- Python Programming
- C Programming
- Numerical Methods
- Dart Language
- Computer Basics
- Deep Learning
- C Programming Examples
- Python Programming Examples
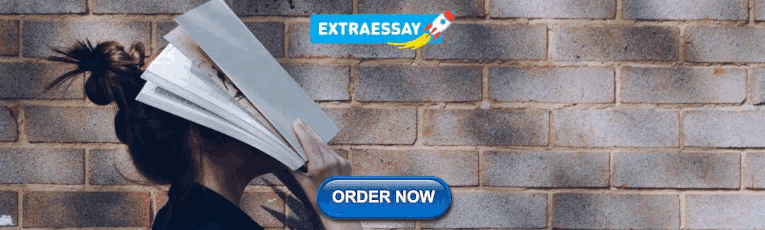
Augmented Assignment Operators in Python with Examples
Unlike normal assignment operator, Augmented Assignment Operators are used to replace those statements where binary operator takes two operands says var1 and var2 and then assigns a final result back to one of operands i.e. var1 or var2 .
For example: statement var1 = var1 + 5 is same as writing var1 += 5 in python and this is known as augmented assignment operator. Such type of operators are known as augmented because their functionality is extended or augmented to two operations at the same time i.e. we're adding as well as assigning.
List Of Augmented Assignment Operators in Python
Python has following list of augmented assignment operators:
A Comprehensive Guide to Augmented Assignment Operators in Python
Augmented assignment operators are a vital part of the Python programming language. These operators provide a shortcut for assigning the result of an operation back to a variable in an expressive and efficient manner.
In this comprehensive guide, we will cover the following topics related to augmented assignment operators in Python:
Table of Contents
What are augmented assignment operators, arithmetic augmented assignments, bitwise augmented assignments, sequence augmented assignments, advantages of augmented assignment operators, augmented assignment with mutable types, operator precedence and order of evaluation, updating multiple references, augmented assignment vs normal assignment, comparisons to other languages, best practices and style guide.
Augmented assignment operators are a shorthand technique that combines an arithmetic or bitwise operation with an assignment.
The augmented assignment operator performs the operation on the current value of the variable and assigns the result back to the same variable in a compact syntax.
For example:
Here x += 3 is equivalent to x = x + 3 . The += augmented assignment operator adds the right operand 3 to the current value of x , which is 2 . It then assigns the result 5 back to x .
This shorthand allows you to reduce multiple lines of code into a concise single line expression.
Some key properties of augmented assignment operators in Python:
- Operators act inplace directly modifying the variable’s value
- Work on mutable types like lists, sets, dicts unlike normal operators
- Generally have equivalent compound statement forms using standard operators
- Have right-associative evaluation order unlike arithmetic operators
- Available for arithmetic, bitwise, and sequence operations
Now let’s look at the various augmented assignment operators available in Python.
Augmented Assignment Operators List
Python supports augmented versions of all the arithmetic, bitwise, and sequence assignment operators.
Operator | Example | Equivalent |
---|---|---|
These perform the standard arithmetic operations like addition or exponentiation and assign the result back to the variable.
Operator | Example | Equivalent |
---|---|---|
These allow you to perform bitwise AND, OR, XOR, right shift, and left shift operations combined with assignment.
Operator | Example | Equivalent |
---|---|---|
The += operator can also be used to concatenate sequences like lists, tuples, and strings.
These operators provide a shorthand for sequence concatenation.
Some key advantages of using augmented assignment operators:
Conciseness : Performs an operation and assignment in one concise expression rather than multiple lines or steps.
Readability : The operator itself makes the code’s intention very clear. x += 3 is more readable than x = x + 3 .
Efficiency : Saves executing multiple operations and creates less intermediate objects compared to chaining or sequencing the operations. The variable is modified in-place.
For these reasons, augmented assignments should be preferred over explicit expansion into longer compound statements in most cases.
Some examples of effective usage:
- Incrementing/decrementing variables: index += 1
- Accumulating sums: total += price
- Appending to sequences: names += ["Sarah", "John"]
- Bit masking tasks: bits |= 0b100
So whenever you need to assign the result of some operation back into a variable, consider using the augmented version.
Common Mistakes to Avoid
While augmented assignment operators are very handy, some common mistakes can occur:
Augmented assignments act inplace and modify the existing object. This can be problematic with mutable types like lists:
In contrast, normal operators with immutable types create a new object:
So be careful when using augmented assignments with mutable types, as they modify the object in-place rather than creating a new object.
Augmented assignment operators have right-associativity. This can cause unexpected results:
The right-most operation y += 1 is evaluated first updating y. Then x += y uses the new value of y.
To avoid this, use parenthesis to control order of evaluation:
When you augmented assign to a variable, it updates all references to that object:
y also reflects the change since it points to the same mutable list as x .
To avoid this, reassign the variable rather than using augmented assignment:
While the augmented assignment operators provide a shorthand, they differ from standard assignment in some key ways:
Inplace modification : Augmented assignment acts inplace and modifies the existing variable rather than creating a new object.
Mutable types : Works directly on mutable types like lists, sets, and dicts unlike normal assignment.
Order of evaluation : Has right-associativity unlike left-associativity of normal assignment.
Multiple references : Affects all references to a mutable object unlike normal assignment.
In summary, augmented assignment operators combine both an operation and assignment but evaluate differently than standard operators.
Augmented assignments exist in many other languages like C/C++, Java, JavaScript, Go, Rust, etc. Some key differences to Python:
In C/C++ augmented assignments return the assigned value allowing usage in expressions unlike Python which returns None .
Java and JavaScript don’t allow augmented assignment with strings unlike Python which supports += for concatenation.
Go doesn’t have an increment/decrement operator like ++ and -- . Python’s += 1 and -= 1 serves a similar purpose.
Rust doesn’t allow built-in types like integers to be reassigned with augmented assignment and requires mutable variables be defined with mut .
So while augmented assignment is common across languages, Python provides some unique behaviors to be aware of.
Here are some best practices when using augmented assignments in Python:
Use whitespace around the operators: x += 1 rather than x+=1 for readability.
Limit chaining augmented assignments like x = y = 0 . Use temporary variables if needed for clarity.
Don’t overuse augmented assignment especially with mutable types. Reassignment may be better if the original object shouldn’t be changed.
Watch the order of evaluation with multiple augmented assignments on one line due to right-associativity.
Consider parentheses for explicit order of evaluation: x += (y + z) rather than relying on precedence.
For increments/decrements, prefer += 1 and -= 1 rather than x = x + 1 and x = x - 1 .
Use normal assignment for updating multiple references to avoid accidental mutation.
Following PEP 8 style, augmented assignments should have the same spacing and syntax as normal assignment operators. Just be mindful of potential pitfalls.
Augmented assignment operators provide a compact yet expressive shorthand for modifying variables in Python. They combine an operation and assignment into one atomic expression.
Key takeaways:
Augmented operators perform inplace modification and behave differently than standard operators in some cases.
Know the full list of arithmetic, bitwise, and sequence augmented assignment operators.
Use augmented assignment to write concise and efficient updates to variables and sequences.
Be mindful of right-associativity order of evaluation and behavior with mutable types to avoid bugs.
I hope this guide gives you a comprehensive understanding of augmented assignment in Python. Use these operators appropriately to write clean, idiomatic Python code.
- python
- variables-and-operators

Augmented Assignments
1.9. augmented assignments #.
An augmented assignment merges an assignment statement with an operator, resulting in a more concise statement. The execution of an augmented assignment involves the following steps:
Evaluate the expression on the right side of the = sign to yield a value.
Apply the operator associated with the = sign to the variable on the left of the = and the generated value. This produces another value. Store the memory address of this value in the variable on the left of the = .
Table Table 1.2 shows all the augmented assignments.
Symbol | Example | Result |
---|---|---|
+= | x = 7, x += 2 | x refers to 9 |
-= | x = 7, x -= 2 | x refers 5 |
*= | x = 7, x *= 2 | x refers to 14 |
/= | x = 7, x /= 2 | x refers to 3.5 |
//= | x = 7, x //= 2 | x refers to 3 |
%= | x = 7, x %= 2 | x refers to 1 |
**= | x = 7, x **=2 | x refers to 49 |
Python Augmented Assignment: Streamlining Your Code
Python's augmented assignment operators provide a shortcut for assigning the result of an arithmetic or bitwise operation. They are a perfect example of Python's commitment to code readability and efficiency. This blog post delves into the concept of augmented assignment in Python, exploring how these operators can make your code more concise and expressive.
Introduction to Augmented Assignment in Python
Augmented assignment is a combination of a binary operation and an assignment operation. It offers a shorthand way of writing operations that update a variable.
What is Augmented Assignment?
- Simplified Syntax : Augmented assignment operators allow you to perform an operation on a variable and then assign the result back to that variable in one concise step.
- Supported Operations : Python supports augmented assignment for various operations, including arithmetic, bitwise, and more.
Common Augmented Assignment Operators
Here are some of the most commonly used augmented assignment operators in Python:
Arithmetic Operators
Addition ( += ) : Adds a value to a variable.
Subtraction ( -= ) : Subtracts a value from a variable.
Multiplication ( *= ) : Multiplies a variable by a value.
Division ( /= ) : Divides a variable by a value.
Bitwise Operators
Bitwise AND ( &= ) , OR ( |= ) , XOR ( ^= ) : Perform bitwise operations and assign the result.
Other Operators
Modulus ( %= ) , Floor Division ( //= ) , Exponentiation ( **= ) : Useful for more complex mathematical operations.
Advantages of Using Augmented Assignment
Augmented assignment operators not only reduce the amount of code but also improve readability. They indicate an operation that modifies the variable, rather than producing a new value.
Efficiency and Readability
- Less Code : Augmented assignments reduce the verbosity of expressions.
- Clarity : These operators make it clear that the value of the variable is being updated.
Best Practices and Considerations
When using augmented assignment operators, there are a few best practices to keep in mind:
Readability : Ensure the use of augmented assignment enhances the readability of your code.
Mutability : Be cautious with mutable types. Augmented assignments on objects like lists or dictionaries modify the object in place, which can affect other references to the object.
Augmented assignment in Python is a testament to the language's philosophy of simplicity and efficiency. By incorporating these operators into your coding practice, you can write more concise and readable code, reducing the potential for errors and enhancing overall code quality. Whether you are working on mathematical operations, manipulating strings, or performing bitwise operations, augmented assignment operators are valuable tools in the Python programmer’s toolkit.
Python Essentials by Steven F. Lott
Get full access to Python Essentials and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
Augmented assignment
The augmented assignment statement combines an operator with assignment. A common example is this:
This is equivalent to
When working with immutable objects (numbers, strings, and tuples) the idea of an augmented assignment is syntactic sugar. It allows us to write the updated variable just once. The statement a += 1 always creates a fresh new number object, and replaces the value of a with the new number object.
Any of the operators can be combined with assignment. The means that += , -= , *= , /= , //= , %= , **= , >>= , <<= , &= , ^= , and |= are all assignment operators. We can see obvious parallels between sums using += , and products using *= .
In the case of mutable objects, this augmented assignment can take on special ...
Get Python Essentials now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
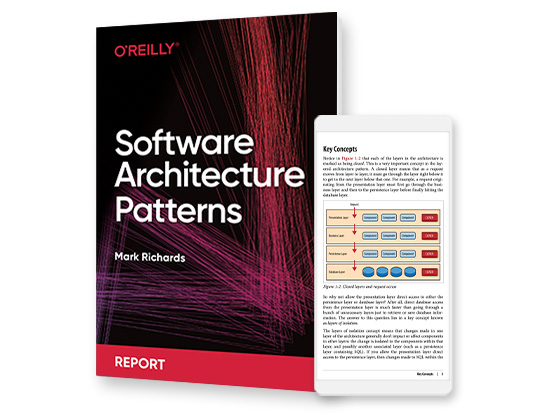
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
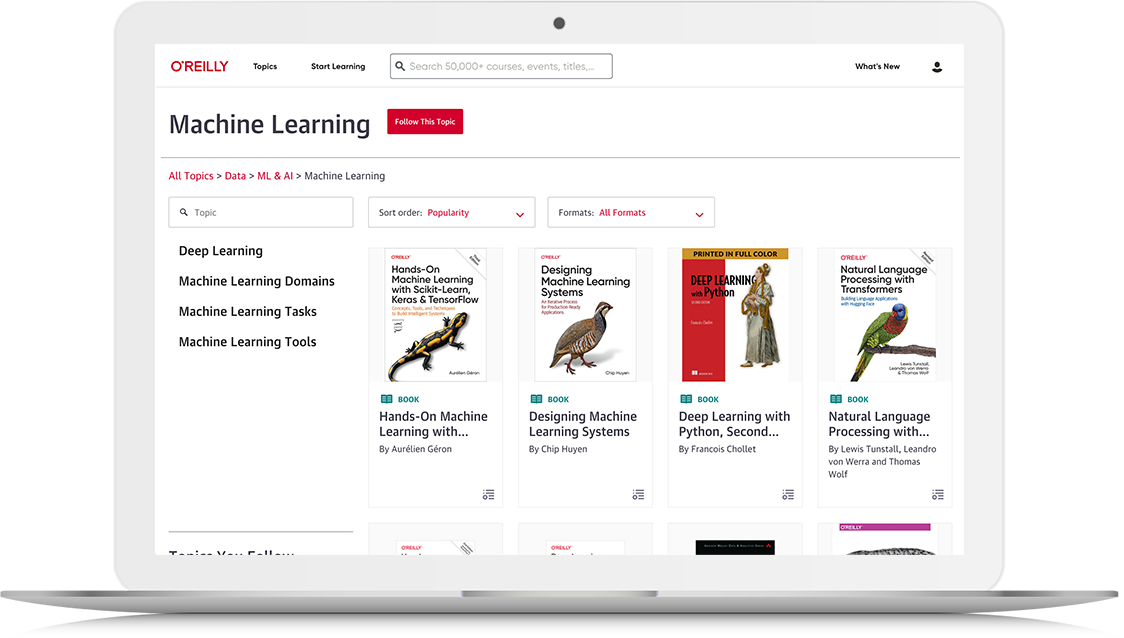
4.2 Augmented Assignment Operators
Chapter table of contents, section table of contents, previous section, next section.
Unit 1: Augmented Assignment Operators
Table of contents, augmented assignment operators.
A lot of times in programming you’ll need to update a value relative to itself. For instance, when you’re playing a video game and you collect a coin, your score might go up by 5 points relative to what it was before.
In Java, that would look like this (assume a variable score has already been delcared and initialized):
Augmented assignment operators allow us to do that with less typing:
There are augmented assignment operators for each of the arithmetic operators. In the table below, assume var is a variable that has been declared and initialized.
Augmented Assignment Operator | Normal | Augmented Assignment |
---|---|---|
Adventures in Machine Learning
Python increment operations: from augmented assignment to optimizing code, how to increment a variable in python.
If you’re new to programming in Python, you may be wondering how to increment a variable. While other programming languages have the “++” operator, Python does not.
This may seem like an inconvenience, but it’s actually a design decision based on the Pythonic way of doing things. In this article, we’ll explore how to increment a variable in Python and the reasoning behind the decision to not include the “++” operator.
In Python, you can increment a variable using the augmented assignment operator “+=”. This operator adds the value on the right side of the operator to the current value of the variable on the left side of the operator.
Let’s take a look at an example:
In this example, we first set the variable x equal to 5. Then we use the “+=” operator to add 1 to the current value of x.
Finally, we print the value of x, which is now 6. It’s worth noting that you can use the augmented assignment operator with other arithmetic operators as well.
For example:
In this example, we use the “*=” operator to multiply the current value of x by 2, effectively doubling its value. The Absence of the “++” Operator in Python
As mentioned earlier, Python does not have the “++” operator.
So why not? The answer lies in the Pythonic way of doing things.
Python is designed to be a simple, readable, and expressive language. The absence of the “++” operator is just one example of this philosophy.
When you write code in Python, you should strive to make it as clear and concise as possible. Using the “+=” operator to increment a variable is a simple and clear way to do this.
On the other hand, the “++” operator can be ambiguous and confusing, especially for newer programmers. Reasoning Behind the Decision to Not Include “++” Operator
The decision to not include the “++” operator in Python was a deliberate one.
Guido van Rossum, the creator of Python, has explained the reasoning behind this decision:
“I reject the ++ and — operators from C and C++. There is no good reason to use them, and they increase the risk of silly mistakes.”
This is a sentiment that many seasoned Python programmers echo.
By using the “+=” operator to increment a variable, you can avoid a lot of potential mistakes.
Examples of Python Incrementation
Now that we’ve covered the basics of incrementing a variable in Python, let’s take a look at some examples. Example 1: Incrementing an Integer Variable
In this example, we increment an integer variable named x by 1. The output will be 11.
Example 2: Appending to a String Using “+=”
In this example, we append the string “Doe” to the original string “John” using the “+=” operator. The output will be “John Doe”.
Example 3: Attempting to Use “++” Operator
In this example, we attempt to use the “++” operator to increment the variable x by 1. However, this will result in a syntax error.
If you try to run this code, you’ll get an error message that says:
“SyntaxError: invalid syntax”
This error occurs because Python does not recognize the “++” operator.
In summary, while Python does not have the “++” operator, you can still easily increment a variable using the augmented assignment operator “+=”. By using this operator instead of the “++” operator, you can write code that is clearer and less prone to errors.
Remember, in Python, it’s all about simplicity and readability. By following these principles, you can write code that is easy to understand and easy to maintain.
Evaluating Python Augmented Assignment Operator: Understanding its Difference from Regular Assignment and Optimizing Code at Runtime
In Python, there are two ways to assign values to variables: regular assignment and augmented assignment. Regular assignment simply assigns a value to a variable, whereas augmented assignment combines an assignment operation with an arithmetic or bitwise operation.
In this article, we’ll explore the difference between these two types of assignment and the importance of understanding them in optimizing code at runtime.
Difference between Regular Assignment and Augmented Assignment
Regular assignment is the most basic form of assignment in Python. Here’s an example:
This assigns the integer value 5 to the variable x.
The value of x is now 5. Augmented assignment, on the other hand, combines an assignment operation with an arithmetic or bitwise operation.
Here are some examples:
In each of these examples, we are both assigning a value to a variable and performing an arithmetic or bitwise operation at the same time. Note that the augmented assignment operator (e.g. “+=”) always comes after the variable name.
Precedence of Left Side Evaluation Before Right Side
It’s important to understand the order in which Python evaluates augmented assignments. The left-hand side of an augmented assignment is evaluated before the right-hand side.
This means that if you reference the variable being incremented in the same expression, the old value will be used on the right-hand side. Here’s an example to illustrate this:
In this example, we first set the value of x to 5. Then, we use the “+=” operator to increment x by the result of an expression.
The expression on the right-hand side is evaluated after the original value of x (i.e. 5) is used on the left-hand side. So, the expression on the right-hand side evaluates to 7, and the sum of that with the original value of x (5) is 12.
Optimizing Code at Runtime
Augmented assignment can be useful for optimizing code at runtime. For example, consider the following code:
In this code, we initialize the variable x to 0, and then we use a loop to add the values of i (0 to 9) to x. This is a common pattern in Python, and it can be optimized using augmented assignment.
Here’s the optimized code:
This code is functionally the same as the previous example, but it’s more efficient. By using augmented assignment instead of regular assignment, we avoid creating a temporary variable to hold the result of the addition operation.
Alternative to the “++” Operator in Python
As we’ve seen, augmented assignment is a simple and clear way to increment a variable in Python. The absence of the “++” operator is a byproduct of the Pythonic way of doing things, which prioritizes simplicity, readability, and expressiveness.
By using augmented assignment, we can avoid ambiguity and potential errors in our code.
Importance of Understanding Python Increment Operation
Understanding how to increment a variable in Python is an important skill for any Python programmer. By using augmented assignment instead of the “++” operator, we can write code that is clearer, less prone to errors, and more efficient.
By understanding the difference between regular assignment and augmented assignment, as well as the order in which Python evaluates augmented assignments, we can optimize our code at runtime and make our programs more efficient. In conclusion, this article has explored the difference between regular assignment and augmented assignment in Python.
Augmented assignment is a simple and clear way to increment a variable and can be more efficient than regular assignment. By understanding how Python evaluates augmented assignments, we can optimize our code at runtime.
Additionally, the absence of the “++” operator in Python is a byproduct of the Pythonic way of doing things, which prioritizes simplicity and readability. The key takeaway from this article is the importance of understanding Python increment operation to write clear, efficient, and error-free code.
Popular Posts
Mastering pandas: setting column widths in jupyter notebooks, unleashing the power of matplotlib in jupyter notebooks, mastering random boolean values in python.
- Terms & Conditions
- Privacy Policy
Python Enhancement Proposals
- Python »
- PEP Index »
PEP 577 – Augmented Assignment Expressions
Pep withdrawal, augmented assignment expressions, adding an inline assignment operator, assignment operator precedence, augmented assignment to names in block scopes, augmented assignment to names in scoped expressions, allowing complex assignment targets, augmented assignment or name binding only, postponing a decision on expression level target declarations, ignoring scoped expressions when determining augmented assignment targets, treating inline assignment as an augmented assignment variant, disallowing augmented assignments in class level scoped expressions, comparison operators vs assignment operators, simplifying retry loops, simplifying if-elif chains, capturing intermediate values from comprehensions, allowing lambda expressions to act more like re-usable code thunks, relationship with pep 572, acknowledgements.
While working on this PEP, I realised that it didn’t really address what was actually bothering me about PEP 572 ’s proposed scoping rules for previously unreferenced assignment targets, and also had some significant undesirable consequences (most notably, allowing >>= and <<= as inline augmented assignment operators that meant something entirely different from the >= and <= comparison operators).
I also realised that even without dedicated syntax of their own, PEP 572 technically allows inline augmented assignments to be written using the operator module:
The restriction to simple names as inline assignment targets means that the target expression can always be repeated without side effects, and thus avoids the ambiguity that would arise from allowing actual embedded augmented assignments (it’s still a bad idea, since it would almost certainly be hard for humans to read, this note is just about the theoretical limits of language level expressiveness).
Accordingly, I withdrew this PEP without submitting it for pronouncement. At the time I also started writing a replacement PEP that focused specifically on the handling of assignment targets which hadn’t already been declared as local variables in the current scope (for both regular block scopes, and for scoped expressions), but that draft never even reached a stage where I liked it better than the ultimately accepted proposal in PEP 572 , so it was never posted anywhere, nor assigned a PEP number.
This is a proposal to allow augmented assignments such as x += 1 to be used as expressions, not just statements.
As part of this, NAME := EXPR is proposed as an inline assignment expression that uses the new augmented assignment scoping rules, rather than implicitly defining a new local variable name the way that existing name binding statements do. The question of allowing expression level local variable declarations at function scope is deliberately separated from the question of allowing expression level name bindings, and deferred to a later PEP.
This PEP is a direct competitor to PEP 572 (although it borrows heavily from that PEP’s motivation, and even shares the proposed syntax for inline assignments). See Relationship with PEP 572 for more details on the connections between the two PEPs.
To improve the usability of the new expressions, a semantic split is proposed between the handling of augmented assignments in regular block scopes (modules, classes, and functions), and the handling of augmented assignments in scoped expressions (lambda expressions, generator expressions, and comprehensions), such that all inline assignments default to targeting the nearest containing block scope.
A new compile time TargetNameError is added as a subclass of SyntaxError to handle cases where it is deemed to be currently unclear which target is expected to be rebound by an inline assignment, or else the target scope for the inline assignment is considered invalid for another reason.
Syntax and semantics
The language grammar would be adjusted to allow augmented assignments to appear as expressions, where the result of the augmented assignment expression is the same post-calculation reference as is being bound to the given target.
For example:
For mutable targets, this means the result is always just the original object:
Augmented assignments to attributes and container subscripts will be permitted, with the result being the post-calculation reference being bound to the target, just as it is for simple name targets:
In these cases, __getitem__ and __getattribute__ will not be called after the assignment has already taken place (they will only be called as needed to evaluate the in-place operation).
Given only the addition of augmented assignment expressions, it would be possible to abuse a symbol like |= as a general purpose assignment operator by defining a Target wrapper type that worked as follows:
This is similar to the way that storing a single reference in a list was long used as a workaround for the lack of a nonlocal keyword, and can still be used today (in combination with operator.itemsetter ) to work around the lack of expression level assignments.
Rather than requiring such workarounds, this PEP instead proposes that PEP 572 ’s “NAME := EXPR” syntax be adopted as a new inline assignment expression that uses the augmented assignment scoping rules described below.
This cleanly handles cases where only the new value is of interest, and the previously bound value (if any) can just be discarded completely.
Note that for both simple names and complex assignment targets, the inline assignment operator does not read the previous reference before assigning the new one. However, when used at function scope (either directly or inside a scoped expression), it does not implicitly define a new local variable, and will instead raise TargetNameError (as described for augmented assignments below).
To preserve the existing semantics of augmented assignment statements, inline assignment operators will be defined as being of lower precedence than all other operators, include the comma pseudo-operator. This ensures that when used as a top level expression the entire right hand side of the expression is still interpreted as the value to be processed (even when that value is a tuple without parentheses).
The difference this introduces relative to PEP 572 is that where (n := first, second) sets n = first in PEP 572 , in this PEP it would set n = (first, second) , and getting the first meaning would require an extra set of parentheses ( ((n := first), second) ).
PEP 572 quite reasonably notes that this results in ambiguity when assignment expressions are used as function call arguments. This PEP resolves that concern a different way by requiring that assignment expressions be parenthesised when used as arguments to a function call (unless they’re the sole argument).
This is a more relaxed version of the restriction placed on generator expressions (which always require parentheses, except when they’re the sole argument to a function call).
No target name binding changes are proposed for augmented assignments at module or class scope (this also includes code executed using “exec” or “eval”). These will continue to implicitly declare a new local variable as the binding target as they do today, and (if necessary) will be able to resolve the name from an outer scope before binding it locally.
At function scope, augmented assignments will be changed to require that there be either a preceding name binding or variable declaration to explicitly establish the target name as being local to the function, or else an explicit global or nonlocal declaration. TargetNameError , a new SyntaxError subclass, will be raised at compile time if no such binding or declaration is present.
For example, the following code would compile and run as it does today:
The follow examples would all still compile and then raise an error at runtime as they do today:
Whereas the following would raise a compile time DeprecationWarning initially, and eventually change to report a compile time TargetNameError :
As a conservative implementation approach, the compile time function name resolution change would be introduced as a DeprecationWarning in Python 3.8, and then converted to TargetNameError in Python 3.9. This avoids potential problems in cases where an unused function would currently raise UnboundLocalError if it was ever actually called, but the code is actually unused - converting that latent runtime defect to a compile time error qualifies as a backwards incompatible change that requires a deprecation period.
When augmented assignments are used as expressions in function scope (rather than as standalone statements), there aren’t any backwards compatibility concerns, so the compile time name binding checks would be enforced immediately in Python 3.8.
Similarly, the new inline assignment expressions would always require explicit predeclaration of their target scope when used as part of a function, at least for Python 3.8. (See the design discussion section for notes on potentially revisiting that restriction in the future).
Scoped expressions is a new collective term being proposed for expressions that introduce a new nested scope of execution, either as an intrinsic part of their operation (lambda expressions, generator expressions), or else as a way of hiding name binding operations from the containing scope (container comprehensions).
Unlike regular functions, these scoped expressions can’t include explicit global or nonlocal declarations to rebind names directly in an outer scope.
Instead, their name binding semantics for augmented assignment expressions would be defined as follows:
- augmented assignment targets used in scoped expressions are expected to either be already bound in the containing block scope, or else have their scope explicitly declared in the containing block scope. If no suitable name binding or declaration can be found in that scope, then TargetNameError will be raised at compile time (rather than creating a new binding within the scoped expression).
- if the containing block scope is a function scope, and the target name is explicitly declared as global or nonlocal , then it will be use the same scope declaration in the body of the scoped expression
- if the containing block scope is a function scope, and the target name is a local variable in that function, then it will be implicitly declared as nonlocal in the body of the scoped expression
- if the containing block scope is a class scope, than TargetNameError will always be raised, with a dedicated message indicating that combining class scopes with augmented assignments in scoped expressions is not currently permitted.
- if a name is declared as a formal parameter (lambda expressions), or as an iteration variable (generator expressions, comprehensions), then that name is considered local to that scoped expression, and attempting to use it as the target of an augmented assignment operation in that scope, or any nested scoped expression, will raise TargetNameError (this is a restriction that could potentially be lifted later, but is being proposed for now to simplify the initial set of compile time and runtime semantics that needs to be covered in the language reference and handled by the compiler and interpreter)
For example, the following code would work as shown:
While the following examples would all raise TargetNameError :
As augmented assignments currently can’t appear inside scoped expressions, the above compile time name resolution exceptions would be included as part of the initial implementation rather than needing to be phased in as a potentially backwards incompatible change.
Design discussion
The initial drafts of this PEP kept PEP 572 ’s restriction to single name targets when augmented assignments were used as expressions, allowing attribute and subscript targets solely for the statement form.
However, enforcing that required varying the permitted targets based on whether or not the augmented assignment was a top level expression or not, as well as explaining why n += 1 , (n += 1) , and self.n += 1 were all legal, but (self.n += 1) was prohibited, so the proposal was simplified to allow all existing augmented assignment targets for the expression form as well.
Since this PEP defines TARGET := EXPR as a variant on augmented assignment, that also gained support for assignment and subscript targets.
PEP 572 makes a reasonable case that the potential use cases for inline augmented assignment are notably weaker than those for inline assignment in general, so it’s acceptable to require that they be spelled as x := x + 1 , bypassing any in-place augmented assignment methods.
While this is at least arguably true for the builtin types (where potential counterexamples would probably need to focus on set manipulation use cases that the PEP author doesn’t personally have), it would also rule out more memory intensive use cases like manipulation of NumPy arrays, where the data copying involved in out-of-place operations can make them impractical as alternatives to their in-place counterparts.
That said, this PEP mainly exists because the PEP author found the inline assignment proposal much easier to grasp as “It’s like += , only skipping the addition step”, and also liked the way that that framing provides an actual semantic difference between NAME = EXPR and NAME := EXPR at function scope.
That difference in target scoping behaviour means that the NAME := EXPR syntax would be expected to have two primary use cases:
- as a way of allowing assignments to be embedded as an expression in an if or while statement, or as part of a scoped expression
- as a way of requesting a compile time check that the target name be previously declared or bound in the current function scope
At module or class scope, NAME = EXPR and NAME := EXPR would be semantically equivalent due to the compiler’s lack of visibility into the set of names that will be resolvable at runtime, but code linters and static type checkers would be encouraged to enforce the same “declaration or assignment required before use” behaviour for NAME := EXPR as the compiler would enforce at function scope.
At least for Python 3.8, usage of inline assignments (whether augmented or not) at function scope would always require a preceding name binding or scope declaration to avoid getting TargetNameError , even when used outside a scoped expression.
The intent behind this requirement is to clearly separate the following two language design questions:
- Can an expression rebind a name in the current scope?
- Can an expression declare a new name in the current scope?
For module global scopes, the answer to both of those questions is unequivocally “Yes”, because it’s a language level guarantee that mutating the globals() dict will immediately impact the runtime module scope, and global NAME declarations inside a function can have the same effect (as can importing the currently executing module and modifying its attributes).
For class scopes, the answer to both questions is also “Yes” in practice, although less unequivocally so, since the semantics of locals() are currently formally unspecified. However, if the current behaviour of locals() at class scope is taken as normative (as PEP 558 proposes), then this is essentially the same scenario as manipulating the module globals, just using locals() instead.
For function scopes, however, the current answers to these two questions are respectively “Yes” and “No”. Expression level rebinding of function locals is already possible thanks to lexically nested scopes and explicit nonlocal NAME expressions. While this PEP will likely make expression level rebinding more common than it is today, it isn’t a fundamentally new concept for the language.
By contrast, declaring a new function local variable is currently a statement level action, involving one of:
- an assignment statement ( NAME = EXPR , OTHER_TARGET = NAME = EXPR , etc)
- a variable declaration ( NAME : EXPR )
- a nested function definition
- a nested class definition
- a with statement
- an except clause (with limited scope of access)
The historical trend for the language has actually been to remove support for expression level declarations of function local names, first with the introduction of “fast locals” semantics (which made the introduction of names via locals() unsupported for function scopes), and again with the hiding of comprehension iteration variables in Python 3.0.
Now, it may be that in Python 3.9, we decide to revisit this question based on our experience with expression level name binding in Python 3.8, and decide that we really do want expression level function local variable declarations as well, and that we want NAME := EXPR to be the way we spell that (rather than, for example, spelling inline declarations more explicitly as NAME := EXPR given NAME , which would permit them to carry type annotations, and also permit them to declare new local variables in scoped expressions, rather than having to pollute the namespace in their containing scope).
But the proposal in this PEP is that we explicitly give ourselves a full release to decide how much we want that feature, and exactly where we find its absence irritating. Python has survived happily without expression level name bindings or declarations for decades, so we can afford to give ourselves a couple of years to decide if we really want both of those, or if expression level bindings are sufficient.
When discussing possible binding semantics for PEP 572 ’s assignment expressions, Tim Peters made a plausible case [1] , [2] , [3] for assignment expressions targeting the containing block scope, essentially ignoring any intervening scoped expressions.
This approach allows use cases like cumulative sums, or extracting the final value from a generator expression to be written in a relatively straightforward way:
Guido also expressed his approval for this general approach [4] .
The proposal in this PEP differs from Tim’s original proposal in three main areas:
- it applies the proposal to all augmented assignment operators, not just a single new name binding operator
- as far as is practical, it extends the augmented assignment requirement that the name already be defined to the new name binding operator (raising TargetNameError rather than implicitly declaring new local variables at function scope)
- it includes lambda expressions in the set of scopes that get ignored for target name binding purposes, making this transparency to assignments common to all of the scoped expressions rather than being specific to comprehensions and generator expressions
With scoped expressions being ignored when calculating binding targets, it’s once again difficult to detect the scoping difference between the outermost iterable expressions in generator expressions and comprehensions (you have to mess about with either class scopes or attempting to rebind iteration Variables to detect it), so there’s also no need to tinker with that.
One of the challenges with PEP 572 is the fact that NAME = EXPR and NAME := EXPR are entirely semantically equivalent at every scope. This makes the two forms hard to teach, since there’s no inherent nudge towards choosing one over the other at the statement level, so you end up having to resort to “ NAME = EXPR is preferred because it’s been around longer” (and PEP 572 proposes to enforce that historical idiosyncrasy at the compiler level).
That semantic equivalence is difficult to avoid at module and class scope while still having if NAME := EXPR: and while NAME := EXPR: work sensibly, but at function scope the compiler’s comprehensive view of all local names makes it possible to require that the name be assigned or declared before use, providing a reasonable incentive to continue to default to using the NAME = EXPR form when possible, while also enabling the use of the NAME := EXPR as a kind of simple compile time assertion (i.e. explicitly indicating that the targeted name has already been bound or declared and hence should already be known to the compiler).
If Guido were to declare that support for inline declarations was a hard design requirement, then this PEP would be updated to propose that EXPR given NAME also be introduced as a way to support inline name declarations after arbitrary expressions (this would allow the inline name declarations to be deferred until the end of a complex expression rather than needing to be embedded in the middle of it, and PEP 8 would gain a recommendation encouraging that style).
While modern classes do define an implicit closure that’s visible to method implementations (in order to make __class__ available for use in zero-arg super() calls), there’s no way for user level code to explicitly add additional names to that scope.
Meanwhile, attributes defined in a class body are ignored for the purpose of defining a method’s lexical closure, which means adding them there wouldn’t work at an implementation level.
Rather than trying to resolve that inherent ambiguity, this PEP simply prohibits such usage, and requires that any affected logic be written somewhere other than directly inline in the class body (e.g. in a separate helper function).
The OP= construct as an expression currently indicates a comparison operation:
Both this PEP and PEP 572 propose adding at least one operator that’s somewhat similar in appearance, but defines an assignment instead:
This PEP then goes much further and allows all 13 augmented assignment symbols to be uses as binary operators:
Of those additional binary operators, the most questionable would be the bitshift assignment operators, since they’re each only one doubled character away from one of the inclusive ordered comparison operators.
There are currently a few different options for writing retry loops, including:
Each of the available options hides some aspect of the intended loop structure inside the loop body, whether that’s the state modification, the exit condition, or both.
The proposal in this PEP allows both the state modification and the exit condition to be included directly in the loop header:
if-elif chains that need to rebind the checked condition currently need to be written using nested if-else statements:
As with PEP 572 , this PEP allows the else/if portions of that chain to be condensed, making their consistent and mutually exclusive structure more readily apparent:
Unlike PEP 572 , this PEP requires that the assignment target be explicitly indicated as local before the first use as a := target, either by binding it to a value (as shown above), or else by including an appropriate explicit type declaration:
The proposal in this PEP makes it straightforward to capture and reuse intermediate values in comprehensions and generator expressions by exporting them to the containing block scope:
This PEP allows the classic closure usage example:
To be abbreviated as:
While the latter form is still a conceptually dense piece of code, it can be reasonably argued that the lack of boilerplate (where the “def”, “nonlocal”, and “return” keywords and two additional repetitions of the “x” variable name have been replaced with the “lambda” keyword) may make it easier to read in practice.
The case for allowing inline assignments at all is made in PEP 572 . This competing PEP was initially going to propose an alternate surface syntax ( EXPR given NAME = EXPR ), while retaining the expression semantics from PEP 572 , but that changed when discussing one of the initial motivating use cases for allowing embedded assignments at all: making it possible to easily calculate cumulative sums in comprehensions and generator expressions.
As a result of that, and unlike PEP 572 , this PEP focuses primarily on use cases for inline augmented assignment. It also has the effect of converting cases that currently inevitably raise UnboundLocalError at function call time to report a new compile time TargetNameError .
New syntax for a name rebinding expression ( NAME := TARGET ) is then added not only to handle the same use cases as are identified in PEP 572 , but also as a lower level primitive to help illustrate, implement and explain the new augmented assignment semantics, rather than being the sole change being proposed.
The author of this PEP believes that this approach makes the value of the new flexibility in name rebinding clearer, while also mitigating many of the potential concerns raised with PEP 572 around explaining when to use NAME = EXPR over NAME := EXPR (and vice-versa), without resorting to prohibiting the bare statement form of NAME := EXPR outright (such that NAME := EXPR is a compile error, but (NAME := EXPR) is permitted).
The PEP author wishes to thank Chris Angelico for his work on PEP 572 , and his efforts to create a coherent summary of the great many sprawling discussions that spawned on both python-ideas and python-dev, as well as Tim Peters for the in-depth discussion of parent local scoping that prompted the above scoping proposal for augmented assignments inside scoped expressions.
Eric Snow’s feedback on a pre-release version of this PEP helped make it significantly more readable.
This document has been placed in the public domain.
Source: https://github.com/python/peps/blob/main/peps/pep-0577.rst
Last modified: 2023-10-11 12:05:51 GMT
- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Assignment Operators in Python
The Python Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, and bitwise computations. The value the operator operates on is known as the Operand. Here, we will cover Different Assignment operators in Python .
Operators |
| ||
---|---|---|---|
| = | Assign the value of the right side of the expression to the left side operand | c = a + b |
| += | Add right side operand with left side operand and then assign the result to left operand | a += b |
| -= | Subtract right side operand from left side operand and then assign the result to left operand | a -= b |
| *= | Multiply right operand with left operand and then assign the result to the left operand | a *= b |
| /= | Divide left operand with right operand and then assign the result to the left operand | a /= b |
| %= | Divides the left operand with the right operand and then assign the remainder to the left operand | a %= b |
| //= | Divide left operand with right operand and then assign the value(floor) to left operand | a //= b |
| **= | Calculate exponent(raise power) value using operands and then assign the result to left operand | a **= b |
| &= | Performs Bitwise AND on operands and assign the result to left operand | a &= b |
| |= | Performs Bitwise OR on operands and assign the value to left operand | a |= b |
| ^= | Performs Bitwise XOR on operands and assign the value to left operand | a ^= b |
| >>= | Performs Bitwise right shift on operands and assign the result to left operand | a >>= b |
| <<= | Performs Bitwise left shift on operands and assign the result to left operand | a <<= b |
| := | Assign a value to a variable within an expression | a := exp |
Here are the Assignment Operators in Python with examples.
Assignment Operator
Assignment Operators are used to assign values to variables. This operator is used to assign the value of the right side of the expression to the left side operand.
Addition Assignment Operator
The Addition Assignment Operator is used to add the right-hand side operand with the left-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the addition assignment operator which will first perform the addition operation and then assign the result to the variable on the left-hand side.
S ubtraction Assignment Operator
The Subtraction Assignment Operator is used to subtract the right-hand side operand from the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the subtraction assignment operator which will first perform the subtraction operation and then assign the result to the variable on the left-hand side.
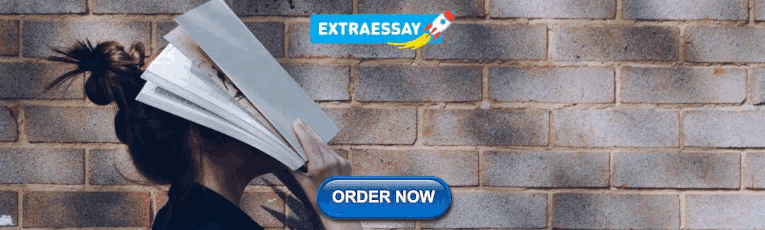
M ultiplication Assignment Operator
The Multiplication Assignment Operator is used to multiply the right-hand side operand with the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the multiplication assignment operator which will first perform the multiplication operation and then assign the result to the variable on the left-hand side.
D ivision Assignment Operator
The Division Assignment Operator is used to divide the left-hand side operand with the right-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the division assignment operator which will first perform the division operation and then assign the result to the variable on the left-hand side.
M odulus Assignment Operator
The Modulus Assignment Operator is used to take the modulus, that is, it first divides the operands and then takes the remainder and assigns it to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the modulus assignment operator which will first perform the modulus operation and then assign the result to the variable on the left-hand side.
F loor Division Assignment Operator
The Floor Division Assignment Operator is used to divide the left operand with the right operand and then assigs the result(floor value) to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the floor division assignment operator which will first perform the floor division operation and then assign the result to the variable on the left-hand side.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator is used to calculate the exponent(raise power) value using operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the exponentiation assignment operator which will first perform exponent operation and then assign the result to the variable on the left-hand side.
Bitwise AND Assignment Operator
The Bitwise AND Assignment Operator is used to perform Bitwise AND operation on both operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise AND assignment operator which will first perform Bitwise AND operation and then assign the result to the variable on the left-hand side.
Bitwise OR Assignment Operator
The Bitwise OR Assignment Operator is used to perform Bitwise OR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise OR assignment operator which will first perform bitwise OR operation and then assign the result to the variable on the left-hand side.
Bitwise XOR Assignment Operator
The Bitwise XOR Assignment Operator is used to perform Bitwise XOR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise XOR assignment operator which will first perform bitwise XOR operation and then assign the result to the variable on the left-hand side.
Bitwise Right Shift Assignment Operator
The Bitwise Right Shift Assignment Operator is used to perform Bitwise Right Shift Operation on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise right shift assignment operator which will first perform bitwise right shift operation and then assign the result to the variable on the left-hand side.
Bitwise Left Shift Assignment Operator
The Bitwise Left Shift Assignment Operator is used to perform Bitwise Left Shift Opertator on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise left shift assignment operator which will first perform bitwise left shift operation and then assign the result to the variable on the left-hand side.
Walrus Operator
The Walrus Operator in Python is a new assignment operator which is introduced in Python version 3.8 and higher. This operator is used to assign a value to a variable within an expression.
Example: In this code, we have a Python list of integers. We have used Python Walrus assignment operator within the Python while loop . The operator will solve the expression on the right-hand side and assign the value to the left-hand side operand ‘x’ and then execute the remaining code.
Assignment Operators in Python – FAQs
What are assignment operators in python.
Assignment operators in Python are used to assign values to variables. These operators can also perform additional operations during the assignment. The basic assignment operator is = , which simply assigns the value of the right-hand operand to the left-hand operand. Other common assignment operators include += , -= , *= , /= , %= , and more, which perform an operation on the variable and then assign the result back to the variable.
What is the := Operator in Python?
The := operator, introduced in Python 3.8, is known as the “walrus operator”. It is an assignment expression, which means that it assigns values to variables as part of a larger expression. Its main benefit is that it allows you to assign values to variables within expressions, including within conditions of loops and if statements, thereby reducing the need for additional lines of code. Here’s an example: # Example of using the walrus operator in a while loop while (n := int(input("Enter a number (0 to stop): "))) != 0: print(f"You entered: {n}") This loop continues to prompt the user for input and immediately uses that input in both the condition check and the loop body.
What is the Assignment Operator in Structure?
In programming languages that use structures (like C or C++), the assignment operator = is used to copy values from one structure variable to another. Each member of the structure is copied from the source structure to the destination structure. Python, however, does not have a built-in concept of ‘structures’ as in C or C++; instead, similar functionality is achieved through classes or dictionaries.
What is the Assignment Operator in Python Dictionary?
In Python dictionaries, the assignment operator = is used to assign a new key-value pair to the dictionary or update the value of an existing key. Here’s how you might use it: my_dict = {} # Create an empty dictionary my_dict['key1'] = 'value1' # Assign a new key-value pair my_dict['key1'] = 'updated value' # Update the value of an existing key print(my_dict) # Output: {'key1': 'updated value'}
What is += and -= in Python?
The += and -= operators in Python are compound assignment operators. += adds the right-hand operand to the left-hand operand and assigns the result to the left-hand operand. Conversely, -= subtracts the right-hand operand from the left-hand operand and assigns the result to the left-hand operand. Here are examples of both: # Example of using += a = 5 a += 3 # Equivalent to a = a + 3 print(a) # Output: 8 # Example of using -= b = 10 b -= 4 # Equivalent to b = b - 4 print(b) # Output: 6 These operators make code more concise and are commonly used in loops and iterative data processing.
Please Login to comment...
Similar reads.
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Join us and get access to thousands of tutorials and a community of expert Pythonistas.
This lesson is for members only. Join us and get access to thousands of tutorials and a community of expert Pythonistas.
Augmented Assignment (Sets)
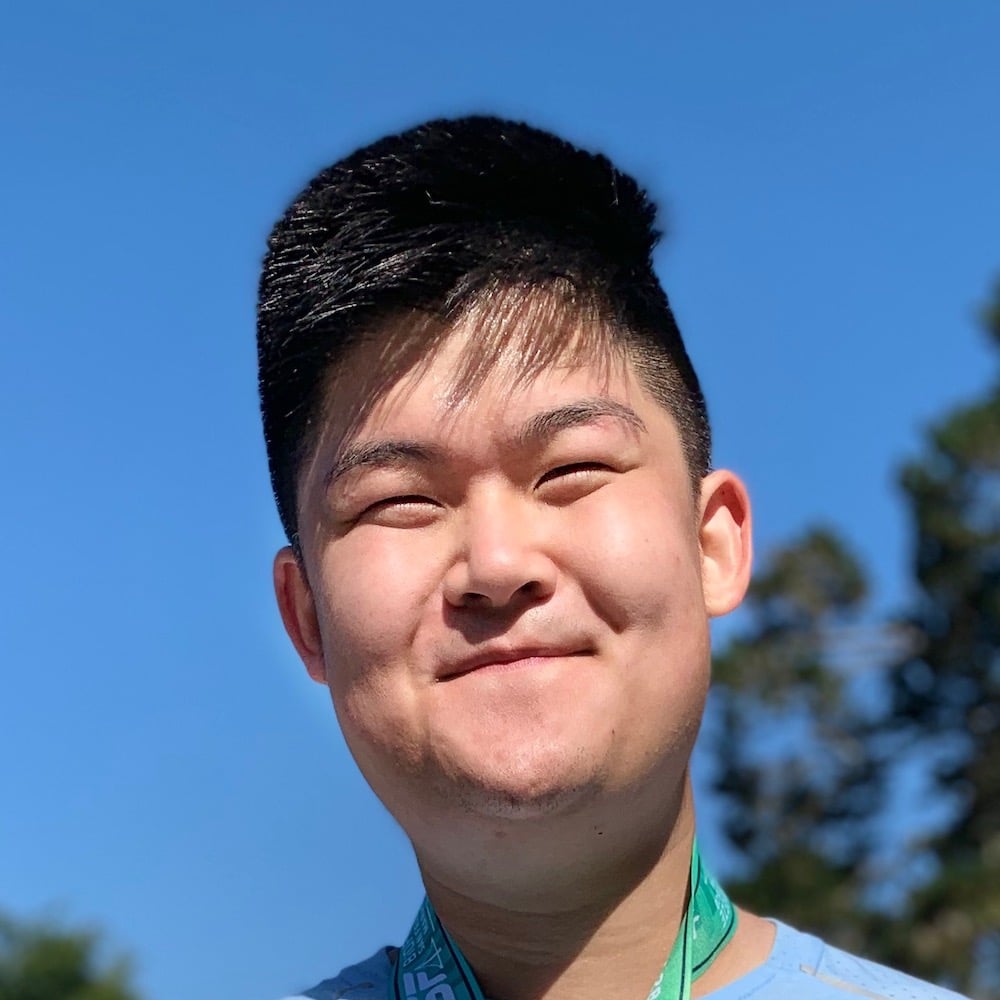
00:00 Let’s take a deep dive into how augmented assignment actually works. You saw that many of the modifying set methods have a corresponding augmented assignment.
00:09 Like we saw, intersection update ( &= ), and difference update ( -= ), and symmetric difference update ( ^= ). These are not the same as their expanded out counterparts.
00:19 For example, x &= {1} is not the same as x = x & {1} .
00:29 Here’s an example where you will see that. Here we have x = {1} , y = x . That will make y point to the same set that x is pointing to.
00:41 Now we will use update ( |= ) to update x with {2} .
00:47 Here, x becomes {1, 2} and y also becomes {1, 2} because they are pointing to the same object. Versus x = {1} , y = x , x = x | {2} . x will become {1, 2} , but y will become nothing.
01:08 It will actually be the original {1} . This is because x = x | {2} created a new set of {1, 2} and then bound that back to x . y still pointed to the original {1} .
01:25 The first example mutates x , the second example reassigns x .
Become a Member to join the conversation.
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
//= | x //= 3 | x = x // 3 | |
**= | x **= 3 | x = x ** 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |
Related Pages

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
overloading augmented arithmetic assignments in python
I'm new to Python so apologies in advance if this is a stupid question.
For an assignment I need to overload augmented arithmetic assignments(+=, -=, /=, *=, **=, %=) for a class myInt. I checked the Python documentation and this is what I came up with:
self.a and other.a refer to the int stored in each class instance. I tried testing this out as follows, but each time I get 'None' instead of the expected value 5:
Can anyone tell me why this is happening? Thanks in advance.
- operator-overloading
- 2 I believe there are no stupid questions.. – Srinivas Reddy Thatiparthy Commented Feb 15, 2010 at 17:22
3 Answers 3
You need to add return self to your method. Explanation:
The semantics of a += b , when type(a) has a special method __iadd__ , are defined to be:
so if __iadd__ returns something different than self , that's what will be bound to name a after the operation. By missing a return statement, the method you posted is equivalent to one with return None .
- 1 Alex,I always love your answers! – Srinivas Reddy Thatiparthy Commented Feb 15, 2010 at 17:24
Augmented operators in Python have to return the final value to be assigned to the name they are called on, usually (and in your case) self . Like all Python methods, missing a return statement implies returning None .
- Never ever ever raise Exception , which is impossible to catch sanely. The code to do so would have to say except Exception , which will catch all exceptions. In this case you want ValueError or TypeError .
- Don't typecheck with type(foo) == SomeType . In this (and virtually all) cases, isinstance works better or at least the same.
- Whenever you make your own type, like myInt , you should name it with capital letters so people can recognize it as a class name.

Yes, you need "return self", it will look like this:
- 2 Also, you should make your Exception a TypeError . – jcdyer Commented Feb 15, 2010 at 17:21
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged python operators operator-overloading or ask your own question .
- The Overflow Blog
- Ryan Dahl explains why Deno had to evolve with version 2.0
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
- What does a new user need in a homepage experience on Stack Overflow?
Hot Network Questions
- What is the origin of this quote on telling a big lie?
- What is the cardinality of all possible wave function?
- How soon to fire rude and chaotic PhD student?
- How can flyby missions work?
- How to make outhouses less icky?
- What did Scott Lang mean by "living as a tenant of the state"?
- How to add content security headers in Next.js which are configurable from cms side? where to add?
- Why are swimming goggles typically made from a different material than diving masks?
- Can I enter the US with my still valid ESTA and B2 visa application after moving to Mexico?
- Is math a bad discipline for teaching jobs or is it just me?
- Does "any computer(s) you have" refer to one or all the computers?
- I can't select a certain record with like %value%
- Is the Garmin Edge 530 still a good choice for a beginner in 2024?
- What other goals could a space project with the primary goal of experience building with heavy lift rockets preform?
- Which BASIC dialect first featured a single-character comment introducer?
- Is it possible for a company to dilute my shares to the point they are insignificant
- Passport - small damage on passport
- How is Nationality Principle applied in practice?
- block diagonal matrix
- How does DS18B20 temperature sensor get the temperature?
- The beloved shepherd isn't Solomon?
- Find all pairs of positive compatible numbers less than 100 by proof
- What prevents applications from misusing private keys?
- Inconsistent width of relational operators in newtxmath
Information
- Author Services
Initiatives
You are accessing a machine-readable page. In order to be human-readable, please install an RSS reader.
All articles published by MDPI are made immediately available worldwide under an open access license. No special permission is required to reuse all or part of the article published by MDPI, including figures and tables. For articles published under an open access Creative Common CC BY license, any part of the article may be reused without permission provided that the original article is clearly cited. For more information, please refer to https://www.mdpi.com/openaccess .
Feature papers represent the most advanced research with significant potential for high impact in the field. A Feature Paper should be a substantial original Article that involves several techniques or approaches, provides an outlook for future research directions and describes possible research applications.
Feature papers are submitted upon individual invitation or recommendation by the scientific editors and must receive positive feedback from the reviewers.
Editor’s Choice articles are based on recommendations by the scientific editors of MDPI journals from around the world. Editors select a small number of articles recently published in the journal that they believe will be particularly interesting to readers, or important in the respective research area. The aim is to provide a snapshot of some of the most exciting work published in the various research areas of the journal.
Original Submission Date Received: .
- Active Journals
- Find a Journal
- Proceedings Series
- For Authors
- For Reviewers
- For Editors
- For Librarians
- For Publishers
- For Societies
- For Conference Organizers
- Open Access Policy
- Institutional Open Access Program
- Special Issues Guidelines
- Editorial Process
- Research and Publication Ethics
- Article Processing Charges
- Testimonials
- Preprints.org
- SciProfiles
- Encyclopedia
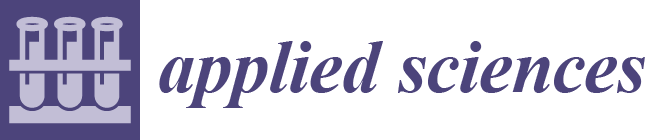
Article Menu
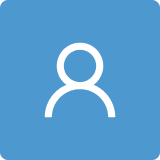
- Subscribe SciFeed
- Recommended Articles
- Google Scholar
- on Google Scholar
- Table of Contents
Find support for a specific problem in the support section of our website.
Please let us know what you think of our products and services.
Visit our dedicated information section to learn more about MDPI.
JSmol Viewer
Optical medieval music recognition—a complete pipeline for historic chants.
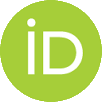
1. Introduction
- Development of a comprehensive automatic pipeline tailored for digitizing handwritten historical monophonic music depicted in squared notation. The pipeline processes input from a book and produces digitized chants (in formats such as MusicXML [ 2 ], MEI [ 3 ], Monodi [ 4 ]) corresponding to the content of the book. Additionally, position-related data are extracted, including the positions of the staff lines, layout, symbols, and syllables/lyrics on the page to support manual post-correction and training.
- Extended layout analysis capabilities, facilitating the detection and extraction of chants within the book, such as individual chants, which may extend across multiple pages.
- Development of an automatic text recognition correction and segmentation mechanism, which involves aligning the automatically extracted lyric of a chant with a pre-existing database of chants to improve the OCR, segmenting the identified lyrics into text lines according to the layout of the page. The transcribed text is finally aligned with the original OCR to obtain positional information (position of the syllables/text on the original document).
- Development of an algorithm to automatically divide text into syllables utilizing grammar rules and a syllable dictionary including a syllable assignment algorithm that subsequently aligns these syllables with the corresponding musical notes automatically.
- Evaluation of the output from the complete automated transcription process, including additional evaluations of individual steps involved in the pipeline’s execution.
- Clef: A clef is mostly at the beginning of a musical region. However, there can also be several clefs in other places in the music regions. Possible subclasses are F-clef and C-clef.
- Note components: These are the symbols that occur most often in a line. The position of note components within the staves in combination with accidentals and preceding clefs determine the note pitch. Note components may be connected to other note components with the following subclasses: start (start of a note sequence), graphically connected (part of a note sequence with graphic connection to the previous note), gapped (part of a note sequence without graphic connection to the previous note).
- Accidental: By far the rarest symbols. The subclasses here are sharp, flat, and natural accidentals.
3. Related Work
Pipeline Step | Notation Type | Method | |||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
[ ] | x | - | - | x | - | - | ✓ | 6–24 | SER | x | - | - | x | - | - | modern | Seq2Seq |
[ ] | x | - | - | x | - | - | ✓ | 94–77 | x | - | - | x | - | - | square | CNN | |
[ ] | x | - | - | x | - | - | ✓ | 17–27 | SER | x | - | - | x | - | - | modern | Seq2Seq |
[ ] | x | - | - | x | - | - | ✓ | 80 | x | - | - | x | - | - | modern | Seq2Seq | |
[ ] | x | - | - | (✓) | n.a. | n.a. | ✓ | 4–12 | SER | x | - | - | x | - | - | mensural | Seq2Seq |
[ ] | x | - | - | - | - | - | ✓ | 7 | SER | x | - | - | x | - | - | mensural | Seq2Seq |
[ ] | (✓) | n.a. | n.a. | (✓) | n.a. | n.a. | (✓) | n.a. | n.a. | x | - | - | x | - | - | square | - |
[ ] | ✓ | 99.7 | (✓) | n.a. | n.a. | ✓ | 89.8 | hSAR | x | - | - | x | - | - | square | FCN | |
[ ] | x | - | - | x | - | - | x | - | - | ✓ | 6.7–50 | CER | ✓ | 90.7–99.7 | F1 | square | LSTM |
[ ] | x | - | - | ✓ | n.a. | n.a. | x | - | - | x | - | - | x | - | - | square | LSTM |
[ ] | x | - | - | x | - | - | ✓ | 94–97 | hSAR | x | - | - | x | - | - | square | FCN |
[ ] | ✓ | 97–99 | x | - | - | x | - | - | x | - | - | x | - | - | modern | CNN | |
[ ] | x | - | - | ✓ | 71.6 | x | - | - | x | - | - | x | - | - | mensural | object det. | |
[ ] | x | - | - | ✓ | 92.5 | x | - | - | x | - | - | x | - | - | mensural | FCN | |
[ ] | x | - | - | ✓ | 89.5 | mIoU | x | - | - | x | - | - | x | - | - | historical | MASK-RCNN |
[ ] | x | - | - | x | - | - | ✓ | 66 | mAP | x | - | - | x | - | - | mensural | RCNN |
[ ] | x | - | - | x | - | - | ✓ | 61–88 | mAP | x | - | - | x | - | - | modern | object det. |
[ ] | x | - | - | x | - | - | x | - | - | - | - | - | ✓ | 16.4–71.3 | CER | (square) | LSTM |
[ ] | x | - | - | x | - | - | x | - | - | - | - | - | ✓ | 78.6–92.9 | IOU | square | LSTM |
[ ] | ✓ | - | - | ✓ | - | - | ✓ | - | - | - | - | - | ✓ | - | - | square | LSTM |
Audiveris | ✓ | n.a. | - | ✓ | n.a. | - | ✓ | n.a. | - | ✓ | n.a. | - | ✓ | n.a. | - | modern | - |
Ours | ✓ | 99.94 | F1 | ✓ | 99.99 | F1 | ✓ | 96.7 | hsar | ✓ | 4.2 | CER | ✓ | 97.3 | F1 | square | FCN, LSTM |
5. Workflow
5.1. preprocessing.
- Deskewing: Removal of rotation errors in the image
- Grayscale conversion: Conversion of a multichannel images (e.g., color images) to a grayscale image
- Binarization: Conversion of an grayscale image to an image in which every pixel is 0 or 1 (white or black)
5.2. Staff Line Detection
5.3. layout analysis, 5.4. symbol detection and recognition, 5.5. lyric transcription.
- Summarization into a single text block.
- Removal of upper cases.
- Removal of white spaces.
5.6. Syllable Assignment
5.7. reconstruction and generation of the target encoding, 6. architectures, 6.1. staff line and symbol segmentation.
- The encoder (contracting path), which progressively reduces the spatial dimensions of the input image and as such encodes the input image into feature representation at multiple hierarchical levels.
- The decoder (expansive path), which complements the encoder by projecting the context information features learned during the encoding stage onto the pixel space. This process involves upsampling the encoded features and merging them with corresponding features from the contracting path. The decoder’s objective is to reconstruct the original image with precise segmentation, utilizing the combined spatial and contextual information.
6.2. Text and Syllable Recognition
7. experiments, 7.1. experimental setting, quantitative evaluation results, 7.2. qualitative evaluation, 8. discussion, 8.1. text recognition, 8.2. syllable detection, 9. conclusions, author contributions, institutional review board statement, informed consent statement, data availability statement, acknowledgments, conflicts of interest, abbreviations.
TP | True Positive |
FP | False Positive |
FN | False Negative |
GT | Ground Truth |
CC | Connected Component |
HTR | Handwritten Text Recognition |
OMR | Optical Music Recogniton |
CNN | Convolutional Neural Network |
LSTM | Long Short-Term Memory |
RNN | Recurrent Neural Network |
FCN | Fully Convolutional Network |
dSAR | diplomatic Symbol Accuracy Rate |
dSER | diplomatic Symbol Error Rate |
hSAR | harmonic Symbol Accuracy Rate |
hSER | harmonic Symbol Accuracy Rate |
NC | Note Component |
SGD | Stochastic gradient descent |
PiS | Position in staff |
GCN | Graphical connection between notes |
CER | Character Error Rate |
ACC | Accuracy |
Accid | Accidental |
Syl | Syllable |
RLE | Run Length Encoding |
- Parrish, C. The Notation of Medieval Music ; Pendragon Press: Hillsdale, NY, USA, 1978; Volume 1. [ Google Scholar ]
- Good, M.D. MusicXML: An Internet-Friendly Format for Sheet Music. Proceedings of XML 2001 (Boston, December 9–14, 2001). Available online: http://michaelgood.info/publications/music/musicxml-an-internet-friendly-format-for-sheet-music/ (accessed on 10 August 2024).
- Hankinson, A.; Roland, P.; Fujinaga, I. The Music Encoding Initiative as a Document-Encoding Framework. In Proceedings of the 12th International Society for Music Information Retrieval Conference (ISMIR 2011), Miami, FL, USA, 24–28 October 2011; pp. 293–298. [ Google Scholar ]
- Eipert, T.; Herrman, F.; Wick, C.; Puppe, F.; Haug, A. Editor Support for Digital Editions of Medieval Monophonic Music. In Proceedings of the 2nd International Workshop on Reading Music Systems, Delft, The Netherlands, 2 November 2019; pp. 4–7. [ Google Scholar ]
- Calvo-Zaragoza, J.; Hajič, J., Jr.; Pacha, A. Understanding Optical Music Recognition. ACM Comput. Surv. 2021 , 53 , 1–35. [ Google Scholar ] [ CrossRef ]
- Ríos Vila, A.; Rizo, D.; Iñesta, J.; Calvo-Zaragoza, J. End-to-end Optical Music Recognition for Pianoform Sheet Music. Int. J. Doc. Anal. Recognit. 2023 , 26 , 347–362. [ Google Scholar ] [ CrossRef ]
- Ríos Vila, A.; Iñesta, J.; Calvo-Zaragoza, J. End-To-End Full-Page Optical Music Recognition for Mensural Notation. In Proceedings of the International Society for Music Information Retrieval Conference (ISMIR 2022), Bengaluru, India, 4–8 December 2022. [ Google Scholar ] [ CrossRef ]
- Fujinaga, I.; Vigliensoni, G. Optical Music Recognition Workflow for Medieval Music Manuscripts. In Proceedings of the 5th International Workshop on Reading Music Systems, Milan, Italy, 4 November 2023; pp. 4–6. [ Google Scholar ]
- Fujinaga, I.; Vigliensoni, G. The Art of Teaching Computers: The SIMSSA Optical Music Recognition Workflow System. In Proceedings of the 2019 27th European Signal Processing Conference (EUSIPCO), A Coruna, Spain, 2–6 September 2019; pp. 1–5. [ Google Scholar ] [ CrossRef ]
- Wick, C.; Reul, C.; Puppe, F. Calamari—A High-Performance Tensorflow-based Deep Learning Package for Optical Character Recognition. Digit. Humanit. Q. 2020 , 14 . Available online: https://www.digitalhumanities.org/dhq/vol/14/2/000451/000451.html (accessed on 10 August 2024).
- de Reuse, T.; Fujinaga, I. Robust Transcript Alignment on Medieval Chant Manuscripts. In Proceedings of the 2nd International Workshop on Reading Music Systems, Delft, The Netherlands, 2 November 2019; pp. 21–26. [ Google Scholar ]
- Calvo-Zaragoza, J.; Pertusa, A.; Oncina, J. Staff-line Detection and Removal using a Convolutional Neural Network. Mach. Vis. Appl. 2017 , 28 , 1–10. [ Google Scholar ] [ CrossRef ]
- Wick, C.; Hartelt, A.; Puppe, F. Staff, Symbol and Melody Detection of Medieval Manuscripts Written in Square Notation Using Deep Fully Convolutional Networks. Appl. Sci. 2019 , 9 , 2646. [ Google Scholar ] [ CrossRef ]
- Ronneberger, O.; Fischer, P.; Brox, T. U-Net: Convolutional Networks for Biomedical Image Segmentation. In Proceedings of the Medical Image Computing and Computer-Assisted Intervention—MICCAI 2015, Munich, Germany, 5–9 October 2018; pp. 234–241. [ Google Scholar ]
- Quirós, L.; Vidal, E. Evaluation of a Region Proposal Architecture for Multi-task Document Layout Analysis. arXiv 2021 , arXiv:2106.11797. [ Google Scholar ]
- He, K.; Gkioxari, G.; Dollár, P.; Girshick, R. Mask R-CNN. In Proceedings of the 2017 IEEE International Conference on Computer Vision (ICCV), Venice, Italy, 22–29 October 2017; pp. 2980–2988. [ Google Scholar ] [ CrossRef ]
- Quirós, L.; Toselli, A.H.; Vidal, E. Multi-task Layout Analysis of Handwritten Musical Scores. In Proceedings of the Pattern Recognition and Image Analysi, Madrid, Spain, 1–4 July 2019; pp. 123–134. [ Google Scholar ]
- Castellanos, F.J.; Garrido-Munoz, C.; Ríos-Vila, A.; Calvo-Zaragoza, J. Region-based Layout Analysis of Music Score Images. Expert Syst. Appl. 2022 , 209 , 118211. [ Google Scholar ] [ CrossRef ]
- Everingham, M.; Van Gool, L.; Williams, C.; Winn, J.; Zisserman, A. The Pascal Visual Object Classes (VOC) challenge. Int. J. Comput. Vis. 2010 , 88 , 303–338. [ Google Scholar ] [ CrossRef ]
- Calvo-Zaragoza, J.; Castellanos, F.J.; Vigliensoni, G.; Fujinaga, I. Deep Neural Networks for Document Processing of Music Score Images. Appl. Sci. 2018 , 8 , 654. [ Google Scholar ] [ CrossRef ]
- Alfaro-Contreras, M.; Iñesta, J.M.; Calvo-Zaragoza, J. Optical Music Recognition for Homophonic Scores with Neural Networks and Synthetic Music Generation. Int. J. Multimed. Inf. Retr. 2023 , 12 , 12. [ Google Scholar ]
- Castellanos, F.; Calvo-Zaragoza, J.; Iñesta, J. A Neural Approach for Full-Page Optical Music Recognition of Mensural Documents. In Proceedings of the 21st International Society for Music Information Retrieval Conference, Virtual Conference, 11–16 October 2020. [ Google Scholar ]
- Calvo-Zaragoza, J.; Toselli, A.H.; Vidal, E. Handwritten Music Recognition for Mensural Notation with Convolutional Recurrent Neural Networks. Pattern Recognit. Lett. 2019 , 128 , 115–121. [ Google Scholar ] [ CrossRef ]
- van Der Wel, E.; Ullrich, K. Optical Music Recognition with Convolutional Sequence-To-Sequence Models. In Proceedings of the 18th ISMIR Conference, Suzhou, China, 23–27 October 2017. [ Google Scholar ]
- Pacha, A.; Choi, K.Y.; Coüasnon, B.; Ricquebourg, Y.; Zanibbi, R.; Eidenberger, H. Handwritten Music Object Detection: Open Issues and Baseline Results. In Proceedings of the 2018 13th IAPR International Workshop on Document Analysis Systems (DAS), Vienna, Austria, 24–27 April 2018; pp. 163–168. [ Google Scholar ] [ CrossRef ]
- Pacha, A.; Calvo-Zaragoza, J. Optical Music Recognition in Mensural Notation with Region-Based Convolutional Neural Networks. In Proceedings of the 19th ISMIR Conference, Paris, France, 23–27 September 2018. [ Google Scholar ] [ CrossRef ]
- Ren, S.; He, K.; Girshick, R.; Sun, J. Faster R-CNN: Towards Real-time Object Detection with Region Proposal. IEEE Trans. Pattern Anal. Mach. Intell. 2017 , 39 , 1137–1149. [ Google Scholar ]
- Redmon, J.; Divvala, S.; Girshick, R.; Farhadi, A. You Only Look Once: Unified, Real-Time Object Detection. In Proceedings of the IEEE Conference on Computer Vision and Pattern Recognition, Las Vegas, NV, USA, 27–30 June 2016; pp. 779–788. [ Google Scholar ]
- Wick, C.; Hartelt, A.; Puppe, F. Lyrics Recognition and Syllable Assignment of Medieval Music Manuscripts. In Proceedings of the 2020 17th International Conference on Frontiers in Handwriting Recognition (ICFHR), Dortmund, Germany, 8–10 September 2020; pp. 187–192. [ Google Scholar ] [ CrossRef ]
- Hankinson, A.; Porter, A.; Burgoyne, J.; Thompson, J.; Vigliensoni, G.; Liu, W.; Chiu, R.; Fujinaga, I. Digital Document Image Retrieval using Optical Music Recognition. In Proceedings of the 13th International Society for Music Information Retrieval Conference (ISMIR 2012), Porto, Portugal, 8–12 October 2012; pp. 577–582. [ Google Scholar ]
- Breuel, T. Recent Progress on the OCRopus OCR System. In Proceedings of the International Workshop on Multilingual OCR, MOCR ’09, Barcelona, Spain, 25 July 2009. [ Google Scholar ] [ CrossRef ]
- Martinez-Sevilla, J.C.; Rios-Vila, A.; Castellanos, F.J.; Calvo-Zaragoza, J. Towards Music Notation and Lyrics Alignment: Gregorian Chants as Case Study. In Proceedings of the 5th International Workshop on Reading Music Systems, Milan, Italy, 4 November 2023; 4 November 2023; pp. 15–19. [ Google Scholar ]
- Burgoyne, J.A.; Ouyang, Y.; Himmelman, T.; Devaney, J.; Pugin, L.; Fujinaga, I. Lyric Extraction and Recognition on Digital Images of Early Music Sources. In Proceedings of the 10th International Society for Music Information Retrieval Conference, Kobe, Japan, 26–30 October 2009; Volume 10, pp. 723–727. [ Google Scholar ]
- Hartelt, A.; Puppe, F. Optical Medieval Music Recognition using Background Knowledge. Algorithms 2022 , 15 , 221. [ Google Scholar ] [ CrossRef ]
- Wick, C.; Hartelt, A.; Puppe, F. OMMR4all—Ein Semiautomatischer Online-Editor für Mittelalterliche Musiknotationen. In Proceedings of the DHd 2020 Spielräume: Digital Humanities zwischen Modellierung und Interpretation. 7. Tagung des Verbands “Digital Humanities im deutschsprachigen Raum” (DHd 2020), Paderborn, Germany, 2–6 March 2020. [ Google Scholar ] [ CrossRef ]
- Breuel, T.M. The OCRopus Open Source OCR System. In Proceedings of the Document Recognition and Retrieval XV, San Jose, CA, USA, 29–31 January 2008; Volume 6815, p. 68150F. [ Google Scholar ] [ CrossRef ]
- Sauvola, J.; Seppänen, T.; Haapakoski, S.; Pietikäinen, M. Adaptive Document Binarization. In Proceedings of the Fourth International Conference on Document Analysis and Recognition, Ulm, Germany, 18–20 August 1997; Volume 33, pp. 147–152. [ Google Scholar ] [ CrossRef ]
- Cardoso, J.; Rebelo, A. Robust Staffline Thickness and Distance Estimation in Binary and Gray-Level Music Scores. In Proceedings of the 2010 20th International Conference on Pattern Recognition, Istanbul, Turkey, 23–26 August 2010; pp. 1856–1859. [ Google Scholar ] [ CrossRef ]
- Fujinaga, I. Staff Detection and Removal. In Visual Perception of Music Notation: On-Line and Off-Line Recognition ; IGI Global: Hershey, PA, USA, 2004; pp. 1–39. [ Google Scholar ] [ CrossRef ]
- Long, J.; Shelhamer, E.; Darrell, T. Fully Convolutional Networks for Semantic Segmentation. In Proceedings of the 2015 IEEE Conference on Computer Vision and Pattern Recognition (CVPR), Boston, MA, USA, 7–12 June 2015; pp. 3431–3440. [ Google Scholar ] [ CrossRef ]
Click here to enlarge figure
Dataset | Pages | Staffs/Page | Symbols/Page | Clefs | Accids | Annotated | |||
---|---|---|---|---|---|---|---|---|---|
Nevers P1 | 14 | 9 | 279 | 152 | 24 | ✓ | x | ✓ | x |
Nevers P2 | 27 | 12 | 389 | 345 | 37 | ✓ | x | ✓ | x |
Nevers P3 | 8 | 9 | 209 | 83 | 1 | ✓ | x | ✓ | x |
Latin 14819 | 182 | 9 | 191 | 2108 | 291 | ✓ | ✓ | ✓ | ✓ |
Mul 2 | 102 | 7 | 216 | 846 | 192 | ✓ | ✓ | ✓ | ✓ |
#Pages | #Chants | #Symbols | #Syllables | |
---|---|---|---|---|
without text variations | 124 | 649 | 69672 | 27629 |
incl. minor text variations | 275 | 1622 | 264186 | 71443 |
incl. major text variations | 304 | 1892 | 305746 | 81223 |
Dataset | #Pages | #Chants | #Staff | #Symb | #Clefs | #Notes | #Accids | #Chars | #Syllabels |
---|---|---|---|---|---|---|---|---|---|
Mul2 | 102 | 186 | 621 | 18,643 | 711 | 17,747 | 185 | 16,416 | 5233 |
Graduale no | 124 | 649 | 649 | 69,672 | 691 | 68,732 | 249 | 92,312 | 28,702 |
Graduale minor | 275 | 1622 | 1622 | 264,192 | 2470 | 260,613 | 1109 | 230,735 | 71,443 |
Graduale all | 304 | 1892 | 1892 | 305,746 | 2962 | 301,564 | 1220 | 262,333 | 81,223 |
Mul2 Sub1 | 20 | 58 | 560 | 4548 | 164 | 4363 | 21 | 3914 | 1271 |
Mul2 Sub2 | 20 | 53 | 139 | 3971 | 164 | 3776 | 31 | 4081 | 1292 |
Mul2 | Graduale Synopticum | |||
---|---|---|---|---|
Layout | ||||
Lines | 0% | 0% | 0% | 0% |
Lines & Regions | 0% | 0% | 0% | 0% |
Lines & Regions & Chants | 0.42% | - | - | - |
Symbols | ||||
Existence | 1.48/0.71% | 0.22% | 0.38% | 0.39% |
Existence & Type | 1.71/0.82% | 0.23% | 0.39% | 0.39% |
Existence & Type & Pitch | 2.22/1.53% | 0.58% | 0.90% | 0.91% |
Existence & Type & Pitch & Reading Order | 4.40/2.4% | 1.26% | 1.69% | 1.70% |
Existence & Type & Pitch & Reading Order & Graphical Connections | 13.9/7.4% | 2.70% | 3.92% | 4.07% |
Text | ||||
Text only | 14.9% | - | - | - |
Text with syllables and line segmentation | ||||
without GT text, but song repository | 3.8% | - | - | - |
with GT text | 1.48% | - | - | - |
Syllable Alignment | ||||
with inferred symbols and inferred text | 13.06/11.2% | - | - | - |
with inferred symbols and GT text | 9.0/7.12% | 3.63% | 4.36% | 6.61% |
with GT symbols and GT text | 2.3% | 2.70% | 2.70% | 2.70% |
Corrector | #Pages | Symbols Level | Text Level | Total Time | Time/ Page | Tool | Dataset |
---|---|---|---|---|---|---|---|
Person 1 | 10 | 11.6 | 20 | 31.6 | 3.16 | OMMR4all | Mul2 Sub1 (10) |
20 | 22.8 | 39.23 | 62.03 | 3.1 | OMMR4all | Mul2 Sub1 (all) | |
Person 2 | 10 | 9.72 | 22 | 31.72 | 3.72 | OMMR4all | Mul2 Sub1 (10) |
20 | 19.83 | 43 | 62.83 | 3.14 | OMMR4all | Mul2 Sub1 (all) | |
Person 3 | 10 | - | - | 25.95 | 2.60 | OMMR4all | Mul2 Sub2 (10) |
20 | - | - | 39.43 | 1.975 | OMMR4all | Mul2 Sub2 (all) | |
Person 2 | 5 | 55.4 | 33.5 | 88.9 | 17.78 | Monodi+ | Mul2 Sub1 (5) |
Post-Processing | Experiment | Line CER | WER | Chant CER |
---|---|---|---|---|
No | Mixed | 24.1% | 60.3% | 23.3% |
Fine-tuned | 14.9% | 43.9% | 14.2% | |
Yes | Mixed | 4.7% | 12.1% | 3.4% |
Fine-tuned | 3.8% | 9.3% | 2.6% |
Post-Processing | Experiment | M1 | M2 |
---|---|---|---|
No | Mixed | 36.7/35.3% | 16.1/14.0% |
Fine-tuned | 26.1/24.8% | 12.3/10.1% | |
GT (Symbols) no Fine-tune | 31.9% | 8.2% | |
GT (Symbols) with Fine-tune | 21.4% | 5.2% | |
GT (Symbols & Text) | 2.3% | 0.1% | |
Yes | Mixed | 14.1/12.8% | 10.2/8.4% |
Fine-tuned | 13.1/11.2% | 9.7/7.5% | |
GT (Symbols) no Fine-tune | 8.4% | 3.6% | |
GT (Symbols) with Fine-tune | 7.2% | 3.0% | |
GT (Symbols & Text) | 2.3% | 0.1% |
The statements, opinions and data contained in all publications are solely those of the individual author(s) and contributor(s) and not of MDPI and/or the editor(s). MDPI and/or the editor(s) disclaim responsibility for any injury to people or property resulting from any ideas, methods, instructions or products referred to in the content. |
Share and Cite
Hartelt, A.; Eipert, T.; Puppe, F. Optical Medieval Music Recognition—A Complete Pipeline for Historic Chants. Appl. Sci. 2024 , 14 , 7355. https://doi.org/10.3390/app14167355
Hartelt A, Eipert T, Puppe F. Optical Medieval Music Recognition—A Complete Pipeline for Historic Chants. Applied Sciences . 2024; 14(16):7355. https://doi.org/10.3390/app14167355
Hartelt, Alexander, Tim Eipert, and Frank Puppe. 2024. "Optical Medieval Music Recognition—A Complete Pipeline for Historic Chants" Applied Sciences 14, no. 16: 7355. https://doi.org/10.3390/app14167355
Article Metrics
Article access statistics, further information, mdpi initiatives, follow mdpi.

Subscribe to receive issue release notifications and newsletters from MDPI journals
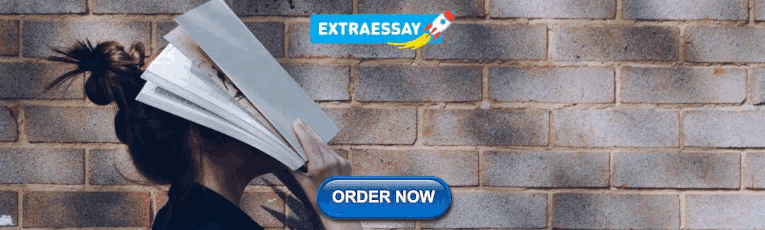
IMAGES
COMMENTS
Augmented assignment operators have a special role to play in Python programming. It basically combines the functioning of the arithmetic or bitwise operator with the assignment operator. ... Examples: Input: N = 11 Output: Odd Input: N = 10 Output: Even Following Bitwise Operators can be used to check if a number is odd or even: 1. Using ...
Unlike normal assignment operator, Augmented Assignment Operators are used to replace those statements where binary operator takes two operands says var1 and var2 and then assigns a final result back to one of operands i.e. var1 or var2. For example: statement var1 = var1 + 5 is same as writing var1 += 5 in python and this is known as augmented ...
Augmented assignment (or compound assignment) is the name given to certain assignment operators in certain programming languages (especially those derived from C).An augmented assignment is generally used to replace a statement where an operator takes a variable as one of its arguments and then assigns the result back to the same variable. A simple example is x += 1 which is expanded to x = x + 1.
The augmented assignment operator performs the operation on the current value of the variable and assigns the result back to the same variable in a compact syntax. For example: x = 2x += 3 # x = x + 3print(x) # 5. Here x += 3 is equivalent to x = x + 3. The += augmented assignment operator adds the right operand 3 to the current value of x ...
Python supports what are known as augmented assignments. An augmented assignment combines the assignment operator with another operator to make the statement more concise. ... Another interesting example of using an augmented assignment is when you need to implement a countdown while loop to reverse an iterable. In this case, you can use the ...
This PEP describes the augmented assignment proposal for Python 2.0. This PEP tracks the status and ownership of this feature, slated for introduction in Python 2.0. It contains a description of the feature and outlines changes necessary to support the feature. This PEP summarizes discussions held in mailing list forums [1], and provides URLs ...
Augmented assignments in Python. Augmented assignments create a shorthand that incorporates a binary expression to the actual assignment. For instance, the following two statements are equivalent: a = a + b. a += b # augmented assignment. In the code fragment below we present all augmented assignments allowed in Python language. a += b. a -= b.
Augmented Assignments — EAS503: Python for Data Scientists. 1.9. Augmented Assignments #. An augmented assignment merges an assignment statement with an operator, resulting in a more concise statement. The execution of an augmented assignment involves the following steps: Evaluate the expression on the right side of the = sign to yield a value.
#aiinsightswithahsanUnlock the full potential of Python with our in-depth tutorial on augmented assignment operators! In this video, we focus on two powerful...
Augmented assignment in Python is a testament to the language's philosophy of simplicity and efficiency. By incorporating these operators into your coding practice, you can write more concise and readable code, reducing the potential for errors and enhancing overall code quality. Whether you are working on mathematical operations, manipulating ...
The augmented assignment expression is also known as the inline augmented assignment. As indicated by its line, it's an inline operation such that we define a variable where it's freshly evaluated — in essence, we combine evaluation and assignment into a single step. ... Consider the following example for a plausible use case. def ...
The augmented assignment statement combines an operator with assignment. A common example is this: a += 1. This is equivalent to. a = a + 1. When working with immutable objects (numbers, strings, and tuples) the idea of an augmented assignment is syntactic sugar. It allows us to write the updated variable just once.
Enter augmented assignment operators. Don't be afraid of their fancy name — these operators are simply an arithmetic operator and an assignment operator all in one. In the example above, I could do the same thing with an augmented assignment operator like so:
score = score + 5; Augmented assignment operators allow us to do that with less typing: score += 5; There are augmented assignment operators for each of the arithmetic operators. In the table below, assume var is a variable that has been declared and initialized. Augmented Assignment Operator.
In Python, you can increment a variable using the augmented assignment operator "+=". This operator adds the value on the right side of the operator to the current value of the variable on the left side of the operator. ... Let's take a look at an example: x = 5 x += 1 print(x) # outputs 6 In this example, we first set the variable x ...
Introduction: Augmented Assignment Operators In Python. As you may already know that assignment (=) operator is used to assign values. If we just use assignment operator then it'll be a simple ...
Abstract. This is a proposal to allow augmented assignments such as x += 1 to be used as expressions, not just statements. As part of this, NAME := EXPR is proposed as an inline assignment expression that uses the new augmented assignment scoping rules, rather than implicitly defining a new local variable name the way that existing name binding ...
An augmented assignment is generally used to replace a statement where an operator takes a variable as one of its arguments and then assigns the result back to the same variable. A simple example is x += 1 which is expanded to x = x + 1. augmented-assignment. augmented-assignment ...
Example: In this code we have two variables 'a' and 'b ... Augmented assignment operators have a special role to play in Python programming. It basically combines the functioning of the arithmetic or bitwise operator with the assignment operator. 4 min read. Increment += and Decrement -= Assignment Operators in Python.
01:08 It will actually be the original {1}. This is because x = x | {2} created a new set of {1, 2} and then bound that back to x. y still pointed to the original {1}. 01:25 The first example mutates x, the second example reassigns x. Let's take a deep dive into how augmented assignment actually works. You saw that many of the modifying set ...
10. Yes, those are the same. Python's augmented assignment is not an expression, it is a statement, and doesn't play in expression precedence rules. += is not an operator, and instead it's part of the augmented assignment statement syntax. So everything to the right of the += is an expression, but += itself is not, so the assignment will always ...
Python Assignment Operators. Assignment operators are used to assign values to variables: Operator Example Same As Try it = x = 5: x = 5: ... Examples might be simplified to improve reading and learning. Tutorials, references, and examples are constantly reviewed to avoid errors, but we cannot warrant full correctness ...
Augmented operators in Python have to return the final value to be assigned to the name they are called on, usually (and in your case) self. Like all Python methods, missing a return statement implies returning None. Also, Never ever ever raise Exception, which is impossible to catch sanely.
Augmented passive content learning strategies: Note-taking ... Examples of these active learning activities included: practice questions, real-life problem-solving, analysing and making predictions from data, research questions, at home experiments, opinion polling, simulations, dilemmas, and scenarios, as well as collaborative tutorials ...
The following elements need to be extracted and examples are highlighted in the image: Music-lyric region pairs, note components (start, gapped, i.e., connected, looped, i.e., graphically connected), clefs (C-clef, F-clef), accidentals (sharp, flat, natural), drop capitals (orange marked), individual chants (blue marked), lyrics and the ...