
- Data Structure
- Coding Problems
- C Interview Programs
- C++ Aptitude
- Java Aptitude
- C# Aptitude
- PHP Aptitude
- Linux Aptitude
- DBMS Aptitude
- Networking Aptitude
- AI Aptitude
- MIS Executive
- Web Technologie MCQs
- CS Subjects MCQs
- Databases MCQs
- Programming MCQs
- Testing Software MCQs
- Digital Mktg Subjects MCQs
- Cloud Computing S/W MCQs
- Engineering Subjects MCQs
- Commerce MCQs
- More MCQs...
- Machine Learning/AI
- Operating System
- Computer Network
- Software Engineering
- Discrete Mathematics
- Digital Electronics
- Data Mining
- Embedded Systems
- Cryptography
- CS Fundamental
- More Tutorials...
- Tech Articles
- Code Examples
- Programmer's Calculator
- XML Sitemap Generator
- Tools & Generators
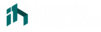
- C++ Programs
- C++ Programs - Home
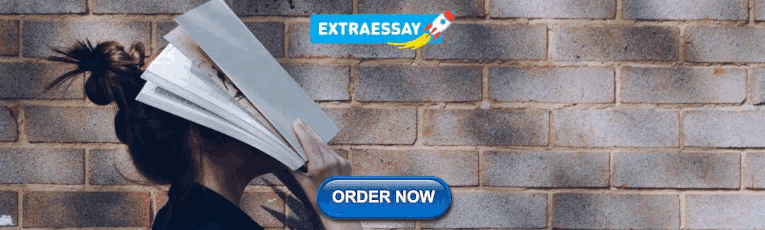
C++ Most popular & Searched Programs
- C++ Basic Input/Output Programs
C++ Constructor & Destructor Programs
C++ manipulators programs, c++ inheritance programs, c++ operator overloading programs, c++ file handling programs, c++ bit manipulation programs.
- C++ Classes & Object programs (Set 1)
- C++ Classes & Object programs (Set 2)
- C++ - Print right angled pyramid of numbers
- C++ - Keep calculate sum of digits of a number
- C++ - Skip some of array elements
- C++ - Declaring & printing different constants
- C++ - Example of delay() function
- C++ - Print your name randomly
- C++ - Print maximum possible time using six of nine given single digits
- C++ - Find last index of a character in a string
- C++ - Get week day from given date
- C++ - Set date & time
- C++ - Reading date & time
- C++ - Get previous date of given date
- C++ - Find total number of days in given month of year
- C++ - Set network settings for IPv6 Network
- C++ - Pad octets of IP Address with Zeros
- C++ - Set MAC address in Linux Devices
- C++ - Get MAC address of Linux based network device
- C++ - Set IP address, subnet mask, network gateway
- C++ - Check given string is numeric or not
- C++ - Check given date is in valid format or not
- C++ - Add seconds to the time
- C++ - Find Fibonacci number
- C++ - Find next greatest number from the same set of digits
- C++ - Convert number to word
- C++ - Check whether a string2 can be formed from string1
- C++ - Print a spiral matrix
- C++ - Find the frequency of a character in a string
- C++ - Find factorial of large numbers using array
- C++ - Generate random alphabets
- C++ - Print pattern of stars till N number of rows
- C++ - Print a chessboard pattern
- C++ - Print a Pascal Triangle
- C++ - Reverse a number
- C++ - Sort an array in Descending Order
- C++ - Sort an array in Ascending Order
- C++ - Convert lowercase to uppercase & vice versa
- C++ - Check leap year
- C++ - Check if a number is even using Recursion
- C++ - Find odd or even number without using modulus operator
- C++ - Check EVEN or ODD
- C++ - Add two times
- C++ - Display prime numbers
- C++ - Check prime number
- C++ - Find factorial of a number
- C++ - Display name & age
- C++ - Read a string
- C++ - Add two integer numbers using class
- C++ - Add two integer numbers using pointers
- C++ - Add two integer numbers using function
- C++ - Add two integer numbers
C++ Basic I/O Programs
- C++ - Print "Hello World"
- C++ - Read character array
- C++ - Cascading cout & cin
- C++ - Use of Resolution Operator(SRO)
- C++ - Use of reference variable
- C++ - Use function as a LVALUE using reference variable
- C++ - Inline Function Example
- C++ - Default Argument Example
- C++ - Methods of passing in function
- C++ - Function overloading example
- C++ - Read string using cin.getline()
- C++ - Generate random numbers
- C++ - Print Reverse Triangle Bridge Pattern
- C++ - Example of default constructor or no argument constructor
- C++ - Example of parameterized constructor
- C++ - Example of copy constructor
- C++ - Example of constructor overloading
- C++ - Example of member initializer list
- C++ - Example of destructors
- C++ - Example of constructor using this pointer
- C++ - Example of constructor with default arguments
- C++ - Dynamic Initialization of Objects
- C++ - Set values of data members
- C++ - Create a class with inline functions
- C++ - Create a constructor with default arguments
- C++ - Create a class with two constructors
- C++ - Example of endl manipulator
- C++ - Example of setw manipulator
- C++ - Example of setfill manipulator
- C++ - Example of setprecision manipulator
- C++ - Example of setbase manipulator
- C++ - Example of simple inheritance
- C++ - Example of private simple inheritance
- C++ - Read & print student's information using two classes and simple inheritance
- C++ - Example of multilevel inheritance
- C++ - Read ≈ print employee information using multiple inheritance
- C++ - Example of multiple inheritance
- C++ - Get square and cube of a number
- C++ - Read & print employee information with department and pf information using hierarchical inheritance
- C++ - Unary minus (-) operator overloading
- C++ - Unary increment (++) and decrement (--) operator overloading
- C++ - Unary logical NOT operator overloading
- C++ - Add two objects using binary plus (+) operator overloading
- C++ - Add two distances using binary plus (+) operator overloading
- C++ - Create a file
- C++ - Read a text file
- C++ - Write & read text in/from file
- C++ - Write & read values using variables in/from file
- C++ - Write & read object using read() & write() functions
- C++ - Eample of tellg() & tellp() function
- C++ - Example of tellg(), seekg(), & seekp() Functions
- C++ - Read integers from a text file with ifstream
- C++ - Read data from a text file
- C++ - Append text to a text file
- C++ - Print all possible subset of a set
- C++ - Change case of a character using bit manipulation
- C++ - Find total Number of bits required to represent a number in binary
- C++ - Find next & previous power of two of a given number
C++ Classes & Object Programs
- C++ - Create a simple class & object
- C++ - Create an object of a class & access class attributes
- C++ - Create multiple objects of a class
- C++ - Create class methods
- C++ - Define a class method
- C++ - Assign values to private data members w/o using constructor
- C++ -Create an empty class
- C++ - Create a class with setter & getter methods
- C++ - Create a class to read & add two distance
- C++ - Create a class for student
- C++ - Create a class for student to get & print details of N students
- C++ - Create class to read & add two times
- C++ - Create class convert time in HH:MM:SS format
- C++ - Create class convert time seconds
- C++ - Example of friend function with class
- C++ - Count created objects
- C++ - Check the year is leap year or not
- C++ - Add N natural numbers
- C++ - Find largest number b/w three numbers
- C++ - Check if character is vowel or consonant
- C++ - Check if number is odd or even
- C++ - Find power of a number
- C++ - Check if number is palindrome
- C++ - Find reverse of a number
- C++ - Change string from uppercase to lowercase
- C++ - Check number is Armstrong number or not
- C++ - Find largest number of an array
- C++ - Count vowels, consonant, digits & special characters
- C++ - Find length of a string
- C++ - Find addition of two matrices
- C++ - Find multiplication of two matrices
- C++ - Find transpose of a matrix
- C++ - Find all roots of a quadratic equation
- C++ - Convert binary number to octal number
- C++ - Convert octal number to binary number
- C++ - Convert decimal number to octal number
- C++ - Convert octal number to decimal number
- C++ - reverse the string
- C++ - Change string from lowercase to uppercase using class
- C++ - Change string to sentence case
- C++ - Find smallest number in the array
- C++ - Sort an array in ascending order
- C++ - Sort an array in descending order
- C++ - Convert the temperature from Celsius to Fahrenheit
- C++ - Convert the temperature from Fahrenheit to Celsius
- C++ - Print prime numbers in a range
- C++ - Print odd numbers in a range
- C++ - Print even numbers in a range
- C++ - Print Armstrong numbers in a range
- C++ - Print palindrome numbers in a range
- C++ - Find sum of all elements of the array
- C++ - Find product of all elements of the array
- C++ - Find product of all digits of a number
- C++ - Find sum of all digits of a number
- C++ - Check if the number is perfect or not
- C++ - Print the perfect numbers in the given range
- C++ - Convert octal number to hexadecimal
- C++ - Convert hexadecimal to octal number
- C++ - Convert hexadecimal to decimal number
- C++ - Convert decimal number to hexadecimal
- C++ - Find second largest number in the array
- C++ - Find second smallest number in the array
- C++ - Change string to toggle case
- C++ - Change string to title case
- C++ - count total words in the string
- C++ - Convert characters of a string to opposite case using class
- C++ - Find mean of the array
- C++ - Find median of the array
- C++ - Find mode of the array
- C++ - Find sum of digits of numbers between numbers
- C++ - Calculate the minutes between two time periods
- C++ - Check if the numbers in the array are in arithmetic progression
- C++ - Convert the minutes to time
- C++ - Replace a word by another given word in the string
- C++ - Print the leap years in the given time period
- C++ - Find sum of all integers in a string
- C++ - Find sum of prime numbers in a range
- C++ - Find product of prime numbers in a range
- C++ - Find product of digits of numbers between a given range
- C++ - Find product of all integers in a string
- C++ - Check if the numbers in the array are in geometric progression
- C++ - Find difference between the largest & smallest numbers in the array
- C++ - Find area of shapes
- C++ - Find perimeter of shapes
- C++ - Swap first & last digits of a number
- C++ - Add two binary numbers
- C++ - Find area of polygon of several sides
- C++ - Find area of hexagon
- C++ - Calculate the simple interest
- C++ - Calculate the compound interest
- C++ - Find third angle of triangle
- C++ - Convert speed from km/h to miles/h
- C++ - Convert speed from miles/h to km/h
- C++ - Print the sum of all odd & even numbers in a range
- C++ - Print the product of all odd & even numbers in a range
- C++ - Find volume of shapes
- C++ - Find surface area of shapes
- C++ - Reverse name & surname
- C++ - Convert temperature from Celsius to Kelvin
- C++ - Convert temperature from Kelvin to Celsius
- C++ - Convert temperature from Fahrenheit to Kelvin
- C++ - Convert temperature from Kelvin to Fahrenheit
- C++ - Find area of a triangle by heron's formula
- C++ - Find product of largest number & smallest number in array
- C++ - Find largest character in string
- C++ - Sort string of characters in ascending order
- C++ - Sort string of characters in descending order
- C++ - Find smallest character in string
- C++ - Find second largest character in string
- C++ - Find second smallest character in string
- C++ - Check if string is palindrome
- C++ - Find sum of largest number & smallest number in array
- C++ - Check if string is in alphanumeric
- C++ - Check if string is in uppercase
- C++ - Check if string is in lowercase
- C++ - Check if string is in numeric
- C++ - Check if string contains only alphabets
- C++ - Reverse every word of a string
- C++ - identify missing character in string
- C++ - Print Fibonacci series up to N
- C++ - Find quotient & remainder
- C++ - Convert decimal number to binary
- C++ - Convert binary to decimal number
- C++ - Print number & string entered by user
- C++ - Check whether number is prime or not
- C++ - Find LCM of two numbers
- C++ - Find GCD of two numbers
- C++ - Print table of number entered
- C++ - Find sum of adjacent elements of array
- C++ - Find product of adjacent elements of array
- C++ - Find difference of adjacent elements of array
- C++ - Find occurrence of number in array
- C++ - Find most occurring element in an array of integers
- C++ - Separate even & odd numbers in array
- C++ - Find sum of even & odd numbers of array
- C++ - Find product of even & odd numbers of array
- C++ - Search an element in array
- C++ - Sort array in even-odd form
- C++ - Merge two arrays
- C++ - Add two arrays
- C++ - Find product of two arrays
- C++ - Find sum of two arrays one in reverse
- C++ - Find product of two arrays one in reverse
- C++ - Check whether matrix is an identity matrix or not
- C++ - Check if matrices are equal or not
- C++ - Find sum of each row of matrix
- C++ - Check whether matrix is a sparse matrix or not
- C++ - Find sum of both diagonals of matrix
- C++ - Find product of both diagonals of matrix
- C++ - Find product of each row of matrix
- C++ - Find subtraction of two matrices
- C++ - Delete an element of an array
- C++ - Insert an element of an array
- C++ - Check if number is ugly or not
- C++ - Print ugly numbers in a range
- C++ - Check if number is abundant or not
- C++ - Print abundant numbers in a range
- C++ - Check if number is deficient or not
- C++ - Print deficient numbers in a range
- C++ - Check if number is kaprekar or not
- C++ - Print kaprekar numbers in a range
- C++ - Check if number is narcissistic or not
- C++ - Print narcissistic numbers in a range
- C++ - Check if number is happy or not
- C++ - Print happy numbers in a range
- C++ - Check if number is disarium or not
- C++ - Print disarium numbers in a range
- C++ - Check if number is pronic or not
- C++ - Print pronic numbers in a range
- C++ - Check if number is automorphic or not
- C++ - Print automorphic numbers in a range
- C++ - Convert number from integer to roman
- C++ - Convert number from roman to integer
- C++ - Find sum of each column of matrix
- C++ - Find product of each column of matrix
- C++ - count total occurrences of each element in an array
Advertisement
Home » C++ programs
C++ Inheritance programs/examples
Inheritance is a feature of object oriented programming system , by which a class can inherit the commonly used properties/features of another classes. In this section you will get solved c++ programs using inheritance : simple inheritance , multiple inheritance , multilevel inheritance , hybrid inheritance , hierarchical inheritance .
We are providing here set of programs on privately and publicly inheritance.
List of C++ Inheritance programs
- C++ program to demonstrate example of simple inheritance .
- C++ program to demonstrate example of private simple inheritance .
- C++ program to read and print student's information using two classes and simple inheritance .
- C++ program to demonstrate example of multilevel inheritance .
- C++ program to read and print employee information using multiple inheritance .
- C++ program to demonstrate example of multiple inheritance .
- C++ program to demonstrate example of hierarchical inheritance to get square and cube of a number.
- C++ program to read and print employee information with department and pf information using hierarchical inheritance .
List of most popular & searched C++ programs
Showing latest posts on top
- C++ Program to print right angled (Right oriented) pyramid of numbers. In this program, we are going to learn how to print numbered pyramids of numbers (which is right angled and right oriented)? This post contains solved program with output and explanation.
- C++ Program to print right angled pyramid of numbers. In this program, we are going to learn how to print numbered pyramids of numbers? This post contains solved program with output and explanation.
- C++ program to keep calculate the sum of the digits of a number until the number is a single digit. In this program, we are going to implement logic to find sum of digits until the number is a single digits in C++ programming language.
- How to skip some of the array elements in C++? Learn: How to skip some of the array elements using C++ program? Here, using an example - we going to understand the concept of skipping some of the elements in C++.
- Example of declaring and printing different constants in C++. Declaring and printing different constant in C++: Here, we will learn to declare and print the value of different type of constants using C++ program.
- C++ program to demonstrate example of delay() function. dos.h delay() function in C++: Here, we will learn about the delay() function of dos.h header file in C++ through a simple example/program.
- C++ program to print your name randomly on the screen with colored text. Print your name on output screen randomly with colored text and background color using C++ program: Here you will learn some of the in-built functions that are using to print randomly, colored, print text of specified position etc.
- Sieve of Eratosthenes to find prime numbers. Learn: What is Sieve of Eratosthenes? And how to find prime numbers using this algorithm? This article contain solved program in C++ based on Sieve of Eratosthenes algorithm.
- C++ program to print the maximum possible time using six of nine given single digits. The Objective is to form the maximum possible time (in HH:MM:SS and 12 hour format) using any six of nine given single digits (not necessarily distinct).
- Find last index of a character in a string using C++ program. In this post, we are going to learn: how we can get the last index of a character in a string using C/C++ program using simple method?
- C++ program to get week day from given date. Learn: How to get weekday from a given date in C++ program? Here I am writing a C++ code by using you can able to access the weekday of given date.
- Set date and time in Linux Operating System using C++ program. Learn: How to define/set date and time in Linux Operating System using C++ program? Here we are using system() function to set date time.
- Reading date and time from Linux operating system using C++ program. Learn: How to get current date and time from Linux Operating System using C++ program? This program will read date, time from Operating system and print on the standard output device.
- C++ program to get previous date of given date. Given a date in DD, MM and YYYY format, you have to find the previous date.
- C++ program to find total number of days in given month of year. C++ program to find/print total number of days in a month, in this program, we will input month, year and program will print total number of days in that month, year.
- C++ program to set network settings for IPv6 Network in Linux Devices. Learn: How to set network settings for IPv6 based Network in Linux Devices? Here, we will learn to set IP address, prefix and Gateway for IPv6 based Network.
- C++ program to pad octets of IP Address with Zeros. Learn: How to pad IP Address octets with Zeros? In this C++ program, we will learn to pad each octet of an IP Address.
- C++ program to set MAC address in Linux Devices. Learn: How to set MAC address (physical address) in Linux devices using C++ program, this program is compiled and executed in G++ compiler.
- C++ program to get MAC address of Linux based network device. Learn: How to get/display MAC address of a Linux based network devices using C++ program? This program is compiled and executed in G++ compiler.
- C++ program to set IP address, subnet mask, network gateway in Linux System. Learn: How to set network settings like IP Address, Subnet Mask, Network Gateway using C++ program in Linux System using G++ Compiler?
- C++ program to check given string is numeric or not. Learn: How to check given string is numeric or not? In this C++ program, we are going to learn how to check whether a given a string is numeric or not?
- C++ program to check given date is in valid format or not. In this C++ program, we will read date and check whether it is in correct format or not?
- C++ program to add seconds to the time. Learn: How to add seconds in given time and print the time in HH:MM:SS format using C++ program, here we will add seconds in time (with using all conditions like time truncating etc).
- C++ program to find Fibonacci number using different methods. Learn: What to find fibonacci number using different methods, here you will find multiple methods to find the fibonacci number using C++ programs.
- C++ program to find the next greatest number from the same set of digits. Learn: How to find next greatest number from the same set of digits using a C++ program?
- C++ program to convert number to word (up to four digits only). Write a C++ program that will read an integer number (up to four digits) and convert it into words.
- C++ program to check whether a string2 can be formed from string1. This C++ program reads two Strings (String1 and String2) and checks whether String2 can be formed from String1, by printing ‘Yes' otherwise ‘No'.
- C++ program to print a spiral matrix. Printing a spiral matrix up to given number using C++ program: A spiral matrix is a matrix consist of natural numbers up to n^2.
- C++ program to find the frequency of a character in a string using Count Array. This C++ program will read a string and count frequency of a character using count array.
- C++ program to find factorial of large numbers using array. Learn: How to find factorial of large numbers in C++ using array, this program will explain finding the factorial of large number.
- C++ program to generate random alphabets and store them into a character array. In this program first we take two character arrays, one to store all the alphabets and other to print random characters into it. To keep it simple we have taken the length as 20.
- C++ program to print pattern of stars till N number of rows. This C++ program will read total number of rows from the user and print the star pattern till N rows.
- C++ Program to print a chessboard pattern . This C++ program will print a chessboard like pattern using loops.
- C++ Program to print a Pascal Triangle upto N depth . This C++ program will read the depth and print the Pascal Trainable up to input depth.
- C++ program to reverse a number . This program will read and integer number and print its reverse number in C++.
- C++ program to sort an array in Descending Order . This program will read an integer array (one dimensional array) and sort its elements in descending order.
- C++ program to sort an array in Ascending Order . This program will read an integer array (one dimensional array) and sort its elements in ascending order.
- C++ program to convert lowercase character to uppercase and vice versa . This program will read a character and converts lowercase to uppercase and uppercase to lowercase character.
- C++ program to check leap year . This program will read a year from the user and check whether it is Leap year or not.
- C++ Program to check if a number is even using Recursion . This program will read a number and check whether it is EVEN or ODD using recursion in C++.
- C++ Program to find odd or even number without using modulus operator . This program will read an integer number and check whether it is EVEN or ODD without using Modulus (%) Operator.
- C++ program to check EVEN or ODD . This program will read an integer number and check whether it is EVEN or ODD using three different methods.
- C++ program to add two times . This program will read two times and calculate their addition (total times in hours, minutes and seconds).
- C++ program to display prime numbers . This program will read maximum range of the numbers (N) and print all prime numbers between 2 to N.
- C++ program to check prime number . This program will read an integer number and check whether it is Prime or Not using User Defined Function.
- C++ program to find factorial of a number . This program will read an integer number and find its factorial using loop and user defined function.
- C++ program to display name and age . This program will read name and age of the person and display them on separate lines.
- C++ program to read a string . This program will read a string using cin and cin.getline().
- C++ program to add two integer numbers using class . This program will read two integer numbers from the user and calculate their addition/sum using class.
- C++ program to add two integer numbers using pointers . This program will read two integer numbers from the user and calculate their addition/sum using pointer.
- C++ program to add two integer numbers using function . This program will read two integer numbers from the user and calculate their addition/sum using user defined function.
- C++ program to add two integer numbers . This program will read two integer numbers from the user and calculate their addition/sum.
Comments and Discussions!
Load comments ↻
- Marketing MCQs
- Blockchain MCQs
- Artificial Intelligence MCQs
- Data Analytics & Visualization MCQs
- Python MCQs
- Python Programs
- Java Programs
- D.S. Programs
- Golang Programs
- C# Programs
- JavaScript Examples
- jQuery Examples
- CSS Examples
- C++ Tutorial
- Python Tutorial
- ML/AI Tutorial
- MIS Tutorial
- Software Engineering Tutorial
- Scala Tutorial
- Privacy policy
- Certificates
- Content Writers of the Month
Copyright © 2024 www.includehelp.com. All rights reserved.
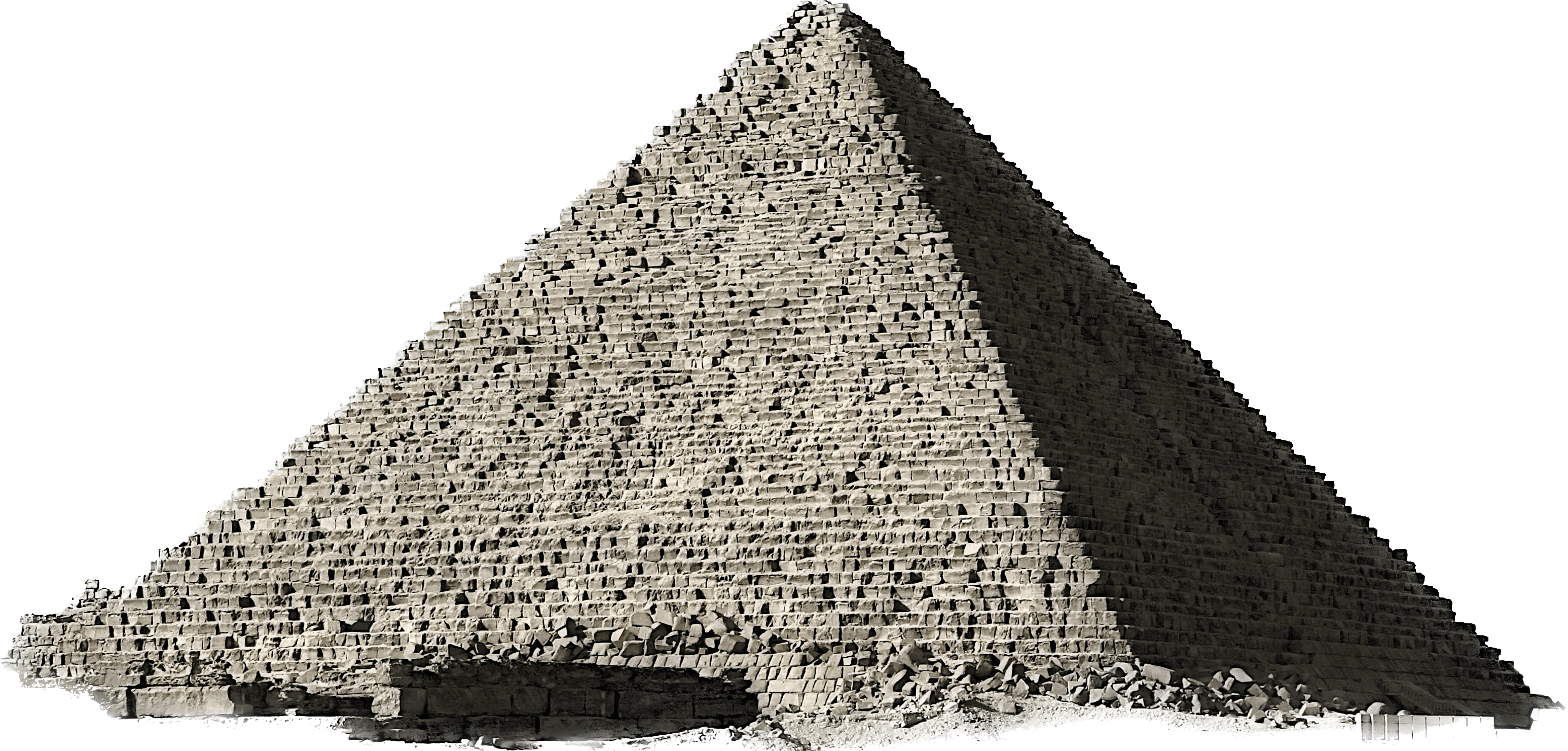
Khufu: Object-Oriented Programming using C++
10.1. exercises #.
Many of these exercises are taken from past exams of ECE 244 Programming Fundamentals courses at University of Toronto. The solutions are provided in the answer boxes.
Headings in this page classify the exercises into different categories: [Easy] , [Intermediate] , and [Challenging] . I suggest you start by easy exercises and work your way up to the challenging ones.
Question 11 in Fall 2018 Final Exam [Easy]
Consider the following class definitions and implementations, as well as a main function that uses them. All the code appears in one file and it compiles with no errors.
Indicate what each statement in the above main function prints, using the table below. If no output is produced, write “NONE”.
Statement | Output |
---|---|
a; | |
b("G"); | |
c ( "N"); | |
d(8); |
Statement | Output |
---|---|
a; |
|
b("G"); |
|
c ( "N"); |
|
d(8); |
|
Question 14 in Fall 2019 Final Exam [Easy]
Consider the following class definitions and implementations of classes, Shape and Circle . They are followed by a main function that utilizes these classes.
Indicate which of the following statements that appear in the main function compile with no errors or produce a compile time error.
Indicate also the reason in the table below.
Code snippet | Does the code snippet compile? If yes, Why? |
---|---|
s; | |
c; | |
r; | |
d; |
Code snippet | Does the code snippet compile? If yes, Why? |
---|---|
s; | No, an object of an abstract class cannot be created |
c; | No, Circle is an abstract class too as it doesn’t implement print |
r; | Yes |
d; | No, Rectangle::length is a protected data member that cannot be accessed outside the class |
Question 12 in Fall 2018 Final Exam [Intermediate]
Consider the following base and derived classes. They compile with no errors.
Consider each of the following main functions that use the above classes. You may assume that each main function includes the code of the classes above, including the #include ’s and the using namespace std;
For each main function, indicate if the function compiles with no errors or not (ignore warnings). If the function compiles with no errors, then indicate the output produced by the function?
Does the program compile with no errors? If it does compile with no errors, what is the output produced by the execution?
No, it doesn’t compile.
We cannot create objects of abstract classes.
Yes, the program compiles.
delete pc calls the destructor of Desert only as pc is a pointer of type Desert . We cannot access the destructor of Cookies through pc .
delete pi calls the destructor of IceCream first, then the destructor of Desert . pi is of type IceCream , and through it we can access both destructors.
No, the program doesn’t compile.
ps = pi; is not valid. Sundae is derived from IceCream . ps cannot point to an object IceCream , as it cannot access its members that don’t exist.
ps->cost() will call the cost() of IceCream as cost() is a virtual function, hence ps will call the function according to the type of the object, not the pointer.
Question 15 in Fall 2019 Final Exam [Easy]
Consider the following class definitions.
The following declaration appears in the nohelp member function of the class DerivedC .
Indicate whether the following statements is correct code (i.e., compiles with no errors), or incorrect code (i.e., generates a compile time error). Assume the statements in the rows of the table also appear in the nohelp member function of the class DerivedC .
Statement | Correct/Incorrect |
---|---|
= 8; | |
= 10; | |
= 12; | |
= 4; |
Statement | Correct/Incorrect |
---|---|
= 8; | Incorrect ( is a private member of the class) |
= 10; | Correct ( is protected in and can be access in ) |
= 12; | Correct ( is private member of the class and can be accessed in ) |
= 4; | Correct ( is a public member of ) |
Question 16 in Fall 2019 Final Exam [Intermediate]
Consider each of the following main functions that use the above classes. You may assume that each main function includes the code of the classes above, including the #include ’s and the using namespace std; .
For each main function, if the function compiles with no errors (ignore warnings), then write the output produced by the function. If the function compiles with errors, then write: “compile errors”.
We assume that p is destructed before c .
The tricky part is that delete pr will execute the destructor of the type of pr , since the destructor is not a virtual function.
Compile-time error for line c = new CircuitElement(5); , as you cannot make a pointer to a derived object c point to a base object CircuitElement .
Compile-time error for line pr = r; , as pr is a pointer of derived object that cannot point to a base object.
We assume we destruct e then p . It is possible to do e = r as e is a base object and r is derived.
We can make a base object point to a derived object, hence CircuitElement* e = new PowerCapacitor(500, 1000); is possible.
Since the print() function is virtual in CircuitElement , it is virtual in all derived classes. Therefore when e->print() is executed, the print() function of the object e is pointing, which is PowerCapacitor to is called.
The getPower function is not virtual, therefore when c->getPower() is called we call the getPower function of Capacitor – the type of c .
Since the memory is dynamically allocated and not freed, no destructors are called before returning from main .
Compile-time error.
The error exists at r->powerResistance() . powerResistance() is not a virtual function, hence when r->powerResistance() is executed, powerResistance() of Resistor is called. There is no member named powerResistance in Resistor class.
Question 5 in Fall 2022 Final Exam [Intermediate] :
The University of Toronto wants to manage students from different departments using a C++ program. Students from all departments will share the following base class:
Derived from student class, the programmers then write another class for engineering students. All engineering students have access to the ECF server:
Derived from eng_student , programmers then write two classes for ECE students and MIE students. Unlike MIE students, ECE students have their designated server called UG server:
In addition, programmers write a new derived class for Computer Science (CS) students. Unlike engineering students, they use Markus servers instead of ECF servers:
Read the implementations of these five classes carefully and answer the following questions.
You write the following piece of code to declare four students. However, you get a compile-time error. Why doesn’t it compile? (Note: you don’t need to fix anything in the class declarations and implementations given above.)
student class is an abstract function. eng_student inherits from student class; however, it is an abstract class too as it did not implement print_department pure virtual function that was in student class.
Now, an array named student_list is declared and initialized as follows. Write the output generated by std::cout .
new cs_student("Leo", 0, 10) would call the constructor of the base class student , then derived class cs_student . This prints.
new ece_student("Bill", 1, 11, 0) would call the constructor of the base class student , then eng_student , then ece_student . This prints.
The same idea follows for new mie_student("Ellie", 2, 12) and new ece_student("Haley", 3, 13, 1) .
After the student_list initialized in question 2, the following for loops will be executed. What will be the output generated by these two loops?
Since print_department(); and print_server() are virtual functions, the function invoked would be determined based on the type student_list[i] points to. In the student_list array, we have CS, ECE, MIE and ECE, and these would be the objects on which the functions get invoked.
C++ Tutorial
C++ functions, c++ classes, c++ data s tructures, c++ reference, c++ examples, c++ inheritance, inheritance.
In C++, it is possible to inherit attributes and methods from one class to another. We group the "inheritance concept" into two categories:
- derived class (child) - the class that inherits from another class
- base class (parent) - the class being inherited from
To inherit from a class, use the : symbol.
In the example below, the Car class (child) inherits the attributes and methods from the Vehicle class (parent):
Why And When To Use "Inheritance"?
- It is useful for code reusability: reuse attributes and methods of an existing class when you create a new class.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Browse Course Material
Course info, instructors.
- Kyle Murray
Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
- Software Design and Engineering
Learning Resource Types
Introduction to c and c++, c++ inheritance, lecture notes.
Lecture 6: C++ Inheritance (PDF)
Lab Exercises
Take a look at this example code:
Put it in a file named lab6.cpp and then compile it like this:
Verify your understanding of how the virtual keyword and method overriding work by performing a few experiments:
- Remove the virtual keyword from each location individually, recompiling and running each time to see how the output changes. Can you predict what will and will not work?
- Try making Shape::draw non-pure by removing = 0 from its declaration.
- Try changing shape (in main() ) from a pointer to a stack-allocated variable.
Assignment 6
In the file rps.cpp, implement a class called Tool . It should have an int field called strength and a char field called type . You may make them either private or protected. The Tool class should also contain the function void setStrength(int) , which sets the strength for the Tool .
Create 3 more classes called Rock , Paper , and Scissors , which inherit from Tool . Each of these classes will need a constructor which will take in an int that is used to initialize the strength field. The constructor should also initialize the type field using 'r' for Rock, 'p' for Paper , and 's' for Scissors .
These classes will also need a public function bool fight(Tool) that compares their strengths in the following way:
- Rock’s strength is doubled (temporarily) when fighting scissors, but halved (temporarily) when fighting paper.
- In the same way, paper has the advantage against rock, and scissors against paper.
- The strength field shouldn’t change in the function, which returns true if the original class wins in strength and false otherwise.
You may also include any extra auxiliary functions and/or fields in any of these classes. Run the program without changing the main function, and verify that the results are correct.
Solutions are not available for this assignment.

You are leaving MIT OpenCourseWare
Table of Contents
What is inheritance in c++, what are child and parent classes, importance of inheritance in c++, implementing inheritance in c++, why and when to use inheritance, visibility modes, types of inheritance in c++, diamond problem, how to make a private member inheritable, advantages of inheritance in c++, base class and derived class in c++, access control in c++, modes of inheritance, choose the right software development program, final thoughts, types of inheritance in c++: explained with examples.
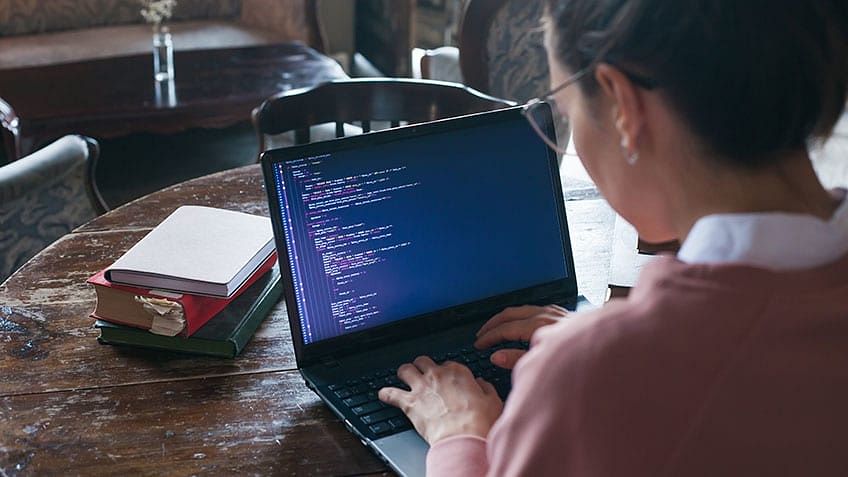
Inheritance is one of four pillars of Object-Oriented Programming (OOPs) . It is a feature that enables a class to acquire properties and characteristics of another class . Inheritance allows web developers to reuse your code since the derived class or the child class can reuse the members of the base class by inheriting them. Consider a real-life example to clearly understand the concept of inheritance. A child inherits some properties from his/her parents, such as the ability to speak, walk, eat, and so on. But these properties are not especially inherited in his parents only. His parents inherit these properties from another class called mammals. This mammal class again derives these characteristics from the animal class. Inheritance works in the same manner.
During inheritance, the data members of the base class get copied in the derived class and can be accessed depending upon the visibility mode used. The order of the accessibility is always in a decreasing order i.e., from public to protected. There are mainly five types of Inheritance in C++ that you will explore in this article. They are as follows:
Single Inheritance
Multiple inheritance, multilevel inheritance, hierarchical inheritance, hybrid inheritance.
Inheritance is a method through which one class inherits the properties from its parent class. Inheritance is a feature in which one new class is derived from the existing ones. The new class derived is termed a derived class, and the current class is termed a Parent or base class. Inheritance is one of the most essential features of Object Oriented Programming . Rather than defining new data functions while creating a class, you can inherit the existing class's data functions. The derived class takes over all the properties of the parent class and adds some new features to itself. For example, using inheritance, you can add the members' names and descriptions to a new class.
Want a Top Software Development Job? Start Here!
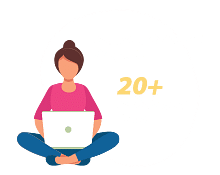
To clearly understand the concept of Inheritance, you must learn about two terms on which the whole concept of inheritance is based - Child class and Parent class.
- Child class: The class that inherits the characteristics of another class is known as the child class or derived class. The number of child classes that can be inherited from a single parent class is based upon the type of inheritance. A child class will access the data members of the parent class according to the visibility mode specified during the declaration of the child class.
- Parent class: The class from which the child class inherits its properties is called the parent class or base class. A single parent class can derive multiple child classes (Hierarchical Inheritance) or multiple parent classes can inherit a single base class (Multiple Inheritance). This depends on the different types of inheritance in C++ .
The syntax for defining the child class and parent class in all types of Inheritance in C++ is given below:
class parent_class
//class definition of the parent class
class child_class : visibility_mode parent_class
//class definition of the child class
Syntax Description
- parent_class: Name of the base class or the parent class.
- child_class: Name of the derived class or the child class.
- visibility_mode: Type of the visibility mode (i.e., private, protected, and public) that specifies how the data members of the child class inherit from the parent class.
Get the Coding Skills You Need to Succeed
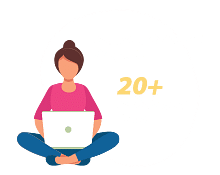
Instead of trying to replicate what already exists, it is always ideal to reuse it since it saves time and enhances reliability. In C++, inheritance is used to reuse code from existing classes. C++ highly supports the principle of reusability. Inheritance is used when two classes in a program share the same domain, and the properties of the class and its superclass should remain the same. Inheritance is a technique used in C++ to reuse code from pre-existing classes. C++ actively supports the concept of reusability.
To create a parent class that is derived from the base class below is a syntax that you should follow:
<derived_class_name> : <access-specifier>
<base_class_name>
/ /body
Here, class is a keyword which is used to create a new class, derived_class_name is the new class name which will inherit the properties of a base class, and access-specifier defines through mode the derived class has been created, whether through public, private or protected mode and the base_class_name is the name of the base class.
Public Inheritance: The public members of the base class remain public even in the derived class; the same applies to the protected members.
Private Inheritance: This makes public members and protected members from the base class to be protected in the derived class. The derived class has no access to the base class's private members.
Protected Inheritance protects the Public and protected members from the derived class's base class.
Inheritance makes the programming more efficient and is used because of the benefits it provides. The most important usages of inheritance are discussed below:
- Code reusability: One of the main reasons to use inheritance is that you can reuse the code. For example, consider a group of animals as separate classes - Tiger, Lion, and Panther. For these classes, you can create member functions like the predator() as they all are predators, canine() as they all have canine teeth to hunt, and claws() as all the three animals have big and sharp claws. Now, since all the three functions are the same for these classes, making separate functions for all of them will cause data redundancy and can increase the chances of error. So instead of this, you can use inheritance here. You can create a base class named carnivores and add these functions to it and inherit these functions to the tiger, lion, and panther classes.
- Transitive nature: Inheritance is also used because of its transitive nature. For example, you have a derived class mammal that inherits its properties from the base class animal. Now, because of the transitive nature of the inheritance, all the child classes of ‘mammal’ will inherit the properties of the class ‘animal’ as well. This helps in debugging to a great extent. You can remove the bugs from your base class and all the inherited classes will automatically get debugged.
Preparing Your Blockchain Career for 2024
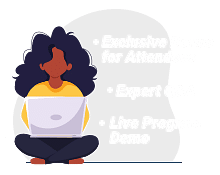
The visibility mode specifies how the features of the base class will be inherited by the derived class. There are three types of visibility modes for all types of Inheritance in C++:
Public Visibility Mode:
In the public visibility mode, it retains the accessibility of all the members of the base class. The members specified as public, protected, and private in the base class remain public, protected, and private respectively in the derived class as well. So, the public members are accessible by the derived class and all other classes. The protected members are accessible only inside the derived class and its members. However, the private members are not accessible to the derived class.
The following code snippet illustrates how to apply the public visibility mode in a derived class:
class base_class_1
// class definition
class derived_class: public base_class_1
The following code displays the working of public visibility mode with all three access specifiers of the base class:
class base_class
//class member
int base_private;
int base_protected;
int base_public;
class derived_class : public base_class
int derived_private;
// int base_private;
int derived_protected;
// int base_protected;
int derived_public;
// int base_public;
// Accessing members of base_class using object of the //derived_class:
derived_class obj;
obj.base_private; // Not accessible
obj.base_protected; // Not accessible
obj.base_public; // Accessible
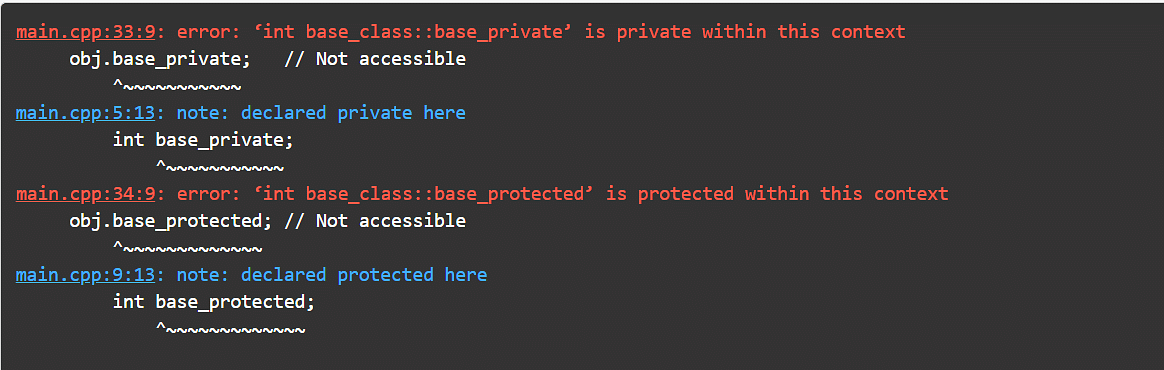
In the above example, the derived class inherits the base class as public. The private members are not accessible at all by the derived class. The protected members are only accessible inside the derived class and not accessible outside the class. And the public members are accessible inside and outside the class.
Private Visibility Mode:
In the private visibility mode, all the members of the base class become private in the derived class. This restricts the access of these members outside the derived class. They can only be accessed by the member functions of the derived class. And in this case, the derived class does not inherit the private members.
The following code snippet illustrates how to apply the private visibility mode in a derived class:
class derived_class: private base_class_1
The following code displays the working of private visibility mode with all three access specifiers of the base class:
int base_private;
class derived_class : private base_class
// int base_public
// Accessing members of base_class using object of the derived_class:
obj.base_public; // Not Accessible
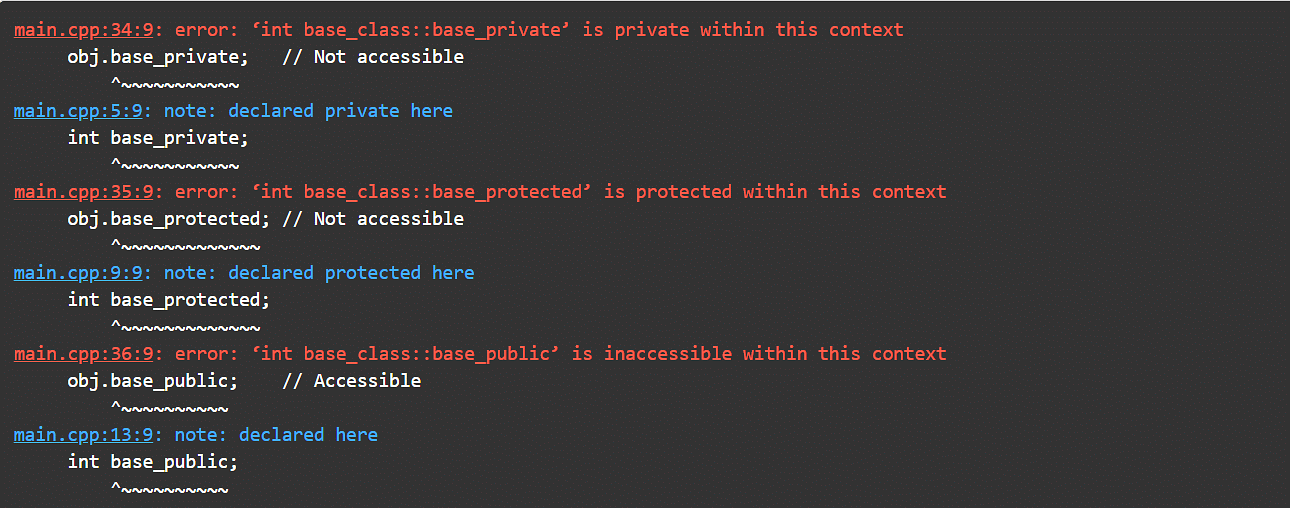
In the above example, the derived class inherits the base class privately. So, all the members of the base class have become private in the derived class. The error is thrown when the object of the derived class tries to access these members outside the class.
Protected Visibility Mode:
In the protected visibility mode, all the members of the base class become protected members of the derived class. These members are now only accessible by the derived class and its member functions . These members can also be inherited and will be accessible to the inherited subclasses. However, objects of the derived classes cannot access these members outside the class.
The following code snippet illustrates how to apply the protected visibility mode in a derived class:
class derived_class: protected base_class_1
The following code displays the working of protected visibility mode with all three access specifiers of the base class:
class derived_class : protected base_class
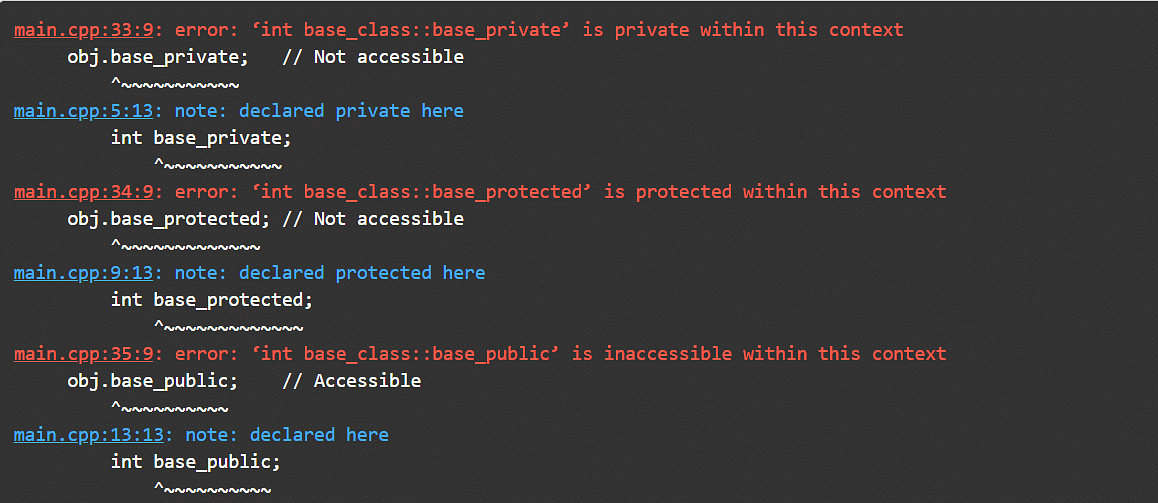
In the above example, the derived class inherits the base class in protected mode. All the members of the base class are now only accessible inside the derived class and not anywhere outside the class. So it throws an error when the object obj of the derived class tries to access these members outside the class.
The following table illustrates the control of the derived classes over the members of the base class in different visibility modes:
PUBLIC | Public | Protected | Private |
PROTECTED | Protected | Protected | Private |
PRIVATE | Not Inherited / Remains Private | Not Inherited / Remains Private | Not Inherited / Remains Private |
There are five types of inheritance in C++ based upon how the derived class inherits its features from the base class. These five types are as follows:
Single Inheritance is the most primitive among all the types of inheritance in C++. In this inheritance, a single class inherits the properties of a base class. All the data members of the base class are accessed by the derived class according to the visibility mode (i.e., private, protected, and public) that is specified during the inheritance.
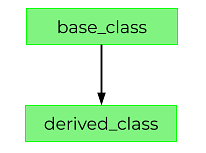
class derived_class: visibility_mode base_class_1
Description
A single derived_class inherits a single base_class. The visibility_mode is specified while declaring the derived class to specify the control of base class members within the derived class.
The following example illustrates Single Inheritance in C++:
#include <iostream>
using namespace std;
// base class
class electronicDevice
// constructor of the base class
electronicDevice()
cout << "I am an electronic device.\n\n";
// derived class
class Computer: public electronicDevice
// constructor of the derived class
Computer()
cout << "I am a computer.\n\n";
// create object of the derived class
Computer obj; // constructor of base class and
// derived class will be called
return 0;
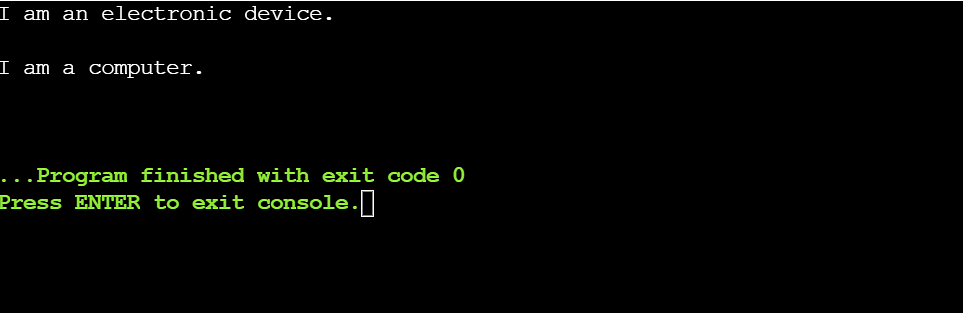
In the above example, the subclass Computer inherits the base class electronicDevice in a public mode. So, all the public and protected member functions and data members of the class electronicDevice are directly accessible to the class Computer. Since there is a single derived class inheriting a single base class, this is Single Inheritance.
The inheritance in which a class can inherit or derive the characteristics of multiple classes, or a derived class can have over one base class, is known as Multiple Inheritance. It specifies access specifiers separately for all the base classes at the time of inheritance. The derived class can derive the joint features of all these classes and the data members of all the base classes are accessed by the derived or child class according to the access specifiers.
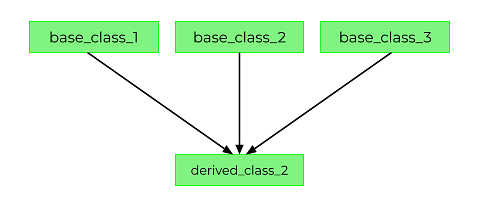
class base_class_2
class derived_class: visibility_mode_1 base_class_1, visibility_mode_2 base_class_2
Description
The derived_class inherits the characteristics of two base classes, base_class_1 and base_class_2. The visibility_mode is specified for each base class while declaring a derived class. These modes can be different for every base class.
The following example illustrates Multiple Inheritance in C++:
public:
// constructor of the base class 1
electronicDevice()
cout << "I am an electronic device.\n\n";
// class_B
class Computer
// constructor of the base class 2
Computer()
cout << "I am a computer.\n\n";
// class_C inheriting class_A and class_B
class Linux_based : public electronicDevice, public Computer
Linux_based obj; // constructor of base class A,
// base class B and derived class
// will be called
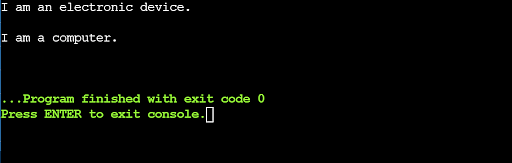
In the above example, there are separate base classes, electronicDevice, and Computer. The derived class Linux_based inherits both of these classes forming a Multiple Inheritance structure. The derived class Linux_based has inherited the attributes of both base classes in public mode. When you create an object of the derived class, it calls the constructor of both the base classes.
The inheritance in which a class can be derived from another derived class is known as Multilevel Inheritance. Suppose there are three classes A, B, and C. A is the base class that derives from class B. So, B is the derived class of A. Now, C is the class that is derived from class B. This makes class B, the base class for class C but is the derived class of class A. This scenario is known as the Multilevel Inheritance. The data members of each respective base class are accessed by their respective derived classes according to the specified visibility modes.
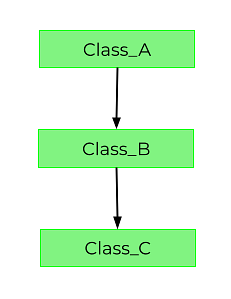
class class_A
class class_B: visibility_mode class_A
class class_C: visibility_mode class_B
The class_A is inherited by the sub-class class_B. The class_B is inherited by the subclass class_C. A subclass inherits a single class in each succeeding level.
The following example illustrates Multilevel Inheritance in C++:
// class_B inheriting class_A
// class_C inheriting class_B
class Linux_based : public Computer
// constructor of the derived class
Linux_based()
cout << "I run on Linux.\n\n";;
// create object of the derived class
Linux_based obj; // constructor of base class 1,
// base class 2, derived class will be called
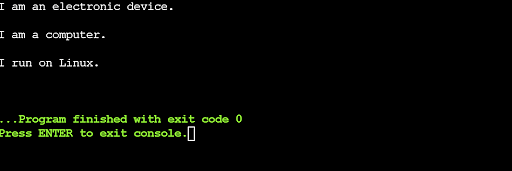
In the above example, the base class electronicDevice is inherited by the subclass Computer which is further inherited by the subclass Linux_based. Since one class is inherited by a single class at each level, it is Multilevel Inheritance. The object of the derived class Linux_based can access the members of the class electronicDevice and Computer directly.
The inheritance in which a single base class inherits multiple derived classes is known as the Hierarchical Inheritance. This inheritance has a tree-like structure since every class acts as a base class for one or more child classes. The visibility mode for each derived class is specified separately during the inheritance and it accesses the data members accordingly.
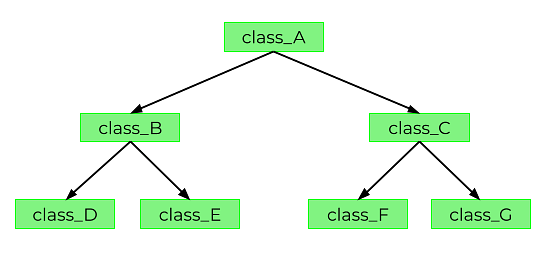
class class_C : visibility_mode class_A
class class_D: visibility_mode class_B
class class_E: visibility_mode class_C
The subclasses class_B and class_C inherit the attributes of the base class class_A. Further, these two subclasses are inherited by other subclasses class_D and class_E respectively.
The following example illustrates Hierarchical Inheritance in C++:
// base class
// constructor of the base class 1
// derived class inheriting base class
class Linux_based : public electronicDevice
// create object of the derived classes
Computer obj1; // constructor of base class will be called
Linux_based obj2; // constructor of base class will be called
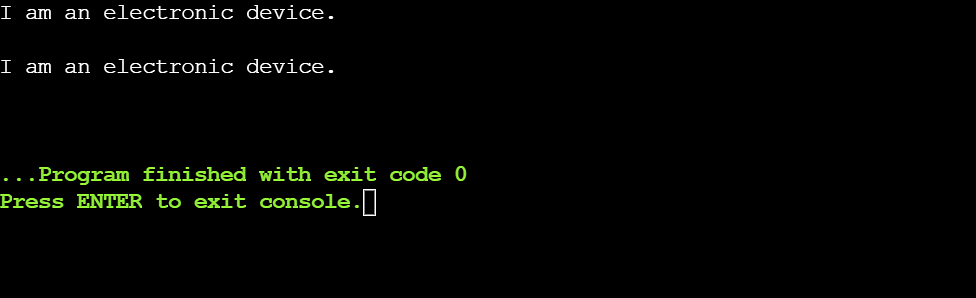
In the above example, the base class electronicDevice is inherited by two subclasses Computer and Linux_based. The class structure represents Hierarchical Inheritance. Both the derived classes can access the public members of the base class electronicDevice. When it creates objects of these two derived classes, it calls the constructor of the base class for both objects.
Hybrid Inheritance , as the name suggests, is the combination of two or over two types of inheritances. For example, the classes in a program are in such an arrangement that they show both single inheritance and hierarchical inheritance at the same time. Such an arrangement is known as the Hybrid Inheritance. This is arguably the most complex inheritance among all the types of inheritance in C++. The data members of the base class will be accessed according to the specified visibility mode.
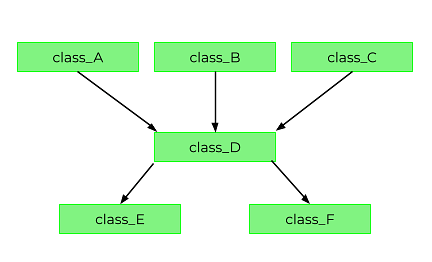
class class_B
class class_C: visibility_mode class_A, visibility_mode class_B
class class_D: visibility_mode class_C
The derived class class_C inherits two base classes that are, class_A and class_B. This is the structure of Multiple Inheritance. And two subclasses class_D and class_E, further inherit class_C. This is the structure of Hierarchical Inheritance. The overall structure of Hybrid Inheritance includes more than one type of inheritance.
The following example illustrates the Hybrid Inheritance in C++:
// base class 1
// base class 2
// constructor of the base class 2
// derived class 1 inheriting base class 1 and base class 2
// derived class 2 inheriting derived class 1
class Debian: public Linux_based
// create an object of the derived class
Debian obj; // constructor of base classes and
// derived class will be called
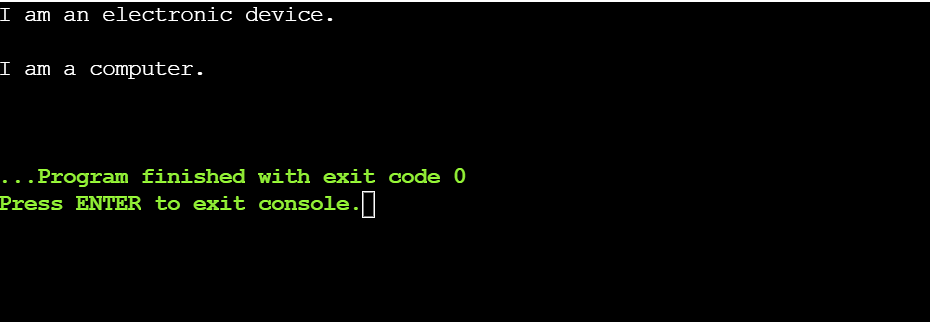
In the above example, the three classes electronicDevice, Computer, and Linux_based form the structure of Multiple Inheritance. And the class Debian inherits the class Linux_base forming the structure of Single Inheritance. When an object of the derived class Debian is created, the constructors of all its superclasses are called.
Become a Full Stack Developer in 6 Months!
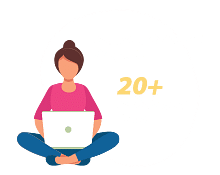
The diamond problem in inheritance happens when there is a derived class inheriting the attributes of 2 superclasses, and these superclasses have a common base class. The following diagram represents the structure of a diamond problem.
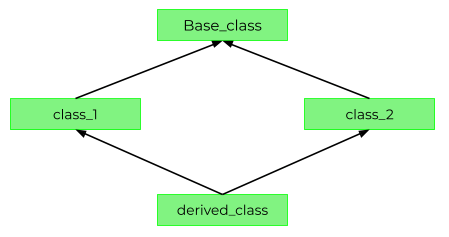
In a diamond problem, when the two classes class_1 and class_2 inherit the same base class, it creates two copies of the Base_class. So when an object of the derived_class accesses a member of the base class, it causes ambiguity. This ambiguous situation is caused because it is unclear which copy of the base_class member needs to be accessed. And in such a situation the compiler throws an error.
The following example illustrates the ambiguous situation caused by a diamond structured inheritance.
class Base_class
int x;
class class_1 : public Base_class
class class_2 : public Base_class
// derived class 3
class derived_class : public class_1, public class_2
int sum;
// create an object of the derived_class
obj.x = 10; // ambiguous
obj.y = 20;
obj.z = 30;
obj.sum = obj.x + obj.y + obj.z;
cout << "The sum is: " << obj.sum << "\n\n";
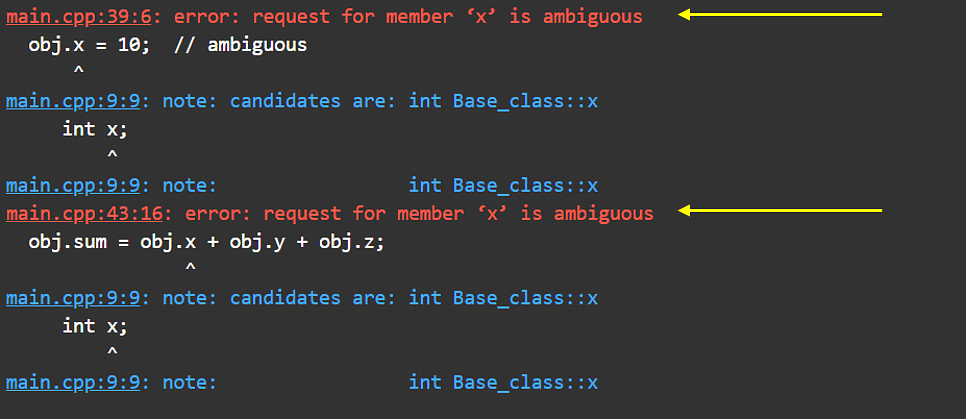
There are two ways to avoid the ambiguous situation in a diamond problem.
- Using the scope resolution operator.
- Using virtual base class keyword.
The following example illustrates the working of the scope resolution operator to remove ambiguity in the diamond problem.
int x;
obj.class_1::x = 10; // it is now unambiguous
obj.sum = obj.class_1::x + obj.y + obj.z;
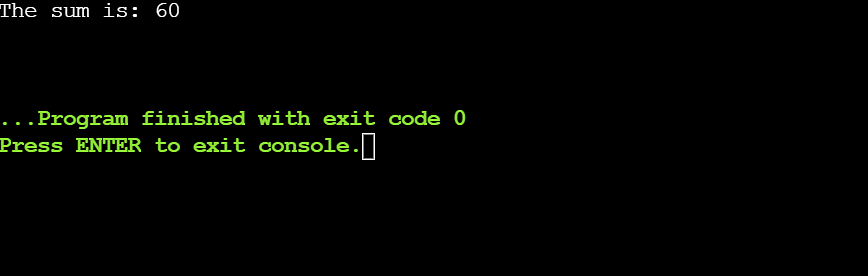
In the above example, the following expression uses the scope resolution operator to specify which copy of “x” is to be used.
obj.class_1::x;
Here, the class_1’s version of “x” is accessed. In this case, no error is thrown as the ambiguous statement has been resolved to become unambiguous.
Although the scope resolution operator removes the ambiguity and produces correct output, there are still two copies of the base class. If you require only one copy of the base class, then there is the virtual keyword for this. The virtual keyword allows only one copy of the base class to be created, and the object of the derived class can access the members of the base class in the usual way.
The following example illustrates the working of the virtual keyword to remove ambiguity in the diamond problem.
class class_1 : virtual public Base_class
class class_2 : virtual public Base_class
derived_class obj;
obj.x = 10; // it is now unambiguous
return 0;
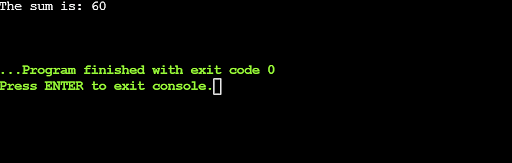
In the above example, two classes class_1 and class_2 inherit the base class as virtual. Any Multiple Inheritance involving these subclasses creates only a single copy of the base_class. So, now the derived_class has only one copy of the base_class, which makes the following statement valid and unambiguous.
obj.x = 10;
In inheritance, the private members of a base class are not inherited by the derived classes. So these members of the base class are not accessible to the objects of the derived class. Only the public and the protected members are inherited and can be accessed by the derived classes.
The private members of the base class can be made inheritable in two ways:
Modifying the Visibility Mode From Private to Public.
Making the access modifier of the private member public makes it inheritable by the derived classes. However, a problem arises with this approach. The data hiding property is no longer there for that member as it is now accessible to all other functions of the program.
Modifying the Visibility Mode From Private to Protected.
This approach retains the data hiding property of the private member. Modifying the access specifier of a private member as protected, makes it inheritable and accessible by the derived class. If these members are required to be inheritable further beyond the immediately derived class, then they should be inherited as public. Otherwise, they should be inherited as private which will end the inheritance hierarchy beyond the immediately derived class.
Here's How to Land a Top Software Developer Job
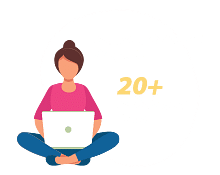
Reusability of Code
In C++, inheritance enables you to build new classes based on existing ones, allowing you to reuse code and avoid writing the same function from scratch. As a result, developing new applications may save you a lot of time and effort.
Overriding
The ability to alter functions in a derived class in C++ allows you to modify a program's behavior without changing the source code. This is an excellent method for modifying existing code to your needs.
Polymorphism
Through inheritance, you can build objects that can take various forms; it depends on the parent class from which they inherit; this is called polymorphism . This helps the flexibility and responsiveness of the code to the changing needs.
Organization
In C++, inheritance aids in your code's logical and hierarchical organization. By establishing classes that derive from other classes, you can construct a structure of related classes that allows you to comprehend how your code works more readily.
Base Class: Parent class is another name for a base class. A base class is a pre-existing class which inherits its properties from other classes. All base class members are present in the class that inherits it, and it can also add some new properties.
Derived Class: Child class is another name for the derived class. This class is derived from pre-existing classes. The derived class inherits functions from a base class. The derived class can acquire the operations from the base class with some additional properties.
Access control is used to hide data in Object oriented programming. Access controls allow you to distinguish between a class's public interface and its protected elements, which are only accessible to derived classes. A derived class has access to its base class's non-private members. Subsequently, base-class features not available to derived class member functions should be made private in the base class.
Publicly Derived Class: When a class is derived from a public base class, the members of base class become public members of the derived class, and the base class's protected members become protected members of the derived class. The private members from the base class are not directly available from a derived class.
Privately Derived Class: When a class is generated by private inheritance, its base class's Public and protected elements are converted into private members. This implies that the derived Object's public interface does not inherit the base class's methods.
The features of a base class can be privately derived, publicly derived or protected depending on the visibility mode specified in the specification of the derived class. Depending on the visibility mode, the public or private inheritance of the base class's features is allowed. The visibility modes control the access specifier for inheritable base-class elements in the derived class.
Public Visibility Mode: The characteristics of the base class have the least privacy under the Public Visibility mode. The derived class can access the Public and protected members of the base class but not the private members if the visibility mode is set to Public.
Private Visibility Mode: The setting provides the most privacy for the base class attributes. If the visibility mode is set to private, the derived class can secretly access the Public and protected members of a base class.
Protected Visibility Mode: Between public and private visibility modes comes protected visibility mode. The derived class can access the protected and public members of the base class protectively when the visibility mode is protected.
This table compares various courses offered by Simplilearn, based on several key features and details. The table provides an overview of the courses' duration, skills you will learn, additional benefits, among other important factors, to help learners make an informed decision about which course best suits their needs.
Program Name Full Stack Java Developer Automation Testing Masters Program Geo IN All University Simplilearn Simplilearn Course Duration 11 Months 11 Months Coding Experience Required Basic Knowledge Basic Knowledge Skills You Will Learn 15+ Skills Including Core Java, SQL, AWS, ReactJS, etc. Java, AWS, API Testing, TDD, etc. Additional Benefits Interview Preparation Exclusive Job Portal 200+ Hiring Partners Structured Guidance Learn From Experts Hands-on Training Cost $$ $$ Explore Program Explore Program
In this comprehensive guide on the types of inheritance in C++, you started with a brief introduction to C++ and the concept of parent and child classes. You understood why and when to use which types of inheritance in C++ along with the different visibility modes that can be used with the classes and members.
You understood the five different types of inheritance, their use-cases, examples, and the fundamental differences between them. You looked at a very common problem that arises due to multiple inheritances called the diamond problem and the solution to it. Finally, you saw how to make a private member inheritable.
If you want to learn more about such concepts of C++ with examples, you can check out our guide on C++ for beginners .
Although learning inheritance in C++ is essential for creating intricate programs, expanding your programming knowledge to encompass many languages and frameworks will greatly improve your chances of landing a good job. A java full stack developer course can provide you the ability to work on both the client and server sides of an application, making you a versatile asset in the tech business, for those wishing to expand their experience beyond C++ and dive into web development.
If you want to land your foot in Full Stack Web Development, you should check out Simplilearn’s comprehensive training program on Full Stack Java Developer. This program will help you to learn top technical skills like DevOps, Agile, HTML, CSS, Servlets, JS, Java, and its libraries such as Spring, Hibernate, JPA, etc. This course is led by top industry experts and they are available throughout the course to help you solve your queries.
If you have any queries related to our article on “Types of inheritance in C++” or any other suggestion, please feel free to drop a comment on the comment box. Our experts will get back to you as soon as possible.
Happy Learning!
Our Software Development Courses Duration And Fees
Software Development Course typically range from a few weeks to several months, with fees varying based on program and institution.
Program Name | Duration | Fees |
---|---|---|
Cohort Starts: | 4 Months | € 2,499 |
Cohort Starts: | 7 months | € 1,500 |
Cohort Starts: | 6 Months | € 1,500 |
Cohort Starts: | 8 months | € 1,099 |
Recommended Reads
Free eBook: Salesforce Developer Salary Report
Understanding the Essentials of Multiple Inheritance in C++
What is Inheritance in Java and How to Implement It
Free eBook: Guide to Mobile App Development
Understanding Inheritance In C++
C++ Object-Oriented Programming : The Best Way to Learn C++ Oops
Get Affiliated Certifications with Live Class programs
Full stack java developer masters program.
- Kickstart Full Stack Java Developer career with industry-aligned curriculum by experts
- Hands-on practice through 20+ projects, assessments, and tests
Full Stack Web Developer - MEAN Stack
- Comprehensive Blended Learning program
- 8X higher interaction in live online classes conducted by industry experts
- PMP, PMI, PMBOK, CAPM, PgMP, PfMP, ACP, PBA, RMP, SP, and OPM3 are registered marks of the Project Management Institute, Inc.
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
C Exercises – Practice Questions with Solutions for C Programming
The best way to learn C programming language is by hands-on practice. This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more.
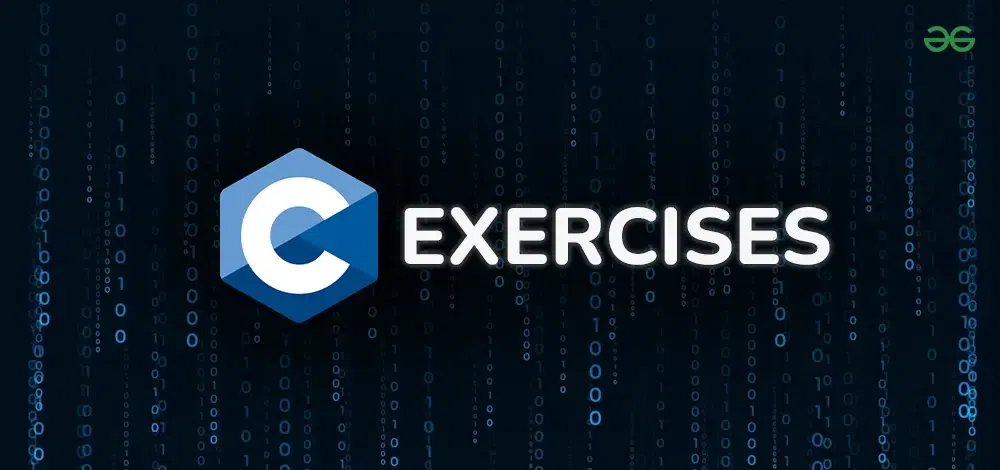
So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
C Programming Exercises
The following are the top 30 programming exercises with solutions to help you practice online and improve your coding efficiency in the C language. You can solve these questions online in GeeksforGeeks IDE.
Q1: Write a Program to Print “Hello World!” on the Console.
In this problem, you have to write a simple program that prints “Hello World!” on the console screen.
For Example,
Click here to view the solution.
Q2: write a program to find the sum of two numbers entered by the user..
In this problem, you have to write a program that adds two numbers and prints their sum on the console screen.
Q3: Write a Program to find the size of int, float, double, and char.
In this problem, you have to write a program to print the size of the variable.
Q4: Write a Program to Swap the values of two variables.
In this problem, you have to write a program that swaps the values of two variables that are entered by the user.
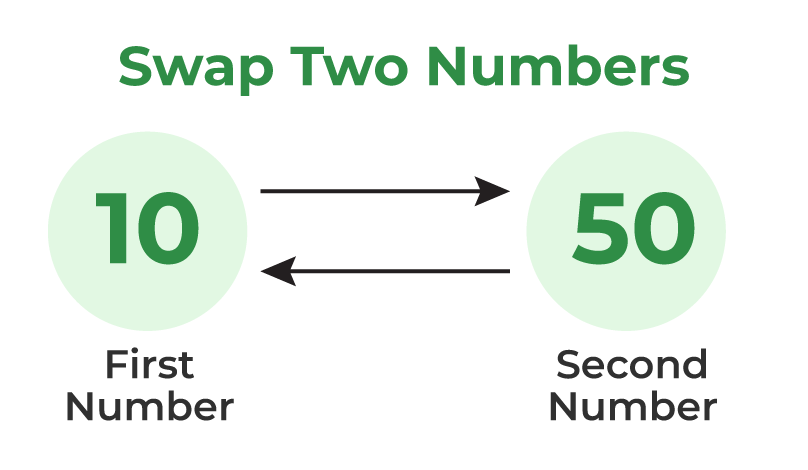
Swap two numbers
Q5: Write a Program to calculate Compound Interest.
In this problem, you have to write a program that takes principal, time, and rate as user input and calculates the compound interest.
Q6: Write a Program to check if the given number is Even or Odd.
In this problem, you have to write a program to check whether the given number is even or odd.
Q7: Write a Program to find the largest number among three numbers.
In this problem, you have to write a program to take three numbers from the user as input and print the largest number among them.
Q8: Write a Program to make a simple calculator.
In this problem, you have to write a program to make a simple calculator that accepts two operands and an operator to perform the calculation and prints the result.
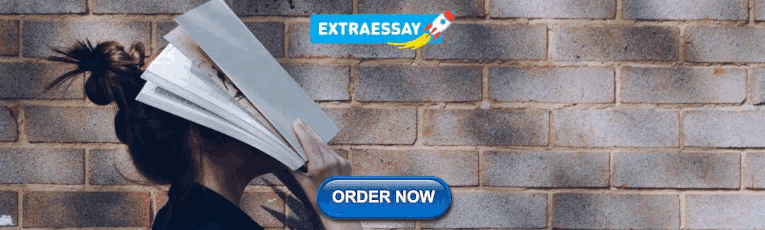
Q9: Write a Program to find the factorial of a given number.
In this problem, you have to write a program to calculate the factorial (product of all the natural numbers less than or equal to the given number n) of a number entered by the user.
Q10: Write a Program to Convert Binary to Decimal.
In this problem, you have to write a program to convert the given binary number entered by the user into an equivalent decimal number.
Q11: Write a Program to print the Fibonacci series using recursion.
In this problem, you have to write a program to print the Fibonacci series(the sequence where each number is the sum of the previous two numbers of the sequence) till the number entered by the user using recursion.
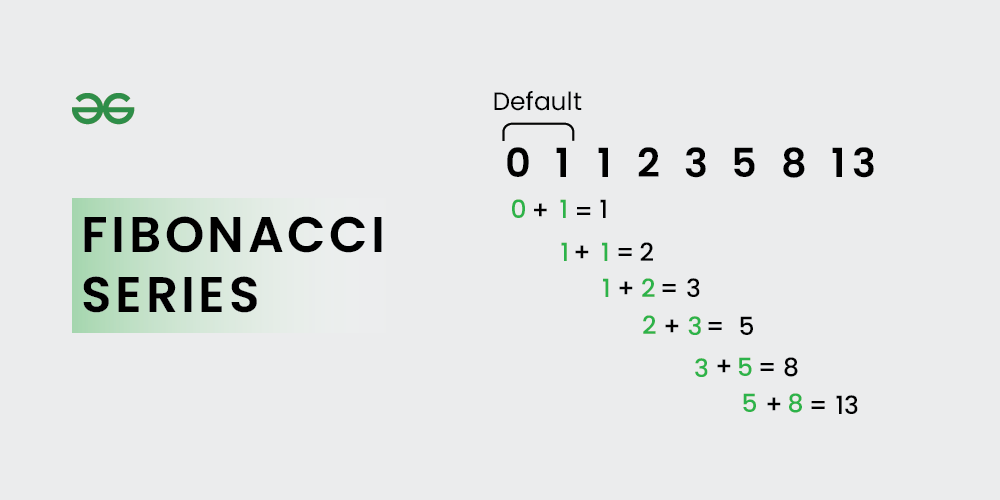
Fibonacci Series
Q12: Write a Program to Calculate the Sum of Natural Numbers using recursion.
In this problem, you have to write a program to calculate the sum of natural numbers up to a given number n.
Q13: Write a Program to find the maximum and minimum of an Array.
In this problem, you have to write a program to find the maximum and the minimum element of the array of size N given by the user.
Q14: Write a Program to Reverse an Array.
In this problem, you have to write a program to reverse an array of size n entered by the user. Reversing an array means changing the order of elements so that the first element becomes the last element and the second element becomes the second last element and so on.
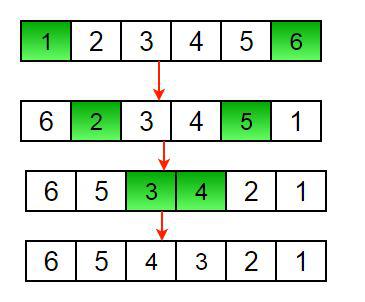
Reverse an array
Q15: Write a Program to rotate the array to the left.
In this problem, you have to write a program that takes an array arr[] of size N from the user and rotates the array to the left (counter-clockwise direction) by D steps, where D is a positive integer.
Q16: Write a Program to remove duplicates from the Sorted array.
In this problem, you have to write a program that takes a sorted array arr[] of size N from the user and removes the duplicate elements from the array.
Q17: Write a Program to search elements in an array (using Binary Search).
In this problem, you have to write a program that takes an array arr[] of size N and a target value to be searched by the user. Search the target value using binary search if the target value is found print its index else print ‘element is not present in array ‘.
Q18: Write a Program to reverse a linked list.
In this problem, you have to write a program that takes a pointer to the head node of a linked list, you have to reverse the linked list and print the reversed linked list.
Q18: Write a Program to create a dynamic array in C.
In this problem, you have to write a program to create an array of size n dynamically then take n elements of an array one by one by the user. Print the array elements.
Q19: Write a Program to find the Transpose of a Matrix.
In this problem, you have to write a program to find the transpose of a matrix for a given matrix A with dimensions m x n and print the transposed matrix. The transpose of a matrix is formed by interchanging its rows with columns.
Q20: Write a Program to concatenate two strings.
In this problem, you have to write a program to read two strings str1 and str2 entered by the user and concatenate these two strings. Print the concatenated string.
Q21: Write a Program to check if the given string is a palindrome string or not.
In this problem, you have to write a program to read a string str entered by the user and check whether the string is palindrome or not. If the str is palindrome print ‘str is a palindrome’ else print ‘str is not a palindrome’. A string is said to be palindrome if the reverse of the string is the same as the string.
Q22: Write a program to print the first letter of each word.
In this problem, you have to write a simple program to read a string str entered by the user and print the first letter of each word in a string.
Q23: Write a program to reverse a string using recursion
In this problem, you have to write a program to read a string str entered by the user, and reverse that string means changing the order of characters in the string so that the last character becomes the first character of the string using recursion.
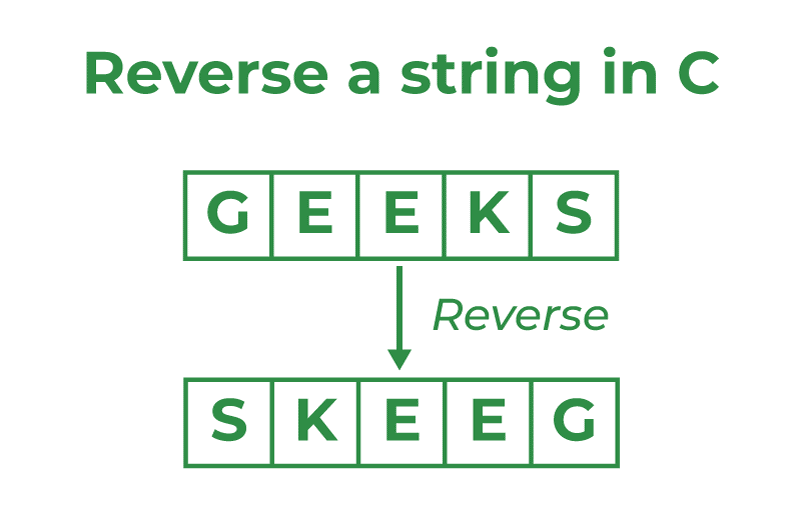
reverse a string
Q24: Write a program to Print Half half-pyramid pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print the half-pyramid pattern of numbers. Half pyramid pattern looks like a right-angle triangle of numbers having a hypotenuse on the right side.
Q25: Write a program to print Pascal’s triangle pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print Pascal’s triangle pattern. Pascal’s Triangle is a pattern in which the first row has a single number 1 all rows begin and end with the number 1. The numbers in between are obtained by adding the two numbers directly above them in the previous row.
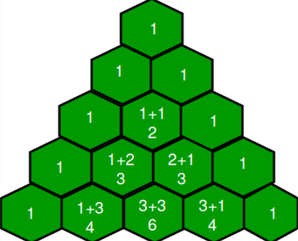
Pascal’s Triangle
Q26: Write a program to sort an array using Insertion Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending or descending order using insertion sort.
Q27: Write a program to sort an array using Quick Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending order using quick sort.
Q28: Write a program to sort an array of strings.
In this problem, you have to write a program that reads an array of strings in which all characters are of the same case entered by the user and sort them alphabetically.
Q29: Write a program to copy the contents of one file to another file.
In this problem, you have to write a program that takes user input to enter the filenames for reading and writing. Read the contents of one file and copy the content to another file. If the file specified for reading does not exist or cannot be opened, display an error message “Cannot open file: file_name” and terminate the program else print “Content copied to file_name”
Q30: Write a program to store information on students using structure.
In this problem, you have to write a program that stores information about students using structure. The program should create various structures, each representing a student’s record. Initialize the records with sample data having data members’ Names, Roll Numbers, Ages, and Total Marks. Print the information for each student.
We hope after completing these C exercises you have gained a better understanding of C concepts. Learning C language is made easier with this exercise sheet as it helps you practice all major C concepts. Solving these C exercise questions will take you a step closer to becoming a C programmer.
Frequently Asked Questions (FAQs)
Q1. what are some common mistakes to avoid while doing c programming exercises.
Some of the most common mistakes made by beginners doing C programming exercises can include missing semicolons, bad logic loops, uninitialized pointers, and forgotten memory frees etc.
Q2. What are the best practices for beginners starting with C programming exercises?
Best practices for beginners starting with C programming exercises: Start with easy codes Practice consistently Be creative Think before you code Learn from mistakes Repeat!
Q3. How do I debug common errors in C programming exercises?
You can use the following methods to debug a code in C programming exercises Read the error message carefully Read code line by line Try isolating the error code Look for Missing elements, loops, pointers, etc Check error online
Please Login to comment...
- OpenAI o1 AI Model Launched: Explore o1-Preview, o1-Mini, Pricing & Comparison
- How to Merge Cells in Google Sheets: Step by Step Guide
- How to Lock Cells in Google Sheets : Step by Step Guide
- PS5 Pro Launched: Controller, Price, Specs & Features, How to Pre-Order, and More
- #geekstreak2024 – 21 Days POTD Challenge Powered By Deutsche Bank
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, introduction to c++.
- Getting Started With C++
- Your First C++ Program
- C++ Comments
C++ Fundamentals
- C++ Keywords and Identifiers
- C++ Variables, Literals and Constants
- C++ Data Types
- C++ Type Modifiers
- C++ Constants
- C++ Basic Input/Output
- C++ Operators
Flow Control
- C++ Relational and Logical Operators
- C++ if, if...else and Nested if...else
- C++ for Loop
- C++ while and do...while Loop
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ switch..case Statement
- C++ Ternary Operator
- C++ Functions
- C++ Programming Default Arguments
- C++ Function Overloading
- C++ Inline Functions
- C++ Recursion
Arrays and Strings
- C++ Array to Function
- C++ Multidimensional Arrays
- C++ String Class
Pointers and References
- C++ Pointers
- C++ Pointers and Arrays
- C++ References: Using Pointers
- C++ Call by Reference: Using pointers
- C++ Memory Management: new and delete
Structures and Enumerations
- C++ Structures
- C++ Structure and Function
- C++ Pointers to Structure
- C++ Enumeration
Object Oriented Programming
- C++ Classes and Objects
- C++ Constructors
- C++ Constructor Overloading
- C++ Destructors
- C++ Access Modifiers
- C++ Encapsulation
- C++ friend Function and friend Classes
Inheritance & Polymorphism
C++ Inheritance
C++ Public, Protected and Private Inheritance
- C++ Multiple, Multilevel and Hierarchical Inheritance
- C++ Function Overriding
- C++ Virtual Functions
C++ Abstract Class and Pure Virtual Function
STL - Vector, Queue & Stack
- C++ Standard Template Library
- C++ STL Containers
- C++ std::array
- C++ Vectors
- C++ Forward List
- C++ Priority Queue
STL - Map & Set
- C++ Multimap
- C++ Multiset
- C++ Unordered Map
- C++ Unordered Set
- C++ Unordered Multiset
- C++ Unordered Multimap
STL - Iterators & Algorithms
- C++ Iterators
- C++ Algorithm
- C++ Functor
Additional Topics
- C++ Exceptions Handling
- C++ File Handling
- C++ Ranged for Loop
- C++ Nested Loop
- C++ Function Template
- C++ Class Templates
- C++ Type Conversion
- C++ Type Conversion Operators
- C++ Operator Overloading
Advanced Topics
- C++ Namespaces
- C++ Preprocessors and Macros
- C++ Storage Class
- C++ Bitwise Operators
- C++ Buffers
- C++ istream
- C++ ostream
C++ Tutorials
C++ Shadowing Base Class Member Function
- C++ Polymorphism
- C++ Virtual Functions and Function Overriding
C++ Multiple, Multilevel, Hierarchical and Virtual Inheritance
Inheritance is one of the core features of an object-oriented programming language. It allows software developers to derive a new class from the existing class. The derived class inherits the features of the base class (existing class).
There are various models of inheritance in C++ programming.
- C++ Multilevel Inheritance
In C++ programming, not only can you derive a class from the base class but you can also derive a class from the derived class. This form of inheritance is known as multilevel inheritance.
Here, class B is derived from the base class A and the class C is derived from the derived class B .
- Example 1: C++ Multilevel Inheritance
In this program, class C is derived from class B (which is derived from base class A ).
The obj object of class C is defined in the main() function.
When the display() function is called, display() in class A is executed. It's because there is no display() function in class C and class B .
The compiler first looks for the display() function in class C . Since the function doesn't exist there, it looks for the function in class B (as C is derived from B ).
The function also doesn't exist in class B , so the compiler looks for it in class A (as B is derived from A ).
If display() function exists in C , the compiler overrides display() of class A (because of member function overriding ).
- C++ Multiple Inheritance
In C++ programming, a class can be derived from more than one parent. For example, A class Bat is derived from base classes Mammal and WingedAnimal . It makes sense because bat is a mammal as well as a winged animal.
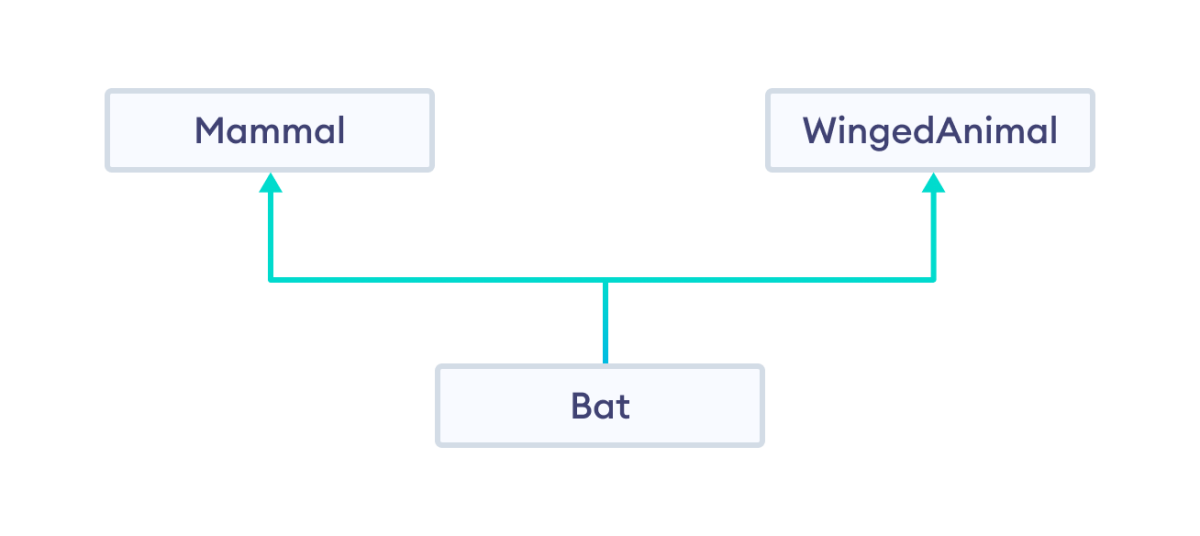
- Example 2: Multiple Inheritance in C++ Programming
- Ambiguity in Multiple Inheritance
The most obvious problem with multiple inheritance occurs during function overriding.
Suppose two base classes have the same function which is not overridden in the derived class.
If you try to call the function using the object of the derived class, the compiler shows error. It's because the compiler doesn't know which function to call. For example,
This problem can be solved using the scope resolution function to specify which function to class either base1 or base2 .
- C++ Hierarchical Inheritance
If more than one class is inherited from the base class, it's known as hierarchical inheritance . In hierarchical inheritance, all features that are common in child classes are included in the base class.
For example, Physics, Chemistry, Biology are derived from Science class. Similarly, Dog, Cat, Horse are derived from Animal class.
Syntax of Hierarchical Inheritance
- Example 3: Hierarchical Inheritance in C++ Programming
Here, both the Dog and Cat classes are derived from the Animal class. As such, both the derived classes can access the info() function belonging to the Animal class.
- C++ Virtual Inheritance
Virtual inheritance is a C++ technique that makes sure that the grandchild derived classes inherit only one copy of a base class's member variables.
Let's consider the following class hierarchy.
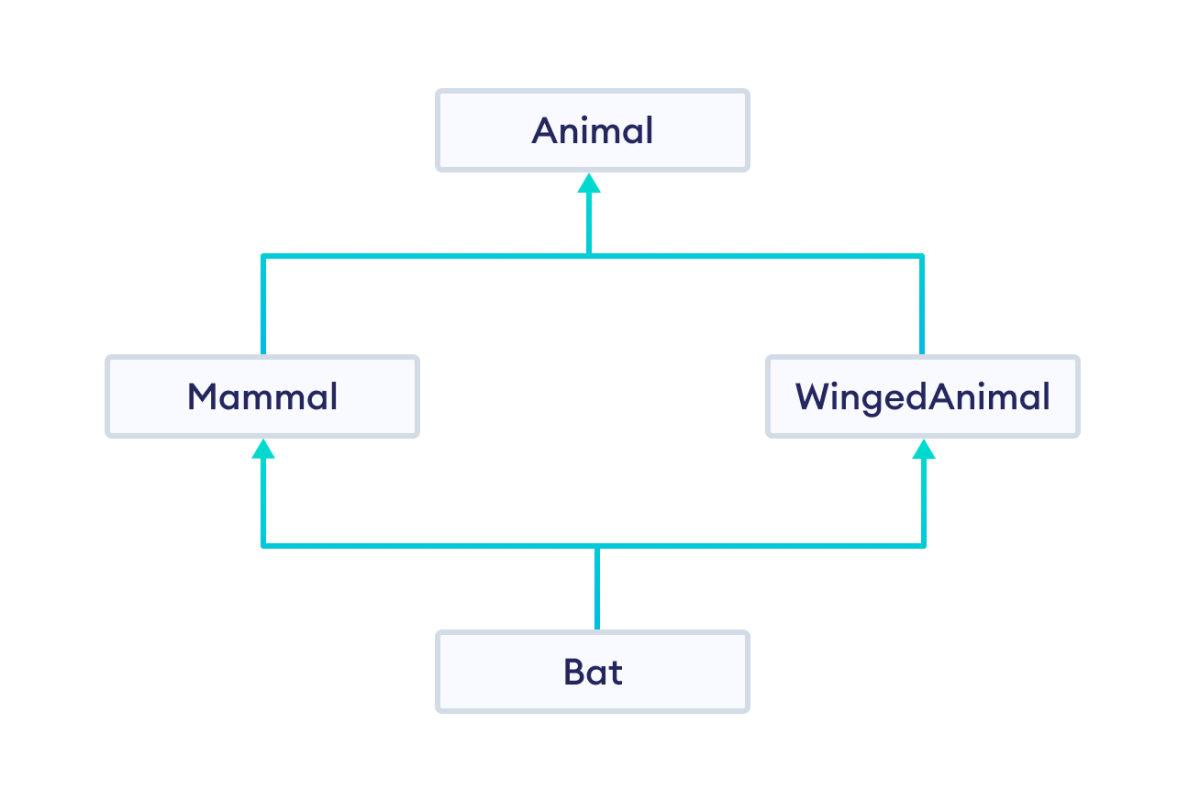
Here, when Bat inherits from multiple classes; Mammal and WingedAnimal having the same base class; Animal , it may inherit multiple instances of the base class. This is known as the diamond problem.
We can avoid this problem using virtual inheritance.
Here, Derived1 and Derived2 both inherit from Base virtually, ensuring that Derived3 will have only one set of Base member variables, even though it inherits from both Derived1 and Derived2 .
- Example 4: Virtual Inheritance
In this example, the Bat class is derived from both WingedAnimal and Mammal , which are in turn derived from Animal using virtual inheritance.
This ensures that only one Animal base class constructor is called when an instance of Bat is created and the species_name is set to "Bat" .
Note : In the above program, if the virtual keyword was not used, the Bat class would inherit multiple copies of the member variable of the Animal class. This would result in an error.
Table of Contents
- Introduction
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
C++ Tutorial
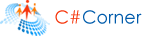
- TECHNOLOGIES
- An Interview Question

11 Code Excercises on Inheritance in C# .NET

- Prabhat Kumar
- Feb 12, 2023
- Other Artcile
This article explains the various behaviors of inheritance in C# and .NET.
Introduction This article explains the various behaviors of inheritance in OOP in various cases. Inheritance is creating classes that inherit certain aspects from parent classes. If you are not familiar with object-oriented programming, please read A Complete Guide To Object Oriented Programming In C# . Objective To understand inheritance and its behavior in various cases and to understand keywords like virtual, override, new and abstract. CASE 1: What will happen when the virtual keyword is used with a method without implementation? For example:
RESULT Error 1 'ConsoleApplication.A.Show()' must declare a body because it is not marked abstract, extern, or partial CASE 2 : What will happen when a method is used without implementation? For example:
Error 1 'ConsoleApplication.A.Show()' must declare a body because it is not marked abstract, extern, or partial CASE 3: What will be the output of the C# .NET code snippet given below in which the base class method is overridden by a derived class using the override keyword?
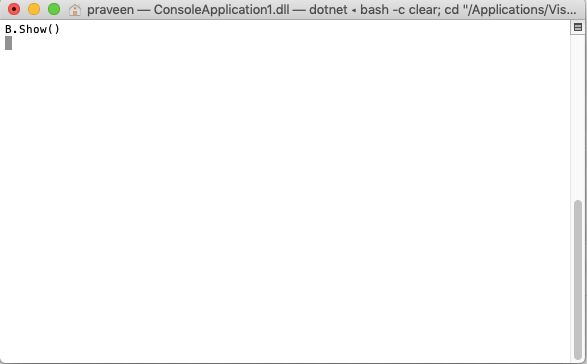
RESULT Warning 1 'ConsoleApplication.B.Show()' hides inherited member 'ConsoleApplication.A.Show()'. To make the current member override that implementation, add the override keyword. Otherwise, add the new keyword. Output: A.Show() CASE 6: What happens when the abstract method is used with a non-abstract class?
Error 1 'ConsoleApplication.A.Show()' cannot declare a body because it is marked abstract Error 2 'ConsoleApplication.A.Show()' is abstract but it is contained in non-abstract class 'ConsoleApplication.A' CASE 7: What will be the output of the C# .NET code snippet given below?
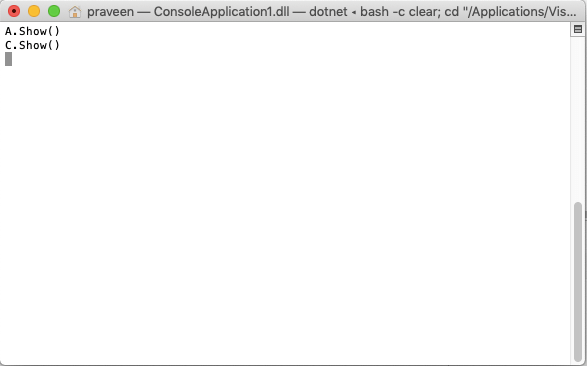
- Types of Inheritance in C#
- Multiple Inheritance in C#
- Inheritance
- Inheritance in C#
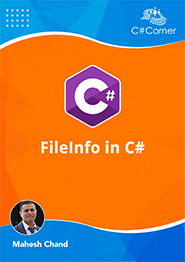
FileInfo in C#
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
C++ inheritance and assignment operator
I am using CRTP to implement a C++ feature.
Now I've faced a situation like this.
This code does not compile saying no viable overloaded=
How can I resolve the issue without adding any code to struct A and struct B ?
- inheritance
- operator-overloading
- assignment-operator
2 Answers 2
You need to create the assign operator which takes B as reference:
Short explanation why you need this (I hope I am not wrong):
The + operator returns a B reference as B is the template parameter, not C . So ignoring the + there would be an assignment C = B which is not possible. To be clear, you would not need your first assignment operator to run your code.
Maybe also this is clearer and better:
Now your assignment operator takes B as input and output.
You also have the possibility to overload the operator+ for the C class to allow the c1 + c2 code to return a C object instead of a B object (as it currently does due to the operator+ defined in the B class). In this way, you obtain a C object as a result of c1+c2 that may be assigned using the defined operator= in class C .
For instance, add this function to the C class like the following:
Beware though of the idea behind your choice as it may compile but not do what you would like it to do.
Overloading + or = may depend on what you would like to do with your code and the type you would like to obtain from the operation c1+c2 .

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c++ inheritance operator-overloading assignment-operator crtp or ask your own question .
- The Overflow Blog
- The world’s largest open-source business has plans for enhancing LLMs
- Featured on Meta
- User activation: Learnings and opportunities
- Site maintenance - Mon, Sept 16 2024, 21:00 UTC to Tue, Sept 17 2024, 2:00...
- What does a new user need in a homepage experience on Stack Overflow?
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- Is transit visa required for KSA if I am there for only 6 hours
- If one is arrested, but has a baby/pet in their house, what are they supposed to do?
- What properties of the fundamental group functor are needed to uniquely determine it upto natural isomorphism?
- Should I be careful about setting levels too high at one point in a track?
- Stuck as a solo dev
- Would a scientific theory of everything be falsifiable?
- Rocky Mountains Elevation Cutout
- Why is steaming food faster than boiling it?
- Trying to find air crash for a case study
- View undo history of Windows Explorer on Win11
- What is the oldest open math problem outside of number theory?
- 1950s comic book about bowling ball looking creatures that inhabit the underground of Earth
- Can All Truths Be Scientifically Verified?
- How to deal with coauthors who just do a lot of unnecessary work and exploration to be seen as hard-working and grab authorship?
- What’s the name of this horror movie where a girl dies and comes back to life evil?
- Copyright Fair Use: Is using the phrase "Courtesy of" legally acceptable when no permission has been given?
- тем и есть (syntax and meaning)
- The walk radical in Traditional Chinese
- Odorless color less , transparent fluid is leaking underneath my car
- How do I completly remove "removed" disks from mdadm array
- Positivity for the mild solution of a heat equation on the torus
- Is it true that before European modernity, there were no "nations"?
- security concerns of executing mariadb-dump with password over ssh
- How to use Modus Ponens in L axiomatic system
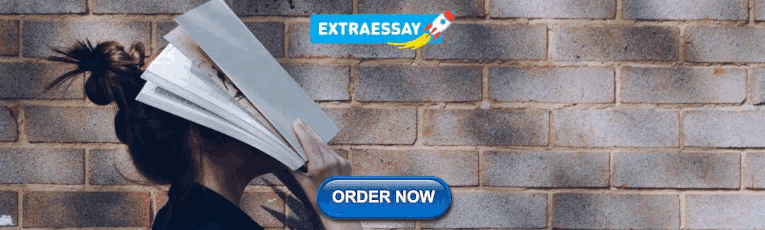
IMAGES
VIDEO
COMMENTS
C++ Inheritance programs/examples. Inheritance is a feature of object oriented programming system, by which a class can inherit the commonly used properties/features of another classes. In this section you will get solved c++ programs using inheritance: simple inheritance, multiple inheritance, multilevel inheritance, hybrid inheritance ...
Area of a rectangle is calculated as: height * width (or length * width) Submit your .cpp file. TEST DATA: For an triangle with a base of 2 and a height of 5 the area would be 5. For a rectangle with a base (length) of 5 and a base of 3 the area would be 15. This page titled 4.10: Programming Assignment- Inheritance is shared under a CC BY-SA ...
Create two classes: Cuboid The Cuboid class should have three data fields- length, width and height of int types. The class should have display() method, to print the length, width and height of
7. Yes, you can emulate inheritance in C using the "type punning" technique. That is, the declaration of the base class (struct) inside the derived class, and cast the derived as a base: struct base_class {. int x; }; struct derived_class {. struct base_class base; int y;
Exercises — Khufu: Object-Oriented Programming using C++. 10.1. Exercises. Many of these exercises are taken from past exams of ECE 244 Programming Fundamentals courses at University of Toronto. The solutions are provided in the answer boxes. Headings in this page classify the exercises into different categories: [Easy], [Intermediate], and ...
C++ Inheritance Quiz will help you to test and validate your C++ Quiz knowledge. It covers a variety of questions, from basic to advanced. The quiz contains 15 questions. You just have to assess all the given options and click on the correct answer. A Computer Science portal for geeks. It contains well written, well thought and well explained ...
People think of the world in terms of interacting objects: we'd talk about interactions between the steering wheel, the pedals, the wheels, etc. OOP allows programmers to pack away details into neat, self-contained boxes (objects) so that they can think of the objects more abstractly and focus on the interactions between them.
The is-a relationship represents. Inheritance. Study with Quizlet and memorize flashcards containing terms like From most restrictive to least restrictive, the access modifiers are:, Select the false statement regarding inheritance., Which of the following is not a kind of inheritance in C++? and more.
In C++, we have 5 types of inheritances: Single inheritance. Multilevel inheritance. Multiple inheritance. Hierarchical inheritance. Hybrid inheritance. 1. Single Inheritance. In single inheritance, a class is allowed to inherit from only one class. i.e. one base class is inherited by one derived class only.
Study with Quizlet and memorize flashcards containing terms like 1 Q1: Select the false statement regarding inheritance. a. A derived class can contain more attributes and behaviors than its base class. b. A derived class can be the base class for other derived classes. c. Some derived classes can have multiple base classes. d. Base classes are usually more specific than derived classes., 1 Q2 ...
In C++, it is possible to inherit attributes and methods from one class to another. We group the "inheritance concept" into two categories: derived class (child) - the class that inherits from another class. base class (parent) - the class being inherited from. To inherit from a class, use the : symbol.
Lecture 6: C++ Inheritance (PDF) Lab Exercises. Take a look at this example code: ... Assignment 6. rps (CPP) In the file rps.cpp, implement a class called Tool. It should have an int field called strength and a char field called type. You may make them either private or protected.
Inheritance is one of four pillars of Object-Oriented Programming (OOPs).It is a feature that enables a class to acquire properties and characteristics of another class.Inheritance allows web developers to reuse your code since the derived class or the child class can reuse the members of the base class by inheriting them. Consider a real-life example to clearly understand the concept of ...
This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more. So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
L18: C++ Inheritance I CSE333, Summer 2018 Overview of Next Two Lectures vC++ inheritance §Review of basic idea (pretty much the same as in Java) §What's different in C++ (compared to Java) •Static vs dynamic dispatch -virtual functions and vtables •Pure virtual functions, abstract classes, why no Java "interfaces" •Assignment slicing, using class hierarchies with STL
4.6: Types of Inheritance in C++; 4.7: Types of Inheritance; 4.8: Types of Inheritance; 4.9: Types of Inheritance; 4.10: Programming Assignment- Inheritance; This page titled 4: Inheritence is shared under a CC BY-SA license and was authored, remixed, and/or curated by Patrick McClanahan.
A::operator=(const A& value); will be executed. obj1 implicitly cast (static_cast) to const A&. So your obj=obj1; is equivalent to. obj = static_cast<const A&>(obj1); Obviously implicitly generated assignment will assign every non-static member of A. To deny this use private/protected inheritance. By using public inheritance you claim that all ...
C++ Hierarchical Inheritance. If more than one class is inherited from the base class, it's known as hierarchical inheritance. In hierarchical inheritance, all features that are common in child classes are included in the base class. For example, Physics, Chemistry, Biology are derived from Science class. Similarly, Dog, Cat, Horse are derived ...
Derived& operator=(const Base& a); in your Derived class. A default assignment operator, however, is created: Derived& operator=(const Derived& a); and this calls the assignment operator from Base. So it's not a matter of inheriting the assignment operator, but calling it via the default generated operator in your derived class.
11 Code Excercises on Inheritance in C# .NET. This article explains the various behaviors of inheritance in OOP in various cases. Inheritance is creating classes that inherit certain aspects from parent classes. If you are not familiar with object-oriented programming, please read A Complete Guide To Object Oriented Programming In C#.
In this way, you obtain a C object as a result of c1+c2 that may be assigned using the defined operator= in class C. For instance, add this function to the C class like the following: C& operator+(const C& other){ // for example add the two operands' characters this->a += other.a; return *this; }