to remove the first four characters from a string.
- remove_chars("PYnative", 2) so output must be native . Here, you need to remove the first two characters from a string.
Note : n must be less than the length of the string.
Use string slicing to get a substring. For example, remove the first four characters using s[4:] .
Also, try to solve Python string exercises
Exercise 5: Check if the first and last numbers of a list are the same
Write a code to return True if the list’s first and last numbers are the same. If the numbers are different, return False .
Exercise 6: Display numbers divisible by 5
Write a Python code to display numbers from a list divisible by 5
Also, try to solve Python list Exercise
Exercise 7: Find the number of occurrences of a substring in a string
Write a Python code to find how often the substring “ Emma ” appears in the given string.
Use string method count() .
Solution 1 : Use the count() method
Solution 2 : Without the string method
Exercise 8: Print the following pattern
Hint : Print Pattern using for loop
Exercise 9: Check Palindrome Number
Write a Python code to check if the given number is palindrome. A palindrome number is a number that is the same after reverse. For example, 545 is the palindrome number .
- Reverse the given number and save it in a different variable
- Use the if condition to check if the original and reverse numbers are identical. If yes, return True .
Exercise 10: Merge two lists using the following condition
Given two lists of numbers, write a Python code to create a new list such that the latest list should contain odd numbers from the first list and even numbers from the second list .
- Create an empty list named result_list
- Iterate the first list using a for loop
- In each iteration check if the current number is odd using the num % 2 != 0 formula. If the current number is odd, add it to the result list
- Now, Iterate the second list using a loop.
- In each iteration check if the current number is even using the num % 2 == 0 formula. If the current number is even, add it to the result list.
- Print the result list.
Note: Try to solve the Python list exercises
Exercise 11: Get each digit from a number in the reverse order.
For example, If the given integer number is 7536 , the output shall be “ 6 3 5 7 “, with a space separating the digits.
Use while loop
Exercise 12: Calculate income tax
Calculate income tax for the given income by adhering to the rules below
Taxable Income | Rate (in %) |
---|---|
First $10,000 | 0 |
Next $10,000 | 10 |
The remaining | 20 |
For example, suppose the income is 45000, and the income tax payable is
10000*0% + 10000*10% + 25000*20% = $6000.
Exercise 13: Print multiplication table from 1 to 10
See : How to use nested loops in Python
- Create the outer for loop to iterate numbers from 1 to 10. Thus, the outer loop’s total number of iterations is 10.
- Create an inner loop to iterate 10 times.
- For each outer loop iteration, the inner loop will execute 10 times.
- The number is 1 in the first iteration of the outer loop. In the next, it is 2, and so on until it reaches 10.
- In each iteration of an inner loop, we perform a simple calculation: the multiplication of two numbers. (current outer number and current inner number)
Exercise 14: Print a downward half-pyramid pattern of stars
Exercise 15: get an int value of base raises to the power of exponent.
Write a function called exponent(base, exp) that returns an int value of base raises to the power of exp.
Note here exp is a non-negative integer, and the base is an integer.
Expected output
I want to hear from you. What do you think of this essential exercise? If you have better alternative answers to the above questions, please help others by commenting on this exercise.
I have shown only 15 questions in this exercise because we have Topic-specific exercises to cover each topic exercise in detail. Please refer to all Python exercises .
Did you find this page helpful? Let others know about it. Sharing helps me continue to create free Python resources.
About Vishal

I’m Vishal Hule , the Founder of PYnative.com. As a Python developer, I enjoy assisting students, developers, and learners. Follow me on Twitter .
Related Tutorial Topics:
Python exercises and quizzes.
Free coding exercises and quizzes cover Python basics, data structure, data analytics, and more.
- 15+ Topic-specific Exercises and Quizzes
- Each Exercise contains 10 questions
- Each Quiz contains 12-15 MCQ
Loading comments... Please wait.
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .
Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Online Python Code Editor
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
Crafting an effective problem statement
Aug 31, 2024
Posted by: Regine Fe Arat
Crafting a clear and concise problem statement is an essential skill in project management. It’s a powerful tool that you can use for effective problem-solving as it guides teams toward innovative solutions and measurable outcomes.
Whether you’re a seasoned project manager or a newcomer to the field, being able to write problem statements can significantly enhance your ability to tackle complex challenges and drive meaningful change.
A problem statement concisely describes an issue or challenge that needs to be addressed. An effective problem statement frames the issue in a way that facilitates a deeper understanding and guides the problem-solving process.
At its core, a well-crafted problem statement should capture the essence of the challenge at hand, providing enough context for stakeholders to grasp the issue’s significance. It helps you find the most appropriate solution and ensures that all team members are aligned in their understanding of the problem’s scope and implications.
In this comprehensive guide, you’ll find out what problem statements are and what types you can use. You’ll also find practical examples and actionable tips to help you create impactful problem statements of your own.
What are the key components of a problem statement?
Here are the three key components of a problem statement:
The problem
You should clearly state the core issue or challenge to be addressed. This is the heart of your problem statement. Articulate it in a way that’s easy to understand and free from ambiguity.
The method used to solve the problem
While the problem statement itself shouldn’t propose specific solutions, it can outline the general approach or methodology you’ll use to address the issue. For example, you might mention the type of research, analysis or problem-solving techniques your team will employ.
The purpose, statement of objective and scope
This component outlines why addressing the problem is important and what the desired outcome looks like. It should clarify the goals of the problem-solving effort and define the boundaries of what you’ll address. This helps focus efforts and set clear expectations for what the project or initiative aims to achieve.
When to use a problem statement
A problem statement is a versatile tool that you can use across various scenarios in both professional and personal contexts. They are particularly valuable in the following cases:
- Initiating new projects: a problem statement helps define the project’s purpose and sets clear objectives from the outset.
- Addressing organizational challenges: it provides a structured approach to tackling complex issues within a company or team.
- Conducting research: researchers use problem statements to focus their investigations and define the scope of their studies.
- Presenting ideas to stakeholders: a well-formulated problem statement can effectively communicate the need for change or investment to decision-makers.
- Personal goal-setting: even in individual pursuits, problem statements can help clarify objectives and motivate action.
Types of problem statements
Understanding different types of problem statements can help you choose the best approach for your specific situation.
Let’s explore three common types:
The status quo problem statement
This type of problem statement focuses on the current state of affairs and highlights the gap between the existing situation and the desired outcome.
It’s particularly effective when you are addressing ongoing issues or systemic problems within an organization.
Example: “Our customer support team currently handles 150 tickets, on average, per day with a resolution time of 48 hours. This prolonged response time has led to a 15% decrease in customer satisfaction scores over the past quarter, potentially impacting our retention rates and brand reputation.”
Destination problem statement
A destination problem statement emphasizes the desired future state or goal.
It’s ideal for situations where you want to inspire change and motivate teams to work toward a specific vision.
Example: “We aim to create a seamless onboarding experience for new employees that reduces the time to full productivity from 12 to six weeks while increasing new hire satisfaction scores by 25% within the next fiscal year.”
The stakeholder problem statement
This type of problem statement focuses on the impact of an issue on specific individuals or groups.
It’s particularly useful when you need to highlight the human element of a problem and garner support for change.
Example: “Junior software developers in our organization report feeling overwhelmed and unsupported, with 60% expressing dissatisfaction with their professional growth opportunities. This has resulted in a 30% turnover rate among this group in the past year, leading to increased recruitment costs and knowledge loss.”
How to write a problem statement
Crafting an effective problem statement takes practice and attention to detail. Follow these steps to create impactful problem statements:
Understand the problem
Before putting pen to paper, invest time in thoroughly understanding the issue at hand. Gather data, conduct interviews with stakeholders and observe the problem in action if possible. This deep understanding will form the foundation of your problem statement.
Articulate the problem in simple, straightforward language. Avoid jargon or overly technical terms that might confuse readers. Your goal is to ensure that anyone reading the statement can quickly grasp the core issue.
Provide context
Include relevant background information that helps readers understand the problem’s significance. This might include historical data, industry benchmarks or organizational goals that the issue is affecting.
Identify the root cause
Dig deeper to uncover the underlying reasons for the problem. Avoid focusing on symptoms. Instead, strive to identify the fundamental issues that need to be addressed. Tools like the “5 whys” technique can be helpful in this process.
Be specific
Use concrete details and quantifiable metrics whenever possible. Instead of saying, “Customer satisfaction is low,” specify, “Customer satisfaction scores have dropped by 15% in the past quarter.” This precision helps create a clear picture of the problem’s scope and impact.
Use measurable criteria
Incorporate measurable elements that can be used to track progress and determine when the problem has been resolved. This might include specific metrics, timeframes or benchmarks.
Make it feasible
Ensure the problem statement describes an issue the organization can actually address. You’ll need to be realistic.
Consider your organization’s resources and constraints. While ambition is important, an overly broad or unattainable goal can be demotivating and unhelpful.
Avoid solution language
Resist the temptation to propose solutions in the problem statement. The goal is to clearly define the problem, not to prescribe how it should be solved. This approach encourages creative thinking and enables you and your team to consider a range of potential solutions.
Consider the audience
Tailor your problem statement to the intended audience. The level of detail and technical language may vary depending on whether you’re presenting to executives, team members or external stakeholders.
Seek feedback
Share your draft problem statement with colleagues or stakeholders to gather their input. Fresh perspectives can help identify blind spots or areas that need clarification.
Revise and refine
Refine your problem statement based on the feedback you receive. Don’t be afraid to go through multiple iterations to achieve the most clear and impactful statement possible.
Test for objectivity
Review your problem statement to ensure it remains objective and free from bias. Avoid language that assigns blame or makes assumptions about causes or solutions.
Challenges of writing a problem statement
While problem statements can be a powerful tool for problem-solving, you may face several common challenges when writing yours. Being aware of these pitfalls can help you avoid them and create more effective problem statements.
Making it too complicated and lacking detail
One of the most frequent issues in problem statement writing is finding the right balance between detail and clarity.
Oversimplifying the problem can lead to a statement that’s too vague to be actionable. On the other hand, including too much detail can obscure the core issue and make the statement difficult to understand.
To overcome this challenge, focus on the essential elements of the problem. Start with a clear, concise statement about the issue, then add only the most relevant contextual details. Use specific, measurable criteria to define the problem’s scope and impact, but avoid getting bogged down in excessive technical jargon or minute, unhelpful details.
Ignoring stakeholders’ perspectives
Another common pitfall is failing to consider the diverse perspectives of all the stakeholders the problem affects. This can result in a problem statement that doesn’t fully capture the issue’s complexity or fails to resonate with key decision-makers.
To address this challenge, make an effort to gather input from a wide range of stakeholders before finalizing your problem statement. This might include conducting interviews, surveys or focus groups with employees, customers, partners or other relevant parties.
Incorporate these diverse viewpoints into your problem statement to create a more comprehensive and compelling representation of the issue.
Misalignment with organizational goals
Sometimes, problem statements can be well-crafted but fail to align with broader organizational objectives. This misalignment can lead to wasted resources and efforts on issues that, while important, may not be critical to the company’s overall success.
To ensure your problem statement aligns with the organization’s goals, review your company’s mission statement, strategic plans and key performance indicators before you get started. Consider how the problem you’re addressing relates to these broader objectives.
If possible, explicitly link the problem and its potential resolution to specific goals or metrics in your statement.
Failing to review and revise
An effective problem statement often requires multiple iterations and refinements. Many project managers make the mistake of treating their first draft as the final version, missing opportunities to improve clarity, precision and impact.
To overcome this challenge:
- Build time for revision into your problem statement writing process.
- After crafting your initial draft, step away from it for a short period.
- Return with fresh eyes to critically evaluate and refine your statement.
- Share it with colleagues or mentors for feedback. Be open to making substantive changes based on their input.
The last card
Being able to write problem statements is a valuable skill that can significantly enhance your problem-solving capabilities and drive meaningful change within your organization. They enable you to set the stage for innovative solutions and improved processes – but to do this, you’ll need to clearly articulate challenges, provide context and focus on measurable outcomes.
A well-crafted problem statement is a powerful tool for aligning teams, securing resources and guiding decision-making. It’s the foundation for effective problem-solving strategies. As you get better at writing problem statements, you’ll find that complex challenges become more manageable and your ability to drive positive change increases.
At Pip Decks, we’re passionate about equipping professionals with the tools and knowledge they need to excel in their roles. Whether you’re looking to improve your problem-solving skills, enhance team collaboration or develop your leadership abilities, you’ll find the answers you need in our expert-written card decks.
Level up your career with Pip Club
Join 100,000+ leaders who get unique tips every week on storytelling, leadership and productivity - plus exclusive how-to guides, first-dibs on upcoming Pip Decks and our very best discounts.
Nearly there...
Check your inbox to confirm your email.
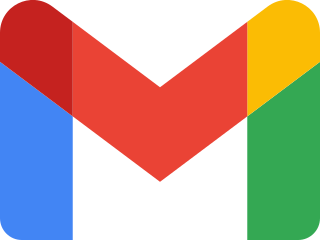
No spam, no email sharing - ever. Privacy Policy
One of the few newsletters I look forward to. — Dave Cunningham, Head of DesignOps @ NHS

- Data Science
- Data Analysis
- Data Visualization
- Machine Learning
- Deep Learning
- Computer Vision
- Artificial Intelligence
- AI ML DS Interview Series
- AI ML DS Projects series
- Data Engineering
- Web Scrapping
How Neural Networks Solve the XOR Problem
The XOR (exclusive OR) is a simple logic gate problem that cannot be solved using a single-layer perceptron (a basic neural network model). We can solve this using neural networks. Neural networks are powerful tools in machine learning.
In this article, we are going to discuss what is XOR problem, how we can solve it using neural networks, and also a simple code to demonstrate this.
Table of Content
What is the XOR Problem?
Why single-layer perceptrons fail, how multi-layer neural networks solve xor, mathematics behind the mlp solution, geometric interpretation, training the neural network to solve xor problem.
The XOR operation is a binary operation that takes two binary inputs and produces a binary output. The output of the operation is 1 only when the inputs are different.
Below is the truth table for XOR:
Input A | Input B | XOR Output |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
The main problem is that a single-layer perceptron cannot solve this problem because the data is not linearly separable i.e. we cannot draw a straight line to separate the output classes (0s and 1s)
A single-layer perceptron can solve problems that are linearly separable by learning a linear decision boundary.
Mathematically, the decision boundary is represented by:
[Tex]y = \text{step}(\mathbf{w} \cdot \mathbf{x} + b)[/Tex]
- [Tex]\mathbf{w}[/Tex] is the weight vector.
- [Tex]\mathbf{x}[/Tex] is the input vector.
- [Tex]b[/Tex] is the bias term.
- [Tex]\text{step}[/Tex] is the activation function, often a Heaviside step function that outputs 1 if the input is positive and 0 otherwise.
For linearly separable data, the perceptron can adjust the weights [Tex]\mathbf{w}[/Tex] and bias [Tex]b[/Tex] during training to correctly classify the data. However, because XOR is not linearly separable, no single line (or hyperplane) can separate the outputs 0 and 1, making a single-layer perceptron inadequate for solving the XOR problem.
A multi-layer neural network which is also known as a feedforward neural network or multi-layer perceptron is able to solve the XOR problem. There are multiple layer of neurons such as input layer, hidden layer, and output layer.
The working of each layer:
- Input Layer: This layer takes the two inputs (A and B).
- Hidden Layer: This layer applies non-linear activation functions to create new, transformed features that help separate the classes.
- Output Layer: This layer produces the final XOR result.
Let’s break down the mathematics behind how an MLP can solve the XOR problem.
Step 1: Input to Hidden Layer Transformation
Consider an MLP with two neurons in the hidden layer, each applying a non-linear activation function (like the sigmoid function). The output of the hidden neurons can be represented as:
[Tex]h_1 = \sigma(w_{11} A + w_{12} B + b_1)[/Tex]
[Tex]h_2 = \sigma(w_{21} A + w_{22} B + b_2)[/Tex]
- [Tex]\sigma(x) = \frac{1}{1 + e^{-x}}[/Tex] is the sigmoid activation function.
- [Tex]w_{ij}[/Tex] are the weights from the input neurons to the hidden neurons.
- [Tex]b_i[/Tex] are the biases for the hidden neurons.
Activation functions such as the sigmoid or ReLU (Rectified Linear Unit) introduce non-linearity into the model. It enables the neural network to handle complex patterns like XOR. Without these functions, the network would behave like a simple linear model, which is insufficient for solving XOR.
Step 2: Hidden Layer to Output Layer Transformation
The output neuron combines the outputs of the hidden neurons to produce the final output:
[Tex]\text{Output} = \sigma(w_{31} h_1 + w_{32} h_2 + b_3)[/Tex]
Where [Tex]w_{3i}[/Tex] are the weights from the hidden neurons to the output neuron, and [Tex]b_3[/Tex] is the bias for the output neuron.
Step 3: Learning Weights and Biases
During the training process, the network adjusts the weights [Tex]w_{ij}[/Tex] and biases [Tex]b_i[/Tex] using backpropagation and gradient descent to minimize the error between the predicted output and the actual XOR output.
Example Configuration:
Let’s consider a specific configuration of weights and biases that solves the XOR problem:
- [Tex]w_{11} = 1 , w_{12} =1, b_1 =0.5[/Tex]
- [Tex]w_{21} =1 , w_{22} =1 , b_2 = -1.5[/Tex]
- [Tex]w_{31} =1, w_{32} =1, b_3 = -1[/Tex]
With these weights and biases, the network produces the correct XOR output for each input pair (A, B).
In the hidden layer, the network effectively transforms the input space into a new space where the XOR problem becomes linearly separable. This can be visualized as bending or twisting the input space such that the points corresponding to different XOR outputs (0s and 1s) are now separable by a linear decision boundary.
The neural network learns to solve the XOR problem by adjusting the weights during training. This is done using backpropagation, where the network calculates the error in its output and adjusts its internal weights to minimize this error over time. This process continues until the network can correctly predict the XOR output for all given input combinations.
The following python code implementation demonstrates how neural networks solve the XOR problem using TensorFlow and Keras:
import numpy as np import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense # Define the XOR input and output data X = np . array ([[ 0 , 0 ], [ 0 , 1 ], [ 1 , 0 ], [ 1 , 1 ]]) y = np . array ([[ 0 ], [ 1 ], [ 1 ], [ 0 ]]) # Build the neural network model model = Sequential () model . add ( Dense ( 2 , input_dim = 2 , activation = 'relu' )) # Hidden layer with 2 neurons model . add ( Dense ( 1 , activation = 'sigmoid' )) # Output layer with 1 neuron # Compile the model model . compile ( optimizer = 'adam' , loss = 'binary_crossentropy' , metrics = [ 'accuracy' ]) # Train the model model . fit ( X , y , epochs = 10000 , verbose = 0 ) # Evaluate the model _ , accuracy = model . evaluate ( X , y ) print ( f "Accuracy: { accuracy * 100 : .2f } %" ) # Make predictions predictions = model . predict ( X ) predictions = np . round ( predictions ) . astype ( int ) print ( "Predictions:" ) for i in range ( len ( X )): print ( f "Input: { X [ i ] } => Predicted Output: { predictions [ i ] } , Actual Output: { y [ i ] } " )
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 168ms/step - accuracy: 0.5000 - loss: 0.6931 Accuracy: 50.00% 1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 46ms/step Predictions: Input: [0 0] => Predicted Output: [0], Actual Output: [0] Input: [0 1] => Predicted Output: [0], Actual Output: [1] Input: [1 0] => Predicted Output: [0], Actual Output: [1] Input: [1 1] => Predicted Output: [0], Actual Output: [0]
The XOR problem is a classic example that highlights the limitations of simple neural networks and the need for multi-layer architectures. By introducing a hidden layer and non-linear activation functions, an MLP can solve the XOR problem by learning complex decision boundaries that a single-layer perceptron cannot. Understanding this solution provides valuable insight into the power of deep learning models and their ability to tackle non-linear problems in various domains.
Please Login to comment...
Similar reads.
- AI-ML-DS QnA
- AI-ML-DS With Python
- How to Delete Discord Servers: Step by Step Guide
- Google increases YouTube Premium price in India: Check our the latest plans
- California Lawmakers Pass Bill to Limit AI Replicas
- Best 10 IPTV Service Providers in Germany
- 15 Most Important Aptitude Topics For Placements [2024]
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
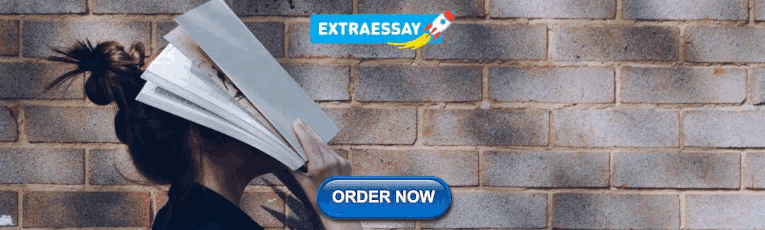
IMAGES
VIDEO
COMMENTS
An algorithm is a process or set of rules to be followed while performing calculations or other problem-solving operations. It is simply a set of steps to accomplish a certain task. In this article, we will discuss 5 major steps for efficient problem-solving. These steps are: Understanding the Problem; Exploring Examples; Breaking the Problem Down
Problem solving in Python. Learn problem solving in Python from our online course and tutorial. You will learn basic math, conditionals and step by step logic building to solve problems easily. 4.5 (3904 reviews) 18 lessons Beginner level. 50.2k Learners.
Each exercise has 10-20 Questions. The solution is provided for every question. Practice each Exercise in Online Code Editor. These Python programming exercises are suitable for all Python developers. If you are a beginner, you will have a better understanding of Python after solving these exercises. Below is the list of exercises.
Module 3 • 4 hours to complete. Everything you've learned in this course about Python is just basic building blocks that programmers use to build bigger building blocks of their own. In this module, we'll do precisely that, turning Python into a little language for drawing pictures, a DIY MS Paint. What's included.
Example: Solving a Sorting Problem. Let's look at a simple algorithmic problem: sorting a list of numbers in ascending order. Here is a Python function that uses the Bubble Sort algorithm to solve this problem: numbers[j], numbers[j + 1] = numbers[j + 1], numbers[j] return numbers. The bubble_sort function sorts the list of numbers by ...
Problem Solving with Algorithms and Data Structures using Python¶. By Brad Miller and David Ranum, Luther College. Assignments; There is a wonderful collection of YouTube videos recorded by Gerry Jenkins to support all of the chapters in this text.
Welcome to the world of problem solving with Python! This first Orientation chapter will help you get started by guiding you through the process of installing Python on your computer. By the end of this chapter, you will be able to: Describe why Python is a useful computer language for problem solvers. Describe applications where Python is used.
Lesson 3 - Problem solving techniques. In the last lesson, we learned control flow statements such as if-else and for. In this lesson, let us try to write a program for this problem: "Given a day, the program should print if it is a weekday or weekend.". $ python3 day_of_the_week.py monday. weekday. $ python3 day_of_the_week.py sunday. weekend.
Course Overview. As a developer, mastering the concepts of algorithms and being proficient in implementing them is essential to improving problem-solving skills. This course aims to equip you with an in-depth understanding of algorithms and how they can be utilized for problem-solving in Python. Starting with the basics, you'll gain a ...
Python Problem Solving. Welcome to the Python Problem Solving section. A collection of many different scenarios and problems that may arise during your Coding journey. Follow the individual links to see the main article which contains a detailed step by step break down of the solution. Q.
A programming problem solving walkthrough is a written guided description of the journey from a problem to a solution. It aims to teach how to solve programming problems in a methodical and thoughtful manner using the model. In other words, the knowledge to be learned is focused on the "how", and not on the programming language per se.
Identify the individual steps or components required to solve the problem. This might involve splitting the problem into sub-problems or identifying common patterns or algorithms that can be applied.
Python Practice Problem 1: Average Expenses for Each Semester. John has a list of his monthly expenses from last year: He wants to know his average expenses for each semester. Using a for loop, calculate John's average expenses for the first semester (January to June) and the second semester (July to December).
Website companion for the book Problem Solving with Python by Peter D. Kazarinoff
One way Problem solvers code solutions faster in Python faster than coding solutions in other programming languages is that Python is easy to learn and use. Python programs tend to be shorter and quicker to write than a program which completes a similar function in another languages. In the rapid design, prototype, test, iterate cycle ...
The several steps of this cycle are as follows : Step by step solution for a problem (Software Life Cycle) 1. Problem Definition/Specification: A computer program is basically a machine language solution to a real-life problem. Because programs are generally made to solve the pragmatic problems of the outside world.
While this solution takes a literal approach to solving the Caesar cipher problem, you could also use a different approach modeled after the .translate() solution in practice problem 2. Solution 2. The second solution to this problem mimics the behavior of Python's built-in method .translate(). Instead of shifting each letter by a given ...
In this tutorial, we learned that solving a Python problem using different methods can enhance our coding and problem-solving skills by broadening our knowledge base. We looked at an example python coding question and went through the steps of solving it. We first planned how we were going to solve it using pseudocode.
Check out the First Steps With Python Guide for information on setup as well as exercises to get you started. Tip #2: Write It Out ... The "driver" writes the code, while the "navigator" helps guide the problem solving and reviews the code as it is written. Switch frequently to get the benefit of both sides.
A free web-service for solving large-scale systems of nonlinear equations (1 million+) is APMonitor.com. There is a browser interface and an API to Python / MATLAB. The API to Python is a single script (apm.py) that is available for download from the apmonitor.com homepage. ... The optimization problem is solved with the following Python script:
*Problem Solving Techniques | Problem Solving using Python | Advance Python Tutorial | upGrad*🔥Liverpool John Moore University MS In Data Science: https://w...
Easy Python (Basic) Max Score: 10 Success Rate: 89.62%. Solve Challenge. Arithmetic Operators. Easy Python (Basic) Max Score: 10 Success Rate: 97.35%. Solve Challenge. ... Problem Solving (Basic) Python (Basic) Problem Solving (Advanced) Python (Intermediate) Difficulty. Easy. Medium. Hard. Subdomains. Introduction. Basic Data Types. Strings ...
Exercise 4: Remove first n characters from a string . Write a Python code to remove characters from a string from 0 to n and return a new string. For Example: remove_chars("PYnative", 4) so output must be tive.Here, you need to remove the first four characters from a string.
An effective problem statement frames the issue in a way that facilitates a deeper understanding and guides the problem-solving process. At its core, a well-crafted problem statement should capture the essence of the challenge at hand, providing enough context for stakeholders to grasp the issue's significance.
The XOR problem is a classic example that highlights the limitations of simple neural networks and the need for multi-layer architectures. By introducing a hidden layer and non-linear activation functions, an MLP can solve the XOR problem by learning complex decision boundaries that a single-layer perceptron cannot.